BLE_Nano nRF51 Central heart rate
Embed:
(wiki syntax)
Show/hide line numbers
ble_db_discovery.h
Go to the documentation of this file.
00001 /* Copyright (c) 2013 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 */ 00011 00012 00013 /**@file 00014 * 00015 * @defgroup ble_sdk_lib_db_discovery Database Discovery 00016 * @{ 00017 * @ingroup ble_sdk_lib 00018 * @brief Database discovery module. 00019 * 00020 * @details This module contains the APIs and types exposed by the DB Discovery module. These APIs 00021 * and types can be used by the application to perform discovery of a service and its 00022 * characteristics at the peer server. This module can also be used to discover the 00023 * desired services in multiple remote devices. 00024 * 00025 * @warning The maximum number of characteristics per service that can be discovered by this module 00026 * is determined by the number of characteristics in the service structure defined in 00027 * db_disc_config.h. If the peer has more than the supported number of characteristics, then 00028 * the first found will be discovered and any further characteristics will be ignored. No 00029 * descriptors other than Client Characteristic Configuration Descriptors will be searched 00030 * for at the peer. 00031 * 00032 * @note Presently only one instance of a Primary Service can be discovered by this module. If 00033 * there are multiple instances of the service at the peer, only the first instance 00034 * of it at the peer is fetched and returned to the application. 00035 * 00036 * @note The application must propagate BLE stack events to this module by calling 00037 * ble_db_discovery_on_ble_evt(). 00038 * 00039 */ 00040 00041 #ifndef BLE_DB_DISCOVERY_H__ 00042 #define BLE_DB_DISCOVERY_H__ 00043 00044 #include <stdint.h> 00045 #include "ble_gattc.h" 00046 #include "ble.h" 00047 #include "nrf_error.h" 00048 #include "ble_srv_common.h " 00049 #include "ble_gatt_db.h " 00050 00051 00052 #define BLE_DB_DISCOVERY_MAX_SRV 6 /**< Maximum number of services supported by this module. This also indicates the maximum number of users allowed to be registered to this module. (one user per service). */ 00053 00054 00055 /**@brief Type of the DB Discovery event. 00056 */ 00057 typedef enum 00058 { 00059 BLE_DB_DISCOVERY_COMPLETE, /**< Event indicating that the GATT Database discovery is complete. */ 00060 BLE_DB_DISCOVERY_ERROR, /**< Event indicating that an internal error has occurred in the DB Discovery module. This could typically be because of the SoftDevice API returning an error code during the DB discover.*/ 00061 BLE_DB_DISCOVERY_SRV_NOT_FOUND, /**< Event indicating that the service was not found at the peer.*/ 00062 BLE_DB_DISCOVERY_AVAILABLE /**< Event indicating that the DB discovery module is available.*/ 00063 } ble_db_discovery_evt_type_t; 00064 00065 00066 00067 /**@brief Structure for holding the information related to the GATT database at the server. 00068 * 00069 * @details This module identifies a remote database. Use one instance of this structure per 00070 * connection. 00071 * 00072 * @warning This structure must be zero-initialized. 00073 */ 00074 typedef struct 00075 { 00076 ble_gatt_db_srv_t services[BLE_DB_DISCOVERY_MAX_SRV]; /**< Information related to the current service being discovered. This is intended for internal use during service discovery.*/ 00077 uint8_t srv_count; /**< Number of services at the peers GATT database.*/ 00078 uint8_t curr_char_ind; /**< Index of the current characteristic being discovered. This is intended for internal use during service discovery.*/ 00079 uint8_t curr_srv_ind; /**< Index of the current service being discovered. This is intended for internal use during service discovery.*/ 00080 bool discovery_in_progress; /**< Variable to indicate if there is a service discovery in progress. */ 00081 uint8_t discoveries_count; /**< Number of service discoveries made, both successful and unsuccessful. */ 00082 uint16_t conn_handle; /**< Connection handle on which the discovery is started*/ 00083 } ble_db_discovery_t; 00084 00085 00086 /**@brief Structure containing the event from the DB discovery module to the application. 