DMX512 send/recv library with STM32 slave support originally from http://mbed.org/users/okini3939/notebook/dmx512
Fork of DMX by
DMX.h
00001 /* 00002 * DMX512 send/recv library 00003 * Copyright (c) 2013 Hiroshi Suga 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 */ 00006 00007 /** @file 00008 * @brief DMX512 send/recv 00009 */ 00010 00011 #ifndef DMX_H 00012 #define DMX_H 00013 00014 #include "mbed.h" 00015 #include "RawSerial.h" 00016 00017 //#define DMX_UART_DIRECT 00018 00019 #define DMX_SIZE 512 00020 #define DMX_START_CODE 0 00021 00022 #define DMX_TIME_BREAK 100 // 100us (88us-1s) 00023 #define DMX_TIME_MAB 12 // 12us (8us-1s) 00024 #define DMX_TIME_MBB 200 // 10us (0-1s) 00025 00026 enum DMX_MODE { 00027 DMX_MODE_BEGIN, 00028 DMX_MODE_START, 00029 DMX_MODE_BREAK, 00030 DMX_MODE_MAB, 00031 DMX_MODE_DATA, 00032 DMX_MODE_ERROR, 00033 DMX_MODE_STOP, 00034 }; 00035 00036 /** DMX512 class (sender/client) 00037 */ 00038 class DMX { 00039 public: 00040 /** init DMX class 00041 * @param p_tx TX serial port (p9, p13, p28) 00042 * @param p_rx RX serial port (p10, p14, p27) 00043 */ 00044 DMX (PinName p_tx, PinName p_rx); 00045 00046 /** Send the data 00047 * @param addr DMX data address (0-511) 00048 * @param data DMX data (0-255) 00049 */ 00050 void put (int addr, int data); 00051 /** Send the data 00052 * @param buf DMX data buffer 00053 * @param addr DMX data address 00054 * @param len data length 00055 */ 00056 void put (unsigned char *buf, int addr = 0, int len = DMX_SIZE); 00057 00058 /** Send the data 00059 * @param addr DMX data address (0-511) 00060 * @return DMX data (0-255) 00061 */ 00062 int get (int addr); 00063 /** Send the data 00064 * @param buf DMX data buffer 00065 * @param addr DMX data address 00066 * @param len data length 00067 */ 00068 void get (unsigned char *buf, int addr = 0, int len = DMX_SIZE); 00069 00070 /** Start DMX send operation 00071 */ 00072 void start (); 00073 /** Stop DMX send operation 00074 */ 00075 void stop (); 00076 /** Clear DMX data 00077 */ 00078 void clear (); 00079 00080 int isReceived (); 00081 int isSent (); 00082 unsigned char *getRxBuffer (); 00083 unsigned char *getTxBuffer (); 00084 int setTimingParameters (int breaktime, int mab, int mbb); 00085 00086 void attach(void (*function)(void)); 00087 00088 protected: 00089 00090 void int_timer (); 00091 void int_tx (); 00092 void int_rx (); 00093 void on_received(); 00094 00095 // Serial _dmx; 00096 RawSerial _dmx; 00097 Timeout timeout01; 00098 volatile DMX_MODE mode_tx, mode_rx; 00099 volatile int addr_tx, addr_rx; 00100 unsigned char data_tx[DMX_SIZE]; 00101 unsigned char data_rx_working[DMX_SIZE]; 00102 unsigned char data_rx[DMX_SIZE]; 00103 int is_received, is_sent; 00104 int time_break, time_mab, time_mbb; 00105 Callback<void()> on_rx; 00106 00107 private: 00108 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) || defined(TARGET_LPC4088) 00109 LPC_UART_TypeDef *_uart; 00110 #elif defined(TARGET_LPC11UXX) 00111 LPC_USART_Type *_uart; 00112 #elif defined(TARGET_LPC11XX) 00113 LPC_UART_TypeDef *_uart; 00114 #elif defined(TARGET_STM) 00115 USART_TypeDef *_uart; 00116 #else 00117 #error "this CPU not supported." 00118 #endif 00119 00120 }; 00121 00122 #endif
Generated on Wed Jul 20 2022 05:54:08 by
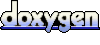