BLE_Nano nRF51 Central heart rate
Embed:
(wiki syntax)
Show/hide line numbers
nrf_gpio.h
00001 /* Copyright (c) 2015 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 * 00011 */ 00012 #ifndef NRF_GPIO_H__ 00013 #define NRF_GPIO_H__ 00014 00015 #include "nrf.h" 00016 #include <stdbool.h> 00017 00018 /** 00019 * @defgroup nrf_gpio GPIO abstraction 00020 * @{ 00021 * @ingroup nrf_drivers 00022 * @brief GPIO pin abstraction and port abstraction for reading and writing byte-wise to GPIO ports. 00023 * 00024 * Here, the GPIO ports are defined as follows: 00025 * - Port 0 -> pin 0-7 00026 * - Port 1 -> pin 8-15 00027 * - Port 2 -> pin 16-23 00028 * - Port 3 -> pin 24-31 00029 */ 00030 00031 #define NUMBER_OF_PINS 32 00032 00033 /** 00034 * @brief Enumerator used for selecting between port 0 - 3. 00035 */ 00036 typedef enum 00037 { 00038 NRF_GPIO_PORT_SELECT_PORT0 = 0, ///< Port 0 (GPIO pin 0-7) 00039 NRF_GPIO_PORT_SELECT_PORT1, ///< Port 1 (GPIO pin 8-15) 00040 NRF_GPIO_PORT_SELECT_PORT2, ///< Port 2 (GPIO pin 16-23) 00041 NRF_GPIO_PORT_SELECT_PORT3, ///< Port 3 (GPIO pin 24-31) 00042 } nrf_gpio_port_select_t; 00043 00044 /** 00045 * @brief Enumerator used for setting the direction of a GPIO port. 00046 */ 00047 typedef enum 00048 { 00049 NRF_GPIO_PORT_DIR_OUTPUT, ///< Output 00050 NRF_GPIO_PORT_DIR_INPUT ///< Input 00051 } nrf_gpio_port_dir_t; 00052 00053 /** 00054 * @brief Pin direction definitions. 00055 */ 00056 typedef enum 00057 { 00058 NRF_GPIO_PIN_DIR_INPUT = GPIO_PIN_CNF_DIR_Input, ///< Input 00059 NRF_GPIO_PIN_DIR_OUTPUT = GPIO_PIN_CNF_DIR_Output ///< Output 00060 } nrf_gpio_pin_dir_t; 00061 00062 /** 00063 * @brief Connection of input buffer 00064 */ 00065 typedef enum 00066 { 00067 NRF_GPIO_PIN_INPUT_CONNECT = GPIO_PIN_CNF_INPUT_Connect, ///< Connect input buffer 00068 NRF_GPIO_PIN_INPUT_DISCONNECT = GPIO_PIN_CNF_INPUT_Disconnect ///< Disconnect input buffer 00069 } nrf_gpio_pin_input_t; 00070 00071 /** 00072 * @brief Enumerator used for selecting the pin to be pulled down or up at the time of pin configuration 00073 */ 00074 typedef enum 00075 { 00076 NRF_GPIO_PIN_NOPULL = GPIO_PIN_CNF_PULL_Disabled, ///< Pin pullup resistor disabled 00077 NRF_GPIO_PIN_PULLDOWN = GPIO_PIN_CNF_PULL_Pulldown, ///< Pin pulldown resistor enabled 00078 NRF_GPIO_PIN_PULLUP = GPIO_PIN_CNF_PULL_Pullup, ///< Pin pullup resistor enabled 00079 } nrf_gpio_pin_pull_t; 00080 00081 /** 00082 * @brief Enumerator used for selecting output drive mode 00083 */ 00084 typedef enum 00085 { 00086 NRF_GPIO_PIN_S0S1 = GPIO_PIN_CNF_DRIVE_S0S1, ///< !< Standard '0', standard '1' 00087 NRF_GPIO_PIN_H0S1 = GPIO_PIN_CNF_DRIVE_H0S1, ///< !< High drive '0', standard '1' 00088 NRF_GPIO_PIN_S0H1 = GPIO_PIN_CNF_DRIVE_S0H1, ///< !< Standard '0', high drive '1' 00089 NRF_GPIO_PIN_H0H1 = GPIO_PIN_CNF_DRIVE_H0H1, ///< !< High drive '0', high 'drive '1'' 00090 NRF_GPIO_PIN_D0S1 = GPIO_PIN_CNF_DRIVE_D0S1, ///< !< Disconnect '0' standard '1' 00091 NRF_GPIO_PIN_D0H1 = GPIO_PIN_CNF_DRIVE_D0H1, ///< !