
You will be able to control a yellow ball through a level like a labyrinth
Dependencies: BSP_DISCO_F746NG LCD_DISCO_F746NG MMA7660FC TS_DISCO_F746NG mbed
main.cpp
00001 #include "mbed.h" 00002 #include "TS_DISCO_F746NG.h" 00003 #include "LCD_DISCO_F746NG.h" 00004 #include <MMA7660FC.h> 00005 00006 #define SLAVE_ADRESS 0x4c 00007 //---USER---// 00008 int level = 1; //Actual Level 00009 int old_charac[2] = {0,0}; //Old Position 00010 int charac[2] = {0,0}; //Actual Position on the grid 00011 float origin[3] = {0.0,0.0,0.0}; //Sensor Position 00012 bool drawn = 0; //Is Character drawn ? 00013 //---BUTTONS---// 00014 int btnNext[4] = {415, 35,465, 85};//Position of the buttons 00015 int btnPrev[4] = {415,115,465,165};// 00016 int btnReset[4] = {415,207,465,257};// 00017 //---labyrinth---// 00018 //Size : 16 * 10 00019 //Square Size : 25 * 25 00020 bool labyrinth1[160] = { 1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1 , 00021 1,0,0,0,1,0,1,0,0,0,1,0,0,0,0,1 , 00022 1,0,1,0,1,0,1,0,1,0,1,0,1,1,0,1 , 00023 0,0,1,1,1,0,1,1,1,0,1,0,1,0,0,1 , 00024 1,0,1,0,0,0,0,0,1,0,1,0,0,1,1,1 , 00025 1,0,0,0,1,0,1,0,0,0,1,1,0,0,0,1 , 00026 1,1,1,0,1,0,1,0,1,1,0,0,0,1,0,0 , 00027 1,0,0,0,0,0,0,0,0,0,1,1,0,1,0,1 , 00028 1,0,1,0,0,1,0,0,1,0,0,0,0,1,0,1 , 00029 1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1 00030 }; 00031 bool labyrinth2[160] = { 1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1 , 00032 1,0,0,0,1,0,1,0,0,0,0,0,0,0,1,1 , 00033 1,0,1,0,0,0,1,0,1,0,1,0,1,0,0,1 , 00034 1,0,1,0,1,0,0,0,1,0,1,1,1,1,0,1 , 00035 1,0,0,0,1,1,1,1,1,0,0,0,0,0,0,1 , 00036 1,0,1,1,1,0,0,0,0,0,1,1,0,1,0,1 , 00037 1,0,0,0,1,0,1,1,1,0,1,0,0,0,0,1 , 00038 1,0,1,0,0,0,0,0,1,0,1,0,1,1,0,1 , 00039 1,0,0,0,1,0,1,0,1,0,0,0,0,0,0,1 , 00040 1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1 00041 }; 00042 00043 00044 bool* labyrinths[2] = {labyrinth1, labyrinth2}; // All labyrinths in one tab 00045 //---PIN SETUP 00046 MMA7660FC sensor(PB_9,PB_8,SLAVE_ADRESS<<1); // Init Sensor 00047 LCD_DISCO_F746NG lcd; // Init LCD 00048 TS_DISCO_F746NG ts; // Init TouchScreen 00049 00050 //---DECLARATION_FUNCTIONS 00051 void checkPos(int,int); 00052 void displayAcc(float,float,float); 00053 void displayCharPos(); 00054 void displayPos(int,int,int); 00055 void displayOrientation(const char*); 00056 void drawChar(); 00057 void drawRect(int,int,int,int); 00058 void drawLabyrinth(int); 00059 void drawLevel(bool[]); 00060 void drawOptions(); 00061 void drawUi(); 00062 void drawUiButtons(); 00063 void drawUiStructure(); 00064 void initLCD(); 00065 void updateChar(float,float,float,bool[]); 00066 void resetPos(); 00067 void nextLvl(); 00068 void prevLvl(); 00069 void isWinning(int); 00070 00071 //---INITIALISATION_FUNCTIONS 00072 /** Function checkPos 00073 * Checking actual position of a finger on the touchscreen 