BLE_Nano nRF51 Central heart rate
Embed:
(wiki syntax)
Show/hide line numbers
nrf_log.h
00001 #ifndef NRF_LOG_H_ 00002 #define NRF_LOG_H_ 00003 00004 #ifndef DOXYGEN 00005 00006 #include <stdint.h> 00007 #include <stdarg.h> 00008 #include <app_util.h > 00009 00010 #ifndef NRF_LOG_USES_RTT 00011 #define NRF_LOG_USES_RTT 0 00012 #endif 00013 00014 #ifndef NRF_LOG_USES_UART 00015 #define NRF_LOG_USES_UART 0 00016 #endif 00017 00018 #ifndef NRF_LOG_USES_RAW_UART 00019 #define NRF_LOG_USES_RAW_UART 0 00020 #endif 00021 00022 #ifndef NRF_LOG_USES_COLORS 00023 #define NRF_LOG_USES_COLORS 1 00024 #endif 00025 00026 #if NRF_LOG_USES_COLORS == 1 00027 #define NRF_LOG_COLOR_DEFAULT "\x1B[0m" 00028 #define NRF_LOG_COLOR_BLACK "\x1B[1;30m" 00029 #define NRF_LOG_COLOR_RED "\x1B[1;31m" 00030 #define NRF_LOG_COLOR_GREEN "\x1B[1;32m" 00031 #define NRF_LOG_COLOR_YELLOW "\x1B[1;33m" 00032 #define NRF_LOG_COLOR_BLUE "\x1B[1;34m" 00033 #define NRF_LOG_COLOR_MAGENTA "\x1B[1;35m" 00034 #define NRF_LOG_COLOR_CYAN "\x1B[1;36m" 00035 #define NRF_LOG_COLOR_WHITE "\x1B[1;37m" 00036 #else 00037 #define NRF_LOG_COLOR_DEFAULT 00038 #define NRF_LOG_COLOR_BLACK 00039 #define NRF_LOG_COLOR_RED 00040 #define NRF_LOG_COLOR_GREEN 00041 #define NRF_LOG_COLOR_YELLOW 00042 #define NRF_LOG_COLOR_BLUE 00043 #define NRF_LOG_COLOR_MAGENTA 00044 #define NRF_LOG_COLOR_CYAN 00045 #define NRF_LOG_COLOR_WHITE 00046 #endif 00047 00048 #if defined(NRF_LOG_USES_RTT) && NRF_LOG_USES_RTT == 1 00049 00050 #define LOG_TERMINAL_NORMAL (0) 00051 #define LOG_TERMINAL_ERROR (1) 00052 #define LOG_TERMINAL_INPUT (0) 00053 00054 /**@brief Function for initializing the SEGGER RTT logger. 00055 * 00056 * @details See <a href="https://www.segger.com/jlink-rtt.html" target="_blank">segger.com</a> 00057 * for information about SEGGER Real Time Transfer (RTT). 00058 * 00059 * This function is available only when NRF_LOG_USES_RTT is defined as 1. 00060 * 00061 * @note Do not call this function directly. Use the macro @ref NRF_LOG_INIT instead. 00062 * 00063 * @retval NRF_SUCCESS If initialization was successful. 00064 * @retval NRF_ERROR Otherwise. 00065 */ 00066 uint32_t log_rtt_init(void); 00067 00068 /**@brief Function for writing a printf string using RTT. 00069 * 00070 * @details The printf implementation in SEGGER's RTT is more efficient than 00071 * the standard implementation. However, printf requires more processor time 00072 * than other logging functions. Therefore, applications that require logging 00073 * but need it to interfere as little as possible with the execution, should 00074 * avoid using printf. 00075 * 00076 * This function is available only when NRF_LOG_USES_RTT is defined as 1. 00077 * 00078 * @note Do not call this function directly. Use one of the following macros instead: 00079 * - @ref NRF_LOG_PRINTF 00080 * - @ref NRF_LOG_PRINTF_DEBUG 00081 * - @ref NRF_LOG_PRINTF_ERROR 00082 * 00083 * @param terminal_index Segger RTT terminal index to use as output. 00084 * @param format_msg Printf format string. 00085 */ 00086 void log_rtt_printf(int terminal_index, char * format_msg, ...); 00087 00088 /**@brief Function for writing a string using RTT. 00089 * 00090 * @details The string to write must be null-terminated, but the null termination will not be stored 00091 * in the ring buffer. 00092 * The impact of running this function should be very low compared to writing to UART. 00093 * 00094 * This function is available only when NRF_LOG_USES_RTT is defined as 1. 00095 * 00096 * @note Do not call this function directly. Use one of the following macros instead: 00097 * - @ref NRF_LOG 00098 * - @ref NRF_LOG_DEBUG 00099 * - @ref NRF_LOG_ERROR 00100 * 00101 * @param terminal_index Segger RTT terminal index to use as output. 