< Disconnect '0', high drive '1' 00092 NRF_GPIO_PIN_S0D1 = GPIO_PIN_CNF_DRIVE_S0D1, ///< !< Standard '0'. disconnect '1' 00093 NRF_GPIO_PIN_H0D1 = GPIO_PIN_CNF_DRIVE_H0D1, ///< !< High drive '0', disconnect '1' 00094 } nrf_gpio_pin_drive_t; 00095 00096 /** 00097 * @brief Enumerator used for selecting the pin to sense high or low level on the pin input. 00098 */ 00099 typedef enum 00100 { 00101 NRF_GPIO_PIN_NOSENSE = GPIO_PIN_CNF_SENSE_Disabled, ///< Pin sense level disabled. 00102 NRF_GPIO_PIN_SENSE_LOW = GPIO_PIN_CNF_SENSE_Low, ///< Pin sense low level. 00103 NRF_GPIO_PIN_SENSE_HIGH = GPIO_PIN_CNF_SENSE_High, ///< Pin sense high level. 00104 } nrf_gpio_pin_sense_t; 00105 00106 00107 /** 00108 * @brief Function for configuring the GPIO pin range as outputs with normal drive strength. 00109 * This function can be used to configure pin range as simple output with gate driving GPIO_PIN_CNF_DRIVE_S0S1 (normal cases). 00110 * 00111 * @param pin_range_start specifies the start number (inclusive) in the range of pin numbers to be configured (allowed values 0-30) 00112 * 00113 * @param pin_range_end specifies the end number (inclusive) in the range of pin numbers to be configured (allowed values 0-30) 00114 * 00115 * @note For configuring only one pin as output use @ref nrf_gpio_cfg_output 00116 * Sense capability on the pin is disabled, and input is disconnected from the buffer as the pins are configured as output. 00117 */ 00118 __STATIC_INLINE void nrf_gpio_range_cfg_output(uint32_t pin_range_start, uint32_t pin_range_end); 00119 00120 /** 00121 * @brief Function for configuring the GPIO pin range as inputs with given initial value set, hiding inner details. 00122 * This function can be used to configure pin range as simple input. 00123 * 00124 * @param pin_range_start specifies the start number (inclusive) in the range of pin numbers to be configured (allowed values 0-30) 00125 * 00126 * @param pin_range_end specifies the end number (inclusive) in the range of pin numbers to be configured (allowed values 0-30) 00127 * 00128 * @param pull_config State of the pin range pull resistor (no pull, pulled down or pulled high) 00129 * 00130 * @note For configuring only one pin as input use @ref nrf_gpio_cfg_input 00131 * Sense capability on the pin is disabled, and input is connected to buffer so that the GPIO->IN register is readable 00132 */ 00133 __STATIC_INLINE void nrf_gpio_range_cfg_input(uint32_t pin_range_start, uint32_t pin_range_end, nrf_gpio_pin_pull_t pull_config); 00134 00135 /** 00136 * @brief Pin configuration function 00137 * 00138 * The main pin configuration function. 00139 * This function allows to set any aspect in PIN_CNF register. 00140 * @param pin_number Specifies the pin number (allowed values 0-31). 