
hi
Dependencies: SDFileSystem mbed
Fork of SDFileSystem_HelloWorld by
main.cpp
00001 #include "mbed.h" 00002 #include "SDFileSystem.h" 00003 #include <string> 00004 #include <vector>\ 00005 #include <stdio.h> 00006 #include <iostream> 00007 00008 00009 SDFileSystem sd(D11, D12, D13, D10, "sd"); // the pinout on the mbed Cool Components workshop board 00010 00011 //.....assumes SDFileSystem is setup in earlier code for device "/sd" 00012 00013 vector<string> filenames; //filenames are stored in a vector string 00014 vector<string> filedata; //file data is stored in a vector string 00015 /////////////////////////////////////////////////////////////////////////////// 00016 using namespace std; 00017 typedef unsigned char BYTE; 00018 00019 long getFileSize(FILE *file) 00020 { 00021 long lCurPos,lEndPos; 00022 lCurPos = ftell(file); 00023 fseek(file,lCurPos, 0); 00024 return lEndPos; 00025 } 00026 ////////////////////////////////////////////////////////////////////////////// 00027 00028 00029 00030 void read_file_names(char *dir) 00031 { 00032 DIR *dp; 00033 struct dirent *dirp; 00034 dp = opendir(dir); 00035 //read all directory and file names in current directory into filename vector 00036 while((dirp = readdir(dp)) != NULL) { 00037 filenames.push_back(string(dirp->d_name)); 00038 } 00039 closedir(dp); 00040 } 00041 void read_file_data (char *dir) 00042 { 00043 DIR *dp; 00044 struct dirent *dirp; 00045 dp = opendir(dir); 00046 //read all directory and file names in current directory into filename vector 00047 while((dirp = readdir(dp)) != NULL) { 00048 filenames.push_back(string(dirp->d_name)); 00049 } 00050 closedir(dp); 00051 } 00052 int main() { 00053 printf("Hello World!\n"); 00054 00055 00056 00057 FILE *fp = fopen("/sd/mydir/sdtest.txt", "w"); 00058 if(fp == NULL) { 00059 error("Could not open file for write\n"); 00060 } 00061 fprintf(fp, "Hello fun SD Card World!"); 00062 00063 fclose(fp); 00064 00065 printf("Goodbye World!\n"); 00066 00067 printf("Reading files!\n"); 00068 00069 read_file_names("/sd"); 00070 //read_file_data("/sd/sky.raw"); 00071 00072 // print filename strings from vector using an iterator 00073 for(vector<string>::iterator it=filenames.begin(); it < filenames.end(); it++) 00074 { 00075 printf("%s\n\r",(*it).c_str()); 00076 } 00077 ////////////////////////////////////////////////////////////////////////////// 00078 const char *filePath = "/sd/sky.raw"; 00079 BYTE *fileBuf; 00080 FILE *file = NULL; 00081 00082 if ((file = fopen(filePath, "rb"))== NULL) 00083 { 00084 //cout << "Could not open the file" << end1 ; 00085 printf("Could not open the file"); 00086 00087 } 00088 else 00089 { 00090 00091 // cout << "opening file successfull" << end1 ; 00092 printf("opening file successfull"); 00093 } 00094 00095 long fileSize = getFileSize(file); 00096 00097 fileBuf = new BYTE[fileSize]; 00098 00099 fread(fileBuf, fileSize, 1, file); 00100 00101 for(int i=0; i<100; i++) 00102 { 00103 printf("%X", fileBuf[i]); 00104 } 00105 00106 cin.get(); 00107 delete[]fileBuf; 00108 fclose(file); 00109 ///////////////////////////////////////////////////////////////////////////////// 00110 00111 00112 00113 ///////////////////////////////////////////////////////////////////////////////// 00114 00115 00116 00117 fp = fopen("/sd/mydir/sdtest.txt", "r+"); 00118 if(fp == NULL){ 00119 printf("error in opening file"); 00120 } 00121 00122 // fread(fp, buff, 10, readBuff); 00123 char line[100]; /* Line buffer */ 00124 // FRESULT fr; /* FatFs return code */ 00125 /* Read all lines and display it */ 00126 while (fgets(line, sizeof line, fp)) { 00127 printf(line); 00128 } 00129 00130 fscanf(fp, line, sizeof line); 00131 00132 printf("Reading line %s", line); 00133 } 00134 00135 00136 00137 00138 00139
Generated on Wed Jul 20 2022 03:14:05 by
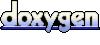