Techshop JAPANのボランティアリフロープログラムです。誰か改造して
Fork of MAX31855 by
Embed:
(wiki syntax)
Show/hide line numbers
max31855.cpp
00001 00002 #include <mbed.h> 00003 #include "max31855.h" 00004 00005 max31855::max31855(SPI& _spi, PinName _ncs) : spi(_spi), ncs(_ncs) { 00006 00007 } 00008 00009 float max31855::read_temp() { 00010 short value = 0; 00011 float temp = 0; 00012 00013 //Variables to hold probe temperature 00014 uint8_t tempProbeHigh=0; 00015 uint8_t tempProbeLow=0; 00016 00017 //Variables to hold chip temperature and device status 00018 uint8_t tempChipHigh=0; 00019 uint8_t tempChipLow=0; 00020 00021 if (pollTimer.read_ms() > 250){ 00022 //Set CS to initiate transfer and stop conversion 00023 select(); 00024 00025 //Read in Probe tempeature 00026 tempProbeHigh = spi.write(0); 00027 tempProbeLow = spi.write(0); 00028 00029 //Get the chip temperature and the fault data 00030 tempChipHigh = spi.write(0); 00031 tempChipLow = spi.write(0); 00032 00033 //Set the chip temperature 00034 chipTemp = (tempChipHigh<<4 | tempChipLow>>4)*0.25; 00035 00036 //Set CS to stop transfer and restart conversion 00037 deselect(); 00038 00039 //Check for a fault (last bit of transfer is fault bit) 00040 if ((tempProbeLow & 1)==1){ 00041 //Chip reports a fault, extract fault from Chip Temperature data 00042 int faultType = (tempChipLow & 7); 00043 00044 faultCode=faultType; 00045 00046 return 2000+faultType; 00047 /*if (faultType==1){ 00048 //Open circuit (no TC) 00049 return 2000 + faultType; 00050 }else if (faultType==2){ 00051 //Short to GND 00052 return 2000 + faultType; 00053 }else if (faultType==4){ 00054 //Short to VCC 00055 return 0.4; 00056 }else{ 00057 return 0.5; 00058 }*/ 00059 }else{ 00060 //Integer value of temperature 00061 value = (tempProbeHigh<< 6 | tempProbeLow>>2); 00062 00063 //Get actual temperature (last 2 bits of integer are decimal 0.5 and 0.25) 00064 //temp = (value*0.15); 00065 temp = (value*0.25); // Multiply the value by 0.25 to get temp in C or 00066 // * (9.0/5.0)) + 32.0; // Convert value to F (ensure proper floats!) 00067 if(temp > 2500){ 00068 temp = 0; 00069 } 00070 return temp; 00071 } 00072 }else{ 00073 //Chip not ready for reading 00074 return -1; 00075 } 00076 } 00077 00078 void max31855::select() { 00079 //Set CS low to start transmission (interrupts conversion) 00080 ncs = 0; 00081 } 00082 00083 void max31855::deselect() { 00084 //Set CS high to stop transmission (restarts conversion) 00085 ncs = 1; 00086 //Reset conversion timer 00087 pollTimer.reset(); 00088 } 00089 00090 void max31855::initialise(int setType) { 00091 //Start the conversion timer 00092 pollTimer.start(); 00093 faultCode=0; 00094 } 00095 00096 int max31855::ready() { 00097 //Check to see if conversion is complete 00098 if (pollTimer.read_ms() > 250) { 00099 //Conversion complete 00100 return 1; 00101 }else{ 00102 //Conversion incomplete 00103 return 0; 00104 } 00105 }
Generated on Sun Jul 17 2022 03:47:11 by
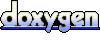