
Demo Adafruit 2.8" TFT Touch Shield for Arduino w/Capacitive Touch
Dependencies: Adafruit_GFX FT6206 SPI_TFT_ILI9341 mbed
main.cpp
00001 #include "mbed.h" 00002 #include "SPI_TFT_ILI9341.h" 00003 #include "FT6206.h" 00004 00005 #include "Arial12x12.h" 00006 00007 #define PIN_XP A3 00008 #define PIN_XM A1 00009 #define PIN_YP A2 00010 #define PIN_YM A0 00011 #define PIN_SCLK D13 00012 #define PIN_MISO D12 00013 #define PIN_MOSI D11 00014 #define PIN_CS_TFT D10 // chip select pin 00015 #define PIN_DC_TFT D9 // data/command select pin. 00016 #define PIN_RESET_TFT D8 00017 //#define PIN_BL_TFT D7 00018 #define PIN_CS_SD D4 00019 00020 #define PORTRAIT 0 00021 #define LANDSCAPE 1 00022 00023 #define PIN_SCL_FT6206 P0_28 00024 #define PIN_SDA_FT6206 P0_27 00025 #define PIN_INT_FT6206 D7 00026 00027 #define ILI9341_TFTWIDTH 320 00028 #define ILI9341_TFTHEIGHT 240 00029 00030 DigitalOut led1(LED1); 00031 DigitalOut led2(LED2); 00032 DigitalOut led3(LED3); 00033 DigitalOut led4(LED4); 00034 00035 //SPI_TFT_ILI9341 TFT(p5, p6, p7, p8, p9, p10,"TFT"); // mosi, miso, sclk, cs, reset, dc 00036 SPI_TFT_ILI9341 TFT(PIN_MOSI, PIN_MISO, PIN_SCLK, PIN_CS_TFT, PIN_RESET_TFT, PIN_DC_TFT, "TFT"); // mosi, miso, sclk, cs, reset, dc 00037 FT6206 FT6206(PIN_SDA_FT6206, PIN_SCL_FT6206, PIN_INT_FT6206); // sda, scl, int 00038 00039 int main() 00040 { 00041 //Configure the display driver 00042 TFT.claim(stdout); 00043 TFT.background(Black); 00044 TFT.foreground(White); 00045 TFT.set_orientation(LANDSCAPE); 00046 TFT.cls(); 00047 00048 //Print a welcome message 00049 TFT.set_font((unsigned char*) Arial12x12); 00050 TFT.locate(0,0); 00051 TFT.printf("Hello mbed!\n"); 00052 00053 FT6206.begin(); 00054 int X1, Y1, X2, Y2; 00055 X2 = -100; 00056 while(1) { 00057 // if (FT6206.touched()) { 00058 if (FT6206.dataReceived()) { 00059 // led1 = !led1; 00060 // Retrieve a point 00061 TS_Point p = FT6206.getPoint(); 00062 X1 = X2; 00063 Y1 = Y2; 00064 X2 = p.x; 00065 Y2 = p.y; 00066 // printf("Touch %3d %3d\n", p.x, p.y); 00067 if ((X1 > 0) && (Y1 > 0) && (X2 > 0) && (Y2 > 0)) { 00068 TFT.line(X1, Y1, X2, Y2, Yellow); 00069 } 00070 } 00071 00072 00073 // TFT.printf("Jacksoft\n"); 00074 // wait(0.05); 00075 } 00076 } 00077 00078 00079 /* 00080 #include "mbed.h" 00081 00082 PwmOut mypwm(PWM_OUT); 00083 00084 DigitalOut myled(LED1); 00085 00086 int main() { 00087 00088 mypwm.period_ms(10); 00089 mypwm.pulsewidth_ms(1); 00090 00091 printf("pwm set to %.2f %%\n", mypwm.read() * 100); 00092 00093 while(1) { 00094 myled = !myled; 00095 wait(1); 00096 } 00097 } 00098 */
Generated on Tue Jul 12 2022 18:40:19 by
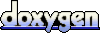