x
Embed:
(wiki syntax)
Show/hide line numbers
PCA9745B.h
00001 #ifndef PCA9745B_H 00002 #define PCA9745B_H 00003 00004 /** 00005 * SPI speed used by the mbed to communicate with the PCA9745B 00006 * The PCA9745B supports up to 10Mhz. 00007 */ 00008 #define SPI_SPEED 1000000 00009 00010 /** 00011 * Using the TLC5 class to control an LED: 00012 * @code 00013 * #include "mbed.h" 00014 * #include "PCA9745B.h" 00015 * 00016 * // Create the TLC5711 instance 00017 * PCA9745B tlc(1, p7, p5); 00018 * 00019 * int main() 00020 * { 00021 * 00022 * while(1) 00023 * { 00024 * // Led1 -> R0 00025 * tlc.setLED(0, 65535, 0, 0); 00026 * tlc.write( ); 00027 * tlc.setLED(1, 0, 0, 0); 00028 * tlc.write( ); 00029 * tlc.setLED(2, 0, 0, 0); 00030 * tlc.write( ); 00031 * tlc.setLED(3, 0, 0, 0); 00032 * tlc.write( ); 00033 * wait( 1 ); 00034 * 00035 * } 00036 * } 00037 * @endcode 00038 */ 00039 00040 class PCA9745B 00041 { 00042 00043 public:/** 00044 * Set up the PCA9745B 00045 * @param SCLK - The SCK pin of the SPI bus 00046 * @param MOSI - The MOSI pin of the SPI bus 00047 * @param number - The number of PCA9745Bs 00048 */ 00049 00050 PCA9745B(uint8_t number, PinName SCLK, PinName MOSI); 00051 00052 void setPWM(uint8_t chan, uint16_t pwm); 00053 void setLED(uint8_t lednum, uint16_t r, uint16_t g, uint16_t b); 00054 void write(void); 00055 00056 uint8_t n; 00057 00058 private: 00059 00060 SPI spi; 00061 00062 uint16_t *pwmbuffer; 00063 00064 uint8_t BCr, BCg, BCb; 00065 int8_t numdrivers; 00066 00067 }; 00068 00069 #endif
Generated on Thu Jul 14 2022 02:17:11 by
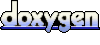