F746 GUI other class.
Dependents: DISCO-F746NG_test001
Circle.hpp
00001 // 00002 // 2016/04/27, Copyright (c) 2016 Takashi Inoue 00003 // Button OverLapping Class Header 00004 // ver 0.9 rev 0.1 2016/5/17 00005 //----------------------------------------------------------- 00006 00007 #ifndef F746_CIRCLE_HPP 00008 #define F746_CIRCLE_HPP 00009 00010 #include "GUIinit.hpp" //GUI init (TI) 00011 00012 using namespace Mikami; 00013 00014 namespace TakaIno 00015 { 00016 class Circle : public GUIinit 00017 { 00018 public: 00019 struct CircleInfo //GUI Circle Infomation 00020 { 00021 int16_t i_Xpos; 00022 int16_t i_Ypos; 00023 int16_t i_Radius; 00024 uint32_t i_txtColor; 00025 uint32_t i_bakColor; 00026 bool i_move_flg; 00027 int16_t i_Xstep; 00028 int16_t i_Ystep; 00029 bool i_disp_c_str; 00030 uint32_t i_c_str_col; 00031 int16_t i_c_str_idx; 00032 string i_c_str[2]; 00033 sFONT *i_c_fonts; 00034 uint16_t i_lcd_width; 00035 }; 00036 00037 Circle(); 00038 00039 Circle(struct CircleInfo cirinfo); 00040 00041 ~Circle(); 00042 00043 bool DrawCircle(int16_t Xpos=100, int16_t Ypos=100, int16_t Radius=50, 00044 uint32_t txtColor=LCD_COLOR_RED, uint32_t bakColor=LCD_COLOR_GREEN, 00045 bool disp_c_str_flg=false, uint32_t c_str_col=LCD_COLOR_BLACK, 00046 int16_t str_idx = 0, 00047 const string c_f_str="", const string c_b_str="", 00048 sFONT &c_fonts=Font16); 00049 00050 bool DrawCircle(bool dsp_circle); 00051 00052 bool ReverseCircleColor(); 00053 00054 bool DispOrEraseCircle(bool disp_flg); 00055 00056 bool CircleTouched(); 00057 00058 bool IsOnCircle(); 00059 00060 bool MoveCircle(bool move_flg, int16_t xstep, int16_t ystep); 00061 bool MoveCircle(bool move_flg); 00062 00063 bool ChangeCirclePos(); 00064 00065 uint16_t PosX(int16_t x, string str, sFONT &sfont); 00066 00067 uint16_t CalX(int16_t x); 00068 protected: 00069 struct CircleInfo m_c_info; 00070 00071 00072 //sFONT &i_c_fonts; 00073 }; 00074 00075 00076 } 00077 00078 #endif //F746_CIRCLE_HPP 00079 00080
Generated on Thu Jul 14 2022 20:47:59 by
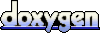