RadioShuttle Lib for the STM32 L4 Heltec Board
Dependents: Turtle_RadioShuttle
RadioStatus.cpp
00001 /* 00002 * The file is licensed under the Apache License, Version 2.0 00003 * (c) 2019 Helmut Tschemernjak 00004 * 30826 Garbsen (Hannover) Germany 00005 */ 00006 00007 00008 #ifdef ARDUINO 00009 #include <Arduino.h> 00010 #include "arduino-mbed.h" 00011 #include <time.h> 00012 #define FEATURE_LORA 1 00013 #endif 00014 #ifdef __MBED__ 00015 #include "mbed.h" 00016 #include "PinMap.h" 00017 #endif 00018 #include "RadioStatusInterface.h" 00019 #include "RadioStatus.h" 00020 #ifdef FEATURE_LORA 00021 00022 00023 MyRadioStatus::MyRadioStatus() 00024 { 00025 _totalTX = 0; 00026 _totalRX = 0; 00027 _totalError = 0; 00028 _totalTimeout = 0; 00029 00030 ledTX = NULL; 00031 ledRX = NULL; 00032 ledTimeout = NULL; 00033 inverted = false; 00034 00035 #ifdef TARGET_STM32L0 00036 ledTX = new DigitalOut(LED3); // blue 00037 *ledTX = 0; 00038 ledRX = new DigitalOut(LED4); // red 00039 *ledRX = 0; 00040 ledTimeout = new DigitalOut(LED1); // green 00041 *ledTimeout = 0; 00042 #endif 00043 #ifdef HELTECL432_REV1 00044 ledTX = new DigitalOut(STATUS_LED); // green 00045 *ledTX = 0; 00046 ledRX = new DigitalOut(LED2); // red 00047 *ledRX = 0; 00048 #endif 00049 #ifdef MyHOME_BOARD_REV4 00050 ledTX = new DigitalOut(LED2); // yellow 00051 *ledTX = 0; 00052 ledRX = new DigitalOut(STATUS_LED); // red 00053 *ledRX = 0; 00054 ledTimeout = new DigitalOut(LED3); // green 00055 *ledTimeout = 0; 00056 #endif 00057 #ifdef __SAMD21G18A__ 00058 inverted = true; 00059 #ifdef PIN_LED_TXL 00060 ledTX = new DigitalOut(PIN_LED_TXL); // yellow 00061 *ledTX = 1; 00062 #endif 00063 #ifdef PIN_LED_RXL 00064 ledRX = new DigitalOut(25); // red 00065 *ledRX = 1; 00066 #endif 00067 #endif 00068 #ifdef ARDUINO_ESP32_DEV // ESP32_ECO_POWER_REV_1 00069 ledTX = new DigitalOut(2); // green 00070 *ledTX = 0; 00071 ledRX = new DigitalOut(12); // red 00072 *ledRX = 0; 00073 #endif 00074 #if defined(ARDUINO_Heltec_WIFI_LoRa_32) || defined(ARDUINO_WIFI_LORA_32) \ 00075 || defined(ARDUINO_WIFI_LORA_32_V2) || defined(ARDUINO_WIRELESS_STICK) \ 00076 || defined(ARDUINO_WIRELESS_STICK_LITE) // the Heltec boards 00077 ledTX = new DigitalOut(25); // white 00078 *ledTX = 0; 00079 #endif 00080 00081 #ifdef HAS_OLED_DISPLAY 00082 invertedDisplay = false; 00083 _line1[0] = 0; 00084 _line2[0] = 0; 00085 _line3[0] = 0; 00086 _line4[0] = 0; 00087 _line5[0] = 0; 00088 displayReset = NULL; 00089 #if defined(ARDUINO_Heltec_WIFI_LoRa_32) || defined(ARDUINO_WIFI_LORA_32) \ 00090 || defined(ARDUINO_WIFI_LORA_32_V2) || defined(ARDUINO_WIRELESS_STICK) 00091 #define DISPLAY_ADDRESS 0x3c 00092 #define DISPLAY_SDA 4 00093 #define DISPLAY_SCL 15 00094 #define DISPLAY_RESET 16 00095 displayReset = new DigitalOut(DISPLAY_RESET); 00096 #endif 00097 #ifdef ARDUINO_ESP32_DEV // ESP32_ECO_POWER_REV_1 00098 #define DISPLAY_ADDRESS 0x3c 00099 #define DISPLAY_SDA 21 00100 #define DISPLAY_SCL 22 00101 #endif 00102 if (displayReset) { 00103 *displayReset = 0; 00104 wait_ms(50); 00105 *displayReset = 1; 00106 } 00107 display = new SSD1306(DISPLAY_ADDRESS, DISPLAY_SDA, DISPLAY_SCL); 00108 display->init(); 00109 // display->flipScreenVertically(); 00110 display->setFont(ArialMT_Plain_16); // ArialMT_Plain_10); 00111 display->clear(); 00112 display->drawString(0, 0, "RadioShuttle 1.4"); 00113 display->setFont(ArialMT_Plain_10); 00114 int yoff = 17; 00115 display->drawString(0, yoff, "Peer-to-Peer LoRa Protcol"); 00116 yoff += 12; 00117 