00141 * @param dir Pin direction 00142 * @param input Connect or disconnect input buffer 00143 * @param pull Pull configuration 00144 * @param drive Drive configuration 00145 * @param sense Pin sensing mechanism 00146 */ 00147 __STATIC_INLINE void nrf_gpio_cfg( 00148 uint32_t pin_number, 00149 nrf_gpio_pin_dir_t dir, 00150 nrf_gpio_pin_input_t input, 00151 nrf_gpio_pin_pull_t pull, 00152 nrf_gpio_pin_drive_t drive, 00153 nrf_gpio_pin_sense_t sense); 00154 00155 /** 00156 * @brief Function for configuring the given GPIO pin number as output with given initial value set, hiding inner details. 00157 * This function can be used to configure pin range as simple input with gate driving GPIO_PIN_CNF_DRIVE_S0S1 (normal cases). 00158 * 00159 * @param pin_number specifies the pin number (allowed values 0-31) 00160 * 00161 * @note Sense capability on the pin is disabled, and input is disconnected from the buffer as the pins are configured as output. 00162 */ 00163 __STATIC_INLINE void nrf_gpio_cfg_output(uint32_t pin_number); 00164 00165 /** 00166 * @brief Function for configuring the given GPIO pin number as input with given initial value set, hiding inner details. 00167 * This function can be used to configure pin range as simple input with gate driving GPIO_PIN_CNF_DRIVE_S0S1 (normal cases). 00168 * 00169 * @param pin_number Specifies the pin number (allowed values 0-30). 00170 * @param pull_config State of the pin range pull resistor (no pull, pulled down or pulled high). 00171 * 00172 * @note Sense capability on the pin is disabled, and input is connected to buffer so that the GPIO->IN register is readable 00173 */ 00174 __STATIC_INLINE void nrf_gpio_cfg_input(uint32_t pin_number, nrf_gpio_pin_pull_t pull_config); 00175 00176 /** 00177 * @brief Function for reseting pin configuration to its default state. 00178 * 00179 * @param pin_number Specifies the pin number (allowed values 0-31). 00180 */ 00181 __STATIC_INLINE void nrf_gpio_cfg_default(uint32_t pin_number); 00182 00183 /** 00184 * @brief Function for configuring the given GPIO pin number as a watcher. Only input is connected. 00185 * 00186 * @param pin_number Specifies the pin number (allowed values 0-31). 00187 * 00188 */ 00189 __STATIC_INLINE void nrf_gpio_cfg_watcher(uint32_t pin_number); 00190 00191 /** 00192 * @brief Function for disconnecting input for the given GPIO. 00193 * 00194 * @param pin_number Specifies the pin number (allowed values 0-31). 00195 * 00196 */ 00197 __STATIC_INLINE void nrf_gpio_input_disconnect(uint32_t pin_number); 00198 00199 /** 00200 * @brief Function for configuring the given GPIO pin number as input with given initial value set, hiding inner details. 00201 * This function can be used to configure pin range as simple input with gate driving GPIO_PIN_CNF_DRIVE_S0S1 (normal cases). 00202 * Sense capability on the pin is configurable, and input is connected to buffer so that the GPIO->IN register is readable. 00203 * 00204 * @param pin_number Specifies the pin number (allowed values 0-30). 00205 * @param pull_config State of the pin pull resistor (no pull, pulled down or pulled high). 