Added to LPC4088-USBHost the USBHostMidi class. Plugin an usb midi interface to the usb host of lpc4088 allows to send midi event to the midi interface. For the moment I can not be able to get event from the interface by using the attacheNoteOn or other triggers...
Dependencies: FATFileSystem mbed-rtos
Fork of LPC4088-USBHost by
USBHostMIDI.h
00001 00002 00003 /* Copyright (c) 2014 mbed.org, MIT License 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00006 * and associated documentation files (the "Software"), to deal in the Software without 00007 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00008 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in all copies or 00012 * substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00015 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00016 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00017 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00018 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00019 */ 00020 00021 #ifndef USBHOSTMIDI_H 00022 #define USBHOSTMIDI_H 00023 00024 #include "USBHostConf.h" 00025 00026 #if USBHOST_MIDI 00027 00028 #include "USBHost.h" 00029 00030 /** 00031 * A class to communicate a USB MIDI device 00032 */ 00033 class USBHostMIDI : public IUSBEnumerator { 00034 public: 00035 /** 00036 * Constructor 00037 */ 00038 USBHostMIDI(); 00039 00040 /** 00041 * Check if a USB MIDI device is connected 00042 * 00043 * @returns true if a midi device is connected 00044 */ 00045 bool connected(); 00046 00047 /** 00048 * Try to connect a midi device 00049 * 00050 * @return true if connection was successful 00051 */ 00052 bool connect(); 00053 00054 /** 00055 * Attach a callback called when miscellaneous function code is received 00056 * 00057 * @param ptr function pointer 00058 * prototype: void onMiscellaneousFunctionCode(uint8_t data1, uint8_t data2, uint8_t data3); 00059 */ 00060 inline void attachMiscellaneousFunctionCode(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00061 miscellaneousFunctionCode = fn; 00062 } 00063 00064 /** 00065 * Attach a callback called when cable event is received 00066 * 00067 * @param ptr function pointer 00068 * prototype: void onCableEvent(uint8_t data1, uint8_t data2, uint8_t data3); 00069 */ 00070 inline void attachCableEvent(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00071 cableEvent = fn; 00072 } 00073 00074 /** 00075 * Attach a callback called when system exclusive is received 00076 * 00077 * @param ptr function pointer 00078 * prototype: void onSystemCommonTwoBytes(uint8_t data1, uint8_t data2); 00079 */ 00080 inline void attachSystemCommonTwoBytes(void (*fn)(uint8_t, uint8_t)) { 00081 systemCommonTwoBytes = fn; 00082 } 00083 00084 /** 00085 * Attach a callback called when system exclusive is received 00086 * 00087 * @param ptr function pointer 00088 * prototype: void onSystemCommonThreeBytes(uint8_t data1, uint8_t data2, uint8_t data3); 00089 */ 00090 inline void attachSystemCommonThreeBytes(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00091 systemCommonThreeBytes = fn; 00092 } 00093 00094 /** 00095 * Attach a callback called when system exclusive is received 00096 * 00097 * @param ptr function pointer 00098 * prototype: void onSystemExclusive(uint8_t *data, uint16_t length, bool hasNextData); 00099 */ 00100 inline void attachSystemExclusive(void (*fn)(uint8_t *, uint16_t, bool)) { 00101 systemExclusive = fn; 00102 } 00103 00104 /** 00105 * Attach a callback called when note on is received 00106 * 00107 * @param ptr function pointer 00108 * prototype: void onNoteOn(uint8_t channel, uint8_t note, uint8_t velocity); 00109 */ 00110 inline void attachNoteOn(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00111 