NVProperty generic key value store using the MCU flash area.
Dependents: Turtle_RadioShuttle
NVProperty_SRAM.h
00001 /* 00002 * This is an unpublished work copyright 00003 * (c) 2019 Helmut Tschemernjak 00004 * 30826 Garbsen (Hannover) Germany 00005 * 00006 * 00007 * Use is granted to registered RadioShuttle licensees only. 00008 * Licensees must own a valid serial number and product code. 00009 * Details see: www.radioshuttle.de 00010 */ 00011 00012 #ifndef __NVSProperty_SRAM__ 00013 #define __NVSProperty_SRAM__ 00014 00015 00016 #ifdef ARDUINO 00017 #undef min 00018 #undef max 00019 #undef map 00020 #endif 00021 #include <map> 00022 00023 00024 class NVProperty_SRAM : public NVPropertyProviderInterface { 00025 public: 00026 NVProperty_SRAM(); 00027 ~NVProperty_SRAM(); 00028 virtual int GetProperty(int key); 00029 virtual int64_t GetProperty64(int key); 00030 virtual const char *GetPropertyStr(int key); 00031 virtual int GetPropertyBlob(int key, const void *blob, int *size); 00032 virtual int SetProperty(int key, int64_t value, int type); 00033 virtual int SetPropertyStr(int key, const char *value, int type); 00034 virtual int SetPropertyBlob(int key, const void *blob, int size, int type); 00035 virtual int EraseProperty(int key); 00036 virtual int ReorgProperties(void); 00037 virtual int OpenPropertyStore(bool forWrite = false); 00038 virtual int ClosePropertyStore(bool flush = false); 00039 00040 private: 00041 struct PropertyEntry { 00042 int key; 00043 uint8_t type; 00044 uint8_t size; 00045 union { 00046 int val32; 00047 int64_t val64; 00048 void *data; 00049 char *str; 00050 }; 00051 }; 00052 std::map<int, PropertyEntry> _props; 00053 }; 00054 00055 #endif // __NVSProperty_SRAM__
Generated on Tue Jul 12 2022 20:44:07 by
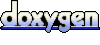