BLE_Nano nRF51 Central heart rate
Embed:
(wiki syntax)
Show/hide line numbers
nrf_log.c
00001 #include "nrf.h" 00002 #include "nrf_log.h" 00003 #include "nrf_error.h" 00004 #include <stdarg.h> 00005 #include <string.h> 00006 #include <stdio.h> 00007 00008 #if defined(NRF_LOG_USES_RTT) && NRF_LOG_USES_RTT == 1 00009 00010 #include <SEGGER_RTT_Conf.h> 00011 #include <SEGGER_RTT.h> 00012 00013 static char buf_normal_up[BUFFER_SIZE_UP]; 00014 static char buf_down[BUFFER_SIZE_DOWN]; 00015 00016 uint32_t log_rtt_init(void) 00017 { 00018 static bool initialized = false; 00019 if (initialized) 00020 { 00021 return NRF_SUCCESS; 00022 } 00023 00024 if (SEGGER_RTT_ConfigUpBuffer(LOG_TERMINAL_NORMAL, 00025 "Normal", 00026 buf_normal_up, 00027 BUFFER_SIZE_UP, 00028 SEGGER_RTT_MODE_NO_BLOCK_TRIM 00029 ) 00030 != 0) 00031 { 00032 return NRF_ERROR_INVALID_STATE; 00033 } 00034 00035 if (SEGGER_RTT_ConfigDownBuffer(LOG_TERMINAL_INPUT, 00036 "Input", 00037 buf_down, 00038 BUFFER_SIZE_DOWN, 00039 SEGGER_RTT_MODE_NO_BLOCK_SKIP 00040 ) 00041 != 0) 00042 { 00043 return NRF_ERROR_INVALID_STATE; 00044 } 00045 00046 initialized = true; 00047 00048 return NRF_SUCCESS; 00049 } 00050 00051 // Forward declaration of SEGGER RTT vprintf function 00052 int SEGGER_RTT_vprintf(unsigned BufferIndex, const char * sFormat, va_list * pParamList); 00053 00054 void log_rtt_printf(int terminal_index, char * format_msg, ...) 00055 { 00056 //lint -save -e526 -e628 -e530 00057 va_list p_args; 00058 va_start(p_args, format_msg); 00059 (void)SEGGER_RTT_vprintf(terminal_index, format_msg, &p_args); 00060 va_end(p_args); 00061 //lint -restore 00062 } 00063 00064 __INLINE void log_rtt_write_string(int terminal_index, int num_args, ...) 00065 { 00066 const char* msg; 00067 //lint -save -e516 -e530 00068 va_list p_args; 00069 va_start(p_args, num_args); 00070 //lint -restore 00071 00072 for (int i = 0; i < num_args; i++) 00073 { 00074 //lint -save -e26 -e10 -e64 -e526 -e628 -e530 00075 msg = va_arg(p_args, const char*); 00076 //lint -restore 00077 (void)SEGGER_RTT_WriteString(terminal_index, msg); 00078 } 00079 va_end(p_args); 00080 } 00081 00082 void log_rtt_write_hex(int terminal_index, uint32_t value) 00083 { 00084 char temp[11]; 00085 temp[0] = '0'; 00086 temp[1] = 'x'; 00087 temp[10] = 0; // Null termination 00088 uint8_t nibble; 00089 uint8_t i = 8; 00090 00091 while(i-- != 0) 00092 { 00093 nibble = (value >> (4 * i)) & 0x0F; 00094 temp[9-i] = (nibble > 9) ? ('A' + nibble - 10) : ('0' + nibble); 00095 } 00096 00097 (void)SEGGER_RTT_WriteString(terminal_index, temp); 00098 } 00099 00100 void log_rtt_write_hex_char(int terminal_index, uint8_t value) 00101 { 00102 char temp[3]; 00103 temp[2] = 0; // Null termination 00104 uint8_t nibble; 00105 uint8_t i = 2; 00106 00107 while(i-- != 0) 00108 { 00109 nibble = (value >> (4 * i)) & 0x0F; 00110 temp[1-i] = (nibble > 9) ? ('A' + nibble - 10) : ('0' + nibble); 00111 } 00112 00113 (void)SEGGER_RTT_WriteString(terminal_index, temp); 00114 } 00115 00116 __INLINE int log_rtt_has_input() 00117 { 00118 return SEGGER_RTT_HasKey(); 00119 } 00120 00121 uint32_t log_rtt_read_input(char * c) 00122 { 00123 int r; 00124 00125 r = SEGGER_RTT_Read(LOG_TERMINAL_INPUT, c, 1); 00126 if (r == 1) 00127 return NRF_SUCCESS; 00128 else 00129 return NRF_ERROR_NULL; 00130 } 00131 00132 #elif defined(NRF_LOG_USES_UART) && NRF_LOG_USES_UART == 1 00133 00134 #include "app_uart.h" 00135 #include "app_error.h " 00136 #include <stdio.h> 00137 #include <string.h> 00138 #include "nrf.h" 00139 #include "bsp.h" 00140 00141 #define