BLE_Nano nRF51 Central heart rate
Embed:
(wiki syntax)
Show/hide line numbers
ble_l2cap.h
00001 /* 00002 * Copyright (c) Nordic Semiconductor ASA 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, this 00009 * list of conditions and the following disclaimer. 00010 * 00011 * 2. Redistributions in binary form must reproduce the above copyright notice, this 00012 * list of conditions and the following disclaimer in the documentation and/or 00013 * other materials provided with the distribution. 00014 * 00015 * 3. Neither the name of Nordic Semiconductor ASA nor the names of other 00016 * contributors to this software may be used to endorse or promote products 00017 * derived from this software without specific prior written permission. 00018 * 00019 * 4. This software must only be used in a processor manufactured by Nordic 00020 * Semiconductor ASA, or in a processor manufactured by a third party that 00021 * is used in combination with a processor manufactured by Nordic Semiconductor. 00022 * 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00025 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00026 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00028 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00029 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00030 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00031 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 /** 00038 @addtogroup BLE_L2CAP Logical Link Control and Adaptation Protocol (L2CAP) 00039 @{ 00040 @brief Definitions and prototypes for the L2CAP interface. 00041 */ 00042 00043 #ifndef BLE_L2CAP_H__ 00044 #define BLE_L2CAP_H__ 00045 00046 #include "ble_types.h" 00047 #include "ble_ranges.h" 00048 #include "ble_err.h" 00049 #include "nrf_svc.h" 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 /**@addtogroup BLE_L2CAP_ENUMERATIONS Enumerations 00056 * @{ */ 00057 00058 /**@brief L2CAP API SVC numbers. */ 00059 enum BLE_L2CAP_SVCS 00060 { 00061 SD_BLE_L2CAP_CID_REGISTER = BLE_L2CAP_SVC_BASE, /**< Register a CID. */ 00062 SD_BLE_L2CAP_CID_UNREGISTER, /**< Unregister a CID. */ 00063 SD_BLE_L2CAP_TX /**< Transmit a packet. */ 00064 }; 00065 00066 /**@brief L2CAP Event IDs. */ 00067 enum BLE_L2CAP_EVTS 00068 { 00069 BLE_L2CAP_EVT_RX = BLE_L2CAP_EVT_BASE /**< L2CAP packet received. */ 00070 }; 00071 00072 /** @} */ 00073 00074 /**@addtogroup BLE_L2CAP_DEFINES Defines 00075 * @{ */ 00076 00077 /**@defgroup BLE_ERRORS_L2CAP SVC return values specific to L2CAP 00078 * @{ */ 00079 #define BLE_ERROR_L2CAP_CID_IN_USE (NRF_L2CAP_ERR_BASE + 0x000) /**< CID already in use. */ 00080 /** @} */ 00081 00082 /**@brief Default L2CAP MTU. */ 00083 #define BLE_L2CAP_MTU_DEF (23) 00084 00085 /**@brief Invalid Channel Identifier. */ 00086 #define BLE_L2CAP_CID_INVALID (0x0000) 00087 00088 /**@brief Dynamic Channel Identifier base. */ 00089 #define BLE_L2CAP_CID_DYN_BASE (0x0040) 00090 00091 /**@brief Maximum amount of dynamic CIDs. */ 00092 #define BLE_L2CAP_CID_DYN_MAX (8) 00093 00094 /** @} */ 00095 00096 /**@addtogroup BLE_L2CAP_STRUCTURES Structures 00097 * @{ */ 00098 00099 /**@brief Packet header format for L2CAP transmission. */ 00100 typedef struct 00101 { 00102 uint16_t len; /**< Length of valid info in data member. */ 00103 uint16_t cid; /**< Channel ID on which packet is transmitted. */ 00104 } ble_l2cap_header_t; 00105 00106 00107 /**@brief L2CAP Received packet event report. */ 00108 typedef struct 00109 { 00110 ble_l2cap_header_t header; /**< L2CAP packet header. */ 00111 uint8_t data[1]; /**< Packet data. @note This is a variable length array. The size of 1 indicated is only a placeholder for compilation. 