00206 * @param sense_config Sense level of the pin (no sense, sense low or sense high). 00207 */ 00208 __STATIC_INLINE void nrf_gpio_cfg_sense_input(uint32_t pin_number, nrf_gpio_pin_pull_t pull_config, nrf_gpio_pin_sense_t sense_config); 00209 00210 /** 00211 * @brief Function for configuring sense level for the given GPIO. 00212 * 00213 * @param pin_number Specifies the pin number of gpio pin numbers to be configured (allowed values 0-30). 00214 * @param sense_config Sense configuration. 00215 * 00216 */ 00217 __STATIC_INLINE void nrf_gpio_cfg_sense_set(uint32_t pin_number, nrf_gpio_pin_sense_t sense_config); 00218 00219 /** 00220 * @brief Function for setting the direction for a GPIO pin. 00221 * 00222 * @param pin_number specifies the pin number (0-31) for which to 00223 * set the direction. 00224 * 00225 * @param direction specifies the direction 00226 */ 00227 __STATIC_INLINE void nrf_gpio_pin_dir_set(uint32_t pin_number, nrf_gpio_pin_dir_t direction); 00228 00229 /** 00230 * @brief Function for setting a GPIO pin. 00231 * 00232 * Note that the pin must be configured as an output for this 00233 * function to have any effect. 00234 * 00235 * @param pin_number Specifies the pin number (0-31) to set. 00236 */ 00237 __STATIC_INLINE void nrf_gpio_pin_set(uint32_t pin_number); 00238 00239 /** 00240 * @brief Function for setting GPIO pins. 00241 * 00242 * Note that the pins must be configured as outputs for this 00243 * function to have any effect. 00244 * 00245 * @param pin_mask Specifies the pins to set. 00246 */ 00247 __STATIC_INLINE void nrf_gpio_pins_set(uint32_t pin_mask); 00248 00249 /** 00250 * @brief Function for clearing a GPIO pin. 00251 * 00252 * Note that the pin must be configured as an output for this 00253 * function to have any effect. 00254 * 00255 * @param pin_number Specifies the pin number (0-31) to clear. 00256 */ 00257 __STATIC_INLINE void nrf_gpio_pin_clear(uint32_t pin_number); 00258 00259 /** 00260 * @brief Function for clearing GPIO pins. 00261 * 00262 * Note that the pins must be configured as outputs for this 00263 * function to have any effect. 00264 * 00265 * @param pin_mask Specifies the pins to clear. 00266 */ 00267 __STATIC_INLINE void nrf_gpio_pins_clear(uint32_t pin_mask); 00268 00269 /** 00270 * @brief Function for toggling a GPIO pin. 00271 * 00272 * Note that the pin must be configured as an output for this 00273 * function to have any effect. 00274 * 00275 * @param pin_number Specifies the pin number (0-31) to toggle. 00276 */ 00277 __STATIC_INLINE void nrf_gpio_pin_toggle(uint32_t pin_number); 00278 00279 /** 00280 * @brief Function for toggling GPIO pins. 00281 * 00282 * Note that the pins must be configured as outputs for this 00283 * function to have any effect. 00284 * 00285 * @param pin_mask Specifies the pins to toggle. 00286 */ 00287 __STATIC_INLINE void nrf_gpio_pins_toggle(uint32_t pin_mask); 00288 00289 /** 00290 * @brief Function for writing a value to a GPIO pin. 00291 * 00292 * Note that the pin must be configured as an output for this 00293 * function to have any effect. 