Nucleo-transfer
Dependencies: ADS1015 MPU6050 PixelArray-Nucleo mbed
Fork of Momo_Pilot_1 by
main.cpp
00001 /********************* CODE INFORMATON ****************************** 00002 Date of creation: 30-09-2017 00003 Authors: Danny Eldering & Ricardo Molenaar 00004 co-authors: Menno Gravemaker 00005 (c) Copyright by Momo Medical BV. 00006 00007 Current version name: 2.1.3 00008 Date of modification: 18-10-2017 00009 Purpose of this file: Code for LPC1768 microcontroller for controlling buttons, LED's and communicate to PI 00010 Update ‘what’s new in this version?’: New structure added. 00011 Readability improved. 00012 Code optimized (variables and functions). 00013 Todo: -> Fix LED issue (yellow and red flashes at random moments); 00014 -> Optimize functions / improve readability; 00015 -> Split functions in seperate files?; 00016 -> Fix when sensorplate is not connected; 00017 -> Rule 570: if statement change to turn off LED's when power is plugged out (also related to rule 106). 00018 -> For the speaker two outputs of the uC are used. Add MOSFET with external supply and control these by uC? 00019 Source file: http://mbed.com/ 00020 00021 Information files: 00022 (1) Flowchart: 00023 (2) Table serial communication: https://docs.google.com/spreadsheets/d/1kHlithHxtoMDGvbcdH8vwSw5W5ArxlwDPsyfra1dtQM/edit?usp=drive_web 00024 (3) Technical manual CU-/software: 00025 */ 00026 00027 /************************ CONFIG ***********************************/ 00028 00029 #include "mbed.h" // Include files and define parameters. 00030 #include "Adafruit_ADS1015.h" 00031 #include "MPU6050.h" 00032 #include "MPU6050_belt.h" 00033 #include "neopixel.h" 00034 00035 #define NUMBER_LED_FRONT (3) // declaren waarvoor dient 00036 #define ONE_COLOR 00037 00038 InterruptIn button_lock(PC_0); // Input on intterupt base decleration. 00039 InterruptIn button_reposition(PC_1); 00040 InterruptIn button_mute(PC_2); 00041 InterruptIn button_new_patient(PC_3); 00042 00043 DigitalOut LED_on_dev_board1(LED1); // Decleration of digital outputs. 00044 DigitalOut LED_on_dev_board2(LED2); 00045 DigitalOut LED_on_dev_board3(LED3); 00046 DigitalOut LED_on_dev_board4(LED4); 00047 DigitalOut speaker1(PC_8); // relatie aangeven! 00048 DigitalOut speaker2(PC_6); 00049 neopixel::PixelArray indicator_LEDs(PA_7); 00050 00051 PwmOut lock_feedback_LED(PB_13); // Declaration of pulse with modulation outputs. 00052 PwmOut mute_feedback_LED(PB_1); 00053 PwmOut new_patient_feedback_LED(PB_14); 00054 PwmOut reposition_feedback_LED(PB_15); 00055 00056 Timer button_lock_hold_timer; // Timer for time lock button should be pressed. 00057 Timer button_calibration_hold_timer; // Timer for calibration function (new patient holding 5 seconds). 00058 Timer delay_between_button_pressed; // Timer for time between two buttons (to prevent pressing buttons simultaneously). 00059 Timer speaker_timer; // Timer for speaker activation. 00060 Timer piezo_electric_sample_timer; // Timer for equally time-spaced samples. 00061 00062 /* 00063 The code underneath this commentbox has some fixed parameters for serial/ADC reading: 00064 -> The address for the angle_device_reference_belt is set to 0x68 in the file MPU6050_belt (rule number: 19); 00065 -> The adress for the angle_device_sensorplate is set to 0x69 in the file MPU6050.h (rule number: 19); 00066 -> This is because of using the same I2C line; 00067 -> For detailed information/questions about this item, please read the technical manual or contact: Ricardo Molenaar | ricardo.molenaar@gmail.com 00068 */ 00069 I2C i2c_sensorplate_adc(PB_9, PB_8); // I2C for sensorplate. 00070 I2C i2c_power_adc(PB_11, PB_10); // I2C for accupack. 00071 MPU6050 angle_device_sensorplate(PB_9, PB_8); // i2c pins // i2c address hardcoded 0x68. 00072 MPU6050_belt angle_device_reference_belt(PB_9, PB_8); // i2c pins // i2c address hardcoded 0x69. 00073 Adafruit_ADS1115 piezo_resistive_adc1(&i2c_sensorplate_adc, 0x48); // i2c pins, i2c address. 00074 Adafruit_ADS1115 piezo_resistive_adc2(&i2c_sensorplate_adc, 0x49); // i2c pins, i2c address. 