.
Embed:
(wiki syntax)
Show/hide line numbers
Display.cpp
00001 #include "Display.h" 00002 extern int timer; //Stores timer mode 00003 extern int Time; //time in 10mS 00004 00005 //A B C d E F g H I J K L M N O P q r S t U V W X y Z 00006 int ImageTable[26]={0x77,0x1F,0x4E,0x3D,0x4F,0x47,0x7B,0x37,0x30,0x3C,0x37,0x0E,0x00,0x76,0x7E,0x67,0x73,0x46,0x5B,0x0F,0x3E,0x3E,0x00,0x37,0x3B,0x6D}; 00007 00008 DigitalOut CS (D2); 00009 SPI Display( PTD2,PTD3,PTD1); 00010 00011 void DisplayInit(void){ 00012 CS =1; //Disable chip select 00013 Display.format(16,3); //16-bit, mode 3 00014 Display.frequency(1000000);//1MHz clock speed 00015 SendData(0x01, ShutDown ); //Normal Operation 00016 SendData(0x0F, Intensity); //Brightness set to full 00017 SendData(0x04, ScanLimit); //Scanning digits 1-5 00018 DisplayBCD(1); //BCD decoder active on all digits 00019 } 00020 00021 void SendData(int data, int address){ 00022 00023 CS=0; //Enable input 00024 data|=(address<<8); //Data format: XXXXAAAADDDDDDDD, X=dont care. A=address. D=data 00025 Display.write(data);//Send SPI data 00026 CS=1; //Disable input 00027 00028 } 00029 00030 void DisplayClear(void){//Only use when not in BCD mode. 00031 00032 SendData(0x00,1); //Send nothing to all the digits. 00033 SendData(0x00,2); 00034 SendData(0x00,3); 00035 SendData(0x00,4); 00036 SendData(0x00,5); 00037 00038 } 00039 00040 void DisplayBCD(int state){//1 to turn on, 0 to turn off. 00041 if(state){ 00042 SendData(0xFF, BCD_Mode); 00043 }else{ 00044 SendData(0x00, BCD_Mode); 00045 } 00046 } 00047 00048 void DisplayUpdate(void){ 00049 00050 if (timer==Start){ //If timer is in start mode, increment the time 00051 Time++; 00052 }else if(timer==Reset){ //If timer is on reset mode, time = 0 00053 Time=0; 00054 } 00055 00056 if(timer!=Disable){ //Display the time on the 7-segment display, if enabled. 00057 DisplayBCD(1); 00058 SendData(DPoint| (Time/6000) , 5); 00059 SendData( ((Time%6000)/1000), 4); 00060 SendData(DPoint|((Time%1000)/100) , 1); 00061 SendData( ((Time%100 )/10) , 2); 00062 SendData( ((Time%10 ) ) , 3); 00063 } 00064 } 00065 00066 void DisplayScroll(char data[40]){//Lower case only 00067 int ScreenText[50]; //Segment data for screen 00068 int n,length; 00069 00070 for(n=0;n<50;n++){ //clear array 00071 ScreenText[n]=0; 00072 } 00073 00074 n=0; 00075 while(n<40 && (data[n]!='\0')){ //keep looping untill the end of the string, max 20 00076 if(data[n]>96 && data[n]<123){ //check for valid characters 00077 ScreenText[n+5]=ImageTable[data[n]-97]; //use a look-up table to convert ASCII to segment data 00078 } 00079 n++; 00080 } 00081 00082 timer=Disable; 00083 DisplayBCD(0); //turn off decoders 00084 00085 length=n; 00086 for(n=0;n<(length+6);n++){ //display data 00087 SendData(ScreenText[n ], 5); 00088 SendData(ScreenText[n+1], 4); 00089 SendData(ScreenText[n+2], 1); 00090 SendData(ScreenText[n+3], 2); 00091 SendData(ScreenText[n+4], 3); 00092 wait(0.3); 00093 } 00094 } 00095 00096 void DisplayNumber(char n1,char n2,char n3,char n4,char n5){ 00097 00098 timer=Disable; 00099 DisplayBCD(1); //turn off decoders 00100 00101 SendData(n1, 5); 00102 SendData(n2, 4); 00103 SendData(n3, 1); 00104 SendData(n4, 2); 00105 SendData(n5, 3); 00106 }
Generated on Fri Jul 15 2022 20:56:28 by
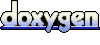