00074 */ 00075 void checkPos(int x, int y) 00076 { 00077 if(x > btnReset[0] && y > btnReset[1] && x < btnReset[2] && y < btnReset[3]) { 00078 resetPos(); 00079 } else if(x > btnNext[0] && y > btnNext[1] && x < btnNext[2] && y < btnNext[3]) { 00080 nextLvl(); 00081 } else if(x > btnPrev[0] && y > btnPrev[1] && x < btnPrev[2] && y < btnPrev[3]) { 00082 prevLvl(); 00083 } 00084 } 00085 /** Function displayAcc 00086 * Displays the actual acceleration of the sensor (not used) 00087 */ 00088 void displayAcc(float x, float y, float z) 00089 { 00090 uint8_t color = lcd.GetTextColor(); 00091 lcd.SetTextColor(LCD_COLOR_BLACK); 00092 char string[50]; 00093 sprintf(string,"AX = %3.3f",x); 00094 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)string, CENTER_MODE); 00095 sprintf(string,"AY = %3.3f",y); 00096 lcd.DisplayStringAt(0, LINE(6), (uint8_t *)string, CENTER_MODE); 00097 sprintf(string,"AZ = %3.3f",z); 00098 lcd.DisplayStringAt(0, LINE(7), (uint8_t *)string, CENTER_MODE); 00099 lcd.SetTextColor(color); 00100 } 00101 /** Function displayCharPos 00102 * Displays the actual position of the character (not used) 00103 */ 00104 void displayCharPos() 00105 { 00106 uint8_t color = lcd.GetTextColor(); 00107 lcd.SetTextColor(LCD_COLOR_BLACK); 00108 char string[50]; 00109 sprintf(string,"X = %3d",charac[0]); 00110 lcd.DisplayStringAt(0, LINE(8), (uint8_t *)string, CENTER_MODE); 00111 sprintf(string,"Y = %3d",charac[1]); 00112 lcd.DisplayStringAt(0, LINE(9), (uint8_t *)string, CENTER_MODE); 00113 lcd.SetTextColor(color); 00114 } 00115 /** Function displayOri 00116 * Displays the origin acceleration of the sensor (not used) 00117 */ 00118 void displayOri() 00119 { 00120 uint8_t color = lcd.GetTextColor(); 00121 lcd.SetTextColor(LCD_COLOR_BLACK); 00122 char string[50]; 00123 sprintf(string,"AX = %3.3f",origin[0]); 00124 lcd.DisplayStringAt(0, LINE(1), (uint8_t *)string, CENTER_MODE); 00125 sprintf(string,"AY = %3.3f",origin[1]); 00126 lcd.DisplayStringAt(0, LINE(2), (uint8_t *)string, CENTER_MODE); 00127 sprintf(string,"AZ = %3.3f",origin[2]); 00128 lcd.DisplayStringAt(0, LINE(3), (uint8_t *)string, CENTER_MODE); 00129 lcd.SetTextColor(color); 00130 } 00131 /** Function displayOrientation 00132 * Displays the actual orientation of the sensor (not used) 00133 */ 00134 void displayOrientation(const char *o) 00135 { 00136 uint8_t color = lcd.GetTextColor(); 00137 lcd.SetTextColor(LCD_COLOR_BLACK); 00138 char string[50]; 00139 sprintf(string,"O = %s",o); 00140 lcd.DisplayStringAt(0, LINE(4), (uint8_t *)string, CENTER_MODE); 00141 lcd.SetTextColor(color); 00142 } 00143 /** Function displayPos 00144 * Displays the actual position of the sensor (not used) 00145 */ 00146 void displayPos(int x, int y, int z) 00147 { 00148 uint8_t color = lcd.GetTextColor(); 00149 lcd.SetTextColor(LCD_COLOR_BLACK); 