00075 Adafruit_ADS1115 piezo_electric_adc(&i2c_sensorplate_adc, 0x4B); // i2c pins, i2c address. 00076 Adafruit_ADS1115 adsAccu(&i2c_power_adc, 0x48); // i2c pins, i2c address. 00077 Serial usb_serial(SERIAL_TX, SERIAL_RX); // tx, rx 00078 Serial pi_serial(PC_10, PC_11); // tx, rx 00079 Ticker total_readout_cycle; // Polling cycle. 00080 // End of commentbox related to the serial configuration/ADC reading components. 00081 00082 int boot_delay_ms = 500; 00083 int total_readout_cycle_time_us = 100000 * 2; // Cycle time in us. 00084 int i2c__frequency = 400000; // I2C Frequency. 00085 int baud_rate = 115200; // Baud rate. 00086 short piezo_resistive_array[8] = {0,0,0,0,0,0,0,0}; // 8 PR sensors 1 time per cycle. 00087 short piezo_electric_array[5] = {0,0,0,0,0}; // 1 PE sensor 5 times per cycle. 00088 int angle = 0; // Accelerometer Z-axis. 00089 float accelerometer_sensorplate[3] = {0.0, 0.0, 0.0}; // Raw accelerometer data. 00090 float gyroscope_sensorplate[3]; // Raw gyroscope data. 00091 float accelerometer_reference_belt[3]; // Raw accelerometer data from belt. 00092 float gyroscope_reference_belt[3]; // Raw gyroscope data from belt. 00093 char LED_colour = 'g'; // Variable to set LED colour (standard set to green, untill PI sends other character). Other possible colours: red ('r') & yellow ('y'). 00094 bool lock_state = false, lock_flag = 0, mute_state = 0, alarm = 0, calibration_flag = 0, intensity_select = 1; // Boolean variables for logging states. 00095 bool mute_flag = 0, new_patient_flag = 0, reposition_flag = 0; // Flag variables. 00096 bool speaker_state = 0, LED_red_state = 0, LED_yellow_state = 0, LED_green_state = 0, power_plug_state = 0; 00097 bool speaker_logged = 0, LED_red_logged = 0, LED_yellow_logged = 0, LED_green_logged = 0, power_plug_logged = 0; // is toevoegen 00098 int locktime_ms = 2000; // Waittime for lock user interface in ms. 00099 int calibrationtime_ms = 5000; // Time to press new_patient button for calibration system. 00100 int calibration_flash = 0; // Variable for flash LED's to indicate calibration. 00101 int buttondelay_ms = 750; // Button delay in ms. 00102 int delay_lock_interface = 3000*60; // Delay for non using interface locktime. 00103 int speaker_active_ms = 750; // Time to iterate speaker on and off when alarm occurs. 00104 int alarm_voltage = 2400; // Needed voltage for alarm expressed as a digital 15 bit value (= 20% of max battery voltage). 00105 int LED_red_intensity = 0, LED_blue_intensity = 0, LED_green_intensity = 0; // Variables to set LED intensity. 00106 short batteryvoltage_current = 0, batteryvoltage_last = 0, powervoltage_current, powervoltage_last; // Variables to manage batteryvoltage. Maybe change current to other? 00107 const int digital_value_ADC_powervoltage_unplugged = 15000; // Digital value to set the indicating LEDs to wall blue (should be set off later). const in hoofdletters 00108 int intensity_day = 40, intensity_night = 10; // Intensity settings for LED's to wall. 00109 double intensity = 0.0, control_LED_intensity = 0.0; // Variable between 0 and 1 to set the intensity of the LED's above the buttons. Intensity change to smart name! 00110 00111 /*************************** TEST ********************************/ 00112 // Verify algoritm function: for belt activation, set test_belt 1 (connect pin p20 to 3.3V). 00113 Timer test_timer; 00114 DigitalIn test_pin(PA_11, PullDown); 00115 00116 // Variable to set if belt is used to test algorithm: 00117 bool test_belt = 0; 00118 00119 // Set test mode on (log functions to pc serial: interrupts, LED intensity and serial messages): 00120 bool test_mode = 1; 00121 00122 // Variable for connection test (should be changed): 00123 int connection_test_sensorplate; 00124 00125 /*************************** CODE ********************************/ 00126 00127 void set_intensity_LEDs() // Function to set the intensity for the LED's. 00128 { 00129 if (intensity_select == 1) { 00130 intensity = intensity_day; 00131 } else { 00132 intensity = intensity_night; 00133 } 00134 control_LED_intensity = (intensity/100); 00135 00136 if (test_mode == 1) { // If statement for test purposal LED_intensity values. if def gebruiken voor testmode 00137 usb_serial.printf("Intensity LED's shines to wall = %f\n", intensity); 00138 usb_serial.printf("Intensity LED's above buttons = %f\n", control_LED_intensity); 00139 } 00140 } 00141 00142 void serial_read() // Function for serial read for select LED intensity and colour. 