FT6206 Library for Adafruit 2.8" TFT Touch Shield for Arduino w/Capacitive Touch
Dependents: ArchPro_TFT ATT_AWS_IoT_demo_v06 ArchPro_TFT TermProject
FT6206.cpp
00001 /* 00002 Copyright (c) 2015 Jack Berkhout 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 00022 This is a library for the Adafruit Capacitive Touch Screens 00023 ----> http://www.adafruit.com/products/1947 00024 This chipset uses I2C to communicate 00025 00026 Based on code by Limor Fried/Ladyada for Adafruit Industries. 00027 MIT license, all text above must be included in any redistribution. 00028 00029 On the shield, these jumpers were bridged: 00030 Int - #7 (default) 00031 SCK - 13 (default) 00032 SO - 12 (default) 00033 SI - 11 00034 SCL - SCL 00035 SDA - SDA 00036 00037 Usage: 00038 00039 #include "mbed.h" 00040 #include "SPI_TFT_ILI9341.h" 00041 #include "FT6206.h" 00042 #include "Arial12x12.h" 00043 00044 #define PIN_XP A3 00045 #define PIN_XM A1 00046 #define PIN_YP A2 00047 #define PIN_YM A0 00048 #define PIN_SCLK D13 00049 #define PIN_MISO D12 00050 #define PIN_MOSI D11 00051 #define PIN_CS_TFT D10 // chip select pin 00052 #define PIN_DC_TFT D9 // data/command select pin. 00053 #define PIN_RESET_TFT D8 00054 //#define PIN_BL_TFT D7 00055 #define PIN_CS_SD D4 00056 00057 #define PORTRAIT 0 00058 #define LANDSCAPE 1 00059 00060 #define PIN_SCL_FT6206 P0_28 00061 #define PIN_SDA_FT6206 P0_27 00062 #define PIN_INT_FT6206 D7 00063 00064 SPI_TFT_ILI9341 TFT(PIN_MOSI, PIN_MISO, PIN_SCLK, PIN_CS_TFT, PIN_RESET_TFT, PIN_DC_TFT, "TFT"); // mosi, miso, sclk, cs, reset, dc 00065 FT6206 FT6206(PIN_SDA_FT6206, PIN_SCL_FT6206, PIN_INT_FT6206); // sda, scl, int 00066 00067 int main() 00068 { 00069 //Configure the display driver 00070 TFT.claim(stdout); 00071 TFT.background(Black); 00072 TFT.foreground(White); 00073 TFT.set_orientation(LANDSCAPE); 00074 TFT.cls(); 00075 00076 //Print a welcome message 00077 TFT.set_font((unsigned char*) Arial12x12); 00078 TFT.locate(0,0); 00079 TFT.printf("Hello mbed!\n"); 00080 00081 while(1) { 00082 int X1, Y1, X2, Y2; 00083 TS_Point p; 00084 if (FT6206.getTouchPoint(p)) { 00085 X1 = X2; 00086 Y1 = Y2; 00087 X2 = p.x; 00088 Y2 = p.y; 00089 TFT.locate(0,12); 00090 printf("Touch %3d %3d\n", p.x, p.y); 00091 if ((X1 > 0) && (Y1 > 0) && (X2 > 0) && (Y2 > 0)) { 00092 TFT.line(X1, Y1, X2, Y2, RGB(255,128,255)); 00093 } 00094 } 00095 } 00096 } 00097 */ 00098 00099 #include "FT6206.h" 00100 00101 FT6206::FT6206(PinName sda, PinName scl, PinName interrupt) : m_i2c(sda, scl), m_interrupt(interrupt) 00102 { 00103 m_addr = (FT6206_ADDR << 1); 00104 m_i2c.frequency(FT6206_I2C_FREQUENCY); 00105 00106 m_interrupt.mode(PullUp); 00107 m_interrupt.enable_irq(); 00108 m_interrupt.fall(this, &FT6206::checkDataReceived); 00109 00110 init(); 00111 00112 // tick.attach(this, &FT6206::checkDataReceived, 0.00001); // Every 10 us id data was received 00113 00114 } 00115 00116 FT6206::~FT6206() 00117 { 00118 00119 } 00120 00121 /**************************************************************************/ 00122 /*! 00123 @brief Setups the HW 00124 */ 00125 /**************************************************************************/ 00126 bool FT6206::init(uint8_t threshhold) { 00127 // change threshhold to be higher/lower 00128 writeRegister8(FT6206_REG_THRESHHOLD, FT6206_DEFAULT_THRESSHOLD); 00129 // writeRegister8(FT6206_REG_POINTRATE, 80); 00130 00131 if (readRegister8(FT6206_REG_VENDID) != 17) 00132 return false; 00133 00134 if (readRegister8(FT6206_REG_CHIPID) != 6) 00135 return false; 00136 00137 // printf("Vend ID: %d\n", readRegister8(FT6206_REG_VENDID)); 00138 // printf("Chip ID: %d\n", readRegister8(FT6206_REG_CHIPID)); 00139 // printf("Firm V: %d\n", readRegister8(FT6206_REG_FIRMVERS)); 00140 // printf("Rate Hz: %d\n", readRegister8(FT6206_REG_POINTRATE)); 00141 // printf("Thresh: %d\n", readRegister8(FT6206_REG_THRESHHOLD)); 00142 00143 // dump all registers 00144 /* 00145 for (int16_t i=0; i<0x20; i++) { 00146 printf("I2C $%02x = 0x %02x\n", i, readRegister8(i)); 00147 } 00148 */ 00149 return true; 00150 } 00151 00152 bool FT6206::touched(void) { 00153 uint8_t n = readRegister8(FT6206_REG_NUMTOUCHES); 00154 if ((n == 1) || (n == 2)) 00155 return true; 00156 return false; 00157 } 00158 00159 void FT6206::checkDataReceived(void) { 00160 // InterruptIn pin used 00161 // // Runs every 0.00001 Sec. in Ticker 00162 // if (dataReceived()) { 00163 DataReceived = true; 00164 // } 00165 } 00166 00167 bool FT6206::getDataReceived(void) { 00168 bool _DataReceived = DataReceived; 00169 DataReceived = false; 00170 return _DataReceived; 00171 } 00172 00173 void FT6206::waitScreenTapped(void) { 00174 DataReceived = false; 00175 while (!getDataReceived()) { 00176 wait(0.01); 00177 } 00178 } 00179 00180 bool FT6206::getTouchPoint(TS_Point &p) { 00181 // if (touched()) { 00182 // if (dataReceived()) { 00183 if (getDataReceived()) { 00184 // Retrieve a point 00185 p = getPoint(); 00186 return true; 00187 } 00188 p = clearPoint(); 00189 return false; 00190 } 00191 00192 /*****************************/ 00193 00194 void FT6206::readData(uint16_t *x, uint16_t *y) { 00195 char i2cdat[16]; 00196 00197 m_i2c.write(m_addr, 0x00, 1); 00198 m_i2c.read(m_addr, i2cdat, 16); 00199 00200 /* 00201 for (int16_t i=0; i<0x20; i++) { 00202 printf("I2C %02x = %02x\n", i, i2cdat[i]);; 00203 } 00204 */ 00205 00206 touches = i2cdat[0x02]; 00207 00208 // printf("touches: %d\n", touches); 00209 if (touches > 2) { 00210 touches = 0; 00211 *x = *y = 0; 00212 } 00213 if (touches == 0) { 00214 *x = *y = 0; 00215 return; 00216 } 00217 00218 /* 00219 if (touches == 2) Serial.print('2'); 00220 for (uint8_t i=0; i<16; i++) { 00221 printf("%02x ", i2cdat[i]); 00222 } 00223 printf("\n"); 00224 */ 00225 00226 /* 00227 printf("\n"); 00228 if (i2cdat[0x01] != 0x00) { 00229 printf("Gesture #%d\n", i2cdat[0x01]); 00230 } 00231 */ 00232 00233 //printf("# Touches: %d", touches); 00234 for (uint8_t i=0; i<2; i++) { 00235 touchY[i] = i2cdat[0x03 + i*6] & 0x0F; 00236 touchY[i] <<= 8; 00237 touchY[i] |= i2cdat[0x04 + i*6]; 00238 touchX[i] = i2cdat[0x05 + i*6] & 0x0F; 00239 touchX[i] <<= 8; 00240 touchX[i] |= i2cdat[0x06 + i*6]; 00241 touchID[i] = i2cdat[0x05 + i*6] >> 4; 00242 } 00243 /* 00244 for (uint8_t i=0; i<touches; i++) { 00245 printf("ID #%d (%d, %d", touchID[i], touchX[i], touchY[i]); 00246 printf(")\n"); 00247 } 00248 */ 00249 *x = ILI9341_TFTWIDTH - touchX[0]; 00250 *y = touchY[0]; 00251 } 00252 00253 TS_Point FT6206::getPoint(void) { 00254 uint16_t x, y; 00255 readData(&x, &y); 00256 return TS_Point(x, y, 1); 00257 } 00258 00259 TS_Point FT6206::clearPoint(void) { 00260 uint16_t x = 0, y = 0; 00261 return TS_Point(x, y, 1); 00262 } 00263 00264 char FT6206::readRegister8(char reg) { 00265 char val; 00266 m_i2c.write(m_addr, ®, 1); 00267 m_i2c.read(m_addr, &val, 1); 00268 return val; 00269 } 00270 00271 void FT6206::writeRegister8(char reg, char val) { 00272 char data[2]; 00273 data[0] = reg; 00274 data[1] = val; 00275 m_i2c.write((int)FT6206_ADDR, data, 2); 00276 } 00277 00278 char FT6206::dataReceived(void) { 00279 return !m_interrupt; 00280 } 00281 00282 /****************/ 00283 00284 TS_Point::TS_Point(void) { 00285 x = y = 0; 00286 } 00287 00288 TS_Point::TS_Point(int16_t x0, int16_t y0, int16_t z0) { 00289 x = x0; 00290 y = y0; 00291 z = z0; 00292 } 00293 00294 bool TS_Point::operator==(TS_Point p1) { 00295 return ((p1.x == x) && (p1.y == y) && (p1.z == z)); 00296 } 00297 00298 bool TS_Point::operator!=(TS_Point p1) { 00299 return ((p1.x != x) || (p1.y != y) || (p1.z != z)); 00300 }
Generated on Thu Jul 14 2022 07:18:42 by
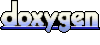