Starting library to get the SI1143 Gesture Sensor to work. Currently only works in forced conversion mode.
Dependents: Gesture_Sensor Gesture_Arm_Robot mbed_gesture Gesture_Sensor_Sample ... more
SI1143.h
00001 /** 00002 * @author Guillermo A Torijano 00003 * 00004 * @section LICENSE 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in 00014 * all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00022 * THE SOFTWARE. 00023 * 00024 * @section DESCRIPTION 00025 * 00026 * Parallax SI1143 Gesture Sensor. 00027 * 00028 * Datasheet: 00029 * 00030 * http://www.silabs.com/Support%20Documents/TechnicalDocs/Si114x.pdf 00031 */ 00032 00033 #ifndef SI1143_h 00034 #define SI1143_h 00035 00036 /** 00037 * Includes 00038 */ 00039 00040 #include "mbed.h" 00041 00042 /** 00043 * Defines 00044 */ 00045 00046 #define IR_ADDRESS 0x5A 00047 #define HW_KEY_VAL0 0x17 //Value to write into the HW Key register 00048 00049 // Register Addresses 00050 00051 #define PART_ID 0x00 00052 #define REV_ID 0x01 00053 #define SEQ_ID 0x02 //Si114x-A11 (MAJOR_SEQ=1, MINOR_SEQ=1) 00054 #define INT_CFG 0x03 00055 #define IRQ_ENABLE 0x04 00056 #define IRQ_MODE1 0x05 00057 #define IRQ_MODE2 0x06 00058 #define HW_KEY 0x07 00059 00060 #define MEAS_RATE 0x08 00061 #define ALS_RATE 0x09 00062 #define PS_RATE 0x0A 00063 00064 #define ALS_LOW_TH0 0x0B 00065 #define ALS_LOW_TH1 0x0C 00066 #define ALS_HI_TH0 0x0D 00067 #define ALS_HI_TH1 0x0E 00068 00069 #define PS_LED21 0x0F 00070 #define PS_LED3 0x10 00071 00072 #define PS1_TH0 0x11 00073 #define PS1_TH1 0x12 00074 #define PS2_TH0 0x13 00075 #define PS2_TH1 0x14 00076 #define PS3_TH0 0x15 00077 00078 #define PS3_TH1 0x16 00079 #define PARAM_WR 0x17 00080 #define COMMAND 0x18 00081 00082 #define RESPONSE 0x20 00083 #define IRQ_STATUS 0x21 00084 00085 #define ALS_VIS_DATA0 0x22 00086 #define ALS_VIS_DATA1 0x23 00087 #define ALS_IR_DATA0 0x24 00088 #define ALS_IR_DATA1 0x25 00089 00090 #define PS1_DATA0 0x26 00091 #define PS1_DATA1 0x27 00092 #define PS2_DATA0 0x28 00093 #define PS2_DATA1 0x29 00094 #define PS3_DATA0 0x2A 00095 #define PS3_DATA1 0x2B 00096 00097 00098 #define AUX_DATA0 0x2C 00099 #define AUX_DATA1 0x2D 00100 00101 #define PARAM_RD 0x2E 00102 #define CHIP_STAT 0x30 00103 #define ANA_IN_KEY 0x3B 00104 00105 // Command Register Values 00106 00107 #define PARAM_QUERY 0x80 //Value is ORed with Parameter Offset 00108 #define PARAM_SET 0xA0 //Value is ORed with Parameter Offset 00109 #define PARAM_AND 0xC0 //Value is ORed with Parameter Offset 00110 #define PARAM_OR 0xE0 //Value is ORed with Parameter Offset 00111 #define NOP 0x00 00112 #define RESET 0x01 00113 #define BUSADDR 0x02 00114 #define PS_FORCE 0x05 00115 #define ALS_FORCE 0x06 00116 #define PSALS_FORCE 0x07 00117 #define PS_PAUSE 0x09 00118 #define ALS_PAUSE 0x0A 00119 #define PSALS_PAUSE 0x0B 00120 #define PS_AUTO 0x0D 00121 #define ALS_AUTO 0x0E 00122 #define PSALS_AUTO 0x0F 00123 00124 // Ram Addresses 00125 00126 #define I2C_ADDR 0x00 00127 #define CHLIST 0x01 00128 #define PSLED12_SELECT 0x02 00129 #define PSLED3_SELECT 0x03 00130 #define FILTER_EN 0x04 00131 #define PS_ENCODING 0x05 00132 #define ALS_ENCODING 0x06 00133 #define PS1_ADCMUX 0x07 00134 #define PS2_ADCMUX 0x08 00135 #define PS3_ADCMUX 0x09 00136 #define PS_ADC_COUNTER 0x0A 00137 #define PS_ADC_GAIN 0x0B 00138 #define PS_ADC_MISC 0x0C 00139 #define ALS1_ADCMUX 0x0D 00140 #define ALS2_ADCMUX 0x0E 00141 #define ALS3_ADCMUX 0x0F 00142 #define ALS_VIS_ADC_COUNTER 0x10 00143 #define ALS_VIS_ADC_GAIN 0x11 00144 #define ALS_VIS_ADC_MISC 0x12 00145 #define ALS_HYST 0x16 00146 #define PS_HYST 0x17 00147 #define PS_HISTORY 0x18 00148 #define ALS_HISTORY 0x19 00149 #define ADC_OFFSET 0x1A 00150 #define SLEEP_CTRL 0x1B 00151 #define LED_REC 0x1C 00152 #define ALS_IR_ADC_COUNTER 0x1D 00153 #define ALS_IR_ADC_GAIN 0x1E 00154 #define ALS_IR_ADC_MISC 0x1F 00155 00156 // Measurement Channel List 00157 00158 #define PS1_TASK 0x01 00159 #define PS2_TASK 0x02 00160 #define PS3_TASK 0x04 00161 #define ALS_VIS_TASK 0x10 00162 #define ALS_IR_TASK 0x20 00163 #define AUX_TASK 0x40 00164 00165 /** 00166 * Parallax SI1143 Gesture Sensor. 