00143 { 00144 if (pi_serial.readable()) { // Function to check if pi is readable. 00145 char message[10]; 00146 pi_serial.scanf("%s", message); 00147 00148 if (test_mode == 1) { // If statement for test purposal. 00149 usb_serial.printf("Message = %s, Intensity_select = %d en LED_colour = %c\n", message, intensity_select, LED_colour); 00150 } 00151 00152 if (intensity_select != (message[0]-'0')) { // Read intensity for LED's variable from PI. 00153 intensity_select = (message[0]-'0'); 00154 } 00155 00156 if (LED_colour != message[1]) { // Read character from PI to set LED_colour. 00157 LED_colour = message[1]; 00158 } 00159 00160 if (test_mode == 1) { 00161 usb_serial.printf("Message: %s\n", message); 00162 usb_serial.printf("Intensity_select = %d en LED_colour = %c\n", intensity_select, LED_colour); 00163 } 00164 } 00165 } 00166 00167 void serial_log() // Function for serial logging. See link to table with code declarations above in code. 00168 { 00169 if (mute_flag == 1) { // If statement to control logging for mute button. 00170 pi_serial.printf(">01\n"); 00171 00172 if (test_mode == 1) { // If statement for test purposal. 00173 usb_serial.printf(">01\n"); 00174 } 00175 00176 mute_flag = 0; 00177 } 00178 00179 if (new_patient_flag == 1) { // If statement to control logging for new patient button. 00180 pi_serial.printf(">03\n"); 00181 00182 if (test_mode == 1) { // If statement for test purposal. 00183 usb_serial.printf(">03\n"); 00184 } 00185 00186 new_patient_flag = 0; 00187 } 00188 00189 if (reposition_flag == 1) { // If statement to control logging for reposition button. 00190 pi_serial.printf(">02\n"); 00191 00192 if (test_mode == 1) { // If statement for test purposal. 00193 usb_serial.printf(">02\n"); 00194 } 00195 00196 reposition_flag = 0; 00197 } 00198 00199 if (batteryvoltage_current != batteryvoltage_last) { // If statement to control logging for batteryvoltage. 00200 pi_serial.printf("%%" "%d\n", batteryvoltage_current); 00201 00202 if (test_mode == 1) { // If statement for test purposal. 00203 usb_serial.printf("%%" "%d\n", batteryvoltage_current); 00204 } 00205 00206 batteryvoltage_last = batteryvoltage_current; 00207 } 00208 00209 if (LED_red_logged != LED_red_state) { // If statement to control logging for LED_red. 00210 if (LED_red_state == 1) { 00211 pi_serial.printf("&04\n"); 00212 LED_red_logged = LED_red_state; 00213 if (test_mode == 1) { 00214 usb_serial.printf("&04\n"); 00215 } 00216 } 00217 00218 if (LED_red_state == 0) { 00219 pi_serial.printf("&40\n"); 00220 LED_red_logged = LED_red_state; 00221 if (test_mode == 1) { 00222 usb_serial.printf("&40\n"); 00223 } 00224 } 00225 } 00226 00227 if (LED_yellow_logged != LED_yellow_state) { // If statement to control logging for LED_yellow. 00228 if (LED_yellow_state == 1) { 00229 pi_serial.printf("&06\n"); 00230 LED_yellow_logged = LED_yellow_state; 00231 if (test_mode == 1) { 00232 usb_serial.printf("&06\n"); 00233 } 00234 } 00235 if (LED_yellow_state == 0) { 00236 pi_serial.printf("&60\n"); 00237 LED_yellow_logged = LED_yellow_state; 00238 if (test_mode == 1) { 00239 usb_serial.printf("&60\n"); 00240 } 00241 } 00242 } 00243 00244 if (LED_green_logged != LED_green_state) { // If statement to control logging for LED_green. 00245 if (LED_green_state == 1) { 00246 pi_serial.printf("&05\n"); 00247 LED_green_logged = LED_green_state; 00248 00249 if (test_mode == 1) { 00250 usb_serial.printf("&05\n"); 00251 } 00252 } 00253 00254 if (LED_green_state == 0) { 00255 pi_serial.printf("&50\n"); 00256 LED_green_logged = LED_green_state; 00257 00258 if (test_mode == 1) { 00259 usb_serial.printf("&50\n"); 00260 } 00261 } 00262 } 00263 00264 if (speaker_logged != speaker_state) { // If statement to control logging for speaker. 