
Plant Monitoring Project
Dependencies: mbed SHT21_ncleee WakeUp SSD1306 DHT Adafruit_TCS34725 DS1820
main.cpp
00001 #include "main.hh" 00002 00003 int main(){ 00004 #ifdef DEEPSLEEP 00005 //WakeUp::calibrate(); 00006 #endif 00007 00008 #ifdef INTERRUPTEUR 00009 interrupteur = 1; 00010 #endif 00011 00012 #ifdef OLED 00013 bouton.rise(interruption_bouton); 00014 initOLED(); 00015 #endif 00016 00017 while(1) { 00018 if(flag_readData) 00019 readData(); 00020 flag_readData = true; 00021 #ifndef DEEPSLEEP 00022 wait(DUREE_OFF); 00023 #endif 00024 #ifdef DEEPSLEEP 00025 if(oled_on){ 00026 wait(DUREE_ECRAN_ON); 00027 turnOffScreen(); 00028 flag_readData = false; 00029 } 00030 else{ 00031 WakeUp::set_ms(DUREE_OFF*1000); 00032 deepsleep(); 00033 } 00034 #endif 00035 } 00036 } 00037 00038 #ifdef AIR_PARAMETERS_DHT22 00039 void air_temp_hum(){ 00040 int err; 00041 wait(1); 00042 do{ 00043 err = dht_sensor.readData(); 00044 wait(1); 00045 }while(err != 0); 00046 temperature_air = dht_sensor.ReadTemperature(CELCIUS); 00047 humidity_air = dht_sensor.ReadHumidity(); 00048 } 00049 #endif 00050 00051 #ifdef FLOOR_TEMPERATURE 00052 void temp_sol() 00053 { 00054 int result = 0; 00055 ds1820.begin(); 00056 ds1820.startConversion(); // start temperature conversion from analog to digital 00057 wait(1.0); // let DS1820 complete the temperature conversion 00058 result = ds1820.read(temperature_sol); // read temperature from DS1820 and perform cyclic redundancy check (CRC) 00059 #ifdef DEBUG 00060 switch (result) { 00061 case 0: // no errors -> 'temp' contains the value of measured temperature 00062 pc.printf("temp = %3.1f C\r\n", temperature_sol); 00063 break; 00064 00065 case 1: // no sensor present -> 'temp' is not updated 00066 pc.printf("no sensor present\n\r"); 00067 break; 00068 00069 case 2: // CRC error -> 'temp' is not updated 00070 pc.printf("CRC error\r\n"); 00071 } 00072 #endif 00073 00074 } 00075 #endif 00076 00077 #ifdef FLOOR_HUMIDITY 00078 int fct_humidity_sol(void) 00079 { 00080 float val_min = 0.377; 00081 float val_max = 0.772; 00082 float mesure, mesure_etalonnee; 00083 mesure = capteur_humidity_sol.read(); 00084 mesure_etalonnee = (1-((mesure - val_min)/(val_max - val_min)))*100; 00085 #ifdef DEBUG 00086 pc.printf("hum sol: %d\n\r", (int) mesure_etalonnee); 00087 #endif 00088 return (int) mesure_etalonnee; 00089 } 00090 #endif 00091 00092 #ifdef RGB 00093 void fct_RGB(void) 00094 { 00095 int somme; 00096 uint16_t clear, red, green, blue; 00097 if (!RGBsens.begin()) 00098 { 00099 #ifdef DEBUG 00100 pc.printf("No TCS34725 found ... check your connections"); 00101 #endif 00102 //while (1); // halt! 00103 } 00104 RGBsens.getRawData(&red, &green, &blue, &clear); 00105 somme = red + green + blue; 00106 pr = red*100/somme; 00107 pg = green*100/somme; 00108 pb = blue*100/somme; 00109 lum = (unsigned short) RGBsens.calculateLux(red, green, blue); 00110 #ifdef DEBUG 00111 pc.printf("luminosite : %d \n\r", lum); 00112 pc.printf("rouge:%d vert:%d bleu:%d \n\r", pr, pg, pb); 00113 #endif 00114 } 00115 #endif 00116 00117 #ifdef SIGFOX 00118 void sendDataSigfox(void){ 00119 #ifdef DEBUG 00120 pc.printf("Sending Data to Sigfox \n\r"); 00121 #endif 00122 short tempSol_short, tempAir_short; 00123 tempSol_short = (short)(temperature_sol*10); 00124 tempAir_short = (short)(temperature_air*10); 00125 00126 