1.0
Dependencies: EthernetInterface mbed-rtos
Telemetry.cpp
00001 /** Ethernet Interface for send/receive Datastructs over udp 00002 * 00003 * 00004 * By Sebastian Donner 00005 * 00006 * Permission is hereby granted, free of charge, to any person 00007 * obtaining a copy of this software and associated documentation 00008 * files (the "Software"), to deal in the Software without 00009 * restriction, including without limitation the rights to use, 00010 * copy, modify, merge, publish, distribute, sublicense, and/or sell 00011 * copies of the Software, and to permit persons to whom the 00012 * Software is furnished to do so, subject to the following 00013 * conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be 00016 * included in all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00019 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES 00020 * OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00021 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT 00022 * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, 00023 * WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING 00024 * FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00025 * OTHER DEALINGS IN THE SOFTWARE. 00026 */ 00027 00028 #include "Telemetry.h" 00029 00030 /**Debug Schnittstelle 00031 */ 00032 #ifdef DEBUG 00033 Serial debug(USBTX, USBRX); 00034 debug.baud(115200); 00035 #endif 00036 00037 /**Konstruktoren 00038 */ 00039 EthernetInterface eth; 00040 TCPSocketConnection sock_tcp; 00041 UDPSocket sock_udp_client; //send socket 00042 UDPSocket sock_udp_server; //receive socket 00043 00044 00045 00046 00047 /** Create a new Ethernet interface 00048 * 00049 */ 00050 Telemetry::Telemetry(PinName ora, PinName gre):orange(ora),green(gre) 00051 { 00052 InitSucceed = false; 00053 green = 0; 00054 orange = 0; 00055 } 00056 00057 00058 /** Init a Serial Debug interface 00059 * 00060 */ 00061 #ifdef DEBUG 00062 void Telemetry::InitUSBSerialConnection() 00063 { 00064 serial.baud(115200); 00065 } 00066 #endif 00067 00068 00069 00070 00071 /** Init Ethernet interface 00072 * mit DHCP max. 10 Sekunden 00073 */ 00074 bool Telemetry::InitEthernetConnection() 00075 { 00076 bool ReturnValue = false; 00077 #ifdef DEBUG 00078 debug.printf("Initalisiere LAN Verbindung mit DHCP\r\n\r\n"); 00079 #endif 00080 00081 //Schnittstelle nur einmal initialisieren, sonst gibt es Fehler! 00082 if (!InitSucceed) 00083 { 00084 if (eth.init()==0) //Init Interface 00085 { 00086 InitSucceed = true; 00087 } 00088 } 00089 00090 //Nur wenn Initialisierung erfolgreich war! 00091 if (InitSucceed) 00092 { 00093 #ifdef DEBUG 00094 serial.printf("Verbinde\r\n\r\n"); 00095 #endif 00096 ip_self = eth.getIPAddress(); 00097 00098 if (eth.connect(10000)==0) //CONNECT 00099 { 00100 #ifdef DEBUG 00101 printf("IP Adresse: %s\r\n\r\n", eth.getIPAddress()); 00102 #endif 00103 ReturnValue = true; 00104 green = 1; 00105 } 00106 else 00107 { 00108 #ifdef DEBUG 00109 serial.printf("DHCP fail!\r\n\r\n"); 00110 #endif 00111 ReturnValue = false; 00112 } 00113 } 00114 00115 return ReturnValue; 00116 } 00117 00118 00119 /** Init Ethernet interface 00120 * ohne DHCP max. 2.5 Sekunden 00121 * @param IPAddress Interface IP 00122 */ 00123 bool Telemetry::InitEthernetConnection(const char* IPAddress, const char* SubNetMask, const char* GateWay) 00124 { 00125 bool ReturnValue = false; 00126 #ifdef DEBUG 00127 printf("Initalisiere LAN Verbindung ohne DHCP\r\n\r\n"); 00128 printf("IP: %s - GateWay: %s - SubNetMask: %s\r\n\r\n",IPAdress, GateWay, SubNetMask); 00129 #endif 00130 00131 //Schnittstelle nur einmal initialisieren, sonst gibt es Fehler! 00132 if (!InitSucceed) 00133 { 00134 if (eth.init(IPAddress, SubNetMask, GateWay)==0) //Init Interface 00135 { 00136 InitSucceed = true; 00137 } 00138 } 00139 00140 //Nur wenn Initialisierung erfolgreich war! 00141 if (InitSucceed) 00142 { 00143 if (eth.connect(2500)==0) //CONNECT 00144 { 00145 green = 1; 00146 #ifdef DEBUG 00147 serial.printf("Init success!"); 00148 #endif 00149 ip_self = eth.getIPAddress(); 00150 ReturnValue = true; 00151 } 00152 else 00153 { 00154 #ifdef DEBUG 00155 serial.printf("Init fail!"); 00156 #endif 00157 ReturnValue = false; 00158 } 00159 } 00160 00161 return ReturnValue; 00162 } 00163 00164 00165 //! Close Connection 00166 void Telemetry::CloseEthernetConnection() 00167 { 00168 eth.disconnect(); 00169 InitSucceed = false; 00170 00171 #ifdef DEBUG 00172 serial.printf("LAN Stack close\r\n\r\n"); 00173 #endif 00174 } 00175 00176 00177 //! Connect Port TCP 00178 void Telemetry::ConnectSocket_TCP(Endpoint Host) 00179 { 00180 sock_tcp.connect(Host.get_address(), Host.get_port()); 00181 sock_tcp.set_blocking(false, 0); 00182 #ifdef DEBUG 00183 serial.printf("Open TCP Socket to IP: %s:%d.