
cc3000_ntp_demo_F446RE
Dependencies: NTPClient2 NVIC_set_all_priorities cc3000_hostdriver_mbedsocket mbed
Fork of cc3000_ntp_demo by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "mbed.h" 00017 #include "cc3000.h" 00018 #include "main.h" 00019 #include "NTPClient.h" 00020 00021 #define CC3000_IRQ D3 // (D3) 00022 #define CC3000_EN D5 // (D5) 00023 #define CC3000_CS D10 // (D10) 00024 #define CC3000_MOSI D11 // (D11) 00025 #define CC3000_MISO D12 // (D12) 00026 #define CC3000_SCLK D13 // (D13) 00027 00028 #define SSID "Prakjaroen" 00029 #define PHRASE "A4B5C6D7E8F9" 00030 #define SECURITY WPA2 00031 00032 #define IP "192.168.2.165" 00033 #define MASK "255.255.255.0" 00034 #define GW "192.168.2.1" 00035 #define DHCP 1 00036 00037 using namespace mbed_cc3000; 00038 00039 #if defined TARGET_NUCLEO_F446RE 00040 //cc3000 wifi(IRQ, EN, CS, SPI(MOSI, MISO, SCLK), SSID, PHRASE, SECURITY, false); 00041 cc3000 wifi(D3, D5, D10, SPI(D11, D12, D13), "Prakjaroen", "A4B5C6D7E8F9", WPA2, false); 00042 Serial pc(USBTX, USBRX); 00043 #endif 00044 00045 /** 00046 * \brief NTP client demo 00047 * \param none 00048 * \return int 00049 */ 00050 int main() { 00051 init(); /* board dependent init */ 00052 pc.baud(230400); 00053 00054 printf("\r\n--------------------------------------------------------------------------------\r\n"); 00055 printf("cc3000 NTP client demo.\r\n"); 00056 #if (DHCP == 1) 00057 printf("Initialize the interface using DHCP...\r\n"); 00058 printf("wifi.init() "); 00059 wifi.init(); 00060 #else 00061 printf("Initialize the interface using a static IP address...\r\n"); 00062 printf("wifi.init(%s, %s, %s) ", IP, MASK, GW); 00063 wifi.init(IP, MASK, GW); 00064 #endif 00065 printf("done.\r\n"); 00066 printf("wifi.connect() "); 00067 if (wifi.connect() == -1) { 00068 printf("failed.\r\n"); 00069 printf("Failed to connect. Please verify connection details and try again. \r\n"); 00070 } else { 00071 printf("done.\r\n"); 00072 printf("IP address: %s \r\n", wifi.getIPAddress()); 00073 } 00074 00075 NTPClient ntp_client; 00076 time_t ct_time; 00077 char time_buffer[80]; 00078 char time_buffer_old[80]; 00079 wait(1); 00080 00081 strcpy(time_buffer_old, ""); 00082 00083 // Parameters 00084 char* domain_name = "0.uk.pool.ntp.org"; 00085 int port_number = 123; 00086 00087 // Read time from server 00088 printf("Reading time...\r\n"); 00089 ntp_client.setTime(domain_name, port_number); 00090 00091 // ct_time = time(NULL); 00092 // strftime(time_buffer, 80, "%a %b %d %T %p %z %Z\n", localtime(&ct_time)); 00093 // printf("UTC/GMT: %s\n", time_buffer); 00094 // printf("UTC %s\n", ctime(&ct_time)); 00095 00096 // Choose standard or daylight savings time, comment out other 00097 // ct_time= time(NULL) + 3600; // Winter time - Convert to Europe/Amsterdam Time 00098 ct_time = time(NULL) + 7200; // Summer time - Convert to Europe/Amsterdam Time 00099 set_time(ct_time); 00100 00101 // ct_time = time(NULL); 00102 strftime(time_buffer, 80, "%a %d-%b-%Y %T", localtime(&ct_time)); 00103 printf("%s\r\n", time_buffer); 00104 00105 while (1) { 00106 ct_time = time(NULL); 00107 strftime(time_buffer, 80, "%S", localtime(&ct_time)); 00108 if (strcmp(time_buffer, time_buffer_old) != 0) { 00109 strcpy(time_buffer_old, time_buffer); 00110 strftime(time_buffer, 80, "%a %d-%b-%Y %T", localtime(&ct_time)); 00111 printf("%s\r\n", time_buffer); 00112 // Sync ones a day 00113 strftime(time_buffer, 80, "%T", localtime(&ct_time)); 00114 if (strcmp(time_buffer, "00:00:00") == 0) { 00115 ntp_client.setTime(domain_name, port_number); 00116 } 00117 } 00118 } 00119 } 00120
Generated on Sun Jul 17 2022 04:53:56 by
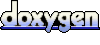