00112 See @ref sd_ble_evt_get for more information on how to use event structures with variable length array members. */ 00113 } ble_l2cap_evt_rx_t; 00114 00115 00116 /**@brief L2CAP event callback event structure. */ 00117 typedef struct 00118 { 00119 uint16_t conn_handle; /**< Connection Handle on which event occured. */ 00120 union 00121 { 00122 ble_l2cap_evt_rx_t rx; /**< RX Event parameters. */ 00123 } params; /**< Event Parameters. */ 00124 } ble_l2cap_evt_t; 00125 00126 /** @} */ 00127 00128 /**@addtogroup BLE_L2CAP_FUNCTIONS Functions 00129 * @{ */ 00130 00131 /**@brief Register a CID with L2CAP. 00132 * 00133 * @details This registers a higher protocol layer with the L2CAP multiplexer, and is requried prior to all operations on the CID. 00134 * 00135 * @mscs 00136 * @mmsc{@ref BLE_L2CAP_API_MSC} 00137 * @endmscs 00138 * 00139 * @param[in] cid L2CAP CID. 00140 * 00141 * @retval ::NRF_SUCCESS Successfully registered a CID with the L2CAP layer. 00142 * @retval ::NRF_ERROR_INVALID_PARAM Invalid parameter(s) supplied, CID must be above @ref BLE_L2CAP_CID_DYN_BASE. 00143 * @retval ::BLE_ERROR_L2CAP_CID_IN_USE L2CAP CID already in use. 00144 * @retval ::NRF_ERROR_NO_MEM Not enough memory to complete operation. 00145 */ 00146 SVCALL(SD_BLE_L2CAP_CID_REGISTER, uint32_t, sd_ble_l2cap_cid_register(uint16_t cid)); 00147 00148 /**@brief Unregister a CID with L2CAP. 00149 * 00150 * @details This unregisters a previously registerd higher protocol layer with the L2CAP multiplexer. 00151 * 00152 * @mscs 00153 * @mmsc{@ref BLE_L2CAP_API_MSC} 00154 * @endmscs 00155 * 00156 * @param[in] cid L2CAP CID. 00157 * 00158 * @retval ::NRF_SUCCESS Successfully unregistered the CID. 00159 * @retval ::NRF_ERROR_INVALID_PARAM Invalid parameter(s) supplied. 00160 * @retval ::NRF_ERROR_NOT_FOUND CID not previously registered. 00161 */ 00162 SVCALL(SD_BLE_L2CAP_CID_UNREGISTER, uint32_t, sd_ble_l2cap_cid_unregister(uint16_t cid)); 00163 00164 /**@brief Transmit an L2CAP packet. 00165 * 00166 * @note It is important to note that a call to this function will <b>consume an application packet</b>, and will therefore 00167 * generate a @ref BLE_EVT_TX_COMPLETE event when the packet has been transmitted. 00168 * Please see the documentation of @ref sd_ble_tx_packet_count_get for more details. 00169 * 00170 * @events 00171 * @event{@ref BLE_EVT_TX_COMPLETE} 00172 * @event{@ref BLE_L2CAP_EVT_RX} 00173 * @endevents 00174 * 00175 * @mscs 00176 * @mmsc{@ref BLE_L2CAP_API_MSC} 00177 * @endmscs 00178 * 00179 * @param[in] conn_handle Connection Handle. 00180 * @param[in] p_header Pointer to a packet header containing length and CID. 00181 * @param[in] p_data Pointer to the data to be transmitted. 00182 * 00183 * @retval ::NRF_SUCCESS Successfully queued an L2CAP packet for transmission. 00184 * @retval ::NRF_ERROR_INVALID_ADDR Invalid pointer supplied. 00185 * @retval ::NRF_ERROR_INVALID_PARAM Invalid parameter(s) supplied, CIDs must be registered beforehand with @ref sd_ble_l2cap_cid_register. 00186 * @retval ::NRF_ERROR_NOT_FOUND CID not found. 00187 * @retval ::NRF_ERROR_NO_MEM Not enough memory to complete operation. 00188 * @retval ::BLE_ERROR_NO_TX_PACKETS Not enough application packets available. 00189 * @retval ::NRF_ERROR_DATA_SIZE Invalid data size(s) supplied, see @ref BLE_L2CAP_MTU_DEF. 00190 */ 00191 SVCALL(SD_BLE_L2CAP_TX, uint32_t, sd_ble_l2cap_tx(uint16_t conn_handle, ble_l2cap_header_t const *p_header, uint8_t const *p_data)); 00192 00193 /** @} */ 00194 00195 #ifdef __cplusplus 00196 } 00197 #endif 00198 #endif // BLE_L2CAP_H__ 00199 00200 /** 00201 @} 00202 */ 00203
Generated on Wed Jul 13 2022 07:07:19 by
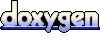