00294 * 00295 * @param pin_number specifies the pin number (0-31) to 00296 * write. 00297 * 00298 * @param value specifies the value to be written to the pin. 00299 * @arg 0 clears the pin 00300 * @arg >=1 sets the pin. 00301 */ 00302 __STATIC_INLINE void nrf_gpio_pin_write(uint32_t pin_number, uint32_t value); 00303 00304 /** 00305 * @brief Function for reading the input level of a GPIO pin. 00306 * 00307 * Note that the pin must have input connected for the value 00308 * returned from this function to be valid. 00309 * 00310 * @param pin_number specifies the pin number (0-31) to 00311 * read. 00312 * 00313 * @return 00314 * @retval 0 if the pin input level is low. 00315 * @retval 1 if the pin input level is high. 00316 * @retval > 1 should never occur. 00317 */ 00318 __STATIC_INLINE uint32_t nrf_gpio_pin_read(uint32_t pin_number); 00319 00320 /** 00321 * @brief Function for reading the input level of all GPIO pins. 00322 * 00323 * Note that the pin must have input connected for the value 00324 * returned from this function to be valid. 00325 * 00326 * @retval Status of input of all pins 00327 */ 00328 __STATIC_INLINE uint32_t nrf_gpio_pins_read(void); 00329 00330 /** 00331 * @brief Function for reading the sense configuration of a GPIO pin. 00332 * 00333 * @param pin_number specifies the pin number (0-31) to 00334 * read. 00335 * 00336 * @retval Sense configuration 00337 */ 00338 __STATIC_INLINE nrf_gpio_pin_sense_t nrf_gpio_pin_sense_get(uint32_t pin_number); 00339 00340 /** 00341 * @brief Generic function for writing a single byte of a 32 bit word at a given 00342 * address. 00343 * 00344 * This function should not be called from outside the nrf_gpio 00345 * abstraction layer. 00346 * 00347 * @param word_address is the address of the word to be written. 00348 * 00349 * @param byte_no is the word byte number (0-3) to be written. 00350 * 00351 * @param value is the value to be written to byte "byte_no" of word 00352 * at address "word_address" 00353 */ 00354 __STATIC_INLINE void nrf_gpio_word_byte_write(volatile uint32_t * word_address, uint8_t byte_no, uint8_t value); 00355 00356 /** 00357 * @brief Generic function for reading a single byte of a 32 bit word at a given 00358 * address. 00359 * 00360 * This function should not be called from outside the nrf_gpio 00361 * abstraction layer. 00362 * 00363 * @param word_address is the address of the word to be read. 00364 * 00365 * @param byte_no is the byte number (0-3) of the word to be read. 00366 * 00367 * @return byte "byte_no" of word at address "word_address". 00368 */ 00369 __STATIC_INLINE uint8_t nrf_gpio_word_byte_read(const volatile uint32_t* word_address, uint8_t byte_no); 00370 00371 /** 00372 * @brief Function for setting the direction of a port. 00373 * 00374 * @param port is the port for which to set the direction. 