Uses the same fonts as the SPI_TFT_ILI9341 Library (I have many, and a html/php font editor for that)
Embed:
(wiki syntax)
Show/hide line numbers
SPI_TFT_ILI9225.h
00001 #ifndef TFT_22_ILI9225_h 00002 #define TFT_22_ILI9225_h 00003 #include "mbed.h" 00004 00005 #define FONT_LENGTH 0 00006 #define FONT_HORZ 1 00007 #define FONT_VERT 2 00008 #define FONT_BYTES_VERT 3 00009 00010 #define ILI9225_CHARS_PER_LINE 36 00011 00012 /* ILI9225 screen size */ 00013 #define ILI9225_LCD_WIDTH 176 00014 #define ILI9225_LCD_HEIGHT 220 00015 00016 #define ILI9225_PORTRAIT_L 0 // SD card at the left side 00017 #define ILI9225_LANDSCAPE_B 1 // SD card at the bottom side 00018 #define ILI9225_PORTRAIT_R 2 // SD card at the right side 00019 #define ILI9225_LANDSCAPE_T 3 // SD card at the top side 00020 00021 /* ILI9225 LCD Registers */ 00022 #define ILI9225_DRIVER_OUTPUT_CTRL (0x01u) // Driver Output Control 00023 #define ILI9225_LCD_AC_DRIVING_CTRL (0x02u) // LCD AC Driving Control 00024 #define ILI9225_ENTRY_MODE (0x03u) // Entry Mode 00025 #define ILI9225_DISP_CTRL1 (0x07u) // Display Control 1 00026 #define ILI9225_BLANK_PERIOD_CTRL1 (0x08u) // Blank Period Control 00027 #define ILI9225_FRAME_CYCLE_CTRL (0x0Bu) // Frame Cycle Control 00028 #define ILI9225_INTERFACE_CTRL (0x0Cu) // Interface Control 00029 #define ILI9225_OSC_CTRL (0x0Fu) // Osc Control 00030 #define ILI9225_POWER_CTRL1 (0x10u) // Power Control 1 00031 #define ILI9225_POWER_CTRL2 (0x11u) // Power Control 2 00032 #define ILI9225_POWER_CTRL3 (0x12u) // Power Control 3 00033 #define ILI9225_POWER_CTRL4 (0x13u) // Power Control 4 00034 #define ILI9225_POWER_CTRL5 (0x14u) // Power Control 5 00035 #define ILI9225_VCI_RECYCLING (0x15u) // VCI Recycling 00036 #define ILI9225_RAM_ADDR_SET1 (0x20u) // Horizontal GRAM Address Set 00037 #define ILI9225_RAM_ADDR_SET2 (0x21u) // Vertical GRAM Address Set 00038 #define ILI9225_GRAM_DATA_REG (0x22u) // GRAM Data Register 00039 #define ILI9225_GATE_SCAN_CTRL (0x30u) // Gate Scan Control Register 00040 #define ILI9225_VERTICAL_SCROLL_CTRL1 (0x31u) // Vertical Scroll Control 1 Register 00041 #define ILI9225_VERTICAL_SCROLL_CTRL2 (0x32u) // Vertical Scroll Control 2 Register 00042 #define ILI9225_VERTICAL_SCROLL_CTRL3 (0x33u) // Vertical Scroll Control 3 Register 00043 #define ILI9225_PARTIAL_DRIVING_POS1 (0x34u) // Partial Driving Position 1 Register 00044 #define ILI9225_PARTIAL_DRIVING_POS2 (0x35u) // Partial Driving Position 2 Register 00045 #define ILI9225_HORIZONTAL_WINDOW_ADDR1 (0x36u) // Horizontal Address Start Position 00046 #define ILI9225_HORIZONTAL_WINDOW_ADDR2 (0x37u) // Horizontal Address End Position 00047 #define ILI9225_VERTICAL_WINDOW_ADDR1 (0x38u) // Vertical Address Start Position 00048 #define ILI9225_VERTICAL_WINDOW_ADDR2 (0x39u) // Vertical Address End Position 00049 #define ILI9225_GAMMA_CTRL1 (0x50u) // Gamma Control 1 00050 #define ILI9225_GAMMA_CTRL2 (0x51u) // Gamma Control 2 00051 #define ILI9225_GAMMA_CTRL3 (0x52u) // Gamma Control 3 00052 #define ILI9225_GAMMA_CTRL4 (0x53u) // Gamma Control 4 00053 #define ILI9225_GAMMA_CTRL5 (0x54u) // Gamma Control 5 00054 #define ILI9225_GAMMA_CTRL6 (0x55u) // Gamma Control 6 00055 #define ILI9225_GAMMA_CTRL7 (0x56u) // Gamma Control 7 00056 #define ILI9225_GAMMA_CTRL8 (0x57u) // Gamma Control 8 00057 #define ILI9225_GAMMA_CTRL9 (0x58u) // Gamma Control 9 00058 #define ILI9225_GAMMA_CTRL10 (0x59u) // Gamma Control 10 00059 00060 #define ILI9225C_INVOFF 0x20 00061 #define ILI9225C_INVON 0x21 00062 00063 00064 /* RGB 24-bits color table definition (RGB888). */ 00065 #define RGB888_RGB565(color) ((((color) >> 19) & 0x1f) << 11) | ((((color) >> 10) & 0x3f) << 5) | (((color) >> 3) & 0x1f) 00066 00067 #define COLOR_BLACK RGB888_RGB565(0x000000u) 00068 #define COLOR_WHITE RGB888_RGB565(0xFFFFFFu) 00069 #define COLOR_BLUE RGB888_RGB565(0x0000FFu) 00070 #define COLOR_GREEN RGB888_RGB565(0x00FF00u) 00071 #define COLOR_RED RGB888_RGB565(0xFF0000u) 00072 #define COLOR_NAVY RGB888_RGB565(0x000080u) 00073 #define COLOR_DARKBLUE RGB888_RGB565(0x00008Bu) 00074 #define COLOR_DARKGREEN RGB888_RGB565(0x006400u) 00075 #define COLOR_DARKCYAN RGB888_RGB565(0x008B8Bu) 00076 #define COLOR_CYAN RGB888_RGB565(0x00FFFFu) 00077 #define COLOR_TURQUOISE RGB888_RGB565(0x40E0D0u) 00078 #define COLOR_INDIGO RGB888_RGB565(0x4B0082u) 00079 #define COLOR_DARKRED RGB888_RGB565(0x800000u) 00080 #define COLOR_OLIVE RGB888_RGB565(0x808000u) 00081 #define COLOR_GRAY RGB888_RGB565(0x808080u) 00082 #define COLOR_SKYBLUE RGB888_RGB565(0x87CEEBu) 00083 #define COLOR_BLUEVIOLET RGB888_RGB565(0x8A2BE2u) 00084 #define COLOR_LIGHTGREEN RGB888_RGB565(0x90EE90u) 00085 #define COLOR_DARKVIOLET RGB888_RGB565(0x9400D3u) 00086 #define COLOR_YELLOWGREEN RGB888_RGB565(0x9ACD32u) 00087 #define COLOR_BROWN RGB888_RGB565(0xA52A2Au) 00088 #define COLOR_DARKGRAY RGB888_RGB565(0xA9A9A9u) 00089 #define COLOR_SIENNA RGB888_RGB565(0xA0522Du) 00090 #define COLOR_LIGHTBLUE RGB888_RGB565(0xADD8E6u) 00091 #define COLOR_GREENYELLOW RGB888_RGB565(0xADFF2Fu) 00092 #define COLOR_SILVER RGB888_RGB565(0xC0C0C0u) 00093 #define COLOR_LIGHTGREY RGB888_RGB565(0xD3D3D3u) 00094 #define COLOR_LIGHTCYAN RGB888_RGB565(0xE0FFFFu) 00095 #define COLOR_VIOLET RGB888_RGB565(0xEE82EEu) 00096 #define COLOR_AZUR RGB888_RGB565(0xF0FFFFu) 00097 #define COLOR_BEIGE RGB888_RGB565(0xF5F5DCu) 00098 #define COLOR_MAGENTA RGB888_RGB565(0xFF00FFu) 00099 #define COLOR_TOMATO RGB888_RGB565(0xFF6347u) 00100 #define COLOR_GOLD RGB888_RGB565(0xFFD700u) 00101 #define COLOR_ORANGE RGB888_RGB565(0xFFA500u) 00102 #define COLOR_SNOW RGB888_RGB565(0xFFFAFAu) 00103 #define COLOR_YELLOW RGB888_RGB565(0xFFFF00u) 00104 00105 /// Main and core class 00106 class TFT_22_ILI9225 : public Stream 00107 { 00108 00109 public: 00110 00111 /** Constructor 00112 * 00113 */ 00114 TFT_22_ILI9225(PinName sdi, PinName clk, PinName cs, PinName rs, PinName rst, PinName led, const char *name); 00115 00116 /** Initialization 00117 * 00118 */ 00119 void init(void); 00120 00121 /** Clear the screen 00122 * 00123 */ 00124 void cls(void); 00125 00126 /** Fill the screen with color 00127 * 00128 * @param color 00129 * 00130 */ 00131 void fill(uint16_t color); 00132 00133 /** Invert screen 00134 * 00135 * @param flag true to invert, false for normal screen 00136 * 00137 */ 00138 void invert(bool flag); 00139 00140 /** Set backlight brightness 00141 * 00142 * @param brightness 00143 * 00144 */ 00145 void setBacklight(double brightness); 00146 00147 /** Switch backlight off 00148 * 00149 */ 00150 void setBacklightOff(void); 00151 00152 /** Switch backlight on 00153 * 00154 */ 00155 void setBacklightOn(void); 00156 00157 /** Switch display on or off 00158 * 00159 * @param flag true=on, false=off 00160 * 00161 */ 00162 void setDisplay(bool flag); 00163 00164 /** Set orientation 00165 * 00166 * @param orientation orientation: 00167 * 0=ILI9225_PORTRAIT_L : SD card at the left side 00168 * 1=ILI9225_LANDSCAPE_B : SD card at the bottom side 00169 * 2=ILI9225_PORTRAIT_R : SD card at the right side 00170 * 3=ILI9225_LANDSCAPE_T : SD card at the top side 00171 * 00172 */ 00173 void