Mbed OS version of IoT.js implementation running on GR-PEACH
Embed:
(wiki syntax)
Show/hide line numbers
peripheral_io_4mbed.h
00001 /* Copyright 2017-present Renesas Electronics Corporation and other contributors 00002 * 00003 * Licensed under the Apache License, Version 2.0 (the "License"); 00004 * you may not use this file except in compliance with the License. 00005 * You may obtain a copy of the License at 00006 * 00007 * http://www.apache.org/licenses/LICENSE-2.0 00008 * 00009 * Unless required by applicable law or agreed to in writing, software 00010 * distributed under the License is distributed on an "AS IS" BASIS 00011 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 * See the License for the specific language governing permissions and 00013 * limitations under the License. 00014 */ 00015 00016 #ifndef PERIPHERAL_IO_4MBED_INCL 00017 #define PERIPHERAL_IO_4MBED_INCL 00018 00019 #ifdef __cplusplus 00020 extern "C" { 00021 #endif 00022 00023 #define P4_API(type,func) type P4(func) 00024 #define VIDEO_API P4_API 00025 #define DISPLAY_API P4_API 00026 #define JPEG_API P4_API 00027 #define GRAPHICS_API P4_API 00028 00029 // VIDEO 00030 typedef void* P4(video_h); 00031 00032 typedef enum { 00033 VIDEO_PIXELFORMAT_YCBCR422, 00034 VIDEO_PIXELFORMAT_RGB565, 00035 VIDEO_PIXELFORMAT_RGB888, 00036 VIDEO_PIXELFORMAT_MAX, 00037 } video_pixel_format_t; 00038 00039 typedef enum { 00040 DEVICETYPE_CMOSCAMERA, 00041 DEVICETYPE_COMPOSITESIGNAL, 00042 DEVICETYPE_MAX, 00043 } device_type_t; 00044 00045 typedef enum { 00046 CAMERATYPE_OV7725, 00047 CAMERATYPE_MAX, 00048 } camera_type_t; 00049 00050 typedef enum { 00051 CHANNEL_0, 00052 CHANNEL_1, 00053 CHANNEL_MAX, 00054 } channel_num_t; 00055 00056 typedef enum { 00057 SIGNALFORMAT_NTSC, 00058 SIGNALFORMAT_PAL, 00059 SIGNALFORMAT_MAX, 00060 } signal_format_t; 00061 00062 typedef struct { 00063 uint32_t width; 00064 uint32_t height; 00065 uint32_t pixel_bytes; 00066 uint8_t* frame_buffer; 00067 00068 device_type_t device; 00069 camera_type_t camera; 00070 video_pixel_format_t pixel_format; 00071 signal_format_t signal_format; 00072 channel_num_t channel; 00073 } video_source_t; 00074 00075 VIDEO_API(P4(video_h), video_open(video_source_t video_source)); 00076 VIDEO_API(bool, video_start(P4(video_h) handle, void* buf)); 00077 VIDEO_API(bool, video_stop(P4(video_h) handle)); 00078 VIDEO_API(bool, video_close(P4(video_h) handle)); 00079 00080 // DISPLAY(LCD) 00081 typedef void* P4(lcd_h); 00082 00083 typedef enum { 00084 DISPLAY_PIXELFORMAT_YCBCR422, 00085 DISPLAY_PIXELFORMAT_RGB565, 00086 DISPLAY_PIXELFORMAT_RGB888, 00087 DISPLAY_PIXELFORMAT_ARGB8888, 00088 DISPLAY_PIXELFORMAT_ARGB4444, 00089 DISPLAY_PIXELFORMAT_MAX, 00090 } display_pixel_format_t; 00091 00092 typedef enum { 00093 LCDTYPE_4_3INCH, 00094 LCDTYPE_MAX, 00095 } lcd_type_t; 00096 00097 typedef enum { 00098 LCDLAYER_0, 00099 LCDLAYER_1, 00100 LCDLAYER_2, 00101 LCDLAYER_3, 00102 LCDLAYER_MAX, 00103 } lcd_layer_id_t; 00104 00105 typedef struct { 00106 lcd_layer_id_t id; 00107 display_pixel_format_t buffer_format; 00108 uint32_t pixel_bytes; 00109 uint8_t* frame_buffer; 00110 } lcd_layer_t; 00111 00112 typedef struct { 00113 lcd_type_t type; 00114 00115 uint32_t width; 00116 uint32_t height; 00117 } lcd_t; 00118 00119 DISPLAY_API(P4(lcd_h), lcd_open(lcd_t *lcd)); 00120 DISPLAY_API(bool, lcd_close(P4(lcd_h) handle)); 00121 DISPLAY_API(bool, lcd_start(P4(lcd_h) handle, lcd_layer_t lcd_layer)); 00122 DISPLAY_API(bool, lcd_stop(P4(lcd_h) handle, lcd_layer_id_t id)); 00123 DISPLAY_API(bool, lcd_update(P4(lcd_h) handle, lcd_layer_t lcd_layer)); 00124 00125 // JPEG 00126 typedef void* P4(jpeg_h); 00127 00128 typedef enum { 00129 JPEG_PIXELFORMAT_YCBCR422, /* encode, decode */ 00130 JPEG_PIXELFORMAT_ARGB8888, /* decode */ 00131 JPEG_PIXELFORMAT_RGB565, /* decode */ 00132 JPEG_PIXELFORMAT_MAX, 00133 } jpeg_pixel_format_t; 00134 00135 typedef struct { 00136 uint32_t width; 00137 uint32_t height; 00138 jpeg_pixel_format_t pixel_format; 00139 uint32_t alpha; 00140 00141 struct { 00142 uint32_t len; 00143 uint8_t* buf; 00144 } src, dst; 00145 } jpeg_convert_data_t; 00146 00147 JPEG_API(int, jpeg_encode(jpeg_convert_data_t encode_data)); 00148 JPEG_API(bool, jpeg_decode(jpeg_convert_data_t decode_data)); 00149 00150 // GRAPHICS 00151 typedef void* P4(graphics_h); 00152 00153 GRAPHICS_API(P4(graphics_h), initFrameBuffer(char *buf, int width, int height, int format)); 00154 GRAPHICS_API(int, deinitFrameBuffer(P4(graphics_h) handle)); 00155 GRAPHICS_API(int, drawLine(P4(graphics_h) handle, int startX, int startY, int endX, int endY, uint32_t color)); 00156 GRAPHICS_API(int, drawRect(P4(graphics_h) handle, int x, int y, int width, int height, uint32_t color, bool fill)); 00157 GRAPHICS_API(int, drawArc(P4(graphics_h) handle, int centerX, int centerY, int radius, int startAngle, int endAngle, uint32_t color)); 00158 GRAPHICS_API(int, drawCircle(P4(graphics_h) handle, int centerX, int centerY, int radius, uint32_t color, bool fill)); 00159 GRAPHICS_API(int, drawEllipse(P4(graphics_h) handle, int centerX, int centerY, int radiusX, int radiusY, uint32_t color, bool fill)); 00160 GRAPHICS_API(int, drawPolygon(P4(graphics_h) handle, int centerX, int centerY, int radius, int sides, uint32_t color, bool fill)); 00161 GRAPHICS_API(int, drawText(P4(graphics_h) handle, char* text, int x, int y, int size, uint32_t color, uint32_t background)); 00162 GRAPHICS_API(int, drawImage(P4(graphics_h) handle, char* image, int x, int y, int width, int height)); 00163 00164 #undef GRAPHICS_API 00165 #undef JPEG_API 00166 #undef DISPLAY_API 00167 #undef VIDEO_API 00168 00169 #if !defined( POSIX4_ITSELF ) 00170 00171 // functions 00172 #define video_open P4(video_open) 00173 #define video_start P4(video_start) 00174 #define video_stop P4(video_stop) 00175 #define video_close P4(video_close) 00176 #define lcd_open P4(lcd_open) 00177 #define lcd_close