Infrared remote library for Arduino: send and receive infrared signals with multiple protocols Port from Arduino-IRremote https://github.com/z3t0/Arduino-IRremote
ir_Sanyo.cpp
00001 #include "IRremote.h" 00002 #include "IRremoteInt.h" 00003 00004 //============================================================================== 00005 // SSSS AAA N N Y Y OOO 00006 // S A A NN N Y Y O O 00007 // SSS AAAAA N N N Y O O 00008 // S A A N NN Y O O 00009 // SSSS A A N N Y OOO 00010 //============================================================================== 00011 00012 // I think this is a Sanyo decoder: Serial = SA 8650B 00013 // Looks like Sony except for timings, 48 chars of data and time/space different 00014 00015 #define SANYO_BITS 12 00016 #define SANYO_HDR_MARK 3500 // seen range 3500 00017 #define SANYO_HDR_SPACE 950 // seen 950 00018 #define SANYO_ONE_MARK 2400 // seen 2400 00019 #define SANYO_ZERO_MARK 700 // seen 700 00020 #define SANYO_DOUBLE_SPACE_USECS 800 // usually ssee 713 - not using ticks as get number wrapround 00021 #define SANYO_RPT_LENGTH 45000 00022 00023 //+============================================================================= 00024 #if DECODE_SANYO 00025 bool IRrecv::decodeSanyo (decode_results *results) 00026 { 00027 long data = 0; 00028 int offset = 0; // Skip first space <-- CHECK THIS! 00029 00030 if (irparams.rawlen < (2 * SANYO_BITS) + 2) return false ; 00031 00032 #if 0 00033 // Put this back in for debugging - note can't use #DEBUG as if Debug on we don't see the repeat cos of the delay 00034 printf("IR Gap: %d\n", results->rawbuf[offset]); 00035 printf("test against: %d\n", results->rawbuf[offset]); 00036 #endif 00037 00038 // Initial space 00039 if (results->rawbuf[offset] < SANYO_DOUBLE_SPACE_USECS) { 00040 //Serial.print("IR Gap found: "); 00041 results->bits = 0; 00042 results->value = REPEAT; 00043 results->decode_type = SANYO; 00044 return true; 00045 } 00046 offset++; 00047 00048 // Initial mark 00049 if (!MATCH_MARK(results->rawbuf[offset++], SANYO_HDR_MARK)) return false ; 00050 00051 // Skip Second Mark 00052 if (!MATCH_MARK(results->rawbuf[offset++], SANYO_HDR_MARK)) return false ; 00053 00054 while (offset + 1 < irparams.rawlen) { 00055 if (!MATCH_SPACE(results->rawbuf[offset++], SANYO_HDR_SPACE)) break ; 00056 00057 if (MATCH_MARK(results->rawbuf[offset], SANYO_ONE_MARK)) data = (data << 1) | 1 ; 00058 else if (MATCH_MARK(results->rawbuf[offset], SANYO_ZERO_MARK)) data = (data << 1) | 0 ; 00059 else return false ; 00060 offset++; 00061 } 00062 00063 // Success 00064 results->bits = (offset - 1) / 2; 00065 if (results->bits < 12) { 00066 results->bits = 0; 00067 return false; 00068 } 00069 00070 results->value = data; 00071 results->decode_type = SANYO; 00072 return true; 00073 } 00074 #endif
Generated on Fri Jul 15 2022 02:03:08 by
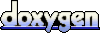