\r\n\r\n",Host.get_address(), Host.get_port()); 00184 #endif 00185 } 00186 00187 00188 //! Connect Port UDP receive 00189 void Telemetry::ConnectSocket_UDP_Client() 00190 { 00191 sock_udp_client.init(); 00192 sock_udp_client.set_blocking(false, 0); 00193 00194 #ifdef DEBUG 00195 serial.printf("Open UDP_Client Socket\r\n\r\n"); 00196 #endif 00197 } 00198 00199 00200 //! Connect Port UDP send 00201 void Telemetry::ConnectSocket_UDP_Server(int Port) 00202 { 00203 sock_udp_server.init(); 00204 sock_udp_server.bind(Port); 00205 #ifdef DEBUG 00206 serial.printf("Open UDP_Server on Port: %d.\r\n\r\n",Port); 00207 #endif 00208 } 00209 00210 00211 //! Close Port TCP 00212 void Telemetry::CloseSocket_TCP() 00213 { 00214 sock_tcp.close(); 00215 00216 #ifdef DEBUG 00217 serial.printf("TCP Socket closed.\r\n\r\n"); 00218 #endif 00219 } 00220 00221 00222 //! Close Port UDP receive 00223 void Telemetry::CloseSocket_UDP_Client() 00224 { 00225 sock_udp_client.close(); 00226 #ifdef DEBUG 00227 serial.printf("UDP Client Socket closed.\r\n\r\n"); 00228 #endif 00229 } 00230 00231 00232 //! Close Port UDP send 00233 void Telemetry::CloseSocket_UDP_Server() 00234 { 00235 sock_udp_server.close(); 00236 00237 #ifdef DEBUG 00238 serial.printf("UDP Server Socket closed.\r\n\r\n"); 00239 #endif 00240 } 00241 00242 00243 //! Struct Check Sum calc 00244 uint8_t Telemetry::do_cs(uint8_t* buffer) 00245 { 00246 uint8_t ck_a=0; 00247 uint8_t ck_b=0; 00248 00249 for(int i=0; i < (buffer[0]-1); i++) 00250 { 00251 ck_a += buffer[i]; 00252 ck_b += ck_a; 00253 } 00254 00255 return ck_b; 00256 } 00257 00258 00259 //! Read UDP Packet on Client Port 00260 int Telemetry::Rec_Data_UDP_Client(char *buffer, int size) 00261 { 00262 return sock_udp_client.receiveFrom(input_Client, buffer, size); 00263 } 00264 00265 //! Read UDP Packet on Server Port 00266 int Telemetry::Rec_Data_UDP_Server(char *buffer, int size) 00267 { 00268 return sock_udp_server.receiveFrom(input_Server, buffer, size); 00269 } 00270 00271 00272 //! Check UDP Packet of containing Struct 00273 bool Telemetry::Rec_Struct(uint8_t *buffer) 00274 { 00275 orange = !orange; 00276 if (buffer[buffer[0]-1] == do_cs(buffer)) return true; 00277 else return false; 00278 } 00279 00280 00281 //! Read TCP Packet 00282 int Telemetry::Rec_Data_TCP(char *buffer,int size) 00283 { 00284 orange = !orange; 00285 return sock_tcp.receive(buffer, size); 00286 } 00287 00288 00289 //! Send UDP Packet as Client 00290 void Telemetry::Send_Data_UDP_Client(Endpoint Server, char* Daten, int size ) 00291 { 00292 sock_udp_client.sendTo(Server, Daten, size); 00293 orange = !orange; 00294 #ifdef DEBUG 00295 serial.printf("UDP Paket gesendet.\r\n\r\n"); 00296 #endif 00297 } 00298 00299 //! Send UDP Packet as Server 00300 void Telemetry::Send_Data_UDP_Server(Endpoint Client, char* Daten, int size ) 00301 { 00302 sock_udp_server.sendTo(Client, Daten, size); 00303 orange = !orange; 00304 #ifdef DEBUG 00305 serial.printf("UDP Paket gesendet.\r\n\r\n"); 00306 #endif 00307 } 00308 00309 //! Send Struct as UDP Client 00310 void Telemetry::Send_Struct_UDP_Client(Endpoint Server, uint8_t* Daten) 00311 { 00312 Daten[(*Daten - 1)] = do_cs(Daten); 00313 sock_udp_client.sendTo(Server, (char*)Daten, *Daten); 00314 orange = !orange; 00315 } 00316 00317 //! Send Struct as UDP Server 00318 void Telemetry::Send_Struct_UDP_Server(Endpoint Client, uint8_t* Daten) 00319 { 00320 Daten[(*Daten - 1)] = do_cs(Daten); 00321 sock_udp_server.sendTo(Client, (char*)Daten, *Daten); 00322 orange = !orange; 00323 } 00324 00325 //! Send TCP Packet 00326 void Telemetry::Send_Data_TCP(char* Host, char* Buffer) 00327 { 00328 00329 /* Umwandeln in char* 00330 const char *DataBuf = datenpaket.c_str(); 00331 char DataPaket[datenpaket.length()]; 00332 strcpy(DataPaket,DataBuf); 00333 */ 00334 #ifdef DEBUG 00335 serial.printf("----\r\n%s----\r\n\r\n",DataPaket); 00336 serial.printf("Sende Paket.\r\n\r\n"); 00337 #endif 00338 00339 sock_tcp.send_all(Buffer, sizeof(Buffer)-1); 00340 00341 #ifdef DEBUG 00342 serial.printf("Paket gesendet.\r\n\r\n"); 00343 #endif 00344 } 00345 00346 00347 00348 00349
Generated on Fri Jul 29 2022 09:32:50 by
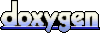