00150 char string[50]; 00151 sprintf(string,"X = %03d",x); 00152 lcd.DisplayStringAt(0, LINE(1), (uint8_t *)string, CENTER_MODE); 00153 sprintf(string,"Y = %03d",y); 00154 lcd.DisplayStringAt(0, LINE(2), (uint8_t *)string, CENTER_MODE); 00155 sprintf(string,"Z = %03d",z); 00156 lcd.DisplayStringAt(0, LINE(3), (uint8_t *)string, CENTER_MODE); 00157 lcd.SetTextColor(color); 00158 } 00159 /** Function drawChar 00160 * Draws the character on the labyrinth 00161 */ 00162 void drawChar() 00163 { 00164 if((old_charac[0] != charac[0] || old_charac[1] != charac[1])||(drawn == 0)) { 00165 uint8_t color = lcd.GetTextColor(); 00166 lcd.SetTextColor(LCD_COLOR_WHITE); 00167 lcd.FillCircle(old_charac[0]*25+11+12,old_charac[1]*25+10+12,11); 00168 lcd.SetTextColor(LCD_COLOR_YELLOW); 00169 lcd.FillCircle(charac[0]*25+11+12,charac[1]*25+10+12,11); 00170 lcd.SetTextColor(color); 00171 old_charac[0] = charac[0]; 00172 old_charac[1] = charac[1]; 00173 drawn = 1; 00174 } 00175 } 00176 /** Function drawLabyrinth 00177 * Sets the position of the character and calls drawLevel() 00178 */ 00179 void drawLabyrinth(int lvl) 00180 { 00181 switch(lvl) { 00182 case 1: 00183 drawLevel(labyrinth1); 00184 charac[0]= 0; 00185 charac[1]= 3; 00186 old_charac[0]= 0; 00187 old_charac[1]= 3; 00188 break; 00189 case 2: 00190 drawLevel(labyrinth2); 00191 charac[0]= 7; 00192 charac[1]= 0; 00193 old_charac[0]= 7; 00194 old_charac[1]= 0; 00195 break; 00196 default: 00197 break; 00198 } 00199 } 00200 /** Function drawLevel 00201 * Displays the labyrinth on the screen 00202 */ 00203 void drawLevel(bool labyrinth[]) 00204 { 00205 uint8_t color = lcd.GetTextColor(); 00206 for(int i = 0; i<10; i++) { 00207 for(int j = 0; j<16; j++) { 00208 if(labyrinth[j+(i*16)] == 1) { 00209 lcd.SetTextColor(LCD_COLOR_BLACK); 00210 lcd.FillRect(11+(j*25),10+(i*25), 25, 25); 00211 } else if(labyrinth[j+(i*16)] == 0) { 00212 lcd.SetTextColor(LCD_COLOR_WHITE); 00213 lcd.FillRect(11+(j*25),10+(i*25), 25, 25); 00214 } 00215 } 00216 } 00217 lcd.SetTextColor(color); 00218 } 00219 /** Function drawLevelNbr 00220 * Displays the level number on the options menu 00221 */ 00222 void drawLevelNbr() 00223 { 00224 uint8_t color = lcd.GetTextColor(); 00225 sFONT *font = lcd.GetFont(); 00226 sFONT f = Font20; 00227 char str[50]; 00228 00229 lcd.SetFont(&f); 00230 lcd.SetTextColor(LCD_COLOR_BLACK); 00231 sprintf(str,"%d",level); 00232 lcd.DisplayStringAt(440-8, 100-7,(uint8_t *) str, LEFT_MODE); 00233 00234 lcd.SetFont(font); 00235 lcd.SetTextColor(color); 00236 } 00237 /** Function drawOptions 00238 * Displays the option tab 00239 */ 00240 void drawOptions() 00241 { 00242 uint8_t color = lcd.GetTextColor(); 00243 sFONT *font = lcd.GetFont(); 00244 sFONT f = Font16; 00245 lcd.SetFont(&f); 00246 uint8_t str[6] = "Level"; 00247 lcd.SetTextColor(LCD_COLOR_BLACK); 