noteOn = fn; 00112 } 00113 00114 /** 00115 * Attach a callback called when note off is received 00116 * 00117 * @param ptr function pointer 00118 * prototype: void onNoteOff(uint8_t channel, uint8_t note, uint8_t velocity); 00119 */ 00120 inline void attachNoteOff(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00121 noteOff = fn; 00122 } 00123 00124 /** 00125 * Attach a callback called when poly keypress is received 00126 * 00127 * @param ptr function pointer 00128 * prototype: void onPolyKeyPress(uint8_t channel, uint8_t note, uint8_t pressure); 00129 */ 00130 inline void attachPolyKeyPress(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00131 polyKeyPress = fn; 00132 } 00133 00134 /** 00135 * Attach a callback called when control change is received 00136 * 00137 * @param ptr function pointer 00138 * prototype: void onControlChange(uint8_t channel, uint8_t key, uint8_t value); 00139 */ 00140 inline void attachControlChange(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00141 controlChange = fn; 00142 } 00143 00144 /** 00145 * Attach a callback called when program change is received 00146 * 00147 * @param ptr function pointer 00148 * prototype: void onProgramChange(uint8_t channel, uint8_t program); 00149 */ 00150 inline void attachProgramChange(void (*fn)(uint8_t, uint8_t)) { 00151 programChange = fn; 00152 } 00153 00154 /** 00155 * Attach a callback called when channel pressure is received 00156 * 00157 * @param ptr function pointer 00158 * prototype: void onChannelPressure(uint8_t channel, uint8_t pressure); 00159 */ 00160 inline void attachChannelPressure(void (*fn)(uint8_t, uint8_t)) { 00161 channelPressure = fn; 00162 } 00163 00164 /** 00165 * Attach a callback called when pitch bend is received 00166 * 00167 * @param ptr function pointer 00168 * prototype: void onPitchBend(uint8_t channel, uint16_t value); 00169 */ 00170 inline void attachPitchBend(void (*fn)(uint8_t, uint16_t)) { 00171 pitchBend = fn; 00172 } 00173 00174 /** 00175 * Attach a callback called when single byte is received 00176 * 00177 * @param ptr function pointer 00178 * prototype: void onSingleByte(uint8_t value); 00179 */ 00180 inline void attachSingleByte(void (*fn)(uint8_t)) { 00181 singleByte = fn; 00182 } 00183 00184 /** 00185 * Send a cable event with 3 bytes event 00186 * 00187 * @param data1 0-255 00188 * @param data2 0-255 00189 * @param data3 0-255 00190 * @return true if message sent successfully 00191 */ 00192 bool sendMiscellaneousFunctionCode(uint8_t data1, uint8_t data2, uint8_t data3); 00193 00194 /** 00195 * Send a cable event with 3 bytes event 00196 * 00197 * @param data1 0-255 00198 * @param data2 0-255 00199 * @param data3 0-255 00200 * @return true if message sent successfully 00201 */ 00202 bool sendCableEvent(uint8_t data1, uint8_t data2, uint8_t data3); 00203 00204 /** 00205 * Send a system common message with 2 bytes event 00206 * 00207 * @param data1 0-255 00208 * @param data2 0-255 00209 * @return true if message sent successfully 00210 */ 00211 bool sendSystemCommmonTwoBytes(uint8_t data1, uint8_t data2); 00212 00213 /** 00214 * Send a system common message with 3 bytes event 00215 * 00216 * @param data1 0-255 00217 * @param data2 0-255 00218 * @param data3 0-255 00219 * @return true if message sent successfully 00220 */ 00221 bool sendSystemCommmonThreeBytes(uint8_t data1, uint8_t data2, uint8_t data3); 00222 00223 /** 00224 * Send a system exclusive event 00225 * 00226 * @param buffer, starts with 0xF0, and end with 0xf7 00227 * @param length 00228 * @return true if message sent successfully 00229 */ 00230 bool sendSystemExclusive(uint8_t *buffer, int length); 00231 00232 /** 00233 * Send a note off event 00234 * 00235 * @param channel 