00265 if (speaker_state == 1) { 00266 pi_serial.printf("&07\n"); 00267 speaker_logged = speaker_state; 00268 00269 if (test_mode == 1) { // If statement for test purposal. 00270 usb_serial.printf("&07\n"); 00271 } 00272 } 00273 00274 if (speaker_state == 0) { 00275 pi_serial.printf("&70\n"); 00276 speaker_logged = speaker_state; 00277 00278 if (test_mode == 1) { // If statement for test purposal. 00279 usb_serial.printf("&70\n"); 00280 } 00281 } 00282 } 00283 00284 if (power_plug_logged != power_plug_state) { // If statement to control the logging for the state of the power plug. 00285 if (power_plug_state == 1) { 00286 pi_serial.printf("#08\n"); 00287 00288 if (test_mode == 1) { // If statement for test purposal. 00289 usb_serial.printf("#08\n"); 00290 } 00291 power_plug_logged = power_plug_state; 00292 } 00293 00294 if (power_plug_state == 0) { 00295 pi_serial.printf("#80\n"); 00296 00297 if (test_mode == 1) { // If statement for test purposal. 00298 usb_serial.printf("#80\n"); 00299 } 00300 power_plug_logged = power_plug_state; 00301 } 00302 } 00303 00304 if (connection_test_sensorplate == 1) { // If statement for sending serial information sensorplate data when connection test is active. 00305 // Receiving order sensor information: 8 resistive sensors, 5 electric readings. Is splitted in two parts - part 1/2. 00306 pi_serial.printf("!,%d,%d,%d,%d,%d,%d,%d,%d,%d,%d,%d,%d,%d,\n", piezo_resistive_array[0], piezo_resistive_array[1], piezo_resistive_array[2], piezo_resistive_array[3], piezo_resistive_array[4], piezo_resistive_array[5], piezo_resistive_array[6], piezo_resistive_array[7], piezo_electric_array[0], piezo_electric_array[1], piezo_electric_array[2], piezo_electric_array[3], piezo_electric_array[4]); // print all to serial port 00307 00308 if (test_mode == 1) { 00309 usb_serial.printf("!,%d,%d,%d,%d,%d,%d,%d,%d,%d,%d,%d,%d,%d,\n", piezo_resistive_array[0], piezo_resistive_array[1], piezo_resistive_array[2], piezo_resistive_array[3], piezo_resistive_array[4], piezo_resistive_array[5], piezo_resistive_array[6], piezo_resistive_array[7], piezo_electric_array[0], piezo_electric_array[1], piezo_electric_array[2], piezo_electric_array[3], piezo_electric_array[4]); // print all to serial port 00310 } 00311 } 00312 00313 } 00314 00315 void colour_select_indicating_LED_wall(char LED_colour) // Function to select the colour for LED's to wall (values comes from algorithm). 00316 { 00317 set_intensity_LEDs(); // Call function set_intensity_LEDs to set the intensity for LED's to wall and above buttons. 00318 00319 if ((LED_colour == 'r') || (LED_colour == 'g') || (LED_colour == 'b') || (LED_colour == 'y')) { // If statement to prevent potential errors in communication. 00320 LED_red_intensity = 0; // Reset 00321 LED_green_intensity = 0; 00322 LED_blue_intensity = 0; 00323 00324 if (LED_colour == 'r') { // Set LED_colour to red. 00325 LED_red_intensity = (2.55*intensity); // 255 / 100 = 2.55 (8 - bit digital value; 0-255 = 256 steps); intensity is a value between 0 and 100. 00326 LED_green_intensity = 0; 00327 LED_blue_intensity = 0; 00328 LED_red_state = 1; 00329 } else { 00330 LED_red_state = 0; 00331 } 00332 00333 if (LED_colour == 'y') { // Set LED_colour to yellow. 00334 LED_red_intensity = (2.55*intensity); 00335 LED_green_intensity = (2.55*intensity); 00336 LED_blue_intensity = 0; 00337 LED_yellow_state = 1; 00338 } else { 00339 LED_green_state = 0; 00340 } 00341 00342 if (LED_colour == 'g') { // Set LED_colour to green. 00343 LED_red_intensity = 0; 00344 LED_green_intensity = (2.55*intensity); 00345 LED_blue_intensity = 0; 00346 LED_green_state = 1; 00347 } else { 00348 LED_green_state = 0; 00349 } 00350 00351 if (LED_colour == 'b') { // Set LED_colour to blue. 00352 LED_red_intensity = 0; 00353 LED_green_intensity = 0; 00354 LED_blue_intensity = (2.55*intensity); 00355 } 00356 } 00357 00358 if (calibration_flash >= 1) { // If statement for flashing LED's (colour = white) when calibration is active. 