00102 * @param num_args Number of arguments. 00103 */ 00104 void log_rtt_write_string(int terminal_index, int num_args, ...); 00105 00106 /**@brief Function for writing an integer as HEX using RTT. 00107 * 00108 * The output data is formatted as, for example, 0x89ABCDEF. 00109 * 00110 * This function is available only when NRF_LOG_USES_RTT is defined as 1. 00111 * 00112 * @note Do not call this function directly. Use one of the following macros instead: 00113 * - @ref NRF_LOG_HEX 00114 * - @ref NRF_LOG_HEX_DEBUG 00115 * - @ref NRF_LOG_HEX_ERROR 00116 * 00117 * @param terminal_index Segger RTT terminal index to use as output. 00118 * @param value Integer value to be printed as HEX. 00119 */ 00120 void log_rtt_write_hex(int terminal_index, uint32_t value); 00121 00122 /**@brief Function for writing a single character as HEX using RTT. 00123 * 00124 * The output string is formatted as, for example, AA. 00125 * 00126 * This function is available only when NRF_LOG_USES_RTT is defined as 1. 00127 * 00128 * @note Do not call this function directly. Use one of the following macros instead: 00129 * - @ref NRF_LOG_HEX_CHAR 00130 * - @ref NRF_LOG_HEX_CHAR_DEBUG 00131 * - @ref NRF_LOG_HEX_CHAR_ERROR 00132 * 00133 * @param terminal_index Segger RTT terminal index to use as output. 00134 * @param value Character to print as HEX. 00135 */ 00136 void log_rtt_write_hex_char(int terminal_index, uint8_t value); 00137 00138 /**@brief Function for checking if data is available in the input buffer. 00139 * 00140 * This function is available only when NRF_LOG_USES_RTT is defined as 1. 00141 * 00142 * @note Do not call this function directly. Use @ref NRF_LOG_HAS_INPUT instead. 00143 * 00144 * @retval 1 If characters are available to read. 00145 * @retval 0 If no characters are available. 00146 */ 00147 int log_rtt_has_input(void); 00148 00149 /**@brief Function for reading one character from the input buffer. 00150 * 00151 * @param[out] p_char Pointer where to store the character. 00152 * 00153 * This function is available only when NRF_LOG_USES_RTT is defined as 1. 00154 * 00155 * @note Do not call this function directly. Use @ref NRF_LOG_READ_INPUT instead. 00156 * 00157 * @retval NRF_SUCCESS If the character was read out. 00158 * @retval NRF_ERROR_INVALID_DATA If no character could be read. 00159 */ 00160 uint32_t log_rtt_read_input(char* p_char); 00161 00162 #define NRF_LOG_INIT() log_rtt_init() /*!< Initialize the module. */ 00163 00164 #define NRF_LOG_PRINTF(...) log_rtt_printf(LOG_TERMINAL_NORMAL, ##__VA_ARGS__) /*!< Print a log message using printf. */ 00165 #define NRF_LOG_PRINTF_DEBUG(...) log_rtt_printf(LOG_TERMINAL_NORMAL, ##__VA_ARGS__) /*!< If DEBUG is set, print a log message using printf. */ 00166 #define NRF_LOG_PRINTF_ERROR(...) log_rtt_printf(LOG_TERMINAL_ERROR, ##__VA_ARGS__) /*!< Print a log message using printf to the error stream. */ 00167 00168 #define NRF_LOG(...) log_rtt_write_string(LOG_TERMINAL_NORMAL, NUM_VA_ARGS(__VA_ARGS__), ##__VA_ARGS__) /*!< Print a log message. The input string must be null-terminated. */ 00169 #define NRF_LOG_DEBUG(...) log_rtt_write_string(LOG_TERMINAL_NORMAL, NUM_VA_ARGS(__VA_ARGS__), ##__VA_ARGS__) /*!< If DEBUG is set, print a log message. The input string must be null-terminated. */ 00170 #define NRF_LOG_ERROR(...) log_rtt_write_string(LOG_TERMINAL_ERROR, NUM_VA_ARGS(__VA_ARGS__), ##__VA_ARGS__) /*!< Print a log message to the error stream. The input string must be null-terminated. */ 00171 00172 #define NRF_LOG_HEX(val) log_rtt_write_hex(LOG_TERMINAL_NORMAL, val) /*!< Log an integer as HEX value (example output: 0x89ABCDEF). */ 00173 #define NRF_LOG_HEX_DEBUG(val) log_rtt_write_hex(LOG_TERMINAL_NORMAL, val) /*!