00167 */ 00168 class SI1143 00169 { 00170 public: 00171 00172 /** 00173 * Constructor. 00174 * 00175 * @param sda mbed pin to use for SDA line of I2C interface. 00176 * @param scl mbed pin to use for SCL line of I2C interface. 00177 */ 00178 SI1143(PinName sda, PinName scl); 00179 00180 /** 00181 * Restarts the device. 00182 */ 00183 void restart(void); 00184 00185 /** 00186 * Creates a baseline for sampling measurements. 00187 * Should be done early in your code and after a reset. 00188 * 00189 * @param ready Tells how many seconds to wait before getting samples from the device. 00190 * @param repeat Tells how many samples to get from each leds on the device. Each repeat takes 12 ms. 00191 */ 00192 void bias(int ready, int repeat); 00193 00194 /** 00195 * Takes a number of samples from the proximity of led1 and returns a raw output. 00196 * 00197 * @param repeat Tells how many samples to get from the device. Each sample takes 4 ms. 00198 * @return In forced conversion output mode, will display a raw output of the average sample 00199 * minus any baseline, where as the greater the value, the closer the object is to the device. 00200 */ 00201 int get_ps1(int repeat); 00202 00203 /** 00204 * Takes a number of samples from the proximity of led2 and returns a raw output. 00205 * 00206 * @param repeat Tells how many samples to get from the device. Each sample takes 4 ms. 00207 * @return In forced conversion output mode, will display a raw output of the average sample 00208 * minus any baseline, where as the greater the value, the closer the object is to the device. 00209 */ 00210 int get_ps2(int repeat); 00211 00212 /** 00213 * Takes a number of samples from the proximity of led3 and returns a raw output. 00214 * 00215 * @param repeat Tells how many samples to get from the device. Each sample takes 4 ms. 00216 * @return In forced conversion output mode, will display a raw output of the average sample 00217 * minus any baseline, where as the greater the value, the closer the object is to the device. 00218 */ 00219 int get_ps3(int repeat); 00220 00221 /** 00222 * Takes a number of samples for ambient light on device and returns a raw output. 00223 * 00224 * @param repeat Tells how many samples to get from the device. Each sample takes 4 ms. 00225 * @return In forced conversion output mode, will display a raw output of the average sample. 00226 */ 00227 int get_vis(int repeat); 00228 00229 /** 00230 * Takes a number of samples for infrared light on device and returns a raw output. 00231 * 00232 * @param repeat Tells how many samples to get from the device. Each sample takes 4 ms. 00233 * @return In forced conversion output mode, will display a raw output of the average sample. 00234 */ 00235 int get_ir(int repeat); 00236 00237 private: 00238 00239 I2C* i2c_; 00240 int bias1,bias2,bias3,PS1,PS2,PS3,VIS,IR; 00241 char LowB,HighB; 00242 00243 /** 00244 * Wait for the device to respond, then send it a specific command. 00245 * 00246 * @param cmd Command to send to the device. Read p20 of the datasheet for more details. 00247 */ 00248 void command(char cmd); 00249 00250 /** 00251 * Read a register from the device. 00252 * 00253 * @param address Register to read from. 00254 * @param num_data Bytes to read (always 1). 00255 * @return Contents of register. 00256 */ 00257 char read_reg(/*unsigned*/ char address, int num_data); 00258 00259 /** 00260 * Write to a register on the device. 00261 * 00262 * @param address Register to write to. 00263 * @param num_data Data that is to be written. 00264 */ 00265 void write_reg(char address, char num_data); 00266 00267 }; 00268 00269 #endif
Generated on Tue Jul 12 2022 21:16:37 by
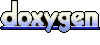