00359 if ((calibration_flash % 2) == 0) { // If value can not be devided by two, set LED's on. 00360 LED_red_intensity = 255; 00361 LED_green_intensity = 255; 00362 LED_blue_intensity = 255; 00363 LED_on_dev_board4 = 1; 00364 } else { // Else set LED's off. 00365 LED_red_intensity = 0; 00366 LED_green_intensity = 0; 00367 LED_blue_intensity = 0; 00368 LED_on_dev_board4 = 0; 00369 } 00370 calibration_flash--; 00371 } 00372 } 00373 00374 void trigger_lock() // If rising edge lock button is detected start locktimer. 00375 { 00376 if (test_mode == 1) { 00377 usb_serial.printf("Lock triggered.\n"); 00378 } 00379 00380 button_lock_hold_timer.reset(); 00381 button_lock_hold_timer.start(); 00382 delay_between_button_pressed.reset(); 00383 delay_between_button_pressed.start(); 00384 } 00385 00386 void end_timer_lock_button() // End timer lock. 00387 { 00388 if (test_mode == 1) { // If statement for test purposal. 00389 usb_serial.printf("Lock released.\n"); 00390 } 00391 lock_flag = 0; // Set lock_flag off. 00392 button_lock_hold_timer.stop(); // Stop and reset holdtimer 00393 button_lock_hold_timer.reset(); 00394 } 00395 00396 void reposition_button_triggered() 00397 { 00398 if (lock_state == 1 | (delay_between_button_pressed.read_ms() < buttondelay_ms)) { // Control statement for lock interface and delay for non using buttons at the same time. 00399 } else { 00400 delay_between_button_pressed.reset(); 00401 delay_between_button_pressed.start(); 00402 if (test_mode == 1) { // If statement for test purposal. 00403 usb_serial.printf("Reposition triggered.\n"); 00404 LED_on_dev_board1 = !LED_on_dev_board1; 00405 } 00406 reposition_flag = 1; 00407 00408 reposition_feedback_LED = control_LED_intensity; 00409 } 00410 } 00411 00412 void rise_reposition() // Interrupt for rising edge reposition function (deactivation; active low). 00413 { 00414 if (test_mode == 1) { // If statement for test purposal. 00415 usb_serial.printf("Reposition released.\n"); 00416 } 00417 reposition_feedback_LED = 0; 00418 00419 } 00420 00421 void mute_button_triggered() 00422 { 00423 00424 if (lock_state == 1 | (delay_between_button_pressed.read_ms() < buttondelay_ms)) { // Control statement for lock interface and delay for non using buttons at the same time. 00425 } else { 00426 delay_between_button_pressed.reset(); 00427 delay_between_button_pressed.start(); 00428 mute_state = !mute_state; 00429 00430 if (mute_state == 1) { // If statement for if mute_state is active, set mute feedback LED active. 00431 mute_feedback_LED = control_LED_intensity; 00432 } else { 00433 mute_feedback_LED = 0; 00434 } 00435 00436 if (test_mode == 1) { // If statement for test purposal. 00437 usb_serial.printf("Mute triggered %d.\n",mute_state); 00438 LED_on_dev_board1 = !LED_on_dev_board1; 00439 } 00440 00441 mute_flag = 1; 00442 } 00443 } 00444 00445 void trigger_new_patient() // Function to trigger hold timer for new patient and calibration function. 00446 { 00447 00448 if (lock_state == 1 | (delay_between_button_pressed.read_ms() < buttondelay_ms)) { 00449 } else { 00450 button_calibration_hold_timer.reset(); // inline ? 00451 button_calibration_hold_timer.start(); 00452 new_patient_feedback_LED = control_LED_intensity;; 00453 00454 if (test_mode == 1) { // If statement for test purposal. 00455 usb_serial.printf("New patient triggered.\n"); 00456 } 00457 } 00458 } 00459 00460 void activate_new_patient_function() // Timer calibration function. 00461 { 00462 if (test_mode == 1) { // If statement for test purposal. 00463 usb_serial.printf("New patient released.\n"); 00464 } 00465 new_patient_feedback_LED = 0; 00466 00467 if (0 < button_calibration_hold_timer.read_ms() < calibrationtime_ms) { // If statement for new_patient function: holdtime for calibration is les then set time to calibrate algorithm. && toevoegen? -. als mogelijk mailtje naar Bart: bart@straightupalgorithms.com 00468 new_patient_flag = 1; 00469 } 00470 00471 button_calibration_hold_timer.stop(); // Timer reset for calibration function of new patient button. 