
Ethernetwebsoc
Dependencies: C12832_lcd LM75B WebSocketClient mbed-rtos mbed Socket lwip-eth lwip-sys lwip
Semaphore.cpp
00001 #include "Semaphore.h" 00002 00003 #include <string.h> 00004 #include "error.h" 00005 00006 namespace rtos { 00007 00008 Semaphore::Semaphore (int32_t count) { 00009 #ifdef CMSIS_OS_RTX 00010 memset(_semaphore_data, 0, sizeof(_semaphore_data)); 00011 _osSemaphoreDef.semaphore = _semaphore_data; 00012 #endif 00013 _osSemaphoreId = osSemaphoreCreate(&_osSemaphoreDef, count); 00014 } 00015 00016 int32_t Semaphore::wait (uint32_t millisec) { 00017 return osSemaphoreWait(_osSemaphoreId, millisec); 00018 } 00019 00020 osStatus Semaphore::release (void) { 00021 return osSemaphoreRelease(_osSemaphoreId); 00022 } 00023 00024 Semaphore::~Semaphore() { 00025 osSemaphoreDelete(_osSemaphoreId); 00026 } 00027 00028 }
Generated on Tue Jul 12 2022 19:26:07 by
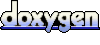