setOrientation(uint8_t orientation); 00174 00175 /** Get orientation 00176 * 00177 * @return orientation orientation, 0=portrait, 1=right rotated landscape, 2=reverse portrait, 3=left rotated landscape 00178 * 00179 */ 00180 uint8_t getOrientation(void); 00181 00182 00183 // Graphics functions 00184 00185 /** Draw pixel 00186 * 00187 * @param x1 point coordinate, x-axis 00188 * @param y1 point coordinate, y-axis 00189 * @param color 16-bit color 00190 * 00191 */ 00192 void pixel(uint16_t x1, uint16_t y1, uint16_t color); 00193 00194 /** Draw line, rectangle coordinates 00195 * 00196 * @param x1 top left coordinate, x-axis 00197 * @param y1 top left coordinate, y-axis 00198 * @param x2 bottom right coordinate, x-axis 00199 * @param y2 bottom right coordinate, y-axis 00200 * @param color 16-bit color 00201 * 00202 */ 00203 void line(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, uint16_t color); 00204 00205 /** Draw hline, rectangle coordinates 00206 * 00207 * @param x1 top left coordinate, x-axis 00208 * @param x2 bottom right coordinate, x-axis 00209 * @param y bottom right coordinate, y-axis 00210 * @param color 16-bit color 00211 * 00212 */ 00213 void hline(uint16_t x1, uint16_t x2, uint16_t y, uint16_t color); 00214 00215 /** Draw vline, rectangle coordinates 00216 * 00217 * @param x top left coordinate, x-axis 00218 * @param y1 top left coordinate, y-axis 00219 * @param y2 bottom right coordinate, y-axis 00220 * @param color 16-bit color 00221 * 00222 */ 00223 void vline(uint16_t x, uint16_t y1, uint16_t y2, uint16_t color); 00224 00225 /** Draw rectangle, rectangle coordinates 00226 * 00227 * @param x1 top left coordinate, x-axis 00228 * @param y1 top left coordinate, y-axis 00229 * @param x2 bottom right coordinate, x-axis 00230 * @param y2 bottom right coordinate, y-axis 00231 * @param color 16-bit color 00232 * 00233 */ 00234 void rect(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, uint16_t color); 00235 00236 /** Draw solid rectangle, rectangle coordinates 00237 * 00238 * @param x1 top left coordinate, x-axis 00239 * @param y1 top left coordinate, y-axis 00240 * @param x2 bottom right coordinate, x-axis 00241 * @param y2 bottom right coordinate, y-axis 00242 * @param color 16-bit color 00243 * 00244 */ 00245 void fillrect(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, uint16_t color); 00246 00247 /** Draw circle 00248 * 00249 * @param x0 center, point coordinate, x-axis 00250 * @param y0 center, point coordinate, y-axis 00251 * @param radius radius 00252 * @param color 16-bit color 00253 * 00254 */ 00255 void circle(uint16_t x0, uint16_t y0, uint16_t radius, uint16_t color); 00256 00257 /** Draw solid circle 00258 * 00259 * @param x0 center, point coordinate, x-axis 00260 * @param y0 center, point coordinate, y-axis 00261 * @param radius radius 00262 * @param color 16-bit color 00263 * 00264 */ 00265 void fillcircle(uint8_t x0, uint8_t y0, uint8_t radius, uint16_t color); 00266 00267 /** Draw triangle, triangle coordinates 00268 * 00269 * @param x1 corner 1 coordinate, x-axis 00270 * @param y1 corner 1 coordinate, y-axis 00271 * @param x2 corner 2 coordinate, x-axis 00272 * @param y2 corner 2 coordinate, y-axis 00273 * @param x3 corner 3 coordinate, x-axis 00274 * @param y3 corner 3 coordinate, y-axis 00275 * @param color 16-bit color 00276 * 00277 */ 00278 void triangle(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, uint16_t x3, uint16_t y3, uint16_t color); 00279 00280 /** Draw solid triangle, triangle coordinates 00281 * 00282 * @param x1 corner 1 coordinate, x-axis 