
Final
Dependencies: DebounceIn NokiaLCD WiflyInterface mbed
Fork of Websocket_Wifly_HelloWorld by
main.cpp
00001 #include "mbed.h" 00002 #include "WiflyInterface.h" 00003 #include "NokiaLCD.h" 00004 #include "picojpeg.h" 00005 #include "DebounceIn.h" 00006 #include "GPS.h" 00007 #include <vector> 00008 00009 #define DEFAULTZOOM 15 00010 00011 /* wifly interface: 00012 * - p13 and p14 are for the serial communication 00013 * - p28 is for the reset pin 00014 * - p27 is for the connection status 00015 * - 5th field is the ssid of the network 00016 * - 6th field is the password 00017 * - WPA is the security 00018 */ 00019 00020 WiflyInterface wifly(p13, p14, p28, p27, "username", "password", WPA); 00021 Serial pc(USBTX,USBRX); 00022 LocalFileSystem local("local");// Create the local filesystem under the name "local" 00023 GPS gps(p9, p10); 00024 DebounceIn ZoomIn(p18); 00025 DebounceIn Refresh(p19); 00026 DebounceIn ZoomOut(p20); 00027 FILE *jpegfile; 00028 int jpeg_filesize = 0; 00029 int jpeg_filepos = 0; 00030 NokiaLCD lcd(p5, p7, p8, p11, NokiaLCD::LCD6610); 00031 int zoom = DEFAULTZOOM; 00032 00033 00034 unsigned char pjpeg_need_bytes_callback(unsigned char* pBuf, unsigned char buf_size, unsigned char *pBytes_actually_read, void *pCallback_data) 00035 { 00036 unsigned int n = min((unsigned int)(jpeg_filesize - jpeg_filepos), (unsigned int)buf_size); 00037 if (n && (fread(pBuf, 1, n, jpegfile) != n)) 00038 return PJPG_STREAM_READ_ERROR; 00039 *pBytes_actually_read = (unsigned char)(n); 00040 jpeg_filepos += n; 00041 return 0; 00042 } 00043 00044 void ReadJPEGFromFile(const char *filename, NokiaLCD *lcd) 00045 { 00046 pjpeg_image_info_t imageInfo; 00047 jpegfile = fopen(filename,"rb"); 00048 if(!jpegfile) { 00049 pc.printf("File not Found: %s\n\r",filename); 00050 } 00051 fseek(jpegfile, 0, SEEK_END); 00052 jpeg_filesize = ftell(jpegfile); 00053 jpeg_filepos = 0; 00054 fseek(jpegfile, 0, SEEK_SET); 00055 int status = pjpeg_decode_init(&imageInfo, pjpeg_need_bytes_callback, NULL); 00056 //const unsigned int row_pitch = imageInfo.m_width * imageInfo.m_comps; 00057 int mcu_x = 0; 00058 int mcu_y = 0; 00059 00060 for ( ; ; ) { 00061 status = pjpeg_decode_mcu(); 00062 00063 if (status) { 00064 if (status != PJPG_NO_MORE_BLOCKS) { 00065 //pc.printf("pjpeg_decode_mcu() failed with status %u\n", status); 00066 fclose(jpegfile); 00067 return; 00068 } 00069 00070 break; 00071 } 00072 00073 if (mcu_y >= imageInfo.m_MCUSPerCol) { 00074 fclose(jpegfile); 00075 return; 00076 } 00077 00078 // Copy MCU's pixel blocks into the destination bitmap. 00079 00080 for (int y = 0; y < imageInfo.m_MCUHeight; y += 8) { 00081 const int by_limit = min(8, imageInfo.m_height - (mcu_y * imageInfo.m_MCUHeight + y)); 00082 for (int x = 0; x < imageInfo.m_MCUWidth; x += 8) { 00083 00084 unsigned int src_ofs = (x * 8U) + (y * 16U); 00085 const unsigned char *pSrcR = imageInfo.m_pMCUBufR + src_ofs; 00086 const unsigned char *pSrcG = imageInfo.m_pMCUBufG + src_ofs; 00087 const unsigned char *pSrcB = imageInfo.m_pMCUBufB + src_ofs; 00088 00089 const int bx_limit = min(8, imageInfo.m_width - (mcu_x * imageInfo.m_MCUWidth + x)); 00090 00091 if (imageInfo.m_scanType == PJPG_GRAYSCALE) { 00092 for (int by = 0; by < by_limit; by++) { 00093 for (int bx = 0; bx < bx_limit; bx++) { 00094 unsigned int color = ((*pSrcR++) << 16); 00095 (*lcd).pixel(mcu_x*imageInfo.m_MCUWidth+x+bx,mcu_y*imageInfo.m_MCUHeight+y+by,color); 00096 } 00097 pSrcR += (8 - bx_limit); 00098 } 00099 } else { 00100 for (int by = 