BLE_Nano nRF51 Central heart rate
Embed:
(wiki syntax)
Show/hide line numbers
app_util.h
Go to the documentation of this file.
00001 /* Copyright (c) 2012 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 * 00011 */ 00012 00013 /** @file 00014 * 00015 * @defgroup app_util Utility Functions and Definitions 00016 * @{ 00017 * @ingroup app_common 00018 * 00019 * @brief Various types and definitions available to all applications. 00020 */ 00021 00022 #ifndef APP_UTIL_H__ 00023 #define APP_UTIL_H__ 00024 00025 #include <stdint.h> 00026 #include <stdbool.h> 00027 #include "compiler_abstraction.h" 00028 #include "nrf.h" 00029 00030 //lint -save -e27 -e10 -e19 00031 #if defined ( __CC_ARM ) 00032 extern char STACK$$Base; 00033 extern char STACK$$Length; 00034 #define STACK_BASE &STACK$$Base 00035 #define STACK_TOP ((void*)((uint32_t)STACK_BASE + (uint32_t)&STACK$$Length)) 00036 #elif defined ( __ICCARM__ ) 00037 extern char CSTACK$$Base; 00038 extern char CSTACK$$Length; 00039 #define STACK_BASE &CSTACK$$Base 00040 #define STACK_TOP ((void*)((uint32_t)STACK_BASE + (uint32_t)&CSTACK$$Length)) 00041 #elif defined ( __GNUC__ ) 00042 extern uint32_t __StackTop; 00043 extern uint32_t __StackLimit; 00044 #define STACK_BASE &__StackLimit 00045 #define STACK_TOP &__StackTop 00046 #endif 00047 //lint -restore 00048 00049 enum 00050 { 00051 UNIT_0_625_MS = 625, /**< Number of microseconds in 0.625 milliseconds. */ 00052 UNIT_1_25_MS = 1250, /**< Number of microseconds in 1.25 milliseconds. */ 00053 UNIT_10_MS = 10000 /**< Number of microseconds in 10 milliseconds. */ 00054 }; 00055 00056 00057 /**@brief Implementation specific macro for delayed macro expansion used in string concatenation 00058 * 00059 * @param[in] lhs Left hand side in concatenation 00060 * @param[in] rhs Right hand side in concatenation 00061 */ 00062 #define STRING_CONCATENATE_IMPL(lhs, rhs) lhs ## rhs 00063 00064 00065 /**@brief Macro used to concatenate string using delayed macro expansion 00066 * 00067 * @note This macro will delay concatenation until the expressions have been resolved 00068 * 00069 * @param[in] lhs Left hand side in concatenation 00070 * @param[in] rhs Right hand side in concatenation 00071 */ 00072 #define STRING_CONCATENATE(lhs, rhs) STRING_CONCATENATE_IMPL(lhs, rhs) 00073 00074 00075 // Disable lint-warnings/errors for STATIC_ASSERT 00076 //lint --emacro(10,STATIC_ASSERT) 00077 //lint --emacro(18,STATIC_ASSERT) 00078 //lint --emacro(19,STATIC_ASSERT) 00079 //lint --emacro(30,STATIC_ASSERT) 00080 //lint --emacro(37,STATIC_ASSERT) 00081 //lint --emacro(42,STATIC_ASSERT) 00082 //lint --emacro(26,STATIC_ASSERT) 00083 //lint --emacro(102,STATIC_ASSERT) 00084 //lint --emacro(533,STATIC_ASSERT) 00085 //lint --emacro(534,STATIC_ASSERT) 00086 //lint --emacro(132,STATIC_ASSERT) 00087 //lint --emacro(414,STATIC_ASSERT) 00088 //lint --emacro(578,STATIC_ASSERT) 00089 //lint --emacro(628,STATIC_ASSERT) 00090 //lint --emacro(648,STATIC_ASSERT) 00091 //lint --emacro(830,STATIC_ASSERT) 00092 00093 00094 /**@brief Macro for doing static (i.e. compile time) assertion. 00095 * 00096 * @note If the EXPR isn't resolvable, then the error message won't be shown. 00097 * 00098 * @note The output of STATIC_ASSERT will be different across different compilers. 00099 * 00100 * @param[in] EXPR Constant expression to be verified. 00101 */ 00102 #if defined ( __COUNTER__ ) 00103 00104 #define STATIC_ASSERT(EXPR) \ 00105 ;enum { STRING_CONCATENATE(static_assert_, __COUNTER__) = 1/(!!