00472 button_calibration_hold_timer.reset(); 00473 00474 if (lock_state == 1 | (delay_between_button_pressed.read_ms() < buttondelay_ms)) { // Control statement for lock interface and delay for non using buttons at the same time. 00475 } else { 00476 if (calibration_flag == 0) { 00477 00478 if (LED_on_dev_board1 == 0) { // If statement for test purposal. 00479 LED_on_dev_board1 = 1; 00480 } else { 00481 LED_on_dev_board1 = 0; 00482 } 00483 00484 } else { 00485 calibration_flag = 0; 00486 } 00487 } 00488 } 00489 00490 void timer_functions() // Function which contains statements using timers. 00491 { 00492 if ((button_lock_hold_timer.read_ms() > locktime_ms) && lock_flag == 0 && button_lock == 0) { // If statement for lock function. 00493 lock_flag = 1; 00494 LED_on_dev_board2 = !LED_on_dev_board2; 00495 lock_state = !lock_state; 00496 00497 if (lock_state == 0) { // If statement to control lock feedback LED above button. 00498 lock_feedback_LED = control_LED_intensity; 00499 } else { 00500 lock_feedback_LED = 0; 00501 } 00502 } 00503 00504 if ((button_calibration_hold_timer.read_ms() > calibrationtime_ms) && calibration_flag == 0 && button_new_patient == 0 && lock_state == 0) { // If statement for calibration algorithm. 00505 calibration_flag = 1; 00506 calibration_flash = 11; 00507 00508 if (test_mode == 1) { // If statement for test purposal. 00509 usb_serial.printf("Calibrate triggered.\n"); 00510 } 00511 00512 pi_serial.printf(">30\n"); // Print statement for serial communication to inform algorithm to calibrate. 00513 } 00514 00515 if (delay_between_button_pressed.read_ms() > delay_lock_interface) { // If buttons are not pressed for 3 minutes, set lock active. 00516 lock_state = 1; 00517 LED_on_dev_board2 = 1; 00518 lock_feedback_LED = 0; 00519 } 00520 } 00521 00522 void generate(neopixel::Pixel * out, uint32_t index, uintptr_t val) // Generate LED colour function (library function PixelArray is used for this item). 00523 { 00524 out->red = LED_red_intensity; 00525 out->green = LED_green_intensity; 00526 out->blue = LED_blue_intensity; 00527 } 00528 00529 void set_userinterface_LED() // Control functions for LED above buttons (added because of failures). 00530 { 00531 if (lock_state == 1) { 00532 } else { 00533 if (button_reposition == 0) { 00534 reposition_feedback_LED = control_LED_intensity; 00535 } else { 00536 reposition_feedback_LED = 0; 00537 } 00538 00539 if (button_new_patient == 0) { 00540 new_patient_feedback_LED = control_LED_intensity; 00541 } else { 00542 new_patient_feedback_LED = 0; 00543 } 00544 } 00545 } 00546 00547 void read_voltage() // Function for reading voltages from power and battery. 00548 { 00549 if (power_plug_state == 1) { // If supplyvoltage (readed from input) is greater then the setted alarmvoltage. 00550 alarm = 0; // Alarm is off. 00551 speaker_state = 0; 00552 } else { 00553 alarm = 1; // Else alarm is on. 00554 speaker_state = 1; 00555 } 00556 00557 00558 if (alarm == 1 && mute_state == 1 && (batteryvoltage_current > alarm_voltage)) {// Set speaker on for 750 ms. Use PWM? => Split in more functions. 00559 speaker1 = 0; // Set speaker. 00560 speaker2 = 0; 00561 } 00562 00563 if ((alarm == 1 && mute_state == 0 && (speaker_timer.read_ms() < speaker_active_ms)) || ((batteryvoltage_current < alarm_voltage) && (speaker_timer.read_ms() < speaker_active_ms) && power_plug_state == 0)) { // Set speaker on for 750 ms. 00564 speaker1 = 1; // Set speaker. 00565 speaker2 = 1; 00566 speaker_timer.start(); // Set timer for speaker to iterate on and off. 00567 } 00568 00569 if ((speaker_timer.read_ms() > speaker_active_ms) && (speaker_timer.read_ms() < (speaker_active_ms*2))) { 00570 speaker1 = 0; // Turn off speaker (use two outputs because of currentlimiting of one). 00571 speaker2 = 0; 00572 } 00573 00574 if (speaker_timer.read_ms() > (speaker_active_ms*2)) { // 00575 speaker_timer.stop(); // Stop speaker timer. 00576 speaker_timer.reset(); 00577 } 00578 // Read channel 0 from external ADC (batteryvoltage); voltagedeviders are used, 00579 batteryvoltage_current = adsAccu.readADC_SingleEnded(0); // because of higher voltage then Vcc of ADC (5.3 V (= Vcc + 0.3 V) max possible at each analog input). 