00283 * @param y1 corner 1 coordinate, y-axis 00284 * @param x2 corner 2 coordinate, x-axis 00285 * @param y2 corner 2 coordinate, y-axis 00286 * @param x3 corner 3 coordinate, x-axis 00287 * @param y3 corner 3 coordinate, y-axis 00288 * @param color 16-bit color 00289 * 00290 */ 00291 void filltriangle(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, uint16_t x3, uint16_t y3, uint16_t color); 00292 00293 /** Draw line/filled rectangle, rectangle coordinates 00294 * 00295 * @param x1 top left coordinate, x-axis 00296 * @param y1 top left coordinate, y-axis 00297 * @param x2 bottom right coordinate, x-axis 00298 * @param y2 bottom right coordinate, y-axis 00299 * @param rad defines the redius of the circle 00300 * @param fill fill yes or no 00301 * @param color 16-bit color 00302 * 00303 */ 00304 void roundrect(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, uint16_t rad, bool fill, uint16_t color); 00305 00306 /** Screen size, x-axis 00307 * 00308 * @return horizontal size of the screen, in pixels 00309 * @note 219 means 0..219 coordinates (decimal) 00310 * 00311 */ 00312 uint16_t maxX(void); 00313 00314 /** Screen size, y-axis 00315 * 00316 * @return vertical size of the screen, in pixels 00317 * @note 175 means 0..175 coordinates (decimal) 00318 * 00319 */ 00320 uint16_t maxY(void); 00321 00322 /** Screen size, x-axis 00323 * 00324 * @return horizontal size of the screen, in pixels 00325 * @note 220 means 220 pixels and thus 0..219 coordinates (decimal) 00326 * 00327 */ 00328 uint16_t width(void); 00329 00330 /** Screen size, y-axis 00331 * 00332 * @return vertical size of the screen, in pixels 00333 * @note 176 means 176 pixels and thus 0..175 coordinates (decimal) 00334 * 00335 */ 00336 uint16_t height(void); 00337 00338 /** Calculate 16-bit color from 8-bit Red-Green-Blue components 00339 * 00340 * @param red red component, 0x00..0xff 00341 * @param green green component, 0x00..0xff 00342 * @param blue blue component, 0x00..0xff 00343 * @return 16-bit color 00344 * 00345 */ 00346 uint16_t setColor(uint8_t red, uint8_t green, uint8_t blue); 00347 00348 /** Calculate 8-bit Red-Green-Blue components from 16-bit color 00349 * 00350 * @param rgb 16-bit color 00351 * @param red red component, 0x00..0xff 00352 * @param green green component, 0x00..0xff 00353 * @param blue blue component, 0x00..0xff 00354 * 00355 */ 00356 void splitColor(uint16_t rgb, uint8_t &red, uint8_t &green, uint8_t &blue); 00357 00358 00359 // Text functions 00360 00361 /** Set current font 00362 * 00363 * @param font Font name 00364 * 00365 */ 00366 void setFont(uint8_t* font); 00367 00368 /** Font size, x-axis 00369 * 00370 * @return horizontal size of current font, in pixels 00371 * 00372 */ 00373 uint8_t fontX(void); 00374 00375 /** Font size, y-axis 00376 * 00377 * @return vertical size of current font, in pixels 00378 * 00379 */ 00380 uint8_t fontY(void); 00381 00382 /** put a char on the screen 00383 * 00384 * @param value char to print 00385 * @returns printed char 00386 * 00387 */ 00388 virtual int putc(int value); 00389 00390 /** draw a character on given position out of the active font to the TFT 00391 * 00392 * @param x x-position of char (top left) 00393 * @param y y-position 00394 * @param c char to print 00395 * 00396 */ 00397 void character(int x, int y, int c); 00398 00399 /** get string width 00400 * 00401 * @param string 00402 * @return string width in pixels 00403 * 00404 */ 00405 uint16_t getStringWidth(char * s); 00406 00407 /** Set text foreground color 00408 * 00409 * @param color 00410 * 00411 */ 00412 void foreground(uint16_t color); 00413 00414 /** Set text background color 00415 * 00416 * @param color 00417 * 00418 */ 00419 void background(uint16_t color); 00420 00421 /** Set grapical cursor position 00422 * 00423 * @param x 00424 * @param y 00425 * 00426 */ 00427 void locate(int x, int y); 00428 00429 /** Set text cursor position 00430 * 00431 * @param x 00432 * @param y 00433 * 00434 */ 00435 void gotoxy(int x, int y); 00436 00437 /** Set text cursor at 0,0 00438 * 00439 */ 00440 void home(void); 00441 00442 /** Set spacing in pixels between text lines 00443 * 00444 * @param line_spacing spacing in pixels between text lines 00445 * 00446 */ 00447 void linespacing(int line_spacing); 00448 00449 /** calculate the max number of char in a line 00450 * 00451 * @returns max columns 00452 * depends on actual font size 00453 * 00454 */ 00455 virtual int columns(void); 00456 00457 /** calculate the max number of columns 00458 * 00459 * @returns max column 00460 * depends on actual font size 00461 * 00462 */ 00463 virtual int rows(void); 00464 00465 /** Make an ascii string from an unicode string 00466 * 00467 * @param uni_str 00468 * @param ascii_str 00469 * 00470 */ 00471 void unicode2ascii(char *uni_str, char *ascii_str); 00472 00473 /** Claim standard output 00474 * 00475 */ 00476 virtual bool claim (FILE *stream); 00477 00478 00479 private: 00480 00481 SPI _spi; 00482 DigitalOut _rst; 00483 DigitalOut _rs; 00484 DigitalOut _cs; 00485 PwmOut _led; 00486 00487 float _brightness; 00488 uint16_t _entryMode; 00489 uint16_t _maxX, _maxY; 00490 uint8_t _orientation; 00491 00492 // for claim 00493 uint16_t _column; 00494 uint16_t _row; 00495 char *_path; 00496 00497 unsigned char* font; 00498 unsigned int char_x; 00499 unsigned int char_y; 00500 unsigned int char_line_spacing; 00501 00502 // colors 00503 uint16_t _foreground; 00504 uint16_t _background; 00505 00506 00507 // Private functions 00508 00509 /** Swap two values 00510 * 00511 * @param a b 00512 * @param a b 00513 * 00514 */ 00515 void _swap(uint16_t &a, uint16_t &b); 00516 00517 /** Set window 00518 * 00519 * @param x0 00520 * @param y0 00521 * @param x1 00522 * @param y1 00523 * 00524 */ 00525 void _setWindow(uint16_t x0, uint16_t y0, uint16_t x1, uint16_t y1); 00526 00527 /** Maximize window to entire screen 00528 * 00529 */ 00530 void _setWindowMax(void); 00531 00532 /** Set coordinates 00533 * 00534 * @param x 00535 * @param y 00536 * 00537 */ 00538 void _orientCoordinates(uint16_t &x, uint16_t &y); 00539 00540 /** Write register 00541 * 00542 * @param uint16_t register 00543 * @param uint16_t data 00544 * 00545 */ 00546 void _writeRegister(uint16_t reg, uint16_t data); 00547 00548 /** Write a command 00549 * 00550 * @param HI 00551 * @param LO 00552 * 00553 */ 00554 void _writeCommand(uint8_t HI, uint8_t LO); 00555 00556 /** Start writing data 00557 * 00558 */ 00559 void _startData(void); 00560 00561 /** Write data 00562 * 00563 * @param uint16_t data 00564 * 00565 */ 00566 void _writeData(uint16_t data); 00567 00568 /** End writing data 00569 * 00570 */ 00571 void _endData(void); 00572 00573 00574 protected: 00575 00576 //used by printf - supply a new _putc virtual function for the new device 00577 virtual int _putc(int c) { 00578 putc(c); //your new LCD put to print an ASCII character on LCD 00579 return 0; 00580 }; 00581 //assuming no reads from LCD 00582 virtual int _getc() { 00583 return -1; 00584 } 00585 00586 }; 00587 00588 #endif
Generated on Sun Jul 17 2022 16:36:39 by
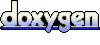