None
Fork of cc3000_hostdriver_mbedsocket by
Embed:
(wiki syntax)
Show/hide line numbers
Endpoint.cpp
00001 /* Copyright (C) 2013 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 #include "Socket/Socket.h" 00019 #include "Socket/Endpoint.h" 00020 #include "Helper/def.h" 00021 #include <cstring> 00022 00023 #include "cc3000.h" 00024 00025 /* Copied from lwip */ 00026 static char *cc3000_inet_ntoa_r(const in_addr addr, char *buf, int buflen) 00027 { 00028 uint32_t s_addr; 00029 char inv[3]; 00030 char *rp; 00031 uint8_t *ap; 00032 uint8_t rem; 00033 uint8_t n; 00034 uint8_t i; 00035 int len = 0; 00036 00037 s_addr = addr.s_addr; 00038 00039 rp = buf; 00040 ap = (uint8_t *)&s_addr; 00041 for(n = 0; n < 4; n++) { 00042 i = 0; 00043 do { 00044 rem = *ap % (uint8_t)10; 00045 *ap /= (uint8_t)10; 00046 inv[i++] = '0' + rem; 00047 } while(*ap); 00048 while(i--) { 00049 if (len++ >= buflen) { 00050 return NULL; 00051 } 00052 *rp++ = inv[i]; 00053 } 00054 if (len++ >= buflen) { 00055 return NULL; 00056 } 00057 *rp++ = '.'; 00058 ap++; 00059 } 00060 *--rp = 0; 00061 return buf; 00062 } 00063 00064 Endpoint::Endpoint() { 00065 _cc3000_module = cc3000::get_instance(); 00066 if (_cc3000_module == NULL) { 00067 error("Endpoint constructor error: no cc3000 instance available!\r\n"); 00068 } 00069 reset_address(); 00070 } 00071 Endpoint::~Endpoint() {} 00072 00073 void Endpoint::reset_address(void) { 00074 _ipAddress[0] = '\0'; 00075 std::memset(&_remote_host, 0, sizeof(sockaddr_in)); 00076 } 00077 00078 int Endpoint::set_address(const char* host, const int port) { 00079 reset_address(); 00080 00081 char address[5]; 00082 char *p_address = address; 00083 00084 signed int add[5]; 00085 00086 // Dot-decimal notation 00087 int result = std::sscanf(host, "%3u.%3u.%3u.%3u", &add[0], &add[1], &add[2], &add[3]); 00088 for (int i=0;i<4;i++) { 00089 address[i] = add[i]; 00090 } 00091 std::memset(_ipAddress,0,sizeof(_ipAddress)); 00092 00093 if (result != 4) { 00094 #ifndef CC3000_TINY_DRIVER 00095 //Resolve DNS address or populate hard-coded IP address 00096 uint32_t address_integer; 00097 int resolveRetCode; 00098 resolveRetCode = _cc3000_module->_socket.gethostbyname((uint8_t *)host, strlen(host) , &address_integer); 00099 00100 if ((resolveRetCode > -1) && (0 != address_integer)) { 00101 _remote_host.sin_addr.s_addr = htonl(address_integer); 00102 cc3000_inet_ntoa_r(_remote_host.sin_addr, _ipAddress, sizeof(_ipAddress)); 00103 } else { 00104 // Failed to resolve the address 00105 DBG_SOCKET("Failed to resolve the hostname : %s",host); 00106 return (-1); 00107 } 00108 #else 00109 return -1; 00110 #endif 00111 } else { 00112 std::memcpy((char*)&_remote_host.sin_addr.s_addr, p_address, 4); 00113 } 00114 00115 _remote_host.sin_family = AF_INET; 00116 _remote_host.sin_port = htons(port); 00117 00118 DBG_SOCKET("remote host address (string): %s",get_address()); 00119 DBG_SOCKET("remote host address from s_addr : %d.%d.%d.%d", 00120 int(_remote_host.sin_addr.s_addr & 0xFF), 00121 int((_remote_host.sin_addr.s_addr & 0xFF00) >> 8), 00122 int((_remote_host.sin_addr.s_addr & 0xFF0000) >> 16), 00123 int((_remote_host.sin_addr.s_addr & 0xFF000000) >> 24)); 00124 DBG_SOCKET("port: %d", port); 00125 00126 return 0; 00127 } 00128 00129 char* Endpoint::get_address() { 00130 if ((_ipAddress[0] == '\0') && (_remote_host.sin_addr.s_addr != 0)) 00131 cc3000_inet_ntoa_r(_remote_host.sin_addr, _ipAddress, sizeof(_ipAddress)); 00132 return _ipAddress; 00133 } 00134 00135 00136 int Endpoint::get_port() { 00137 return ntohs(_remote_host.sin_port); 00138 }
Generated on Fri Jul 15 2022 17:19:25 by
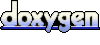