00580 powervoltage_current = adsAccu.readADC_SingleEnded(1); // Read channel 1 from external ADC (powervoltage). 00581 00582 if (powervoltage_current < digital_value_ADC_powervoltage_unplugged) { // If statement to set LED's to blue. 00583 power_plug_state = 0; 00584 LED_colour = 'b'; 00585 } else { 00586 power_plug_state = 1; 00587 } 00588 } 00589 00590 void read_adc() { 00591 piezo_electric_sample_timer.reset(); // Clock gebruiken o.i.d.? 00592 piezo_electric_sample_timer.start(); 00593 connection_test_sensorplate = angle_device_sensorplate.testConnection(); 00594 00595 if (test_mode == 1) { 00596 usb_serial.printf("Connection test sensorplate = %d\n", connection_test_sensorplate); 00597 } 00598 00599 /* 00600 if (connection_test_sensorplate == 0) { 00601 lock_state = 1; 00602 LED_on_dev_board2 = 1; 00603 lock_feedback_LED = 0; 00604 }*/ 00605 00606 if (connection_test_sensorplate == 1) { 00607 piezo_electric_array[0] = piezo_electric_adc.readADC_SingleEnded(0); // First PE readout. 00608 00609 for (uint8_t k = 0; k < 4; ++k) { 00610 piezo_resistive_array[k] = piezo_resistive_adc1.readADC_SingleEnded(k); // First 4 PR readout. 00611 } 00612 00613 while(piezo_electric_sample_timer.read_us()<(1*(total_readout_cycle_time_us/5))) {} // Wait untill 20% of cycle. Energy efficiency is not fine in this situation, correct if low energy is needed. 00614 00615 piezo_electric_array[1] = piezo_electric_adc.readADC_SingleEnded(0); // Second PE readout. 00616 00617 for (uint8_t k = 0; k < 4; ++k) { 00618 piezo_resistive_array[k+4] = piezo_resistive_adc2.readADC_SingleEnded(k); // Last 4 PR readout. 00619 } 00620 00621 while(piezo_electric_sample_timer.read_us()<(2*(total_readout_cycle_time_us/5))) {} // Wait untill 40% of cycle. Energy efficiency is not fine in this situation, correct if low energy is needed. 00622 00623 piezo_electric_array[2] = piezo_electric_adc.readADC_SingleEnded(0); // Third PE readout. 00624 00625 angle_device_sensorplate.getAccelero(accelerometer_sensorplate); // Get accelerometer data. 00626 angle = accelerometer_sensorplate[2]*100; 00627 if(angle == 0) { 00628 MPU6050 angle_device_sensorplate(PB_9, PB_8); 00629 angle_device_sensorplate.getAccelero(accelerometer_sensorplate); 00630 angle = accelerometer_sensorplate[2]*100; 00631 } 00632 angle_device_sensorplate.getGyro(gyroscope_sensorplate); // Get gyroscope data. 00633 00634 if (test_belt == 1) { 00635 angle_device_reference_belt.getGyro(gyroscope_reference_belt); // Get gyroscope data from Belt. 00636 angle_device_reference_belt.getAccelero(accelerometer_reference_belt); // Get accelerometer data from belt. 00637 } 00638 00639 if (connection_test_sensorplate == 1) { // If statement for sending serial information sensorplate data when connection test is active. 00640 // Receiving order sensor information: 3 accelero sensors & 3 gyroscope sensors from sensorplate; 3 accelero sensors & 3 gyroscope sensors from belt. Is splitted in two parts - part 2/2. 00641 pi_serial.printf("?,%f,%f,%f,%f,%f,%f,%f,%f,%f,%f,%f,%f,\n", accelerometer_sensorplate[0], accelerometer_sensorplate[1], accelerometer_sensorplate[2], gyroscope_sensorplate[0], gyroscope_sensorplate[1], gyroscope_sensorplate[2], accelerometer_reference_belt[0], accelerometer_reference_belt[1], accelerometer_reference_belt[2], gyroscope_reference_belt[0], gyroscope_reference_belt[1], gyroscope_reference_belt[2]); 00642 } // binair print and convert in pi 00643 00644 while(piezo_electric_sample_timer.read_us()<(3*(total_readout_cycle_time_us/5))) {} // Wait untill 60% of cycle. Energy efficiency is not fine in this situation, correct if low energy is needed. 00645 00646 piezo_electric_array[3] = piezo_electric_adc.readADC_SingleEnded(0); // Fourth PE readout. 00647 } 00648 00649 timer_functions(); 00650 00651 batteryvoltage_current = batteryvoltage_last; 00652 powervoltage_current = powervoltage_last; 00653 read_voltage(); // Read_voltage function to control alarm. 