< If DEBUG is set, log an integer as HEX value (example output: 0x89ABCDEF). */ 00174 #define NRF_LOG_HEX_ERROR(val) log_rtt_write_hex(LOG_TERMINAL_ERROR, val) /*!< Log an integer as HEX value to the error stream (example output: 0x89ABCDEF). */ 00175 00176 #define NRF_LOG_HEX_CHAR(val) log_rtt_write_hex_char(LOG_TERMINAL_NORMAL, val) /*!< Log a character as HEX value (example output: AA). */ 00177 #define NRF_LOG_HEX_CHAR_DEBUG(val) log_rtt_write_hex_char(LOG_TERMINAL_NORMAL, val) /*!< If DEBUG is set, log a character as HEX value (example output: AA). */ 00178 #define NRF_LOG_HEX_CHAR_ERROR(val) log_rtt_write_hex_char(LOG_TERMINAL_ERROR, val) /*!< Log a character as HEX value to the error stream (example output: AA). */ 00179 00180 #define NRF_LOG_HAS_INPUT() log_rtt_has_input() /*!< Check if the input buffer has unconsumed characters. */ 00181 #define NRF_LOG_READ_INPUT(p_char) log_rtt_read_input(p_char) /*!< Consume a character from the input buffer. */ 00182 00183 #if !defined(DEBUG) && !defined(DOXYGEN) 00184 00185 #undef NRF_LOG_DEBUG 00186 #define NRF_LOG_DEBUG(...) 00187 00188 #undef NRF_LOG_STR_DEBUG 00189 #define NRF_LOG_STR_DEBUG(...) 00190 00191 #undef NRF_LOG_HEX_DEBUG 00192 #define NRF_LOG_HEX_DEBUG(...) 00193 00194 #undef NRF_LOG_HEX_CHAR_DEBUG 00195 #define NRF_LOG_HEX_CHAR_DEBUG(...) 00196 00197 #endif // !defined(DEBUG) && !defined(DOXYGEN) 00198 00199 #elif defined(NRF_LOG_USES_UART) && NRF_LOG_USES_UART == 1 00200 00201 /**@brief Function for initializing the UART logger. 00202 * 00203 * This function is available only when NRF_LOG_USES_UART is defined as 1. 00204 * 00205 * @note Do not call this function directly. Use the macro @ref NRF_LOG_INIT instead. 00206 * 00207 * @retval NRF_SUCCESS If initialization was successful. 00208 * @retval NRF_ERROR Otherwise. 00209 */ 00210 uint32_t log_uart_init(void); 00211 00212 /**@brief Function for logging a printf string to UART. 00213 * 00214 * @details Printf requires more processor time 00215 * than other logging functions. Therefore, applications that require logging 00216 * but need it to interfere as little as possible with the execution, should 00217 * avoid using printf. 00218 * 00219 * This function is available only when NRF_LOG_USES_UART is defined as 1. 00220 * 00221 * @note This function is non-blocking. If too much data is sent to the UART, 00222 * some characters might be skipped. 00223 * 00224 * @note Do not call this function directly. Use one of the following macros instead: 00225 * - @ref NRF_LOG_PRINTF 00226 * - @ref NRF_LOG_PRINTF_DEBUG 00227 * - @ref NRF_LOG_PRINTF_ERROR 00228 * 00229 * @param format_msg Printf format string. 00230 */ 00231 void log_uart_printf(const char * format_msg, ...); 00232 00233 /**@brief Function for logging a single character to UART. 00234 * 00235 * This function is available only when NRF_LOG_USES_UART is defined as 1. 00236 * 00237 * @param c Character. 00238 */ 00239 void log_uart_write_char(const char c); 00240 00241 /**@brief Function for logging null-terminated strings to UART. 00242 * 00243 * @details This function is more efficient than using printf. 00244 * The null termination will not be logged. 00245 * 00246 * This function is available only when NRF_LOG_USES_UART is defined as 1. 00247 * 00248 * @note Do not call this function directly. Use one of the following macros instead: 00249 * - @ref NRF_LOG 00250 * - @ref NRF_LOG_DEBUG 00251 * - @ref NRF_LOG_ERROR 00252 * 00253 * @param num_args Number of arguments. 00254 */ 00255 void log_uart_write_string_many(int num_args, ...); 00256 00257 00258 /**@brief Function for logging a null-terminated string to UART. 00259 * 00260 * @details This function is more efficient than using printf. 