00248 lcd.DisplayStringAt(414, 15, str, LEFT_MODE); 00249 lcd.SetFont(font); 00250 drawUiButtons(); 00251 drawLevelNbr(); 00252 lcd.SetTextColor(color); 00253 } 00254 /** Function drawRect 00255 * Displays a rectangle on the screen 00256 */ 00257 void drawRect(int x1, int y1, int x2, int y2) 00258 { 00259 uint8_t color = lcd.GetTextColor(); 00260 lcd.SetTextColor(LCD_COLOR_BLACK); 00261 lcd.DrawRect(x1, y1, x2-x1, y2-y1); 00262 lcd.SetTextColor(color); 00263 } 00264 /** Function drawUi 00265 * Draws the UI 00266 */ 00267 void drawUi() 00268 { 00269 drawUiStructure(); 00270 drawOptions(); 00271 } 00272 /** Function drawUiButtons 00273 * Draws buttons on the option tab 00274 */ 00275 void drawUiButtons() 00276 { 00277 uint8_t color = lcd.GetTextColor(); 00278 lcd.SetTextColor(LCD_COLOR_BLUE); 00279 drawRect(415, 35,465, 85); // Next Level 00280 lcd.SetTextColor(LCD_COLOR_BLACK); 00281 lcd.DisplayChar(440-8,50,'N'); 00282 00283 lcd.SetTextColor(LCD_COLOR_BLUE); 00284 drawRect(415,115,465,165); // Previous Level 00285 lcd.SetTextColor(LCD_COLOR_BLACK); 00286 lcd.DisplayChar(440-8,130,'P'); 00287 00288 lcd.SetTextColor(LCD_COLOR_BLUE); 00289 drawRect(415,207,465,257); // Reset Pos 00290 lcd.SetTextColor(LCD_COLOR_BLACK); 00291 lcd.DisplayChar(440-8,232-10,'R'); 00292 lcd.SetTextColor(color); 00293 } 00294 /** Function drawUiStructure 00295 * Draws rectangle on the UI as delimiters 00296 */ 00297 void drawUiStructure() 00298 { 00299 uint8_t color = lcd.GetTextColor(); 00300 lcd.SetTextColor(LCD_COLOR_BLACK); 00301 drawRect( 11, 10,411,260); //Partie labyrinth 00302 drawRect(411, 10,470,260); //Partie Utilisateur 00303 lcd.SetTextColor(color); 00304 } 00305 /** Function initLCD 00306 * Initializes the LCD 00307 */ 00308 void initLCD() 00309 { 00310 lcd.Clear(LCD_COLOR_WHITE); 00311 //lcd.SetBackColor(LCD_COLOR_BLUE); 00312 lcd.SetTextColor(LCD_COLOR_BLACK); 00313 wait(0.3); 00314 } 00315 /** Function updateChar 00316 * Updates the position of the character if 00317 * - The sensor is in the range 00318 * - The character is nearby a white square 00319 * - The direction is oriented to a white square 00320 * Checks if the player has won the level 00321 */ 00322 void updateChar(float ax, float ay, float az, bool lab[]) 00323 { 00324 float oldX = origin[0], oldY = origin[1], oldZ = origin[2]; 00325 00326 //Sensor is bended downwards 00327 if ((az >= oldZ+30) || (az <= oldZ-30)) { 00328 //Sensor is bended left 00329 if (ax <= oldX-30) { 00330 if (!(charac[0] <= 0) && (lab[16*charac[1]+charac[0]-1] == 0)) { 00331 charac[0]--; 00332 } 00333 } 00334 //Sensor is bended right 00335 else if (ax >= oldX+30) { 00336 if (!(charac[0] >= 15) && (lab[16*charac[1]+charac[0]+1] == 0)) { 00337 charac[0]++; 00338 } 00339 } 00340 //Sensor is bended forward 00341 else if (ay <= oldY-30) { 00342 if (!