(EXPR)) } 00106 00107 #else 00108 00109 #define STATIC_ASSERT(EXPR) \ 00110 ;enum { STRING_CONCATENATE(assert_line_, __LINE__) = 1/(!!(EXPR)) } 00111 00112 #endif 00113 00114 00115 /**@brief Implementation details for NUM_VAR_ARGS */ 00116 #define NUM_VA_ARGS_IMPL( \ 00117 _0, _1, _2, _3, _4, _5, _6, _7, _8, _9, _10, \ 00118 _11, _12, _13, _14, _15, _16, _17, _18, _19, _20, \ 00119 _21, _22, _23, _24, _25, _26, _27, _28, _29, _30, \ 00120 _31, _32, _33, _34, _35, _36, _37, _38, _39, _40, \ 00121 _41, _42, _43, _44, _45, _46, _47, _48, _49, _50, \ 00122 _51, _52, _53, _54, _55, _56, _57, _58, _59, _60, \ 00123 _61, _62, N, ...) N 00124 00125 00126 /**@brief Macro to get the number of arguments in a call variadic macro call 00127 * 00128 * param[in] ... List of arguments 00129 * 00130 * @retval Number of variadic arguments in the argument list 00131 */ 00132 #define NUM_VA_ARGS(...) NUM_VA_ARGS_IMPL(__VA_ARGS__, 63, 62, 61, \ 00133 60, 59, 58, 57, 56, 55, 54, 53, 52, 51, \ 00134 50, 49, 48, 47, 46, 45, 44, 43, 42, 41, \ 00135 40, 39, 38, 37, 36, 35, 34, 33, 32, 31, \ 00136 30, 29, 28, 27, 26, 25, 24, 23, 22, 21, \ 00137 20, 19, 18, 17, 16, 15, 14, 13, 12, 11, \ 00138 10, 9, 8, 7, 6, 5, 4, 3, 2, 1, 0) 00139 00140 00141 /**@brief type for holding an encoded (i.e. little endian) 16 bit unsigned integer. */ 00142 typedef uint8_t uint16_le_t[2]; 00143 00144 /**@brief Type for holding an encoded (i.e. little endian) 32 bit unsigned integer. */ 00145 typedef uint8_t uint32_le_t[4]; 00146 00147 /**@brief Byte array type. */ 00148 typedef struct 00149 { 00150 uint16_t size; /**< Number of array entries. */ 00151 uint8_t * p_data; /**< Pointer to array entries. */ 00152 } uint8_array_t; 00153 00154 00155 /**@brief Macro for performing rounded integer division (as opposed to truncating the result). 00156 * 00157 * @param[in] A Numerator. 00158 * @param[in] B Denominator. 00159 * 00160 * @return Rounded (integer) result of dividing A by B. 00161 */ 00162 #define ROUNDED_DIV(A, B) (((A) + ((B) / 2)) / (B)) 00163 00164 00165 /**@brief Macro for checking if an integer is a power of two. 00166 * 00167 * @param[in] A Number to be tested. 00168 * 00169 * @return true if value is power of two. 00170 * @return false if value not power of two. 00171 */ 00172 #define IS_POWER_OF_TWO(A) ( ((A) != 0) && ((((A) - 1) & (A)) == 0) ) 00173 00174 00175 /**@brief Macro for converting milliseconds to ticks. 00176 * 00177 * @param[in] TIME Number of milliseconds to convert. 00178 * @param[in] RESOLUTION Unit to be converted to in [us/ticks]. 00179 */ 00180 #define MSEC_TO_UNITS(TIME, RESOLUTION) (((TIME) * 1000) / (RESOLUTION)) 00181 00182 00183 /**@brief Macro for performing integer division, making sure the result is rounded up. 00184 * 00185 * @details One typical use for this is to compute the number of objects with size B is needed to 00186 * hold A number of bytes. 00187 * 00188 * @param[in] A Numerator. 00189 * @param[in] B Denominator. 00190 * 00191 * @return Integer result of dividing A by B, rounded up. 00192 */ 00193 #define CEIL_DIV(A, B) \ 00194 (((A) + (B) - 1) / (B)) 00195 00196 00197 /**@brief Macro for creating a buffer aligned to 4 bytes. 00198 * 00199 * @param[in] NAME Name of the buffor. 00200 * @param[in] MIN_SIZE Size of this buffor (it will be rounded up to multiples of 4 bytes). 00201 */ 00202 #define WORD_ALIGNED_MEM_BUFF(NAME, MIN_SIZE) static uint32_t NAME[CEIL_DIV(MIN_SIZE, sizeof(uint32_t))] 00203 00204 00205 /**@brief Macro for calculating the number of words that are needed to hold a number of bytes. 