00375 * 00376 * @param dir direction to be set for this port. 00377 */ 00378 __STATIC_INLINE void nrf_gpio_port_dir_set(nrf_gpio_port_select_t port, nrf_gpio_port_dir_t dir); 00379 00380 /** 00381 * @brief Function for reading a GPIO port. 00382 * 00383 * @param port is the port to read. 00384 * 00385 * @return the input value on this port. 00386 */ 00387 __STATIC_INLINE uint8_t nrf_gpio_port_read(nrf_gpio_port_select_t port); 00388 00389 /** 00390 * @brief Function for writing to a GPIO port. 00391 * 00392 * @param port is the port to write. 00393 * 00394 * @param value is the value to write to this port. 00395 * 00396 * @sa nrf_gpio_port_dir_set() 00397 */ 00398 __STATIC_INLINE void nrf_gpio_port_write(nrf_gpio_port_select_t port, uint8_t value); 00399 00400 /** 00401 * @brief Function for setting individual pins on GPIO port. 00402 * 00403 * @param port is the port for which to set the pins. 00404 * 00405 * @param set_mask is a mask specifying which pins to set. A bit 00406 * set to 1 indicates that the corresponding port pin shall be 00407 * set. 00408 * 00409 * @sa nrf_gpio_port_dir_set() 00410 */ 00411 __STATIC_INLINE void nrf_gpio_port_set(nrf_gpio_port_select_t port, uint8_t set_mask); 00412 00413 /** 00414 * @brief Function for clearing individual pins on GPIO port. 00415 * 00416 * @param port is the port for which to clear the pins. 00417 * 00418 * @param clr_mask is a mask specifying which pins to clear. A bit 00419 * set to 1 indicates that the corresponding port pin shall be 00420 * cleared. 00421 * 00422 * @sa nrf_gpio_port_dir_set() 00423 */ 00424 __STATIC_INLINE void nrf_gpio_port_clear(nrf_gpio_port_select_t port, uint8_t clr_mask); 00425 00426 #ifndef SUPPRESS_INLINE_IMPLEMENTATION 00427 __STATIC_INLINE void nrf_gpio_range_cfg_output(uint32_t pin_range_start, uint32_t pin_range_end) 00428 { 00429 /*lint -e{845} // A zero has been given as right argument to operator '|'" */ 00430 for (; pin_range_start <= pin_range_end; pin_range_start++) 00431 { 00432 nrf_gpio_cfg_output(pin_range_start); 00433 } 00434 } 00435 00436 __STATIC_INLINE void nrf_gpio_range_cfg_input(uint32_t pin_range_start, uint32_t pin_range_end, nrf_gpio_pin_pull_t pull_config) 00437 { 00438 /*lint -e{845} // A zero has been given as right argument to operator '|'" */ 00439 for (; pin_range_start <= pin_range_end; pin_range_start++) 00440 { 00441 nrf_gpio_cfg_input(pin_range_start, pull_config); 00442 } 00443 } 00444 00445 __STATIC_INLINE void nrf_gpio_cfg( 00446 uint32_t pin_number, 00447 nrf_gpio_pin_dir_t dir, 00448 nrf_gpio_pin_input_t input, 00449 nrf_gpio_pin_pull_t pull, 00450 nrf_gpio_pin_drive_t drive, 00451 nrf_gpio_pin_sense_t sense) 00452 { 00453 NRF_GPIO->PIN_CNF[pin_number] = ((uint32_t)dir << GPIO_PIN_CNF_DIR_Pos) 00454 | ((uint32_t)input << GPIO_PIN_CNF_INPUT_Pos) 00455 | ((uint32_t)pull << GPIO_PIN_CNF_PULL_Pos) 00456 | ((uint32_t)drive << GPIO_PIN_CNF_DRIVE_Pos) 00457 | ((uint32_t)sense << GPIO_PIN_CNF_SENSE_Pos); 00458 } 00459 00460 __STATIC_INLINE void nrf_gpio_cfg_output(uint32_t