(charac[1] <= 0) && (lab[16*charac[1]+charac[0]-16] == 0)) { 00343 charac[1]--; 00344 } 00345 } 00346 //Sensor is bended backward 00347 else if (ay >= oldX+30) { 00348 if (!(charac[1] >= 9) && (lab[16*charac[1]+charac[0]+16] == 0)) { 00349 charac[1]++; 00350 } 00351 } 00352 } 00353 00354 isWinning(level); 00355 //charac[0] = {x}; should be used to update character position on x 00356 //charac[1] = {y}; should be used to update character position on y 00357 } 00358 /** Function resetPos 00359 * Reset the original acceleration of the sensor 00360 */ 00361 void resetPos() 00362 { 00363 float ax=0,ay=0,az=0; 00364 sensor.read_Tilt(&ax,&ay,&az); 00365 origin[0] = ax; 00366 origin[1] = ay; 00367 origin[2] = az; 00368 } 00369 /** Function nextLvl 00370 * Loads the next level 00371 */ 00372 void nextLvl() 00373 { 00374 if(level < 2) 00375 level++; 00376 drawLabyrinth(level); 00377 drawLevelNbr(); 00378 drawn = 0; 00379 drawChar(); 00380 } 00381 /** Function prevLvl 00382 * Loads the previous level 00383 */ 00384 void prevLvl() 00385 { 00386 if(level > 1) 00387 level--; 00388 drawLabyrinth(level); 00389 drawLevelNbr(); 00390 drawn = 0; 00391 drawChar(); 00392 } 00393 /** Function isWinning 00394 * Checks if the player has won the level 00395 */ 00396 void isWinning(int lvl) 00397 { 00398 switch(lvl) { 00399 case 1: 00400 if (charac[0]== 15 && charac[1]== 6) 00401 nextLvl(); 00402 break; 00403 case 2: 00404 if (charac[0]== 7 && charac[1]== 9) 00405 nextLvl(); 00406 break; 00407 default: 00408 break; 00409 } 00410 } 00411 //---MAIN 00412 int main() 00413 { 00414 //Declaration 00415 int x, y, i=0; 00416 float ax=0,ay=0,az=0; 00417 //Initialization 00418 sensor.init(); 00419 initLCD(); 00420 ts.Init(lcd.GetXSize(), lcd.GetYSize()); 00421 TS_StateTypeDef TS_State; 00422 //Setting up the UI 00423 drawUi(); 00424 drawLabyrinth(level); 00425 00426 //Setting up the sensor configuration 00427 sensor.write_reg(0x07,0); 00428 sensor.write_reg(0x04,0x000); 00429 sensor.write_reg(0x07,1); 00430 00431 //Setting original acceleration 00432 resetPos(); 00433 00434 while(1) { 00435 //Getting Values 00436 sensor.read_Tilt(&ax,&ay,&az); 00437 //Getting touchscreen state 00438 ts.GetState(&TS_State); 00439 //Getting pos on the touchscreen 00440 x = TS_State.touchX[0]; 00441 y = TS_State.touchY[0]; 00442 00443 //Check Pos 00444 if(TS_State.touchDetected>0) 00445 checkPos(x,y); 00446 00447 //If timeout updating character position 00448 if(i > 6) { 00449 updateChar(ax,ay,az,labyrinths[level-1]); 00450 i = -1; 00451 } 00452 00453 //---DEBUG 00454 //Printing Values 00455 /*displayAcc(ax,ay,az); 00456 displayCharPos(); 00457 displayOri();//*/ 00458 00459 drawChar(); 00460 i++; 00461 wait_ms(100); 00462 } 00463 }
Generated on Thu Sep 1 2022 21:17:06 by
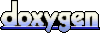