00206 * 00207 * @details Adds 3 and divides by 4. 00208 * 00209 * @param[in] n_bytes The number of bytes. 00210 * 00211 * @return The number of words that @p n_bytes take up (rounded up). 00212 */ 00213 #define BYTES_TO_WORDS(n_bytes) (((n_bytes) + 3) >> 2) 00214 00215 00216 /**@brief The number of bytes in a word. 00217 */ 00218 #define BYTES_PER_WORD (4) 00219 00220 00221 /**@brief Macro for increasing a number to the nearest (larger) multiple of another number. 00222 * 00223 * @param[in] alignment The number to align to. 00224 * @param[in] number The number to align (increase). 00225 * 00226 * @return The aligned (increased) @p number. 00227 */ 00228 #define ALIGN_NUM(alignment, number) ((number - 1) + alignment - ((number - 1) % alignment)) 00229 00230 00231 /**@brief Function for changing the value unit. 00232 * 00233 * @param[in] value Value to be rescaled. 00234 * @param[in] old_unit_reversal Reversal of the incoming unit. 00235 * @param[in] new_unit_reversal Reversal of the desired unit. 00236 * 00237 * @return Number of bytes written. 00238 */ 00239 static __INLINE uint64_t value_rescale(uint32_t value, uint32_t old_unit_reversal, uint16_t new_unit_reversal) 00240 { 00241 return (uint64_t)ROUNDED_DIV((uint64_t)value * new_unit_reversal, old_unit_reversal); 00242 } 00243 00244 /**@brief Function for encoding a uint16 value. 00245 * 00246 * @param[in] value Value to be encoded. 00247 * @param[out] p_encoded_data Buffer where the encoded data is to be written. 00248 * 00249 * @return Number of bytes written. 00250 */ 00251 static __INLINE uint8_t uint16_encode(uint16_t value, uint8_t * p_encoded_data) 00252 { 00253 p_encoded_data[0] = (uint8_t) ((value & 0x00FF) >> 0); 00254 p_encoded_data[1] = (uint8_t) ((value & 0xFF00) >> 8); 00255 return sizeof(uint16_t); 00256 } 00257 00258 /**@brief Function for encoding a three-byte value. 00259 * 00260 * @param[in] value Value to be encoded. 00261 * @param[out] p_encoded_data Buffer where the encoded data is to be written. 00262 * 00263 * @return Number of bytes written. 00264 */ 00265 static __INLINE uint8_t uint24_encode(uint32_t value, uint8_t * p_encoded_data) 00266 { 00267 p_encoded_data[0] = (uint8_t) ((value & 0x000000FF) >> 0); 00268 p_encoded_data[1] = (uint8_t) ((value & 0x0000FF00) >> 8); 00269 p_encoded_data[2] = (uint8_t) ((value & 0x00FF0000) >> 16); 00270 return 3; 00271 } 00272 00273 /**@brief Function for encoding a uint32 value. 00274 * 00275 * @param[in] value Value to be encoded. 00276 * @param[out] p_encoded_data Buffer where the encoded data is to be written. 00277 * 00278 * @return Number of bytes written. 00279 */ 00280 static __INLINE uint8_t uint32_encode(uint32_t value, uint8_t * p_encoded_data) 00281 { 00282 p_encoded_data[0] = (uint8_t) ((value & 0x000000FF) >> 0); 00283 p_encoded_data[1] = (uint8_t) ((value & 0x0000FF00) >> 8); 00284 p_encoded_data[2] = (uint8_t) ((value & 0x00FF0000) >> 16); 00285 p_encoded_data[3] = (uint8_t) ((value & 0xFF000000) >> 24); 00286 return sizeof(uint32_t); 00287 } 00288 00289 /**@brief Function for encoding a uint48 value. 00290 * 00291 * @param[in] value Value to be encoded. 00292 * @param[out] p_encoded_data Buffer where the encoded data is to be written. 00293 * 00294 * @return Number of bytes written. 