MAX_TEST_DATA_BYTES (15U) /**< max number of test bytes to be used for tx and rx. */ 00142 #define UART_TX_BUF_SIZE 512 /**< UART TX buffer size. */ 00143 #define UART_RX_BUF_SIZE 1 /**< UART RX buffer size. */ 00144 00145 static uint8_t m_uart_data; 00146 static bool m_uart_has_input; 00147 00148 void uart_error_cb(app_uart_evt_t * p_event) 00149 { 00150 if (p_event->evt_type == APP_UART_COMMUNICATION_ERROR) 00151 { 00152 APP_ERROR_HANDLER(p_event->data.error_communication); 00153 } 00154 else if (p_event->evt_type == APP_UART_FIFO_ERROR) 00155 { 00156 APP_ERROR_HANDLER(p_event->data.error_code); 00157 } 00158 } 00159 00160 uint32_t log_uart_init() 00161 { 00162 static bool initialized = false; 00163 if (initialized) 00164 { 00165 return NRF_SUCCESS; 00166 } 00167 00168 uint32_t err_code; 00169 const app_uart_comm_params_t comm_params = 00170 { 00171 RX_PIN_NUMBER, 00172 TX_PIN_NUMBER, 00173 RTS_PIN_NUMBER, 00174 CTS_PIN_NUMBER, 00175 APP_UART_FLOW_CONTROL_ENABLED, 00176 false, 00177 UART_BAUDRATE_BAUDRATE_Baud115200 00178 }; 00179 00180 APP_UART_FIFO_INIT(&comm_params, 00181 UART_RX_BUF_SIZE, 00182 UART_TX_BUF_SIZE, 00183 uart_error_cb, 00184 APP_IRQ_PRIORITY_LOW, 00185 err_code); 00186 00187 initialized = true; 00188 00189 return err_code; 00190 } 00191 00192 //lint -save -e530 -e64 00193 void log_uart_printf(const char * format_msg, ...) 00194 { 00195 va_list p_args; 00196 va_start(p_args, format_msg); 00197 (void)vprintf(format_msg, p_args); 00198 va_end(p_args); 00199 } 00200 00201 __INLINE void log_uart_write_string_many(int num_args, ...) 00202 { 00203 const char* msg; 00204 va_list p_args; 00205 va_start(p_args, num_args); 00206 00207 for (int i = 0; i < num_args; i++) 00208 { 00209 msg = va_arg(p_args, const char*); 00210 log_uart_write_string(msg); 00211 } 00212 va_end(p_args); 00213 } 00214 00215 __INLINE void log_uart_write_string(const char* msg) 00216 { 00217 while( *msg ) 00218 { 00219 (void)app_uart_put(*msg++); 00220 } 00221 } 00222 //lint -restore 00223 00224 void log_uart_write_hex(uint32_t value) 00225 { 00226 uint8_t nibble; 00227 uint8_t i = 8; 00228 00229 (void)app_uart_put('0'); 00230 (void)app_uart_put('x'); 00231 while( i-- != 0 ) 00232 { 00233 nibble = (value >> (4 * i)) & 0x0F; 00234 (void)app_uart_put( (nibble > 9) ? ('A' + nibble - 10) : ('0' + nibble) ); 00235 } 00236 } 00237 00238 void log_uart_write_hex_char(uint8_t c) 00239 { 00240 uint8_t nibble; 00241 uint8_t i = 2; 00242 00243 while( i-- != 0 ) 00244 { 00245 nibble = (c >> (4 * i)) & 0x0F; 00246 (void)app_uart_put( (nibble > 9) ? ('A' + nibble - 10) : ('0' + nibble) ); 00247 } 00248 } 00249 00250 __INLINE int log_uart_has_input() 00251 { 00252 if (m_uart_has_input) return 1; 00253 if (app_uart_get(&m_uart_data) == NRF_SUCCESS) 00254 { 00255 m_uart_has_input = true; 00256 return 1; 00257 } 00258 return 0; 00259 } 00260 00261 uint32_t log_uart_read_input(char * c) 00262 { 00263 if (m_uart_has_input) 00264 { 00265 *c = (char)m_uart_data; 00266 m_uart_has_input = false; 00267 return NRF_SUCCESS; 00268 } 00269 if (app_uart_get((uint8_t *)c) == NRF_SUCCESS) 00270 { 00271 return NRF_SUCCESS; 00272 } 00273 return NRF_ERROR_NULL; 00274 } 00275 00276 #elif defined(NRF_LOG_USES_RAW_UART) && NRF_LOG_USES_RAW_UART == 1 00277 00278 #include "app_uart.h" 00279 #include <stdio.h> 00280 #include <string.h> 00281 #include "bsp.h" 00282 00283 uint32_t log_raw_uart_init() 00284 { 00285 // Disable UART 00286 NRF_UART0->ENABLE = UART_ENABLE_ENABLE_Disabled; 00287 00288 // Configure RX/TX pins 00289 nrf_gpio_cfg_output( TX_PIN_NUMBER ); 