00261 * The null termination will not be logged. 00262 * 00263 * This function is available only when NRF_LOG_USES_UART is defined as 1. 00264 * 00265 * @note Do not call this function directly. Use one of the following macros instead: 00266 * - @ref NRF_LOG 00267 * - @ref NRF_LOG_DEBUG 00268 * - @ref NRF_LOG_ERROR 00269 * 00270 * @param msg Null-terminated string. 00271 */ 00272 void log_uart_write_string(const char* msg); 00273 00274 00275 /**@brief Function for logging an integer value as HEX to UART. 00276 * 00277 * @details The output data is formatted as, for example, 0x89ABCDEF. 00278 * This function is more efficient than printf. 00279 * 00280 * This function is available only when NRF_LOG_USES_UART is defined as 1. 00281 * 00282 * @note This function is non-blocking. If too much data is sent to the UART, 00283 * some characters might be skipped. 00284 * 00285 * @note Do not call this function directly. Use one of the following macros instead: 00286 * - @ref NRF_LOG_HEX 00287 * - @ref NRF_LOG_HEX_DEBUG 00288 * - @ref NRF_LOG_HEX_ERROR 00289 * 00290 * @param value Integer value to be printed as HEX. 00291 */ 00292 void log_uart_write_hex(uint32_t value); 00293 00294 /**@brief Function for logging a single character as HEX to UART. 00295 * 00296 * @details The output string is formatted as, for example, AA. 00297 * 00298 * This function is available only when NRF_LOG_USES_UART is defined as 1. 00299 * 00300 * @note This function is non-blocking. If too much data is sent to the UART, 00301 * some characters might be skipped. 00302 * 00303 * @note Do not call this function directly. Use one of the following macros instead: 00304 * - @ref NRF_LOG_HEX_CHAR 00305 * - @ref NRF_LOG_HEX_CHAR_DEBUG 00306 * - @ref NRF_LOG_HEX_CHAR_ERROR 00307 * 00308 * @param c Character. 00309 */ 00310 void log_uart_write_hex_char(uint8_t c); 00311 00312 /**@brief Function for checking if data is available in the input buffer. 00313 * 00314 * This function is available only when NRF_LOG_USES_UART is defined as 1. 00315 * 00316 * @note Do not call this function directly. Use @ref NRF_LOG_HAS_INPUT instead. 00317 * 00318 * @retval 1 If characters are available to read. 00319 * @retval 0 If no characters are available. 00320 */ 00321 int log_uart_has_input(void); 00322 00323 /**@brief Function for reading one character from the input buffer. 00324 * 00325 * @param[out] p_char Pointer where to store the character. 00326 * 00327 * This function is available only when NRF_LOG_USES_UART is defined as 1. 00328 * 00329 * @note Do not call this function directly. Use NRF_LOG_READ_INPUT instead. 00330 * 00331 * @retval NRF_SUCCESS If the character was read out. 00332 * @retval NRF_ERROR_INVALID_DATA If no character could be read. 00333 */ 00334 uint32_t log_uart_read_input(char* p_char); 00335 00336 00337 #define NRF_LOG_INIT() log_uart_init() /*!< Initialize the module. */ 00338 00339 #define NRF_LOG_PRINTF(...) log_uart_printf(__VA_ARGS__) /*!< Print a log message using printf. */ 00340 #define NRF_LOG_PRINTF_DEBUG(...) log_uart_printf(__VA_ARGS__) /*!< If DEBUG is set, print a log message using printf. */ 00341 #define NRF_LOG_PRINTF_ERROR(...) log_uart_printf(__VA_ARGS__) /*!< Print a log message using printf to the error stream. */ 00342 00343 #define NRF_LOG(...) log_uart_write_string_many(NUM_VA_ARGS(__VA_ARGS__), ##__VA_ARGS__) /*!< Print a log message. The input string must be null-terminated. */ 00344 #define NRF_LOG_DEBUG(...) log_uart_write_string_many(NUM_VA_ARGS(__VA_ARGS__), ##__VA_ARGS__) /*!