00295 */ 00296 static __INLINE uint8_t uint48_encode(uint64_t value, uint8_t * p_encoded_data) 00297 { 00298 p_encoded_data[0] = (uint8_t) ((value & 0x0000000000FF) >> 0); 00299 p_encoded_data[1] = (uint8_t) ((value & 0x00000000FF00) >> 8); 00300 p_encoded_data[2] = (uint8_t) ((value & 0x000000FF0000) >> 16); 00301 p_encoded_data[3] = (uint8_t) ((value & 0x0000FF000000) >> 24); 00302 p_encoded_data[4] = (uint8_t) ((value & 0x00FF00000000) >> 32); 00303 p_encoded_data[5] = (uint8_t) ((value & 0xFF0000000000) >> 40); 00304 return 6; 00305 } 00306 00307 /**@brief Function for decoding a uint16 value. 00308 * 00309 * @param[in] p_encoded_data Buffer where the encoded data is stored. 00310 * 00311 * @return Decoded value. 00312 */ 00313 static __INLINE uint16_t uint16_decode(const uint8_t * p_encoded_data) 00314 { 00315 return ( (((uint16_t)((uint8_t *)p_encoded_data)[0])) | 00316 (((uint16_t)((uint8_t *)p_encoded_data)[1]) << 8 )); 00317 } 00318 00319 /**@brief Function for decoding a uint16 value in big-endian format. 00320 * 00321 * @param[in] p_encoded_data Buffer where the encoded data is stored. 00322 * 00323 * @return Decoded value. 00324 */ 00325 static __INLINE uint16_t uint16_big_decode(const uint8_t * p_encoded_data) 00326 { 00327 return ( (((uint16_t)((uint8_t *)p_encoded_data)[0]) << 8 ) | 00328 (((uint16_t)((uint8_t *)p_encoded_data)[1])) ); 00329 } 00330 00331 /**@brief Function for decoding a three-byte value. 00332 * 00333 * @param[in] p_encoded_data Buffer where the encoded data is stored. 00334 * 00335 * @return Decoded value (uint32_t). 00336 */ 00337 static __INLINE uint32_t uint24_decode(const uint8_t * p_encoded_data) 00338 { 00339 return ( (((uint32_t)((uint8_t *)p_encoded_data)[0]) << 0) | 00340 (((uint32_t)((uint8_t *)p_encoded_data)[1]) << 8) | 00341 (((uint32_t)((uint8_t *)p_encoded_data)[2]) << 16)); 00342 } 00343 00344 /**@brief Function for decoding a uint32 value. 00345 * 00346 * @param[in] p_encoded_data Buffer where the encoded data is stored. 00347 * 00348 * @return Decoded value. 00349 */ 00350 static __INLINE uint32_t uint32_decode(const uint8_t * p_encoded_data) 00351 { 00352 return ( (((uint32_t)((uint8_t *)p_encoded_data)[0]) << 0) | 00353 (((uint32_t)((uint8_t *)p_encoded_data)[1]) << 8) | 00354 (((uint32_t)((uint8_t *)p_encoded_data)[2]) << 16) | 00355 (((uint32_t)((uint8_t *)p_encoded_data)[3]) << 24 )); 00356 } 00357 00358 /**@brief Function for decoding a uint32 value in big-endian format. 00359 * 00360 * @param[in] p_encoded_data Buffer where the encoded data is stored. 00361 * 00362 * @return Decoded value. 00363 */ 00364 static __INLINE uint32_t uint32_big_decode(const uint8_t * p_encoded_data) 00365 { 00366 return ( (((uint32_t)((uint8_t *)p_encoded_data)[0]) << 24) | 00367 (((uint32_t)((uint8_t *)p_encoded_data)[1]) << 16) | 00368 (((uint32_t)((uint8_t *)p_encoded_data)[2]) << 8) | 00369 (((uint32_t)((uint8_t *)p_encoded_data)[3]) << 0) ); 00370 } 00371 00372 /**@brief Function for encoding a uint32 value in big-endian format. 00373 * 00374 * @param[in] value Value to be encoded. 00375 * @param[out] p_encoded_data Buffer where the encoded data will be written. 00376 * 00377 * @return Number of bytes written. 00378 */ 00379 static __INLINE uint8_t uint32_big_encode(uint32_t value, uint8_t * p_encoded_data) 00380 { 00381 #ifdef NRF51 00382 p_encoded_data[0] = (uint8_t) ((value & 0xFF000000) >> 24); 00383 p_encoded_data[1] = (uint8_t) ((value & 0x00FF0000) >> 16); 00384 p_encoded_data[2] = (uint8_t) ((value & 0x0000FF00) >> 8); 00385 p_encoded_data[3] = (uint8_t) ((value & 0x000000FF) >> 0); 00386 #elif NRF52 00387 *(uint32_t *)p_encoded_data = __REV(value); 00388 #endif 00389 return sizeof(uint32_t); 00390 } 00391 00392 /**@brief Function for decoding a uint48 value. 