0-15 00236 * @param note 0-127 00237 * @param velocity 0-127 00238 * @return true if message sent successfully 00239 */ 00240 bool sendNoteOff(uint8_t channel, uint8_t note, uint8_t velocity); 00241 00242 /** 00243 * Send a note on event 00244 * 00245 * @param channel 0-15 00246 * @param note 0-127 00247 * @param velocity 0-127 (0 means note off) 00248 * @return true if message sent successfully 00249 */ 00250 bool sendNoteOn(uint8_t channel, uint8_t note, uint8_t velocity); 00251 00252 /** 00253 * Send a poly keypress event 00254 * 00255 * @param channel 0-15 00256 * @param note 0-127 00257 * @param pressure 0-127 00258 * @return true if message sent successfully 00259 */ 00260 bool sendPolyKeyPress(uint8_t channel, uint8_t note, uint8_t pressure); 00261 00262 /** 00263 * Send a control change event 00264 * 00265 * @param channel 0-15 00266 * @param key 0-127 00267 * @param value 0-127 00268 * @return true if message sent successfully 00269 */ 00270 bool sendControlChange(uint8_t channel, uint8_t key, uint8_t value); 00271 00272 /** 00273 * Send a program change event 00274 * 00275 * @param channel 0-15 00276 * @param program 0-127 00277 * @return true if message sent successfully 00278 */ 00279 bool sendProgramChange(uint8_t channel, uint8_t program); 00280 00281 /** 00282 * Send a channel pressure event 00283 * 00284 * @param channel 0-15 00285 * @param pressure 0-127 00286 * @return true if message sent successfully 00287 */ 00288 bool sendChannelPressure(uint8_t channel, uint8_t pressure); 00289 00290 /** 00291 * Send a control change event 00292 * 00293 * @param channel 0-15 00294 * @param key 0(lower)-8191(center)-16383(higher) 00295 * @return true if message sent successfully 00296 */ 00297 bool sendPitchBend(uint8_t channel, uint16_t value); 00298 00299 /** 00300 * Send a single byte event 00301 * 00302 * @param data 0-255 00303 * @return true if message sent successfully 00304 */ 00305 bool sendSingleByte(uint8_t data); 00306 00307 protected: 00308 //From IUSBEnumerator 00309 virtual void setVidPid(uint16_t vid, uint16_t pid); 00310 virtual bool parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol); //Must return true if the interface should be parsed 00311 virtual bool useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir); //Must return true if the endpoint will be used 00312 00313 private: 00314 USBHost * host; 00315 USBDeviceConnected * dev; 00316 USBEndpoint * bulk_in; 00317 USBEndpoint * bulk_out; 00318 uint32_t size_bulk_in; 00319 uint32_t size_bulk_out; 00320 00321 bool dev_connected; 00322 00323 void init(); 00324 00325 uint8_t buf[64]; 00326 00327 void rxHandler(); 00328 00329 uint16_t sysExBufferPos; 00330 uint8_t sysExBuffer[64]; 00331 00332 void (*miscellaneousFunctionCode)(uint8_t, uint8_t, uint8_t); 00333 void (*cableEvent)(uint8_t, uint8_t, uint8_t); 00334 void (*systemCommonTwoBytes)(uint8_t, uint8_t); 00335 void (*systemCommonThreeBytes)(uint8_t, uint8_t, uint8_t); 00336 void (*systemExclusive)(uint8_t *, uint16_t, bool); 00337 void (*noteOff)(uint8_t, uint8_t, uint8_t); 00338 void (*noteOn)(uint8_t, uint8_t, uint8_t); 00339 void (*polyKeyPress)(uint8_t, uint8_t, uint8_t); 00340 void (*controlChange)(uint8_t, uint8_t, uint8_t); 00341 void (*programChange)(uint8_t, uint8_t); 00342 void (*channelPressure)(uint8_t, uint8_t); 00343 void (*pitchBend)(uint8_t, uint16_t); 00344 void (*singleByte)(uint8_t); 00345 00346 bool sendMidiBuffer(uint8_t data0, uint8_t data1, uint8_t data2, uint8_t data3); 00347 00348 int midi_intf; 00349 bool midi_device_found; 00350 00351 }; 00352 00353 #endif /* USBHOST_MIDI */ 00354 00355 #endif /* USBHOSTMIDI_H */ 00356
Generated on Wed Jul 13 2022 13:35:16 by
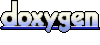