< If DEBUG is set, print a log message. The input string must be null-terminated. */ 00345 #define NRF_LOG_ERROR(...) log_uart_write_string_many(NUM_VA_ARGS(__VA_ARGS__), ##__VA_ARGS__) /*!< Print a log message to the error stream. The input string must be null-terminated. */ 00346 00347 #define NRF_LOG_HEX(val) log_uart_write_hex(val) /*!< Log an integer as HEX value (example output: 0x89ABCDEF). */ 00348 #define NRF_LOG_HEX_DEBUG(val) log_uart_write_hex(val) /*!< If DEBUG is set, log an integer as HEX value (example output: 0x89ABCDEF). */ 00349 #define NRF_LOG_HEX_ERROR(val) log_uart_write_hex(val) /*!< Log an integer as HEX value to the error stream (example output: 0x89ABCDEF). */ 00350 00351 #define NRF_LOG_HEX_CHAR(val) log_uart_write_hex_char(val) /*!< Log a character as HEX value (example output: AA). */ 00352 #define NRF_LOG_HEX_CHAR_DEBUG(val) log_uart_write_hex_char(val) /*!< If DEBUG is set, log a character as HEX value (example output: AA). */ 00353 #define NRF_LOG_HEX_CHAR_ERROR(val) log_uart_write_hex_char(val) /*!< Log a character as HEX value to the error stream (example output: AA). */ 00354 00355 #define NRF_LOG_HAS_INPUT() log_uart_has_input() /*!< Check if the input buffer has unconsumed characters. */ 00356 #define NRF_LOG_READ_INPUT(p_char) log_uart_read_input(p_char) /*!< Consume a character from the input buffer. */ 00357 00358 #if !defined(DEBUG) && !defined(DOXYGEN) 00359 00360 #undef NRF_LOG_DEBUG 00361 #define NRF_LOG_DEBUG(...) 00362 00363 #undef NRF_LOG_PRINTF_DEBUG 00364 #define NRF_LOG_PRINTF_DEBUG(...) 00365 00366 #undef NRF_LOG_STR_DEBUG 00367 #define NRF_LOG_STR_DEBUG(...) 00368 00369 #undef NRF_LOG_HEX_DEBUG 00370 #define NRF_LOG_HEX_DEBUG(...) 00371 00372 #undef NRF_LOG_HEX_CHAR_DEBUG 00373 #define NRF_LOG_HEX_CHAR_DEBUG(...) 00374 00375 #endif // !defined(DEBUG) && !defined(DOXYGEN) 00376 00377 #elif defined(NRF_LOG_USES_RAW_UART) && NRF_LOG_USES_RAW_UART == 1 00378 00379 /**@brief Function for initializing the raw UART logger. 00380 * 00381 * This function is available only when NRF_LOG_USES_RAW_UART is defined as 1. 00382 * 00383 * @note Do not call this function directly. Use the macro @ref NRF_LOG_INIT instead. 00384 * 00385 * @retval NRF_SUCCESS If initialization was successful. 00386 * @retval NRF_ERROR Otherwise. 00387 */ 00388 uint32_t log_raw_uart_init(void); 00389 00390 /**@brief Function for logging a printf string to raw UART. 00391 * 00392 * @details Printf requires more processor time 00393 * than other logging functions. Therefore, applications that require logging 00394 * but need it to interfere as little as possible with the execution, should 00395 * avoid using printf. 00396 * 00397 * This function is available only when NRF_LOG_USES_RAW_UART is defined as 1. 00398 * 00399 * @note This function is non-blocking. If too much data is sent to the UART, 00400 * some characters might be skipped. 00401 * 00402 * @note Do not call this function directly. Use one of the following macros instead: 00403 * - @ref NRF_LOG_PRINTF 00404 * - @ref NRF_LOG_PRINTF_DEBUG 00405 * - @ref NRF_LOG_PRINTF_ERROR 00406 * 00407 * @param format_msg Printf format string. 00408 */ 00409 void log_raw_uart_printf(const char * format_msg, ...); 00410 00411 /**@brief Function for logging a single character to raw UART. 00412 * 00413 * This function is available only when NRF_LOG_USES_RAW_UART is defined as 1. 00414 * 00415 * @param c Character. 00416 */ 00417 void log_raw_uart_write_char(const char c); 00418 00419 /**@brief Function for logging null-terminated strings to raw UART. 00420 * 00421 * @details This function is more efficient than using printf. 00422 * The null termination will not be logged. 00423 * 00424 * This function is available only when NRF_LOG_USES_RAW_UART is defined as 1. 