00393 * 00394 * @param[in] p_encoded_data Buffer where the encoded data is stored. 00395 * 00396 * @return Decoded value. (uint64_t) 00397 */ 00398 static __INLINE uint64_t uint48_decode(const uint8_t * p_encoded_data) 00399 { 00400 return ( (((uint64_t)((uint8_t *)p_encoded_data)[0]) << 0) | 00401 (((uint64_t)((uint8_t *)p_encoded_data)[1]) << 8) | 00402 (((uint64_t)((uint8_t *)p_encoded_data)[2]) << 16) | 00403 (((uint64_t)((uint8_t *)p_encoded_data)[3]) << 24) | 00404 (((uint64_t)((uint8_t *)p_encoded_data)[4]) << 32) | 00405 (((uint64_t)((uint8_t *)p_encoded_data)[5]) << 40 )); 00406 } 00407 00408 /** @brief Function for converting the input voltage (in milli volts) into percentage of 3.0 Volts. 00409 * 00410 * @details The calculation is based on a linearized version of the battery's discharge 00411 * curve. 3.0V returns 100% battery level. The limit for power failure is 2.1V and 00412 * is considered to be the lower boundary. 00413 * 00414 * The discharge curve for CR2032 is non-linear. In this model it is split into 00415 * 4 linear sections: 00416 * - Section 1: 3.0V - 2.9V = 100% - 42% (58% drop on 100 mV) 00417 * - Section 2: 2.9V - 2.74V = 42% - 18% (24% drop on 160 mV) 00418 * - Section 3: 2.74V - 2.44V = 18% - 6% (12% drop on 300 mV) 00419 * - Section 4: 2.44V - 2.1V = 6% - 0% (6% drop on 340 mV) 00420 * 00421 * These numbers are by no means accurate. Temperature and 00422 * load in the actual application is not accounted for! 00423 * 00424 * @param[in] mvolts The voltage in mV 00425 * 00426 * @return Battery level in percent. 00427 */ 00428 static __INLINE uint8_t battery_level_in_percent(const uint16_t mvolts) 00429 { 00430 uint8_t battery_level; 00431 00432 if (mvolts >= 3000) 00433 { 00434 battery_level = 100; 00435 } 00436 else if (mvolts > 2900) 00437 { 00438 battery_level = 100 - ((3000 - mvolts) * 58) / 100; 00439 } 00440 else if (mvolts > 2740) 00441 { 00442 battery_level = 42 - ((2900 - mvolts) * 24) / 160; 00443 } 00444 else if (mvolts > 2440) 00445 { 00446 battery_level = 18 - ((2740 - mvolts) * 12) / 300; 00447 } 00448 else if (mvolts > 2100) 00449 { 00450 battery_level = 6 - ((2440 - mvolts) * 6) / 340; 00451 } 00452 else 00453 { 00454 battery_level = 0; 00455 } 00456 00457 return battery_level; 00458 } 00459 00460 /**@brief Function for checking if a pointer value is aligned to a 4 byte boundary. 00461 * 00462 * @param[in] p Pointer value to be checked. 00463 * 00464 * @return TRUE if pointer is aligned to a 4 byte boundary, FALSE otherwise. 00465 */ 00466 static __INLINE bool is_word_aligned(void const* p) 00467 { 00468 return (((uintptr_t)p & 0x03) == 0); 00469 } 00470 00471 /** 00472 * @brief Function for checking if provided address is located in stack space. 00473 * 00474 * @param[in] ptr Pointer to be checked. 00475 * 00476 * @return true if address is in stack space, false otherwise. 00477 */ 00478 static __INLINE bool is_address_from_stack(void * ptr) 00479 { 00480 if (((uint32_t)ptr >= (uint32_t)STACK_BASE) && 00481 ((uint32_t)ptr < (uint32_t)STACK_TOP) ) 00482 { 00483 return true; 00484 } 00485 else 00486 { 00487 return false; 00488 } 00489 } 00490 00491 #endif // APP_UTIL_H__ 00492 00493 /** @} */ 00494
Generated on Wed Jul 13 2022 07:07:19 by
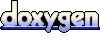