00654 00655 if (test_mode == 1) { 00656 usb_serial.printf("Voltage = %d , %d\n", batteryvoltage_current, powervoltage_current); 00657 } 00658 00659 uint32_t val = 0; 00660 colour_select_indicating_LED_wall(LED_colour); // Function to select colour. 00661 indicator_LEDs.update(generate, NUMBER_LED_FRONT, val); // Function to set the LED's which shines to the wall (indicating change patient position). 00662 set_userinterface_LED(); // Set LED's of user interface (LED's above buttons). 00663 00664 while(piezo_electric_sample_timer.read_us()<(4*(total_readout_cycle_time_us/5))) {} // Wait untill 80% of cycle. Energy efficiency is not fine in this situation, correct if low energy is needed. 00665 00666 if (test_mode == 1){ // If statement for test purposal. 00667 usb_serial.printf("Angle device sensorplate = %d\n",angle_device_sensorplate.testConnection()); 00668 } 00669 00670 if (connection_test_sensorplate == 1) { 00671 piezo_electric_array[4] = piezo_electric_adc.readADC_SingleEnded(0); // Fifth PE readout. 00672 } 00673 00674 while(piezo_electric_sample_timer.read_us()<(4.25*(total_readout_cycle_time_us/5))) {} // Wait untill 85% of cycle. Energy efficiency is not fine in this situation, correct if low energy is needed. 00675 00676 serial_read(); // Call function for reading information from PI by serial connection. 00677 serial_log(); // Call function for logging information to PI by serial connection. 00678 00679 if (test_mode == 1) { // If statements for test purposal (untill * mark). 00680 usb_serial.printf("Loop time: %d ms\n",piezo_electric_sample_timer.read_ms()); 00681 } 00682 if (test_pin == 1) { 00683 test_mode = 1; 00684 usb_serial.printf("%d\n",test_mode); 00685 } 00686 if (test_pin == 0) { 00687 test_mode = 0; 00688 usb_serial.printf("%d\n",test_mode); 00689 } 00690 00691 if (test_mode == 1) { 00692 usb_serial.printf("Loop time: %d ms\n",piezo_electric_sample_timer.read_ms()); 00693 } 00694 // * End of if statements for test purposal. 00695 } 00696 00697 int main() { // Main function. inline function "Momo Init" bijvoorbeeld 00698 wait_ms(boot_delay_ms); // Wait to boot sensorplate first. 00699 i2c_sensorplate_adc.frequency(i2c__frequency); // Set frequency for i2c connection to sensorplate (variable is declared in config part). 00700 i2c_power_adc.frequency(i2c__frequency); // Same as line 695, but now for ADC to read battery- en powervoltage. 00701 usb_serial.baud(baud_rate); // Set serial USB connection baud rate (variable is declared in config part). 00702 pi_serial.baud(baud_rate); // Same as line 697, but now for serial PI connection. 00703 piezo_resistive_adc1.setGain(GAIN_TWOTHIRDS); // Set ranges of ADC to +/-6.144V (end is marked with #): 00704 piezo_resistive_adc2.setGain(GAIN_TWOTHIRDS); 00705 piezo_electric_adc.setGain(GAIN_TWOTHIRDS); 00706 adsAccu.setGain(GAIN_TWOTHIRDS); // #) End of configuration ADC ranges. 00707 pi_serial.format(8, SerialBase::None, 1); // Set serial communication line with PI. 00708 00709 button_lock.fall(&trigger_lock); // Interrupt for rising edge lock button. 00710 button_lock.rise(&end_timer_lock_button); 00711 button_reposition.fall(&reposition_button_triggered); 00712 button_reposition.rise(&rise_reposition); 00713 button_mute.fall(&mute_button_triggered); 00714 button_new_patient.fall(&trigger_new_patient); // New patient/calibration button rising event. 00715 button_new_patient.rise(&activate_new_patient_function); // Falling edge for calibration algorithm option. 00716 delay_between_button_pressed.reset(); // Delaytimer reset en start. 00717 delay_between_button_pressed.start(); 00718 00719 set_intensity_LEDs(); // Initialize intensity for user interface LED's and LED's shines to wall. 00720 lock_feedback_LED = control_LED_intensity; // Lock LED initialization. 00721 00722 00723 total_readout_cycle.attach_us(&read_adc, total_readout_cycle_time_us); // Call function to start reading sensorplate and other functionalities. 00724 00725 while (1) { 00726 wait_us(total_readout_cycle_time_us+1); // Wait indefinitely. 00727 } 00728 }
Generated on Tue Jul 12 2022 21:07:48 by
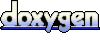