BLE_Nano nRF51 Central heart rate
Embed:
(wiki syntax)
Show/hide line numbers
system_nrf51.c
00001 /* Copyright (c) 2015, Nordic Semiconductor ASA 00002 * All rights reserved. 00003 * 00004 * Redistribution and use in source and binary forms, with or without 00005 * modification, are permitted provided that the following conditions are met: 00006 * 00007 * * Redistributions of source code must retain the above copyright notice, this 00008 * list of conditions and the following disclaimer. 00009 * 00010 * * Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 00014 * * Neither the name of Nordic Semiconductor ASA nor the names of its 00015 * contributors may be used to endorse or promote products derived from 00016 * this software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00019 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00020 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00021 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00022 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00023 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00024 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00025 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00026 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00027 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00028 * 00029 */ 00030 00031 /* NOTE: Template files (including this one) are application specific and therefore expected to 00032 be copied into the application project folder prior to its use! */ 00033 00034 #include <stdint.h> 00035 #include <stdbool.h> 00036 #include "nrf.h" 00037 #include "system_nrf51.h" 00038 00039 /*lint ++flb "Enter library region" */ 00040 00041 00042 #define __SYSTEM_CLOCK (16000000UL) /*!< nRF51 devices use a fixed System Clock Frequency of 16MHz */ 00043 00044 static bool is_manual_peripheral_setup_needed(void); 00045 static bool is_disabled_in_debug_needed(void); 00046 static bool is_peripheral_domain_setup_needed(void); 00047 00048 00049 #if defined ( __CC_ARM ) 00050 uint32_t SystemCoreClock __attribute__((used)) = __SYSTEM_CLOCK; 00051 #elif defined ( __ICCARM__ ) 00052 __root uint32_t SystemCoreClock = __SYSTEM_CLOCK; 00053 #elif defined ( __GNUC__ ) 00054 uint32_t SystemCoreClock __attribute__((used)) = __SYSTEM_CLOCK; 00055 #endif 00056 00057 void SystemCoreClockUpdate(void) 00058 { 00059 SystemCoreClock = __SYSTEM_CLOCK; 00060 } 00061 00062 void SystemInit(void) 00063 { 00064 /* If desired, switch off the unused RAM to lower consumption by the use of RAMON register. 00065 It can also be done in the application main() function. */ 00066 00067 /* Prepare the peripherals for use as indicated by the PAN 26 "System: Manual setup is required 00068 to enable the use of peripherals" found at Product Anomaly document for your device found at 00069 https://www.nordicsemi.com/. The side effect of executing these instructions in the devices 00070 that do not need it is that the new peripherals in the second generation devices (LPCOMP for 00071 example) will not be available. */ 00072 if (is_manual_peripheral_setup_needed()) 00073 { 00074 *(uint32_t volatile *)0x40000504 = 0xC007FFDF; 00075 *(uint32_t volatile *)0x40006C18 = 0x00008000; 00076 } 00077 00078 /* Disable PROTENSET registers under debug, as indicated by PAN 59 "MPU: Reset value of DISABLEINDEBUG 00079 register is incorrect" found at Product Anomaly document for your device found at 00080 https://www.nordicsemi.com/. There is no side effect of using these instruction if not needed. */ 00081 if (is_disabled_in_debug_needed()) 00082 { 00083 NRF_MPU->DISABLEINDEBUG = MPU_DISABLEINDEBUG_DISABLEINDEBUG_Disabled << MPU_DISABLEINDEBUG_DISABLEINDEBUG_Pos; 00084 } 00085 00086 /* Execute the following code to eliminate excessive current in sleep mode with RAM retention in nRF51802 devices, 00087 as indicated by PAN 76 "System: Excessive current in sleep mode with retention" found at Product Anomaly document 00088 for your device found at https://www.nordicsemi.com/. */ 00089 if (is_peripheral_domain_setup_needed()){ 00090 if (*(uint32_t volatile *)0x4006EC00 != 1){ 00091 *(uint32_t volatile *)0x4006EC00 = 0x9375; 00092 while (*(uint32_t volatile *)0x4006EC00 != 1){ 00093 } 00094 } 00095 *(uint32_t volatile *)0x4006EC14 = 0xC0; 00096 } 00097 } 00098 00099 00100 static bool is_manual_peripheral_setup_needed(void) 00101 { 00102 if ((((*(uint32_t *)0xF0000FE0) & 0x000000FF) == 0x1) && (((*(uint32_t *)0xF0000FE4) & 0x0000000F) == 0x0)) 00103 { 00104 if ((((*(uint32_t *)0xF0000FE8) & 0x000000F0) == 0x00) && (((*(uint32_t *)0xF0000FEC) & 0x000000F0) == 0x0)) 00105 { 00106 return true; 00107 } 00108 if ((((*(uint32_t *)0xF0000FE8) & 0x000000F0) == 0x10) && (((*(uint32_t *)0xF0000FEC) & 0x000000F0) == 0x0)) 00109 { 00110 return true; 00111 } 00112 if ((((*(uint32_t *)0xF0000FE8) & 0x000000F0) == 0x30) && (((*(uint32_t *)0xF0000FEC) & 0x000000F0) == 0x0)) 00113 { 00114 return true; 00115 } 00116 } 00117 00118 return false; 00119 } 00120 00121 static bool is_disabled_in_debug_needed(void) 00122 { 00123 if ((((*(uint32_t *)0xF0000FE0) & 0x000000FF) == 0x1) && (((*(uint32_t *)0xF0000FE4) & 0x0000000F) == 0x0)) 00124 { 00125 if ((((*(uint32_t *)0xF0000FE8) & 0x000000F0) == 0x40) && (((*(uint32_t *)0xF0000FEC) & 0x000000F0) == 0x0)) 00126 { 00127 return true; 00128 } 00129 } 00130 00131 return false; 00132 } 00133 00134 static bool is_peripheral_domain_setup_needed(void) 00135 { 00136 if ((((*(uint32_t *)0xF0000FE0) & 0x000000FF) == 0x1) && (((*(uint32_t *)0xF0000FE4) & 0x0000000F) == 0x0)) 00137 { 00138 if ((((*(uint32_t *)0xF0000FE8) & 0x000000F0) == 0xA0) && (((*(uint32_t *)0xF0000FEC) & 0x000000F0) == 0x0)) 00139 { 00140 return true; 00141 } 00142 if ((((*(uint32_t *)0xF0000FE8) & 0x000000F0) == 0xD0) && (((*(uint32_t *)0xF0000FEC) & 0x000000F0) == 0x0)) 00143 { 00144 return true; 00145 } 00146 } 00147 00148 return false; 00149 } 00150 00151 /*lint --flb "Leave library region" */ 00152
Generated on Wed Jul 13 2022 07:07:19 by
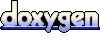