00087 */ 00088 typedef struct 00089 { 00090 ble_db_discovery_evt_type_t evt_type; /**< Type of event. */ 00091 uint16_t conn_handle; /**< Handle of the connection for which this event has occurred. */ 00092 union 00093 { 00094 ble_gatt_db_srv_t discovered_db; /**< Structure containing the information about the GATT Database at the server. This will be filled when the event type is @ref BLE_DB_DISCOVERY_COMPLETE.*/ 00095 uint32_t err_code; /**< nRF Error code indicating the type of error which occurred in the DB Discovery module. This will be filled when the event type is @ref BLE_DB_DISCOVERY_ERROR. */ 00096 } params; 00097 } ble_db_discovery_evt_t; 00098 00099 00100 /**@brief DB Discovery event handler type. */ 00101 typedef void (* ble_db_discovery_evt_handler_t)(ble_db_discovery_evt_t * p_evt); 00102 00103 00104 /**@brief Function for initializing the DB Discovery module. 00105 * 00106 * @param[in] evt_handler Event handler to be called by the DB discovery module when any event 00107 * related to discovery of the registered service occurs. 00108 * 00109 * @retval NRF_SUCCESS On successful initialization. 00110 * @retval NRF_ERROR_NULL If the handler was NULL. 00111 */ 00112 uint32_t ble_db_discovery_init(ble_db_discovery_evt_handler_t evt_handler); 00113 00114 00115 /**@brief Function for closing the DB Discovery module. 00116 * 00117 * @details This function will clear up any internal variables and states maintained by the 00118 * module. To re-use the module after calling this function, the function @ref 00119 * ble_db_discovery_init must be called again. 00120 * 00121 * @retval NRF_SUCCESS Operation success. 00122 */ 00123 uint32_t ble_db_discovery_close(void); 00124 00125 00126 /**@brief Function for registering with the DB Discovery module. 00127 * 00128 * @details The application can use this function to inform which service it is interested in 00129 * discovering at the server. 00130 * 00131 * @param[in] p_uuid Pointer to the UUID of the service to be discovered at the server. 00132 * 00133 * @note The total number of services that can be discovered by this module is @ref 00134 * BLE_DB_DISCOVERY_MAX_SRV. This effectively means that the maximum number of 00135 * registrations possible is equal to the @ref BLE_DB_DISCOVERY_MAX_SRV. 00136 * 00137 * @retval NRF_SUCCESS Operation success. 00138 * @retval NRF_ERROR_NULL When a NULL pointer is passed as input. 00139 * @retval NRF_ERROR_INVALID_STATE If this function is called without calling the 00140 * @ref ble_db_discovery_init. 00141 * @retval NRF_ERROR_NO_MEM The maximum number of registrations allowed by this module 00142 * has been reached. 00143 */ 00144 uint32_t ble_db_discovery_evt_register(const ble_uuid_t * const p_uuid); 00145 00146 00147 /**@brief Function for starting the discovery of the GATT database at the server. 00148 * 00149 * @warning p_db_discovery structure must be zero-initialized. 00150 * 00151 * @param[out] p_db_discovery Pointer to the DB Discovery structure. 00152 * @param[in] conn_handle The handle of the connection for which the discovery should be 00153 * started. 00154 * 00155 * @retval NRF_SUCCESS Operation success. 00156 * @retval NRF_ERROR_NULL When a NULL pointer is passed as input. 00157 * @retval NRF_ERROR_INVALID_STATE If this function is called without calling the 00158 * @ref ble_db_discovery_init, or without calling 00159 * @ref ble_db_discovery_evt_register. 00160 * @retval NRF_ERROR_BUSY If a discovery is already in progress for the current 00161 * connection. 00162 * 00163 * @return This API propagates the error code returned by the 00164 * SoftDevice API @ref sd_ble_gattc_primary_services_discover. 00165 */ 00166 uint32_t ble_db_discovery_start(ble_db_discovery_t * const p_db_discovery, 00167 uint16_t conn_handle); 00168 00169 00170 /**@brief Function for handling the Application's BLE Stack events. 00171 * 00172 * @param[in,out] p_db_discovery Pointer to the DB Discovery structure. 00173 * @param[in] p_ble_evt Pointer to the BLE event received. 00174 */ 00175 void ble_db_discovery_on_ble_evt(ble_db_discovery_t * const p_db_discovery, 00176 const ble_evt_t * const p_ble_evt); 00177 00178 #endif // BLE_DB_DISCOVERY_H__ 00179 00180 /** @} */ 00181
Generated on Wed Jul 13 2022 07:07:19 by
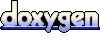