None
Fork of cc3000_hostdriver_mbedsocket by
Embed:
(wiki syntax)
Show/hide line numbers
cc3000_common.h
00001 /***************************************************************************** 00002 * 00003 * C++ interface/implementation created by Martin Kojtal (0xc0170). Thanks to 00004 * Jim Carver and Frank Vannieuwkerke for their inital cc3000 mbed port and 00005 * provided help. 00006 * 00007 * This version of "host driver" uses CC3000 Host Driver Implementation. Thus 00008 * read the following copyright: 00009 * 00010 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00011 * 00012 * Redistribution and use in source and binary forms, with or without 00013 * modification, are permitted provided that the following conditions 00014 * are met: 00015 * 00016 * Redistributions of source code must retain the above copyright 00017 * notice, this list of conditions and the following disclaimer. 00018 * 00019 * Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in the 00021 * documentation and/or other materials provided with the 00022 * distribution. 00023 * 00024 * Neither the name of Texas Instruments Incorporated nor the names of 00025 * its contributors may be used to endorse or promote products derived 00026 * from this software without specific prior written permission. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00029 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00030 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00031 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00032 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00033 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00034 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00035 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00036 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00037 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00038 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00039 * 00040 *****************************************************************************/ 00041 #ifndef CC3000_COMMON_H 00042 #define CC3000_COMMON_H 00043 00044 #include <errno.h> 00045 00046 //#define CC3000_TINY_DRIVER // Driver for small memory model CPUs 00047 00048 #define ESUCCESS 0 00049 #define EFAIL -1 00050 #define EERROR EFAIL 00051 00052 #define CC3000_UNENCRYPTED_SMART_CONFIG // No encryption 00053 00054 #define ERROR_SOCKET_INACTIVE -57 00055 00056 #define HCI_CC_PAYLOAD_LEN 5 00057 00058 #define WLAN_ENABLE (1) 00059 #define WLAN_DISABLE (0) 00060 00061 #define MAC_ADDR_LEN (6) 00062 00063 00064 /*Defines for minimal and maximal RX buffer size. This size includes the spi 00065 header and hci header. 00066 maximal buffer size: MTU + HCI header + SPI header + sendto() args size 00067 minimum buffer size: HCI header + SPI header + max args size 00068 00069 This buffer is used for receiving events and data. 00070 The packet can not be longer than MTU size and CC3000 does not support 00071 fragmentation. Note that the same buffer is used for reception of the data 00072 and events from CC3000. That is why the minimum is defined. 00073 The calculation for the actual size of buffer for reception is: 00074 Given the maximal data size MAX_DATA that is expected to be received by 00075 application, the required buffer Using recv() or recvfrom(): 00076 00077 max(CC3000_MINIMAL_RX_SIZE, MAX_DATA + HEADERS_SIZE_DATA + fromlen + ucArgsize + 1) 00078 00079 Using gethostbyname() with minimal buffer size will limit the host name returned to 99 bytes. 00080 The 1 is used for the overrun detection 00081 */ 00082 00083 #define CC3000_MINIMAL_RX_SIZE (1520 + 1) 00084 #define CC3000_MAXIMAL_RX_SIZE (1520 + 1) 00085 00086 /*Defines for minimal and maximal TX buffer size. 00087 This buffer is used for sending events and data. 00088 The packet can not be longer than MTU size and CC3000 does not support 00089 fragmentation. Note that the same buffer is used for transmission of the data 00090 and commands. That is why the minimum is defined. 