RadioShuttle Lib for the STM32 L4 Heltec Board
Dependents: Turtle_RadioShuttle
RadioSecurityInterface.h
00001 /* 00002 * The file is licensed under the Apache License, Version 2.0 00003 * (c) 2019 Helmut Tschemernjak 00004 * 30826 Garbsen (Hannover) Germany 00005 */ 00006 00007 00008 00009 class RadioSecurityInterface { 00010 public: 00011 virtual ~RadioSecurityInterface() { } 00012 00013 /* 00014 * Get security protocol version to allow 00015 * and differentiate between multiple security versions 00016 */ 00017 virtual int GetSecurityVersion(void) = 0; 00018 00019 /* 00020 * The block size for the hash code (e.g. SHA256) in bytes 00021 */ 00022 virtual int GetHashBlockSize(void) = 0; 00023 00024 /* 00025 * The calculation for the public password hash code utilizes a seed (e.g. random) 00026 * and the cleartext password 00027 */ 00028 virtual void HashPassword(void *seed, int seedLen, void *password, int pwLen, void *hashResult) = 0; 00029 00030 /* 00031 * The encryption/decryption block size in bytes (e.g. 16 bytes for AES128) 00032 */ 00033 virtual int GetEncryptionBlockSize(void) = 0; 00034 00035 /* 00036 * The creation of a context allocates the memory needed, and initializes 00037 * its data (e.g. key and initial vector 'iv' for AES) 00038 */ 00039 virtual void *CreateEncryptionContext(void *key, int keyLen, void *seed = NULL, int seedlen = 0) = 0; 00040 00041 /* 00042 * Release the context and its allocated memory from CreateEncryptionContext 00043 */ 00044 virtual void DestroyEncryptionContext(void *context) = 0; 00045 00046 /* 00047 *Encrypts a cleartext input message into an encrypted output block 00048 */ 00049 virtual void EncryptMessage(void *context, const void *input, void *output, int len) = 0; 00050 00051 /* 00052 * Decrypts an input block into an cleartext output message 00053 */ 00054 virtual void DecryptMessage(void *context, const void *input, void *output, int len) = 0; 00055 00056 virtual void EncryptTest(void) = 0; 00057 };
Generated on Mon Jul 18 2022 05:09:52 by
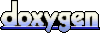