00425 * 00426 * @note Do not call this function directly. Use one of the following macros instead: 00427 * - @ref NRF_LOG 00428 * - @ref NRF_LOG_DEBUG 00429 * - @ref NRF_LOG_ERROR 00430 * 00431 * @param num_args Number of arguments. 00432 */ 00433 void log_raw_uart_write_string_many(int num_args, ...); 00434 00435 00436 /**@brief Function for logging a null-terminated string to raw UART. 00437 * 00438 * @details This function is more efficient than using printf. 00439 * The null termination will not be logged. 00440 * 00441 * This function is available only when NRF_LOG_USES_RAW_UART is defined as 1. 00442 * 00443 * @note Do not call this function directly. Use one of the following macros instead: 00444 * - @ref NRF_LOG 00445 * - @ref NRF_LOG_DEBUG 00446 * - @ref NRF_LOG_ERROR 00447 * 00448 * @param str Null-terminated string. 00449 */ 00450 void log_raw_uart_write_string(const char * str); 00451 00452 /**@brief Function for logging an integer value as HEX to raw UART. 00453 * 00454 * @details The output data is formatted as, for example, 0x89ABCDEF. 00455 * This function is more efficient than printf. 00456 * 00457 * This function is available only when NRF_LOG_USES_RAW_UART is defined as 1. 00458 * 00459 * @note This function is non-blocking. If too much data is sent to the UART, 00460 * some characters might be skipped. 00461 * 00462 * @note Do not call this function directly. Use one of the following macros instead: 00463 * - @ref NRF_LOG_HEX 00464 * - @ref NRF_LOG_HEX_DEBUG 00465 * - @ref NRF_LOG_HEX_ERROR 00466 * 00467 * @param value Integer value to be printed as HEX. 00468 */ 00469 void log_raw_uart_write_hex(uint32_t value); 00470 00471 /**@brief Function for logging a single character as HEX to raw UART. 00472 * 00473 * @details The output string is formatted as, for example, AA. 00474 * 00475 * This function is available only when NRF_LOG_USES_RAW_UART is defined as 1. 00476 * 00477 * @note This function is non-blocking. If too much data is sent to the UART, 00478 * some characters might be skipped. 00479 * 00480 * @note Do not call this function directly. Use one of the following macros instead: 00481 * - @ref NRF_LOG_HEX_CHAR 00482 * - @ref NRF_LOG_HEX_CHAR_DEBUG 00483 * - @ref NRF_LOG_HEX_CHAR_ERROR 00484 * 00485 * @param c Character. 00486 */ 00487 void log_raw_uart_write_hex_char(uint8_t c); 00488 00489 /**@brief Function for checking if data is available in the input buffer. 00490 * 00491 * This function is available only when NRF_LOG_USES_RAW_UART is defined as 1. 00492 * 00493 * @note Do not call this function directly. Use @ref NRF_LOG_HAS_INPUT instead. 00494 * 00495 * @retval 1 If characters are available to read. 00496 * @retval 0 If no characters are available. 00497 */ 00498 int log_raw_uart_has_input(void); 00499 00500 /**@brief Function for reading one character from the input buffer. 00501 * 00502 * @param[out] p_char Pointer where to store the character. 00503 * 00504 * This function is available only when NRF_LOG_USES_RAW_UART is defined as 1. 00505 * 00506 * @note Do not call this function directly. Use NRF_LOG_READ_INPUT instead. 00507 * 00508 * @retval NRF_SUCCESS If the character was read out. 00509 * @retval NRF_ERROR_INVALID_DATA If no character could be read. 00510 */ 00511 00512 uint32_t log_raw_uart_read_input(char* p_char); 00513 00514 #define NRF_LOG_INIT() log_raw_uart_init() /*!< nitialize the module. */ 00515 00516 #define NRF_LOG_PRINTF(...) log_raw_uart_printf(__VA_ARGS__) /*!< Print a log message using printf. */ 00517 #define NRF_LOG_PRINTF_DEBUG(...) log_raw_uart_printf(__VA_ARGS__) /*!< If DEBUG is set, print a log message using printf. */ 00518 #define NRF_LOG_PRINTF_ERROR(...) log_raw_uart_printf(__VA_ARGS__) /*!