
Algorithmus
Embed:
(wiki syntax)
Show/hide line numbers
Controller.h
00001 #ifndef CONTROLLER_H_ 00002 #define CONTROLLER_H_ 00003 #include <cstdlib> 00004 #include <mbed.h> 00005 #include "EncoderCounter.h" 00006 #include "LowpassFilter.h" 00007 00008 class Controller 00009 { 00010 00011 public: 00012 00013 Controller(PwmOut& pwmLeft, PwmOut& pwmRight, 00014 EncoderCounter& counterLeft, EncoderCounter& counterRight); 00015 00016 virtual ~Controller(); 00017 void setDesiredSpeedLeft(float desiredSpeedLeft); 00018 void setDesiredSpeedRight(float desiredSpeedRight); 00019 float getSpeedLeft(); 00020 float getSpeedRight(); 00021 float getIntegralLeft(); 00022 float getIntegralRight(); 00023 float getProportionalLeft(); 00024 float getProportionalRight(); 00025 void counterReset(); 00026 00027 private: 00028 00029 static const float PERIOD; 00030 static const float COUNTS_PER_TURN; 00031 static const float LOWPASS_FILTER_FREQUENCY; 00032 static const float KN; 00033 static const float KP; 00034 static const float KI; 00035 static const float I_MAX; 00036 static const float MAX_VOLTAGE; 00037 static const float MIN_DUTY_CYCLE; 00038 static const float MAX_DUTY_CYCLE; 00039 00040 PwmOut& pwmLeft; 00041 PwmOut& pwmRight; 00042 EncoderCounter& counterLeft; 00043 EncoderCounter& counterRight; 00044 short previousValueCounterLeft; 00045 short previousValueCounterRight; 00046 LowpassFilter speedLeftFilter; 00047 LowpassFilter speedRightFilter; 00048 float desiredSpeedLeft; 00049 float desiredSpeedRight; 00050 float actualSpeedLeft; 00051 float actualSpeedRight; 00052 float iSumLeft; 00053 float iSumRight; 00054 Ticker ticker; 00055 00056 void run(); 00057 00058 }; 00059 00060 #endif /* CONTROLLER_H_ */ 00061 00062 00063 00064 00065
Generated on Wed Jul 13 2022 06:13:06 by
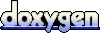