P4(lcd_close) 00178 #define lcd_start P4(lcd_start) 00179 #define lcd_stop P4(lcd_stop) 00180 #define lcd_update P4(lcd_update) 00181 #define jpeg_encode P4(jpeg_encode) 00182 #define jpeg_decode P4(jpeg_decode) 00183 00184 #define initFrameBuffer P4(initFrameBuffer) 00185 #define deinitFrameBuffer P4(deinitFrameBuffer) 00186 #define drawLine P4(drawLine) 00187 #define drawRect P4(drawRect) 00188 #define drawArc P4(drawArc) 00189 #define drawCircle P4(drawCircle) 00190 #define drawEllipse P4(drawEllipse) 00191 #define drawPolygon P4(drawPolygon) 00192 #define drawText P4(drawText) 00193 #define drawImage P4(drawImage) 00194 00195 // typedefs 00196 #define video_h P4(video_h) 00197 #define lcd_h P4(lcd_h) 00198 #define jpeg_h P4(jpeg_h) 00199 #define graphics_h P4(graphics_h) 00200 00201 #endif // #if !defined( POSIX4_ITSELF ) 00202 00203 #ifdef __cplusplus 00204 } 00205 #endif 00206 00207 #ifdef __cplusplus 00208 00209 namespace mbed { 00210 00211 namespace video { 00212 P4(video_h) open(video_source_t video_source); 00213 bool close(P4(video_h) handle); 00214 bool start(P4(video_h) handle, void* buf); 00215 bool stop(P4(video_h) handle); 00216 } // namespace video 00217 00218 namespace lcd { 00219 P4(lcd_h) open(lcd_t *lcd); 00220 bool close(P4(lcd_h) handle); 00221 bool start(P4(lcd_h) handle, lcd_layer_t lcd_layer); 00222 bool stop(P4(lcd_h) handle, lcd_layer_id_t id); 00223 bool update(P4(lcd_h) handle, lcd_layer_t id); 00224 } // namespace lcd 00225 00226 namespace jpeg { 00227 int encode(jpeg_convert_data_t encode_data); 00228 bool decode(jpeg_convert_data_t decode_data); 00229 } // namespace jpeg 00230 00231 namespace graphics { 00232 P4(graphics_h) initFrameBuffer(char *buf, int width, int height, int format); 00233 int deinitFrameBuffer(P4(graphics_h) handle); 00234 int drawLine(P4(graphics_h) handle, int startX, int startY, int endX, int endY, uint32_t color); 00235 int drawRect(P4(graphics_h) handle, int x, int y, int width, int height, uint32_t color, bool fill); 00236 int drawArc(P4(graphics_h) handle, int centerX, int centerY, int radius, int startAngle, int endAngle, uint32_t color); 00237 int drawCircle(P4(graphics_h) handle, int centerX, int centerY, int radius, uint32_t color, bool fill); 00238 int drawEllipse(P4(graphics_h) handle, int centerX, int centerY, int radiusX, int radiusY, uint32_t color, bool fill); 00239 int drawPolygon(P4(graphics_h) handle, int centerX, int centerY, int radius, int sides, uint32_t color, bool fill); 00240 int drawText(P4(graphics_h) handle, char* text, int x, int y, int size, uint32_t color, uint32_t background); 00241 int drawImage(P4(graphics_h) handle, char* image, int x, int y, int width, int height); 00242 } // namespace graphics 00243 00244 } // namespace mbed 00245 00246 using namespace mbed; 00247 00248 #endif 00249 00250 #endif // #ifndef PERIPHERAL_IO_4MBED_INCL
Generated on Wed Jul 13 2022 20:23:11 by
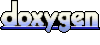