00091 The calculation for the actual size of buffer for transmission is: 00092 Given the maximal data size MAX_DATA, the required buffer is: 00093 Using Sendto(): 00094 00095 max(CC3000_MINIMAL_TX_SIZE, MAX_DATA + SPI_HEADER_SIZE 00096 + SOCKET_SENDTO_PARAMS_LEN + SIMPLE_LINK_HCI_DATA_HEADER_SIZE + 1) 00097 00098 Using Send(): 00099 00100 max(CC3000_MINIMAL_TX_SIZE, MAX_DATA + SPI_HEADER_SIZE 00101 + HCI_CMND_SEND_ARG_LENGTH + SIMPLE_LINK_HCI_DATA_HEADER_SIZE + 1) 00102 00103 The 1 is used for the overrun detection */ 00104 00105 #define CC3000_MINIMAL_TX_SIZE (1520 + 1) 00106 #define CC3000_MAXIMAL_TX_SIZE (1520 + 1) 00107 00108 //TX and RX buffer size - allow to receive and transmit maximum data at lengh 8. 00109 #ifdef CC3000_TINY_DRIVER 00110 #define TINY_CC3000_MAXIMAL_RX_SIZE 44 00111 #define TINY_CC3000_MAXIMAL_TX_SIZE 59 00112 #endif 00113 00114 /*In order to determine your preferred buffer size, 00115 change CC3000_MAXIMAL_RX_SIZE and CC3000_MAXIMAL_TX_SIZE to a value between 00116 the minimal and maximal specified above. 00117 Note that the buffers are allocated by SPI. 00118 */ 00119 00120 #ifndef CC3000_TINY_DRIVER 00121 00122 #define CC3000_RX_BUFFER_SIZE (CC3000_MAXIMAL_RX_SIZE) 00123 #define CC3000_TX_BUFFER_SIZE (CC3000_MAXIMAL_TX_SIZE) 00124 #define SP_PORTION_SIZE 512 00125 00126 //TINY DRIVER: We use smaller rx and tx buffers in order to minimize RAM consumption 00127 #else 00128 #define CC3000_RX_BUFFER_SIZE (TINY_CC3000_MAXIMAL_RX_SIZE) 00129 #define CC3000_TX_BUFFER_SIZE (TINY_CC3000_MAXIMAL_TX_SIZE) 00130 #define SP_PORTION_SIZE 32 00131 #endif 00132 00133 00134 //Copy 8 bit to stream while converting to little endian format. 00135 #define UINT8_TO_STREAM(_p, _val) {*(_p)++ = (_val);} 00136 //Copy 16 bit to stream while converting to little endian format. 00137 #define UINT16_TO_STREAM(_p, _u16) (UINT16_TO_STREAM_f(_p, _u16)) 00138 //Copy 32 bit to stream while converting to little endian format. 00139 #define UINT32_TO_STREAM(_p, _u32) (UINT32_TO_STREAM_f(_p, _u32)) 00140 //Copy a specified value length bits (l) to stream while converting to little endian format. 00141 #define ARRAY_TO_STREAM(p, a, l) {uint32_t _i; for (_i = 0; _i < l; _i++) *(p)++ = ((uint8_t *) a)[_i];} 00142 //Copy received stream to 8 bit in little endian format. 00143 #define STREAM_TO_UINT8(_p, _offset, _u8) {_u8 = (uint8_t)(*(_p + _offset));} 00144 //Copy received stream to 16 bit in little endian format. 00145 #define STREAM_TO_UINT16(_p, _offset, _u16) {_u16 = STREAM_TO_UINT16_f(_p, _offset);} 00146 //Copy received stream to 32 bit in little endian format. 00147 #define STREAM_TO_UINT32(_p, _offset, _u32) {_u32 = STREAM_TO_UINT32_f(_p, _offset);} 00148 #define STREAM_TO_STREAM(p, a, l) {uint32_t _i; for (_i = 0; _i < l; _i++) *(a)++= ((uint8_t *) p)[_i];} 00149 00150 typedef struct _sockaddr_t 00151 { 00152 uint16_t family; 00153 uint8_t data[14]; 00154 } sockaddr; 00155 00156 struct timeval 00157 { 00158 int32_t tv_sec; /* seconds */ 00159 int32_t tv_usec; /* microseconds */ 00160 }; 00161 00162 #define SMART_CONFIG_PROFILE_SIZE 67 // 67 = 32 (max ssid) + 32 (max key) + 1 (SSID length) + 1 (security type) + 1 (key length) 00163 00164 /* patches type */ 00165 #define PATCHES_HOST_TYPE_WLAN_DRIVER 0x01 00166 #define PATCHES_HOST_TYPE_WLAN_FW 0x02 00167 #define PATCHES_HOST_TYPE_BOOTLOADER 0x03 00168 00169 #define SL_SET_SCAN_PARAMS_INTERVAL_LIST_SIZE (16) 00170 #define SL_SIMPLE_CONFIG_PREFIX_LENGTH (3) 00171 #define ETH_ALEN (6) 00172 #define MAXIMAL_SSID_LENGTH (32) 00173 00174 #define SL_PATCHES_REQUEST_DEFAULT (0) 00175 #define SL_PATCHES_REQUEST_FORCE_HOST (1) 00176 #define SL_PATCHES_REQUEST_FORCE_NONE (2) 00177 00178 00179 #define WLAN_SEC_UNSEC (0) 00180 #define WLAN_SEC_WEP (1) 00181 #define WLAN_SEC_WPA (2) 00182 #define WLAN_SEC_WPA2 (3) 00183 00184 00185 #define WLAN_SL_INIT_START_PARAMS_LEN (1) 00186 #define WLAN_PATCH_PARAMS_LENGTH (8) 00187 #define WLAN_SET_CONNECTION_POLICY_PARAMS_LEN (12) 00188 #define WLAN_DEL_PROFILE_PARAMS_LEN (4) 00189 #define WLAN_SET_MASK_PARAMS_LEN (4) 00190 #define WLAN_SET_SCAN_PARAMS_LEN (100) 00191 #define WLAN_GET_SCAN_RESULTS_PARAMS_LEN (4) 00192 #define WLAN_ADD_PROFILE_NOSEC_PARAM_LEN (24) 00193 #define WLAN_ADD_PROFILE_WEP_PARAM_LEN (36) 00194 #define WLAN_ADD_PROFILE_WPA_PARAM_LEN (44) 00195 #define WLAN_CONNECT_PARAM_LEN (29) 00196 #define WLAN_SMART_CONFIG_START_PARAMS_LEN (4) 00197 00198 #endif
Generated on Fri Jul 15 2022 17:19:25 by
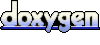