00290 nrf_gpio_cfg_input(RX_PIN_NUMBER, NRF_GPIO_PIN_NOPULL); 00291 00292 // Set a default baud rate of UART0_CONFIG_BAUDRATE 00293 NRF_UART0->PSELTXD = TX_PIN_NUMBER; 00294 NRF_UART0->BAUDRATE = UART0_CONFIG_BAUDRATE; 00295 00296 NRF_UART0->PSELRTS = 0xFFFFFFFF; 00297 NRF_UART0->PSELCTS = 0xFFFFFFFF; 00298 00299 // Disable parity and interrupt 00300 NRF_UART0->CONFIG = (UART_CONFIG_PARITY_Excluded << UART_CONFIG_PARITY_Pos ); 00301 NRF_UART0->CONFIG |= (UART_CONFIG_HWFC_Disabled << UART_CONFIG_HWFC_Pos ); 00302 00303 // Re-enable the UART 00304 NRF_UART0->ENABLE = UART_ENABLE_ENABLE_Enabled; 00305 NRF_UART0->INTENSET = 0; 00306 NRF_UART0->TASKS_STARTTX = 1; 00307 NRF_UART0->TASKS_STARTRX = 1; 00308 00309 return NRF_SUCCESS; 00310 } 00311 00312 void log_raw_uart_printf(const char * format_msg, ...) 00313 { 00314 static char buffer[256]; 00315 00316 va_list p_args; 00317 va_start(p_args, format_msg); 00318 sprintf(buffer, format_msg, p_args); 00319 va_end(p_args); 00320 00321 log_raw_uart_write_string(buffer); 00322 } 00323 00324 __INLINE void log_raw_uart_write_char(const char c) 00325 { 00326 NRF_UART0->TXD = c; 00327 while( NRF_UART0->EVENTS_TXDRDY != 1 ); 00328 NRF_UART0->EVENTS_TXDRDY = 0; 00329 } 00330 00331 __INLINE void log_raw_uart_write_string_many(int num_args, ...) 00332 { 00333 00334 const char* msg; 00335 va_list p_args; 00336 va_start(p_args, num_args); 00337 00338 for (int i = 0; i < num_args; i++) 00339 { 00340 msg = va_arg(p_args, const char*); 00341 log_raw_uart_write_string(msg); 00342 } 00343 va_end(p_args); 00344 } 00345 00346 __INLINE void log_raw_uart_write_string(const char* msg) 00347 { 00348 while( *msg ) 00349 { 00350 NRF_UART0->TXD = *msg++; 00351 while( NRF_UART0->EVENTS_TXDRDY != 1 ); 00352 NRF_UART0->EVENTS_TXDRDY = 0; 00353 } 00354 } 00355 00356 void log_raw_uart_write_hex(uint32_t value) 00357 { 00358 uint8_t nibble; 00359 uint8_t i = 8; 00360 00361 log_raw_uart_write_string( "0x" ); 00362 while( i-- != 0 ) 00363 { 00364 nibble = (value >> (4 * i)) & 0x0F; 00365 log_raw_uart_write_char( (nibble > 9) ? ('A' + nibble - 10) : ('0' + nibble) ); 00366 } 00367 } 00368 00369 void log_raw_uart_write_hex_char(uint8_t c) 00370 { 00371 uint8_t nibble; 00372 uint8_t i = 2; 00373 00374 while( i-- != 0 ) 00375 { 00376 nibble = (c >> (4 * i)) & 0x0F; 00377 log_raw_uart_write_hex( (nibble > 9) ? ('A' + nibble - 10) : ('0' + nibble) ); 00378 } 00379 } 00380 00381 __INLINE int log_raw_uart_has_input() 00382 { 00383 return 0; 00384 } 00385 00386 uint32_t log_raw_uart_read_input(char * c) 00387 { 00388 return NRF_ERROR_NULL; 00389 } 00390 00391 #endif // NRF_LOG_USES_RAW_UART == 1 00392 00393 00394 const char* log_hex_char(const char c) 00395 { 00396 static volatile char hex_string[3]; 00397 hex_string[2] = 0; // Null termination 00398 uint8_t nibble; 00399 uint8_t i = 2; 00400 while(i-- != 0) 00401 { 00402 nibble = (c >> (4 * i)) & 0x0F; 00403 hex_string[1-i] = (nibble > 9) ? ('A' + nibble - 10) : ('0' + nibble); 00404 } 00405 return (const char*) hex_string; 00406 } 00407 00408 const char* log_hex(uint32_t value) 00409 { 00410 static volatile char hex_string[11]; 00411 hex_string[0] = '0'; 00412 hex_string[1] = 'x'; 00413 hex_string[10] = 0; 00414 uint8_t nibble; 00415 uint8_t i = 8; 00416 00417 while(i-- != 0) 00418 { 00419 nibble = (value >> (4 * i)) & 0x0F; 00420 hex_string[9-i] = (nibble > 9) ? ('A' + nibble - 10) : ('0' + nibble); 00421 } 00422 00423 return (const char*)hex_string; 00424 } 00425 00426
Generated on Wed Jul 13 2022 07:07:19 by
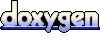