WIZNet W5500 with additional enhancements
Fork of WIZnetInterface by
EthernetInterface.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "EthernetInterface.h" 00020 #include "DHCPClient.h" 00021 00022 #if (not defined TARGET_WIZwiki_W7500) && (not defined TARGET_WIZwiki_W7500P) && (not defined TARGET_WIZwiki_W7500ECO) 00023 EthernetInterface::EthernetInterface(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset) : 00024 WIZnet_Chip(mosi, miso, sclk, cs, reset) 00025 { 00026 ip_set = false; 00027 domainName = NULL; 00028 leaseStart = 0; 00029 _hostname = NULL; 00030 } 00031 00032 EthernetInterface::EthernetInterface(SPI* spi, PinName cs, PinName reset) : 00033 WIZnet_Chip(spi, cs, reset) 00034 { 00035 ip_set = false; 00036 domainName = NULL; 00037 leaseStart = 0; 00038 _hostname = NULL; 00039 } 00040 #endif 00041 00042 EthernetInterface::~EthernetInterface() 00043 { 00044 if (domainName) 00045 free(domainName); 00046 } 00047 00048 int EthernetInterface::init() 00049 { 00050 dhcp = true; 00051 reset(); 00052 00053 return 0; 00054 } 00055 00056 int EthernetInterface::init(uint8_t *mac) 00057 { 00058 dhcp = true; 00059 00060 for (int i =0; i < 6; i++) 00061 this->mac[i] = mac[i]; 00062 00063 reset(); 00064 setmac(); 00065 setSIMR(0xff); // enable interrupts for all sockets 00066 return 0; 00067 } 00068 00069 int EthernetInterface::init(uint8_t * mac, const char* ip, const char* mask, const char* gateway, const char* dnsServer) 00070 { 00071 dhcp = false; 00072 // 00073 for (int i =0; i < 6; i++) this->mac[i] = mac[i]; 00074 // 00075 this->ip = str_to_ip(ip); 00076 strcpy(ip_string, ip); 00077 ip_set = true; 00078 this->netmask = str_to_ip(mask); 00079 this->gateway = str_to_ip(gateway); 00080 if (dnsServer) 00081 this->dnsaddr = str_to_ip(dnsServer); 00082 reset(); 00083 00084 // @Jul. 8. 2014 add code. should be called to write chip. 00085 setmac(); 00086 setip(); 00087 setSIMR(0xff); // enable interrupts for all sockets 00088 return 0; 00089 } 00090 00091 // Connect Bring the interface up, start DHCP if needed. 00092 int EthernetInterface::connect(const char *hostname) 00093 { 00094 if (hostname) 00095 _hostname = hostname; 00096 if (dhcp) { 00097 int r = IPrenew(); 00098 if (r < 0) { 00099 return r; 00100 } 00101 } 00102 if (WIZnet_Chip::setip() == false) return -1; 00103 return 0; 00104 } 00105 00106 // Disconnect Bring the interface down. 00107 int EthernetInterface::disconnect() 00108 { 00109 //if (WIZnet_Chip::disconnect() == false) return -1; 00110 return 0; 00111 } 00112 00113 00114 char* EthernetInterface::getIPAddress() 00115 { 00116 uint32_t ip = reg_rd<uint32_t>(SIPR); 00117 snprintf(ip_string, sizeof(ip_string), "%d.%d.%d.%d", 00118 (uint8_t)((ip>>24)&0xff), 00119 (uint8_t)((ip>>16)&0xff), 00120 (uint8_t)((ip>>8)&0xff), 00121 (uint8_t)(ip&0xff)); 00122 return ip_string; 00123 } 00124 00125 char* EthernetInterface::getNetworkMask() 00126 { 00127 uint32_t ip = reg_rd<uint32_t>(SUBR); 00128 snprintf(mask_string, sizeof(mask_string), "%d.%d.%d.%d", 00129 (uint8_t)((ip>>24)&0xff), 00130 (uint8_t)((ip>>16)&0xff), 00131 (uint8_t)((ip>>8)&0xff), 00132 (uint8_t)(ip&0xff)); 00133 return mask_string; 00134 } 00135 00136 char* EthernetInterface::getGateway() 00137 { 00138 uint32_t ip = reg_rd<uint32_t>(GAR); 00139 snprintf(gw_string, sizeof(gw_string), "%d.%d.%d.%d", 00140 (uint8_t)((ip>>24)&0xff), 00141 (uint8_t)((ip>>16)&0xff), 00142 (uint8_t)((ip>>8)&0xff), 00143 (uint8_t)(ip&0xff)); 00144 return gw_string; 00145 } 00146 00147 00148 char* EthernetInterface::getDNSServer() 00149 { 00150 uint32_t ip = getDNSAddr(); 00151 snprintf(dns_string, sizeof(gw_string), "%d.%d.%d.%d", 00152 (uint8_t)((ip>>24)&0xff), 00153 (uint8_t)((ip>>16)&0xff), 00154 (uint8_t)((ip>>8)&0xff), 00155 (uint8_t)(ip&0xff)); 00156 return dns_string; 00157 } 00158 00159 char* EthernetInterface::getMACAddress() 00160 { 00161 uint8_t mac[6]; 00162 reg_rd_mac(SHAR, mac); 00163 snprintf(mac_string, sizeof(mac_string), "%02X:%02X:%02X:%02X:%02X:%02X", mac[0], mac[1], mac[2], mac[3], mac[4], mac[5]); 00164 //ethernet_address(mac_string); 00165 return mac_string; 00166 00167 } 00168 00169 00170 int EthernetInterface::IPrenew(int timeout_ms) 00171 { 00172 DHCPClient dhcp; 00173 int err = dhcp.setup(_hostname, timeout_ms); 00174 if (err == (-1)) { 00175 return -1; 00176 } 00177 // printf("Connected, IP: %d.%d.%d.%d\n", dhcp.yiaddr[0], dhcp.yiaddr[1], dhcp.yiaddr[2], dhcp.yiaddr[3]); 00178 /* 00179 * Sync DHCP response variables 00180 */ 00181 ip = (dhcp.yiaddr[0] <<24) | (dhcp.yiaddr[1] <<16) | (dhcp.yiaddr[2] <<8) | dhcp.yiaddr[3]; 00182 gateway = (dhcp.gateway[0]<<24) | (dhcp.gateway[1]<<16) | (dhcp.gateway[2]<<8) | dhcp.gateway[3]; 00183 netmask = (dhcp.netmask[0]<<24) | (dhcp.netmask[1]<<16) | (dhcp.netmask[2]<<8) | dhcp.netmask[3]; 00184 dnsaddr = (dhcp.dnsaddr[0]<<24) | (dhcp.dnsaddr[1]<<16) | (dhcp.dnsaddr[2]<<8) | dhcp.dnsaddr[3]; 00185 timesrv = (dhcp.timesrv[0]<<24) | (dhcp.timesrv[1]<<16) | (dhcp.timesrv[2]<<8) | dhcp.timesrv[3]; 00186 leaseTime = (dhcp.leaseTime[0]<<24) | (dhcp.leaseTime[1]<<16) | (dhcp.leaseTime[2]<<8) | dhcp.leaseTime[3]; 00187 leaseStart = time(NULL); 00188 if (domainName) { 00189 free(domainName); 00190 domainName = NULL; 00191 } 00192 if (dhcp.domainName) { 00193 domainName = dhcp.domainName; 00194 dhcp.domainName = NULL; 00195 } 00196 return 0; 00197 } 00198
Generated on Tue Jul 12 2022 17:13:34 by
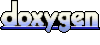