
Ethernetwebsoc
Dependencies: C12832_lcd LM75B WebSocketClient mbed-rtos mbed Socket lwip-eth lwip-sys lwip
RtosTimer.cpp
00001 #include "RtosTimer.h" 00002 00003 #include <string.h> 00004 00005 #include "cmsis_os.h" 00006 #include "error.h" 00007 00008 namespace rtos { 00009 00010 RtosTimer::RtosTimer (void (*periodic_task)(void const *argument), os_timer_type type, void *argument) { 00011 #ifdef CMSIS_OS_RTX 00012 _timer.ptimer = periodic_task; 00013 00014 memset(_timer_data, 0, sizeof(_timer_data)); 00015 _timer.timer = _timer_data; 00016 #endif 00017 _timer_id = osTimerCreate(&_timer, type, argument); 00018 } 00019 00020 osStatus RtosTimer::start (uint32_t millisec) { 00021 return osTimerStart(_timer_id, millisec); 00022 } 00023 00024 osStatus RtosTimer::stop (void) { 00025 return osTimerStop(_timer_id); 00026 } 00027 00028 RtosTimer::~RtosTimer() { 00029 osTimerDelete(_timer_id); 00030 } 00031 00032 }
Generated on Tue Jul 12 2022 19:26:07 by
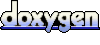