
Ethernetwebsoc
Dependencies: C12832_lcd LM75B WebSocketClient mbed-rtos mbed Socket lwip-eth lwip-sys lwip
Queue.h
00001 /* Copyright (c) 2012 mbed.org */ 00002 #ifndef QUEUE_H 00003 #define QUEUE_H 00004 00005 #include <stdint.h> 00006 #include <string.h> 00007 00008 #include "cmsis_os.h" 00009 #include "error.h" 00010 00011 namespace rtos { 00012 00013 /*! The Queue class allow to control, send, receive, or wait for messages. 00014 A message can be a integer or pointer value to a certain type T that is send 00015 to a thread or interrupt service routine. 00016 \tparam T data type of a single message element. 00017 \tparam queue_sz maximum number of messages in queue. 00018 */ 00019 template<typename T, uint32_t queue_sz> 00020 class Queue { 00021 public: 00022 /*! Create and initialise a message Queue. */ 00023 Queue () { 00024 #ifdef CMSIS_OS_RTX 00025 memset(_queue_q, 0, sizeof(_queue_q)); 00026 _queue_def.pool = _queue_q; 00027 _queue_def.queue_sz = queue_sz; 00028 #endif 00029 _queue_id = osMessageCreate(&_queue_def, NULL); 00030 if (_queue_id == NULL) { 00031 error("Error initialising the queue object\n"); 00032 } 00033 } 00034 00035 /*! Put a message in a Queue. 00036 \param data message pointer. 00037 \param millisec timeout value or 0 in case of no time-out. (default: 0) 00038 \return status code that indicates the execution status of the function. 00039 */ 00040 osStatus put (T* data, uint32_t millisec=0) { 00041 return osMessagePut(_queue_id, (uint32_t)data, millisec); 00042 } 00043 00044 /*! Get a message or Wait for a message from a Queue. 00045 \param millisec timeout value or 0 in case of no time-out. (default: osWaitForever). 00046 \return event information that includes the message and the status code. 00047 */ 00048 osEvent get(uint32_t millisec=osWaitForever) { 00049 return osMessageGet(_queue_id, millisec); 00050 } 00051 00052 private: 00053 osMessageQId _queue_id; 00054 osMessageQDef_t _queue_def; 00055 #ifdef CMSIS_OS_RTX 00056 uint32_t _queue_q[4+(queue_sz)]; 00057 #endif 00058 }; 00059 00060 } 00061 #endif
Generated on Tue Jul 12 2022 19:26:06 by
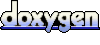