
Lan OLED
Dependencies: Adafruit_GFX WIZnetInterface mbed
Fork of Weather_Forecast_Helloworld_WIZwiki-W7500 by
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "Adafruit_SSD1306.h" 00004 00005 // W7500 onboard LED & Init 00006 DigitalOut rled(LED1,1); 00007 DigitalOut gled(LED2,0); 00008 DigitalOut bled(LED3,1); 00009 00010 // I2C Class 00011 I2C i2c(PA_10,PA_9); 00012 00013 // OLED Class 00014 Adafruit_SSD1306_I2c gOled(i2c,NC,0x78,64,128); 00015 00016 // Declare Ethernet Class 00017 EthernetInterface eth; 00018 00019 // Declare TCP Connection Class 00020 TCPSocketConnection sock; 00021 00022 00023 // LED desplay functions 00024 void clean(){ 00025 rled = 0; gled = 0; bled = 0; 00026 } 00027 void clouds(){ 00028 rled = 1; gled = 1; bled = 1; 00029 } 00030 void thunderstorm(){ 00031 while(1){ 00032 rled = 0; gled = 0; bled = 0; 00033 wait(0.5); 00034 rled = 1; gled = 1; bled = 1; 00035 wait(0.5); 00036 }; 00037 } 00038 void rain(){ 00039 rled = 1; gled = 1; bled = 0; 00040 } 00041 void mist(){ 00042 while(1){ 00043 rled = 1; gled = 1; bled = 0; 00044 wait(0.5); 00045 rled = 1; gled = 1; bled = 1; 00046 wait(0.5); 00047 }; 00048 } 00049 void haze(){ 00050 rled = 0; gled = 1; bled = 0; 00051 } 00052 void fog(){ 00053 while(1){ 00054 rled = 1; gled = 0; bled = 0; 00055 wait(1.0); 00056 rled = 1; gled = 1; bled = 0; 00057 wait(1.0); 00058 }; 00059 } 00060 void error(){ 00061 rled = 1; gled = 1; bled = 1; 00062 } 00063 00064 00065 int main() { 00066 00067 int phy_link; 00068 printf("Wait a second...\r\n"); 00069 00070 //--------- Have to modify the mac address------------- 00071 // 0 1 2 3 4 5 00072 uint8_t mac_addr[6] = {0x00, 0x08, 0xDC, 0xff, 0xff, 0x15}; 00073 // 0~2 회사코드. 00074 00075 00076 eth.init(mac_addr); //Use DHCP 00077 00078 while(1){ 00079 00080 // phy link 00081 do{ 00082 phy_link = eth.ethernet_link(); 00083 printf("..."); 00084 wait(2); 00085 }while(!phy_link); 00086 printf("\r\n"); 00087 00088 eth.connect(); 00089 00090 printf("IP Address is %s\r\n\r\n", eth.getIPAddress()); 00091 printf("MASK Address is %s\r\n\r\n", eth.getNetworkMask()); 00092 printf("GATEWAY Address is %s\r\n\r\n", eth.getGateway()); 00093 printf("MAC Address is %s\r\n\r\n", eth.getMACAddress()); 00094 00095 // TCP socket connect to openweather server 00096 //TCPSocketConnection sock; 00097 sock.connect("api.openweathermap.org", 80); 00098 00099 00100 // GET method, to request weather forecast 00101 //char http_cmd[] = "GET /data/2.5/weather?q=Seoul,kr&appid=a0ca47dd7f6066404629b3e1ad728981 HTTP/1.0\n\n"; 00102 char http_cmd[] = "GET /data/2.5/weather?q=London,uk&appid=be22367918cefc57bf4b4735bcbaf052 HTTP/1.0\n\n"; 00103 00104 // be22367918cefc57bf4b4735bcbaf052 00105 //char http_cmd[] = "GET /data/2.5/weather?q=Berlin,de&appid=a0ca47dd7f6066404629b3e1ad728981 HTTP/1.0\n\n"; 00106 00107 sock.send_all(http_cmd, sizeof(http_cmd)-1); 00108 00109 // get data into buffer 00110 char buffer[2048]; 00111 int ret; 00112 while (true) { 00113 ret = sock.receive(buffer, sizeof(buffer)-1); 00114 if (ret <= 0) 00115 break; 00116 buffer[ret] = '\0'; 00117 printf("Received %d chars from server: %s\n", ret, buffer); 00118 } 00119 printf("\r\n\r\n"); 00120 00121 // parsing current date, weather, city, tempurature 00122 char *date; 00123 char *weather; 00124 char *city; 00125 char *temper; 00126 00127 char cur_date[17] = {0}; 00128 char weather_con[15] = {0}; 00129 char city_name[10] = {0}; 00130 char temper_data[3] = {0}; 00131 00132 int temp; 00133 int num100, num10, num1; 00134 00135 //parding date 00136 date = strstr(buffer, "Date"); 00137 for(int x=0;x<17;x++){ 00138 cur_date[x] = date[x+6]; 00139 } 00140 00141 // parsing weather condition 00142 weather = strstr(buffer, "main"); 00143 for(int i=0; i<15;i++){ 00144 weather_con[i] = weather[i+7]; 00145 if(weather_con[i] == 34){ 00146 weather_con[i] = 0; 00147 break; 00148 } 00149 } 00150 00151 // parsing city name 00152 city = strstr(buffer, "name"); 00153 for(int j=0; j<10;j++){ 00154 city_name[j] = city[j+7]; 00155 if(city_name[j] == 34){ 00156 city_name[j] = 0; 00157 break; 00158 } 00159 } 00160 00161 //parsing current tempurature 00162 temper = strstr(buffer, "temp"); 00163 for(int k=0; k<3;k++){ 00164 temper_data[k] = temper[k+6]; 00165 } 00166 00167 //kelvin to celius converter 00168 num100 = temper_data[0]- 48; 00169 num10 = temper_data[1] - 48; 00170 num1 = temper_data[2]- 48; 00171 temp = (num100*100 + num10*10 + num1) - 273; 00172 00173 // Debug message 00174 printf("city name : %s\r\n", city_name); 00175 printf("weather : %s\r\n", weather_con); 00176 printf("temperature : %d\r\n\r\n", temp); 00177 00178 // OLED Display 00179 gOled.begin(); 00180 gOled.clearDisplay(); 00181 gOled.printf("%s\n\n", cur_date); 00182 gOled.printf("City : %s\n", city_name); 00183 gOled.printf("Weather : %s\n", weather_con); 00184 gOled.printf("Temper : %d\n", temp); 00185 gOled.display(); 00186 gOled.setTextCursor(0,0); 00187 00188 //LED display rely on weather condition 00189 if(strcmp(weather_con,"Clouds")==0) clouds(); 00190 else if(strcmp(weather_con,"Rain")==0) rain(); 00191 else if(strcmp(weather_con,"Thunderstorm")==0) thunderstorm(); 00192 else if(strcmp(weather_con,"Clean")==0) clean(); 00193 else if(strcmp(weather_con,"Mist")==0) mist(); 00194 else if(strcmp(weather_con,"Haze")==0) haze(); 00195 else if(strcmp(weather_con,"Fog")==0) fog(); 00196 else error(); 00197 00198 00199 //Disconnection 00200 sock.close(); 00201 eth.disconnect(); 00202 00203 /* 00204 * everytime in delay, request weather forecast 00205 */ 00206 wait(20.0); 00207 00208 }; 00209 00210 }
Generated on Thu Jul 14 2022 22:03:02 by
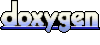