0; by < by_limit; by++) { 00101 for (int bx = 0; bx < bx_limit; bx++) { 00102 unsigned int color = ((*pSrcR++) << 16) | ((*pSrcG++) << 8) | (*pSrcB++); 00103 (*lcd).pixel((130-imageInfo.m_width)/2+mcu_x*imageInfo.m_MCUWidth+x+bx,(130-imageInfo.m_height)/2+mcu_y*imageInfo.m_MCUHeight+y+by,color); 00104 } 00105 00106 pSrcR += (8 - bx_limit); 00107 pSrcG += (8 - bx_limit); 00108 pSrcB += (8 - bx_limit); 00109 00110 } 00111 } 00112 } 00113 } 00114 00115 mcu_x++; 00116 if (mcu_x == imageInfo.m_MCUSPerRow) { 00117 mcu_x = 0; 00118 mcu_y++; 00119 } 00120 } 00121 00122 fclose(jpegfile); 00123 } 00124 00125 void wiflyConnect() 00126 { 00127 wifly.disconnect(); 00128 wifly.init(); 00129 while (!wifly.connect()) { 00130 pc.printf("waiting\n\r"); 00131 }// join the network 00132 } 00133 00134 void getNewImage(float latitude, float longitude, int zoom) 00135 { 00136 TCPSocketConnection sock; 00137 sock.connect("maps.googleapis.com", 80); 00138 int ret = 0; 00139 int total_bytes = 0; 00140 char http_cmd[300]; 00141 sprintf(http_cmd, "GET /maps/api/staticmap?center=%f%%2C%f&zoom=%d&size=130x130&sensor=true&format=jpg-baseline HTTP/1.0\n\n",latitude,longitude,zoom); 00142 sock.send_all(http_cmd, sizeof(http_cmd)-1); 00143 00144 sock.set_blocking(false,10000); 00145 char buffer[300]; 00146 FILE* pFile; 00147 pc.printf("%s\n\r",http_cmd); 00148 pFile = fopen ("/local/image.jpg","w"); 00149 pc.printf("Opening File\n\r"); 00150 bool found_start = false; 00151 bool found_end = false; 00152 while (true) { 00153 ret = sock.receive(buffer, sizeof(buffer)-1); 00154 if (ret <= 0) { 00155 pc.printf("Ret < 0. Break. \n\r"); 00156 //wiflyConnect(); 00157 break; 00158 } 00159 buffer[ret] = '\0'; 00160 total_bytes += ret; 00161 pc.printf("Received %d chars from server, total %d: %s\n\r", ret,total_bytes, buffer); 00162 if(found_start) { 00163 for(int i=1; i<ret; i++) { 00164 if(buffer[i-1]==0xFF && buffer[i]==0xD9) { //jpg file end at FFD9 00165 pc.printf("found end. \n\r"); 00166 fwrite(buffer,1,i+1,pFile); 00167 found_end = true; 00168 break; 00169 } 00170 } 00171 if(!found_end) 00172 fwrite(buffer,1,ret,pFile); 00173 else { 00174 pc.printf("Found Start and End. Break. \n\r"); 00175 break; 00176 } 00177 00178 } else { 00179 for(int i=1; i<ret; i++) { 00180 if(buffer[i-1]==0xFF && buffer[i]==0xD8) { //jpg file starts from FFD8 00181 pc.printf("found start.\n\r"); 00182 fwrite(&buffer[i-1],1,ret-i+1,pFile); 00183 found_start = true; 00184 break; 00185 } 00186 } 00187 } 00188 } 00189 00190 fclose (pFile); 00191 pc.printf("Closing File\n\r"); 00192 sock.close(); 00193 lcd.cls(); 00194 ReadJPEGFromFile("/local/IMAGE.JPG", &lcd); 00195 } 00196 00197 int main() 00198 { 00199 pc.printf("Hello\n\r"); 00200 wifly.init(); //Use DHCP 00201 while (!wifly.connect()) { 00202 pc.printf("waiting\n\r"); 00203 }// join the network 00204 pc.printf("IP Address is %s\n", wifly.getIPAddress()); 00205 00206 float longitude = 0.0; 00207 float latitude = 0.0; 00208 while(1) { 00209 if(gps.sample()) { 00210 // pc.printf("I'm at %f, %f\n", gps.longitude, gps.latitude); 00211 longitude = gps.longitude; 00212 latitude = gps.latitude; 00213 } else { 00214 pc.printf("Oh Dear! No lock :(\n"); 00215 } 00216 if(Refresh) 00217 getNewImage(latitude,longitude,zoom); 00218 else if(ZoomIn) 00219 getNewImage(latitude,longitude,++zoom); 00220 else if(ZoomOut) 00221 getNewImage(latitude,longitude,--zoom); 00222 } 00223 wifly.disconnect(); 00224 }
Generated on Wed Jul 13 2022 05:24:07 by
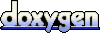