< Print a log message using printf to the error stream. */ 00519 00520 #define NRF_LOG(...) log_raw_uart_write_string_many(NUM_VA_ARGS(__VA_ARGS__), ##__VA_ARGS__) /*!< Print a log message. The input string must be null-terminated. */ 00521 #define NRF_LOG_DEBUG(...) log_raw_uart_write_string_many(NUM_VA_ARGS(__VA_ARGS__), ##__VA_ARGS__) /*!< If DEBUG is set, print a log message. The input string must be null-terminated. */ 00522 #define NRF_LOG_ERROR(...) log_raw_uart_write_string_many(NUM_VA_ARGS(__VA_ARGS__), ##__VA_ARGS__) /*!< Print a log message to the error stream. The input string must be null-terminated. */ 00523 00524 #define NRF_LOG_HEX(val) log_raw_uart_write_hex(val) /*!< Log an integer as HEX value (example output: 0x89ABCDEF). */ 00525 #define NRF_LOG_HEX_DEBUG(val) log_raw_uart_write_hex(val) /*!< If DEBUG is set, log an integer as HEX value (example output: 0x89ABCDEF). */ 00526 #define NRF_LOG_HEX_ERROR(val) log_raw_uart_write_hex(val) /*!< Log an integer as HEX value to the error stream (example output: 0x89ABCDEF). */ 00527 00528 #define NRF_LOG_HEX_CHAR(val) log_raw_uart_write_hex_char(val) /*!< Log a character as HEX value (example output: AA). */ 00529 #define NRF_LOG_HEX_CHAR_DEBUG(val) log_raw_uart_write_hex_char(val) /*!< If DEBUG is set, log a character as HEX value (example output: AA). */ 00530 #define NRF_LOG_HEX_CHAR_ERROR(val) log_raw_uart_write_hex_char(val) /*!< Log a character as HEX value to the error stream (example output: AA). */ 00531 00532 #define NRF_LOG_HAS_INPUT() log_raw_uart_has_input() /*!< Check if the input buffer has unconsumed characters. */ 00533 #define NRF_LOG_READ_INPUT(p_char) log_raw_uart_read_input(p_char) /*!< Consume a character from the input buffer. */ 00534 00535 #if !defined(DEBUG) && !defined(DOXYGEN) 00536 00537 #undef NRF_LOG_DEBUG 00538 #define NRF_LOG_DEBUG(...) 00539 00540 #undef NRF_LOG_PRINTF_DEBUG 00541 #define NRF_LOG_PRINTF_DEBUG(...) 00542 00543 #undef NRF_LOG_STR_DEBUG 00544 #define NRF_LOG_STR_DEBUG(...) 00545 00546 #undef NRF_LOG_HEX_DEBUG 00547 #define NRF_LOG_HEX_DEBUG(...) 00548 00549 #undef NRF_LOG_HEX_CHAR_DEBUG 00550 #define NRF_LOG_HEX_CHAR_DEBUG(...) 00551 00552 #endif // !defined(DEBUG) && !defined(DOXYGEN) 00553 00554 #else 00555 00556 #include "nrf_error.h" 00557 #include "nordic_common.h" 00558 00559 // Empty definitions 00560 00561 #define NRF_LOG_INIT() NRF_SUCCESS 00562 #define NRF_LOG(...) 00563 #define NRF_LOG_DEBUG(...) 00564 #define NRF_LOG_ERROR(...) 00565 00566 #define NRF_LOG_PRINTF(...) 00567 #define NRF_LOG_PRINTF_DEBUG(...) 00568 #define NRF_LOG_PRINTF_ERROR(...) 00569 00570 #define NRF_LOG_HEX(val) 00571 #define NRF_LOG_HEX_DEBUG(val) 00572 #define NRF_LOG_HEX_ERROR(val) 00573 00574 #define NRF_LOG_HEX_CHAR(val) 00575 #define NRF_LOG_HEX_CHAR_DEBUG(val) 00576 #define NRF_LOG_HEX_CHAR_ERROR(val) 00577 00578 #define NRF_LOG_HAS_INPUT() 0 00579 #define NRF_LOG_READ_INPUT(ignore) NRF_SUCCESS 00580 00581 #endif 00582 00583 /**@brief Function for writing HEX values. 00584 * 00585 * @note This function not thread-safe. It is written for convenience. 00586 * If you log from different application contexts, you might get different results. 00587 * 00588 * @retval NULL By default. 00589 */ 00590 const char* log_hex(uint32_t value); 00591 00592 /**@brief Function for writing HEX characters. 00593 * 00594 * @note This function not thread-safe. It is written for convenience. 00595 * If you log from different application contexts, you might get different results. 00596 * 00597 * @retval NULL By default. 