display->drawString(0, yoff, "Efficient, Fast, Secure"); 00118 yoff += 12; 00119 display->drawString(0, yoff, "www.radioshuttle.de"); 00120 display->display(); 00121 #endif 00122 } 00123 00124 MyRadioStatus::~MyRadioStatus() 00125 { 00126 if (ledTX) { 00127 if (inverted) 00128 *ledTX = 1; 00129 else 00130 *ledTX = 0; 00131 delete ledTX; 00132 } 00133 if (ledRX) { 00134 if (inverted) 00135 *ledRX = 1; 00136 else 00137 *ledRX = 0; 00138 delete ledRX; 00139 } 00140 if (ledTimeout) { 00141 if (inverted) 00142 *ledTimeout = 1; 00143 else 00144 *ledTimeout = 0; 00145 delete ledTimeout; 00146 } 00147 } 00148 00149 void 00150 MyRadioStatus::TXStart(int AppID, int toStation, int length, int dBm) 00151 { 00152 UNUSED(AppID); 00153 UNUSED(toStation); 00154 UNUSED(length); 00155 UNUSED(dBm); 00156 if (ledTX) { 00157 if (inverted) 00158 *ledTX = 0; 00159 else 00160 *ledTX = 1; 00161 } 00162 #ifdef HAS_OLED_DISPLAY 00163 snprintf(_line2, sizeof(_line2), "TX(%d) ID(%d) %d dBm", length, toStation, dBm); 00164 UpdateDisplay(true); 00165 #endif 00166 _totalTX++; 00167 } 00168 00169 void 00170 MyRadioStatus::TXComplete(void) 00171 { 00172 if (ledTX) { 00173 if (inverted) 00174 *ledTX = 1; 00175 else 00176 *ledTX = 0; 00177 } 00178 #ifdef HAS_OLED_DISPLAY 00179 UpdateDisplay(false); 00180 #endif 00181 } 00182 00183 void 00184 MyRadioStatus::RxDone(int size, int rssi, int snr) 00185 { 00186 UNUSED(size); 00187 UNUSED(rssi); 00188 UNUSED(snr); 00189 if (ledRX) { 00190 if (inverted) 00191 *ledRX = 0; 00192 else 00193 *ledRX = 1; 00194 } 00195 _totalRX++; 00196 #ifdef HAS_OLED_DISPLAY 00197 snprintf(_line3, sizeof(_line3), "RX(%d) RSSI(%d) SNR(%d)", size, rssi, snr); 00198 UpdateDisplay(true); 00199 #endif 00200 } 00201 00202 void 00203 MyRadioStatus::RxCompleted(void) 00204 { 00205 if (ledRX) { 00206 if (inverted) 00207 *ledRX = 1; 00208 else 00209 *ledRX = 0; 00210 } 00211 #ifdef HAS_OLED_DISPLAY 00212 UpdateDisplay(false); 00213 #endif 00214 } 00215 00216 void 00217 MyRadioStatus::MessageTimeout(int App, int toStation) 00218 { 00219 UNUSED(App); 00220 UNUSED(toStation); 00221 if (ledTimeout) 00222 *ledTimeout = 1; 00223 _totalTimeout++; 00224 #ifdef HAS_OLED_DISPLAY 00225 UpdateDisplay(false); 00226 #endif 00227 } 00228 00229 00230 void 00231 MyRadioStatus::UpdateDisplay(bool invertDisplay) 00232 { 00233 UNUSED(invertDisplay); 00234 #ifdef HAS_OLED_DISPLAY 00235 int yoff = 0; 00236 int hight = 12; 00237 time_t t = time(NULL); 00238 struct tm mytm; 00239 localtime_r(&t, &mytm); 00240 00241 snprintf(_line1, sizeof(_line1), "%s (%d) %02d:%02d:%02d", _radioType, _stationID, 00242 mytm.tm_hour, mytm.tm_min, mytm.tm_sec); 00243 snprintf(_line4, sizeof(_line4), "Packets RX(%d) TX(%d)", _totalRX, _totalTX); 00244 snprintf(_line5, sizeof(_line5), "RXErr(%d) TOut(%d) %.2f %d", _totalError, _totalTimeout, (double)_frequency/1000000.0, _spreadingFactor); 00245 00246 if (invertDisplay) 00247 display->invertDisplay(); 00248 else 00249 display->normalDisplay(); 00250 00251 display->setFont(ArialMT_Plain_10); 00252 display->clear(); 00253 00254 display->drawString(0, yoff, String(_line1)); 00255 yoff += hight; 00256 display->drawString(0, yoff, String(_line2)); 00257 yoff += hight; 00258 display->drawString(0, yoff, String(_line3)); 00259 yoff += hight; 00260 display->drawString(0, yoff, String(_line4)); 00261 yoff += hight; 00262 00263 display->display(); 00264 #endif 00265 } 00266 00267 #endif // FEATURE_LORA
Generated on Mon Jul 18 2022 05:09:52 by
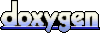