wisol.printf("AT$SF=%04x%02x%04x%02x%04x%02x%02x%02x%02x\r\n",tempSol_short, humidity_sol, tempAir_short, humidity_air, lum, pr, pg, pb, vBat); 00127 } 00128 #endif 00129 00130 #ifdef OLED 00131 void oledData(void){ 00132 #ifdef DEBUG 00133 pc.printf("Displaying Data\r\n"); 00134 #endif 00135 00136 if(!oled_on){ 00137 oled.wake(); 00138 oled.clear(); 00139 oled_on = 1; 00140 } 00141 oled.clear(); 00142 oled.printf("AIR T : %.1f", temperature_air); 00143 oled.printf("\n\r"); 00144 oled.printf("AIR H : %d", humidity_air); 00145 oled.printf("\n\r\n\r"); 00146 oled.printf("FLOOR T : %.1f", temperature_sol); 00147 oled.printf("\n\r"); 00148 oled.printf("FLOOR H : %d", humidity_sol); 00149 oled.printf("\n\r\n\r"); 00150 oled.printf("Light : %d", lum); 00151 oled.printf("\n\r"); 00152 oled.printf("R %d G %d B %d", pr, pg, pb); 00153 oled.update(); 00154 } 00155 #endif 00156 00157 void readData(void){ 00158 #ifdef INTERRUPTEUR 00159 interrupteur = 1; 00160 wait_ms(100); 00161 RGBsens.begin(); 00162 wait_ms(100); 00163 #endif 00164 #ifdef DEBUG 00165 pc.printf("Reading Data\n\r"); 00166 #endif 00167 #ifdef FLOOR_TEMPERATURE 00168 temp_sol(); 00169 #endif 00170 #ifdef FLOOR_HUMIDITY 00171 humidity_sol = fct_humidity_sol(); 00172 #endif 00173 #ifdef AIR_PARAMETERS_DHT22 00174 air_temp_hum(); 00175 #endif 00176 #ifdef DEBUG 00177 printf("hum air: %d\n\r", humidity_air); 00178 printf("temp air: %.1f\n\r", temperature_air); 00179 #endif 00180 #ifdef RGB 00181 fct_RGB(); 00182 #endif 00183 #ifdef BATTERY_LVL 00184 readBatteryLvl(); 00185 #endif 00186 #ifdef SIGFOX 00187 sendDataSigfox(); 00188 #endif 00189 #ifdef OLED 00190 if(oled_on) 00191 oledData(); 00192 #endif 00193 #ifdef INTERRUPTEUR 00194 wait(8); // Il faut du temps au module SigFox pour envoyer les données 00195 interrupteur = 0; 00196 #endif 00197 } 00198 00199 void interruption_bouton(){ 00200 bouton.disable_irq(); 00201 #ifdef DEBUG 00202 pc.printf("Button interrupt\r\n"); 00203 #endif 00204 #ifdef OLED 00205 if(!oled_on){ 00206 oledData(); 00207 } 00208 #endif 00209 bouton.enable_irq(); 00210 } 00211 00212 #ifdef OLED 00213 void turnOffScreen(void){ 00214 #ifdef DEBUG 00215 pc.printf("Turning off screen \n\r"); 00216 #endif 00217 oled_on = 0; 00218 oled.sleep(); 00219 } 00220 #endif 00221 00222 #ifdef OLED 00223 void initOLED(void){ 00224 oled.on(); 00225 oled.initialise(); 00226 oled.clear(); 00227 oled.set_contrast(255); 00228 oled.set_font(bold_font, 8); 00229 oled.printf("================"); 00230 oled.printf("\n\r"); 00231 oled.printf(" 2PA2S"); 00232 oled.printf("\n\r\n\r"); 00233 oled.printf("FRAYSSE GERMAIN"); 00234 oled.printf("\n\r\n\r"); 00235 oled.printf(" DUPLESSIS"); 00236 oled.printf("\n\r"); 00237 oled.printf("================"); 00238 oled.update(); 00239 wait(10); 00240 oled.clear(); 00241 oled.update(); 00242 oled.sleep(); 00243 } 00244 #endif 00245 00246 void readBatteryLvl(void){ 00247 float calcVbat = 0; 00248 int batIn = 0; 00249 float ain = battery.read(); 00250 for(int i = 0; i < NB_MESURES; i++){ 00251 batIn = 100*ain; 00252 calcVbat += ((float)(batIn - BATTERIE_MIN)/(float)(BATTERIE_MAX - BATTERIE_MIN))*100; 00253 } 00254 vBat = (char)(calcVbat/NB_MESURES); 00255 #ifdef DEBUG 00256 pc.printf("batIn = %d\n\r", batIn); 00257 pc.printf("calcVbat = %d\n\r", calcVbat); 00258 pc.printf("vBat = %d\n\r", vBat); 00259 #endif 00260 }
Generated on Wed Jul 13 2022 14:34:52 by
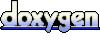