00598 */ 00599 const char* log_hex_char(const char value); 00600 00601 00602 00603 00604 #else // DOXYGEN 00605 00606 /** @defgroup nrf_log UART/RTT logging 00607 * @{ 00608 * @ingroup app_common 00609 * 00610 * @brief Library to output logging information over SEGGER's Real Time Transfer 00611 * (RTT), UART, or raw UART. 00612 * 00613 * This library provides macros that call the respective functions depending on 00614 * which protocol is used. Define LOG_USES_RTT=1 to enable logging over RTT, 00615 * NRF_LOG_USES_UART=1 to enable logging over UART, or NRF_LOG_USES_RAW_UART=1 00616 * to enable logging over raw UART. One of these defines must be set for any of 00617 * the macros to have effect. If you choose to not output information, all 00618 * logging macros can be left in the code without any cost; they will just be 00619 * ignored. 00620 */ 00621 00622 00623 00624 /**@brief Macro for initializing the logger. 00625 * 00626 * @retval NRF_SUCCESS If initialization was successful. 00627 * @retval NRF_ERROR Otherwise. 00628 */ 00629 uint32_t NRF_LOG_INIT(void); 00630 00631 /**@brief Macro for logging null-terminated strings. 00632 * 00633 * @details This function is more efficient than using printf. 00634 * The null termination will not be logged. 00635 * 00636 * @param msg Null-terminated string. 00637 */ 00638 void NRF_LOG(const char* msg); 00639 00640 /**@brief Macro for logging a printf string. 00641 * 00642 * @details Printf requires more processor time 00643 * than other logging functions. Therefore, applications that require logging 00644 * but need it to interfere as little as possible with the execution, should 00645 * avoid using printf. 00646 * 00647 * @note When NRF_LOG_USES_UART is set to 1, this macro is non-blocking. 00648 * If too much data is sent, some characters might be skipped. 00649 * 00650 * @param format_msg Printf format string. 00651 * @param ... Additional arguments replacing format specifiers in format_msg. 00652 */ 00653 void NRF_LOG_PRINTF(const char * format_msg, ...); 00654 00655 /**@brief Macro for logging an integer value as HEX. 00656 * 00657 * @details The output data is formatted as, for example, 0x89ABCDEF. 00658 * This function is more efficient than printf. 00659 * 00660 * @note When NRF_LOG_USES_UART is set to 1, this macro is non-blocking. 00661 * If too much data is sent, some characters might be skipped. 00662 * 00663 * @param value Integer value to be printed as HEX. 00664 */ 00665 void NRF_LOG_HEX(uint32_t value); 00666 00667 /**@brief Macro for logging a single character as HEX. 00668 * 00669 * @details The output string is formatted as, for example, AA. 00670 * 00671 * @note When NRF_LOG_USES_UART is set to 1, this macro is non-blocking. 00672 * If too much data is sent, some characters might be skipped. 00673 * 00674 * @param c Character. 00675 */ 00676 void NRF_LOG_HEX_CHAR(uint8_t c); 00677 00678 /**@brief Macro for checking if data is available in the input buffer. 00679 * 00680 * @note When NRF_LOG_USES_UART is set to 1, this macro is non-blocking. 00681 * If too much data is sent, some characters might be skipped. 00682 * 00683 * @retval 1 If characters are available to read. 00684 * @retval 0 If no characters are available. 00685 */ 00686 int NRF_LOG_HAS_INPUT(void); 00687 00688 /**@brief Macro for reading one character from the input buffer. 00689 * 00690 * @param[out] p_char Pointer where to store the character. 00691 * 00692 * @retval NRF_SUCCESS If the character was read out. 00693 * @retval NRF_ERROR_INVALID_DATA If no character could be read. 00694 */ 00695 uint32_t NRF_LOG_READ_INPUT(char* p_char); 00696 00697 /** @} */ 00698 #endif // DOXYGEN 00699 #endif // NRF_LOG_H_ 00700
Generated on Wed Jul 13 2022 07:07:19 by
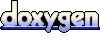