F746 GUI other class.
Dependents: DISCO-F746NG_test001
GUIinit.cpp
00001 // 00002 // 2016/04/27, Copyright (c) 2016 Takashi Inoue 00003 // Button OverLapping Class Source File 00004 // ver 0.9 rev 0.1 2016/5/18 00005 //----------------------------------------------------------- 00006 00007 00008 #include "GUIinit.hpp" 00009 00010 namespace TakaIno 00011 { 00012 // Constructor 00013 00014 GUIinit::GUIinit( 00015 uint16_t x, uint16_t y, sFONT &fonts, 00016 uint32_t textColor, uint32_t backColor, 00017 uint32_t createdColor, uint32_t touchedColor, 00018 uint32_t inactiveColor, uint32_t inactiveTextColor) 00019 00020 :GuiBase(x, y, fonts, //Initializer= GUIinit Const's args --> GuiBase Const. 00021 textColor, backColor, createdColor, 00022 touchedColor, inactiveColor, 00023 inactiveTextColor) 00024 00025 ,m_x(x), m_y(y), m_fonts(&fonts), //member initializer member(arg) 00026 m_text_color(textColor), m_back_color(backColor), 00027 m_created_color(createdColor), 00028 m_touched_color(touchedColor), 00029 m_inactive_color(inactiveColor), 00030 m_inactive_text_color(inactiveTextColor) 00031 00032 { 00033 00034 lcd_p = GetLcdPtr(); 00035 ts_p = GetTsPtr(); 00036 ts_state = GetTsState(); 00037 ts_touch_detected = ts_state.touchDetected; 00038 00039 bk_info.i_txtColor = textColor; 00040 00041 bk_info.i_bakColor = backColor; 00042 00043 g_lcd_width = BSP_LCD_GetXSize() ; 00044 g_lcd_height = BSP_LCD_GetYSize() ; 00045 g_width = g_lcd_width; 00046 g_height_offset = 0; 00047 } 00048 00049 GUIinit::~GUIinit() {;} 00050 00051 //GUI Resource Set Init 00052 void GUIinit::SetInit(sFONT &title_font, uint16_t btn_width) 00053 { 00054 g_lcd_width = BSP_LCD_GetXSize() ; 00055 g_lcd_height = BSP_LCD_GetYSize() ; 00056 00057 g_width = g_lcd_width - btn_width; //X game area limit 00058 g_height_offset = title_font.Height; //Y game area first 00059 00060 00061 } 00062 00063 LCD_DISCO_F746NG* GUIinit::GetLcdPtrOth() 00064 { 00065 return lcd_p; 00066 } 00067 00068 TS_DISCO_F746NG* GUIinit::GetTsPtrOth() 00069 { 00070 return ts_p; 00071 } 00072 00073 TS_StateTypeDef GUIinit::GetTsStateOth() 00074 { 00075 ts_p->GetState(&ts_state); 00076 return ts_state; 00077 } 00078 // If panel touched, return true 00079 bool GUIinit::PanelTouchedOth() 00080 { 00081 ts_p->GetState(&ts_state); 00082 // if (touchDetected is not changed) then return false 00083 if( ts_touch_detected != ts_state.touchDetected ) 00084 { 00085 ts_touch_detected = ts_state.touchDetected; 00086 return (bool)(ts_state.touchDetected); 00087 } 00088 return false; 00089 00090 } 00091 00092 00093 LCD_DISCO_F746NG *GUIinit::lcd_p; 00094 TS_DISCO_F746NG *GUIinit::ts_p; 00095 00096 TS_StateTypeDef GUIinit::ts_state; 00097 00098 uint16_t GUIinit::g_width; 00099 uint16_t GUIinit::g_height_offset; 00100 00101 uint16_t GUIinit::g_lcd_width; 00102 uint16_t GUIinit::g_lcd_height; 00103 00104 00105 //bool GUIinit::ts_touch_detected; 00106 bool ts_touch_detected; 00107 } 00108 00109 00110 00111
Generated on Thu Jul 14 2022 20:47:59 by
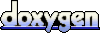