RadioShuttle Lib for the STM32 L4 Heltec Board
Dependents: Turtle_RadioShuttle
RadioStatusInterface.h
00001 /* 00002 * The file is licensed under the Apache License, Version 2.0 00003 * (c) 2019 Helmut Tschemernjak 00004 * 30826 Garbsen (Hannover) Germany 00005 */ 00006 00007 00008 00009 class RadioStatusInterface { 00010 public: 00011 virtual ~RadioStatusInterface() { } 00012 /* 00013 * Signaling that a radio message send has been initiated 00014 */ 00015 virtual void TXStart(int AppID, int toStation, int length, int dBm) = 0; 00016 /* 00017 * Signaling that a radio message send has been completed 00018 */ 00019 virtual void TXComplete(void) = 0; 00020 /* 00021 * Signaling that a radio message input has been received 00022 * and queued for later processing 00023 */ 00024 virtual void RxDone(int size, int rssi, int snr) = 0; 00025 /* 00026 * Signaling that a radio message protocol processing has been completed 00027 */ 00028 virtual void RxCompleted(void) = 0; 00029 /* 00030 * Signaling that a higher-level message received a timeout 00031 * after the specified retry period 00032 */ 00033 virtual void MessageTimeout(int AppID, int toStation) = 0; 00034 00035 void SetStationID(int stationID) { _stationID = stationID; }; 00036 00037 void SetRadioType(const char *radioType) { _radioType = radioType; }; 00038 00039 void SetRadioParams(int frequency, int spreadingFactor) { 00040 _frequency = frequency; _spreadingFactor = spreadingFactor; }; 00041 00042 int _frequency; /* automaticlally set on RadioShuttle::Startup */ 00043 int _spreadingFactor; /* automaticlally set on RadioShuttle::Startup */ 00044 int _stationID; /* automaticlally set */ 00045 const char *_radioType; /* automaticlally set */ 00046 };
Generated on Mon Jul 18 2022 05:09:52 by
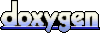