
Chua chaotic oscillator and MCP4922 DAC
Embed:
(wiki syntax)
Show/hide line numbers
MCP4922.h
00001 /* 00002 * MCP4922 - DAC library. 00003 * 00004 * Copyright (c) 2011 Steven Beard, UK Astronomy Technology Centre. 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in 00014 * all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00022 * THE SOFTWARE. 00023 */ 00024 00025 #include "mbed.h" 00026 00027 #ifndef MCP4922_H 00028 #define MCP4922_H 00029 00030 /* Reference: Microchip Technology (2005), MCP4821/MCP4822 DAC Data Sheet. */ 00031 00032 // MCP4922 reference voltage. 00033 #define MCP4922_VREF 2048 // Reference voltage (mV) 00034 00035 /* Define possible combinations of 16-bit command register bits */ 00036 #define MCP4922_REG_A1 0x3000 // Channel A gain 1 00037 #define MCP4922_REG_B1 0xB000 // Channel B gain 1 00038 #define MCP4922_REG_SHDN 0x0000 // Output power down 00039 00040 class MCP4922 { 00041 public: 00042 00043 MCP4922 (PinName mosi, PinName sclk, PinName cs); 00044 00045 ~MCP4922(); 00046 00047 /*+ 00048 * frequency: Set the SPI bus clock frequency in Hz. 00049 * The SPI bus frequency in Hz. Must be within the range 00050 * supported by both the SPI interface and the DAC chips 00051 * (~10 KHz to 20 MHz). 00052 */ 00053 void frequency( int freq ); 00054 00055 void writeA(int value ); 00056 00057 void writeB(int value ); 00058 00059 void write( int nchans, int values[], int gain=2, int latch=1 ); 00060 00061 void latch_enable(); 00062 00063 void latch_disable(); 00064 00065 private: 00066 00067 MCP4922( const MCP4922& rhs ); 00068 00069 void _init(); 00070 00071 int _ndacs; // The number of DACS in the array 00072 int _latched; // Is the "not LDAC" pin used (1=yes; 0=no)? 00073 SPI _spi; // SPI bus object for communicating with DAC. 00074 DigitalOut** _ncs_array; // Array of pointers to DigitalOut objects 00075 // connected to "not CS" pins. 00076 DigitalOut* _nldac; // Pointer to DigitalOut object connected 00077 // to "not LDAC" pin (if any - NULL if none). 00078 }; 00079 00080 #endif
Generated on Tue Jul 12 2022 21:11:13 by
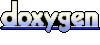