
Clifford chaotic attractor - uVGAII(SGC) 4DGL version 640x480 pin p9 - rx, pin p10 - tx, pin p11 - rst
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 Serial _cmd (p9, p10); 00004 DigitalOut _rst (p11); 00005 00006 void startVGA(); 00007 void baudrate(); 00008 void cls(); 00009 void pixel(int, int, int); 00010 int writeCOM(char *, int); 00011 00012 00013 int main() { 00014 00015 startVGA (); 00016 00017 float x = 1; 00018 float y = 1; 00019 00020 float ix = 0; 00021 float iy = 0; 00022 00023 float a = -1.4; 00024 float b = 1.6; 00025 float c = 1; 00026 float d = 0.7; 00027 00028 while (1) { 00029 00030 ix = sin(a*y) - c*cos(a*x); 00031 iy = sin(b*x) - d*cos(b*y); 00032 00033 pixel(375+(180*ix),255+(150*iy),65534); 00034 00035 x = ix; 00036 y = iy; 00037 00038 } 00039 } 00040 00041 00042 void startVGA () { 00043 00044 _rst = 1; 00045 _rst = 0; 00046 wait_ms(1); 00047 _rst = 1; 00048 wait(3); 00049 00050 while (_cmd.readable()) _cmd.getc(); 00051 00052 char autobaud[1] = ""; 00053 autobaud[0] = '\x55'; 00054 writeCOM(autobaud, 1); 00055 00056 baudrate(); 00057 00058 cls(); 00059 00060 char dispctr[3]= ""; 00061 dispctr[0] = '\x59'; 00062 dispctr[1] = 0x0c; 00063 dispctr[2] = 0x01; 00064 writeCOM(dispctr, 3); 00065 00066 } 00067 00068 00069 void pixel(int x, int y, int color) { 00070 00071 char pixel[7]= ""; 00072 00073 pixel[0] = '\x50'; 00074 00075 pixel[1] = (x >> 8) & 0xFF; 00076 pixel[2] = x & 0xFF; 00077 00078 pixel[3] = (y >> 8) & 0xFF; 00079 pixel[4] = y & 0xFF; 00080 00081 pixel[5] = (color >> 8) & 0xFF; 00082 pixel[6] = color & 0xFF; 00083 00084 writeCOM(pixel, 7); 00085 } 00086 00087 00088 void baudrate() { 00089 00090 char baudrate[2]= ""; 00091 00092 baudrate[0] = '\x51'; 00093 baudrate[1] = '\x0F'; 00094 00095 int i, resp = 0; 00096 00097 while (_cmd.readable()) _cmd.getc(); 00098 00099 long speed = speed = 281000; 00100 00101 for (i = 0; i <2; i++) _cmd.putc(baudrate[i]); 00102 _cmd.baud(speed); 00103 00104 while (!_cmd.readable()) wait_ms(1); 00105 00106 if (_cmd.readable()) resp = _cmd.getc(); 00107 switch (resp) { 00108 case '\x06' : 00109 resp = 1; 00110 break; 00111 case '\x15' : 00112 resp = -1; 00113 break; 00114 default : 00115 resp = 0; 00116 break; 00117 } 00118 00119 } 00120 00121 00122 int writeCOM(char *command, int number) { 00123 00124 int i, resp = 0; 00125 00126 while (_cmd.readable()) _cmd.getc(); 00127 00128 for (i = 0; i < number; i++) _cmd.putc(command[i]); 00129 00130 while (!_cmd.readable()) wait_ms(1); 00131 if (_cmd.readable()) resp = _cmd.getc(); 00132 switch (resp) { 00133 case '\x06' : 00134 resp = 1; 00135 break; 00136 case '\x15' : 00137 resp = -1; 00138 break; 00139 default : 00140 resp = 0; 00141 break; 00142 } 00143 00144 return resp; 00145 } 00146 00147 00148 void cls() { 00149 char cls[1] = ""; 00150 cls[0] = '\x45'; 00151 writeCOM(cls, 1); 00152 }
Generated on Wed Aug 3 2022 03:29:55 by
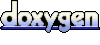