pin_number) 00461 { 00462 nrf_gpio_cfg( 00463 pin_number, 00464 NRF_GPIO_PIN_DIR_OUTPUT, 00465 NRF_GPIO_PIN_INPUT_DISCONNECT, 00466 NRF_GPIO_PIN_NOPULL, 00467 NRF_GPIO_PIN_S0S1, 00468 NRF_GPIO_PIN_NOSENSE); 00469 } 00470 00471 __STATIC_INLINE void nrf_gpio_cfg_input(uint32_t pin_number, nrf_gpio_pin_pull_t pull_config) 00472 { 00473 nrf_gpio_cfg( 00474 pin_number, 00475 NRF_GPIO_PIN_DIR_INPUT, 00476 NRF_GPIO_PIN_INPUT_CONNECT, 00477 pull_config, 00478 NRF_GPIO_PIN_S0S1, 00479 NRF_GPIO_PIN_NOSENSE); 00480 } 00481 00482 __STATIC_INLINE void nrf_gpio_cfg_default(uint32_t pin_number) 00483 { 00484 nrf_gpio_cfg( 00485 pin_number, 00486 NRF_GPIO_PIN_DIR_INPUT, 00487 NRF_GPIO_PIN_INPUT_DISCONNECT, 00488 NRF_GPIO_PIN_NOPULL, 00489 NRF_GPIO_PIN_S0S1, 00490 NRF_GPIO_PIN_NOSENSE); 00491 } 00492 00493 __STATIC_INLINE void nrf_gpio_cfg_watcher(uint32_t pin_number) 00494 { 00495 /*lint -e{845} // A zero has been given as right argument to operator '|'" */ 00496 uint32_t cnf = NRF_GPIO->PIN_CNF[pin_number] & ~GPIO_PIN_CNF_INPUT_Msk; 00497 NRF_GPIO->PIN_CNF[pin_number] = cnf | (GPIO_PIN_CNF_INPUT_Connect << GPIO_PIN_CNF_INPUT_Pos); 00498 } 00499 00500 __STATIC_INLINE void nrf_gpio_input_disconnect(uint32_t pin_number) 00501 { 00502 /*lint -e{845} // A zero has been given as right argument to operator '|'" */ 00503 uint32_t cnf = NRF_GPIO->PIN_CNF[pin_number] & ~GPIO_PIN_CNF_INPUT_Msk; 00504 NRF_GPIO->PIN_CNF[pin_number] = cnf | (GPIO_PIN_CNF_INPUT_Disconnect << GPIO_PIN_CNF_INPUT_Pos); 00505 } 00506 00507 __STATIC_INLINE void nrf_gpio_cfg_sense_input(uint32_t pin_number, nrf_gpio_pin_pull_t pull_config, nrf_gpio_pin_sense_t sense_config) 00508 { 00509 nrf_gpio_cfg( 00510 pin_number, 00511 NRF_GPIO_PIN_DIR_INPUT, 00512 NRF_GPIO_PIN_INPUT_CONNECT, 00513 pull_config, 00514 NRF_GPIO_PIN_S0S1, 00515 sense_config); 00516 } 00517 00518 __STATIC_INLINE void nrf_gpio_cfg_sense_set(uint32_t pin_number, nrf_gpio_pin_sense_t sense_config) 00519 { 00520 /*lint -e{845} // A zero has been given as right argument to operator '|'" */ 00521 //uint32_t cnf = NRF_GPIO->PIN_CNF[pin_number] & ~GPIO_PIN_CNF_SENSE_Msk; 00522 NRF_GPIO->PIN_CNF[pin_number] &= ~GPIO_PIN_CNF_SENSE_Msk; 00523 NRF_GPIO->PIN_CNF[pin_number] |= (sense_config << GPIO_PIN_CNF_SENSE_Pos); 00524 } 00525 00526 __STATIC_INLINE void nrf_gpio_pin_dir_set(uint32_t pin_number, nrf_gpio_pin_dir_t direction) 00527 { 00528 if(direction == NRF_GPIO_PIN_DIR_INPUT) 00529 { 00530 nrf_gpio_cfg( 00531 pin_number, 00532 NRF_GPIO_PIN_DIR_INPUT, 00533 NRF_GPIO_PIN_INPUT_CONNECT, 00534 NRF_GPIO_PIN_NOPULL, 00535 NRF_GPIO_PIN_S0S1, 00536 NRF_GPIO_PIN_NOSENSE); 00537 } 00538 else 00539 { 00540 NRF_GPIO->DIRSET = (1UL << pin_number); 00541 } 00542 } 00543 00544 __STATIC_INLINE void nrf_gpio_pin_set(uint32_t pin_number) 00545 { 00546 NRF_GPIO->OUTSET = (1UL << pin_number); 00547 } 00548 00549 __STATIC_INLINE void nrf_gpio_pins_set(uint32_t pin_mask) 00550 { 00551 NRF_GPIO->OUTSET = pin_mask; 00552 } 00553 00554 __STATIC_INLINE void nrf_gpio_pin_clear(uint32_t pin_number) 00555 { 00556 NRF_GPIO->OUTCLR = (1UL << pin_number); 00557 } 00558 00559 __STATIC_INLINE void nrf_gpio_pins_clear(uint32_t pin_mask) 00560 { 00561 NRF_GPIO->OUTCLR = pin_mask; 00562 } 00563 00564 __STATIC_INLINE void nrf_gpio_pin_toggle(uint32_t pin_number) 00565 { 00566 nrf_gpio_pins_toggle(1UL << pin_number); 00567 } 00568 00569 __STATIC_INLINE void nrf_gpio_pins_toggle(uint32_t pin_mask) 00570 { 00571 uint32_t pins_state = NRF_GPIO->OUT; 00572 NRF_GPIO->OUTSET = (~pins_state & pin_mask); 00573 NRF_GPIO->OUTCLR = ( pins_state & pin_mask); 00574 } 00575 00576 __STATIC_INLINE void nrf_gpio_pin_write(uint32_t pin_number, uint32_t value) 00577 { 00578 if (value == 0) 00579 { 00580 nrf_gpio_pin_clear(pin_number); 00581 } 00582 else 00583 { 00584 nrf_gpio_pin_set(pin_number); 00585 } 00586 } 00587 00588 __STATIC_INLINE uint32_t nrf_gpio_pin_read(uint32_t pin_number) 00589 { 00590 return ((NRF_GPIO->IN >> pin_number) & 1UL); 00591 } 00592 00593 __STATIC_INLINE uint32_t nrf_gpio_pins_read(void) 00594 { 00595 return NRF_GPIO->IN; 00596 } 00597 00598 __STATIC_INLINE nrf_gpio_pin_sense_t nrf_gpio_pin_sense_get(uint32_t pin_number) 00599 { 00600 return (nrf_gpio_pin_sense_t)((NRF_GPIO->PIN_CNF[pin_number] & GPIO_PIN_CNF_SENSE_Msk) >> GPIO_PIN_CNF_SENSE_Pos); 00601 } 00602 00603 __STATIC_INLINE void nrf_gpio_word_byte_write(volatile uint32_t * word_address, uint8_t byte_no, uint8_t value) 00604 { 00605 *((volatile uint8_t*)(word_address) + byte_no) = value; 00606 } 00607 00608 __STATIC_INLINE uint8_t nrf_gpio_word_byte_read(const volatile uint32_t* word_address, uint8_t byte_no) 00609 { 00610 return (*((const volatile uint8_t*)(word_address) + byte_no)); 00611 } 00612 00613 __STATIC_INLINE void nrf_gpio_port_dir_set(nrf_gpio_port_select_t port, nrf_gpio_port_dir_t dir) 00614 { 00615 if (dir == NRF_GPIO_PORT_DIR_OUTPUT) 00616 { 00617 nrf_gpio_word_byte_write(&NRF_GPIO->DIRSET, port, 0xFF); 00618 } 00619 else 00620 { 00621 nrf_gpio_range_cfg_input(port*8, (port+1)*8-1, NRF_GPIO_PIN_NOPULL); 00622 } 00623 } 00624 00625 __STATIC_INLINE uint8_t nrf_gpio_port_read(nrf_gpio_port_select_t port) 00626 { 00627 return nrf_gpio_word_byte_read(&NRF_GPIO->IN, port); 00628 } 00629 00630 __STATIC_INLINE void nrf_gpio_port_write(nrf_gpio_port_select_t port, uint8_t value) 00631 { 00632 nrf_gpio_word_byte_write(&NRF_GPIO->OUT, port, value); 00633 } 00634 00635 __STATIC_INLINE void nrf_gpio_port_set(nrf_gpio_port_select_t port, uint8_t set_mask) 00636 { 00637 nrf_gpio_word_byte_write(&NRF_GPIO->OUTSET, port, set_mask); 00638 } 00639 00640 __STATIC_INLINE void nrf_gpio_port_clear(nrf_gpio_port_select_t port, uint8_t clr_mask) 00641 { 00642 nrf_gpio_word_byte_write(&NRF_GPIO->OUTCLR, port, clr_mask); 00643 } 00644 #endif //SUPPRESS_INLINE_IMPLEMENTATION 00645 /** @} */ 00646 00647 #endif 00648
Generated on Wed Jul 13 2022 07:07:19 by
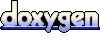