BLE_Nano nRF51 Central heart rate
Embed:
(wiki syntax)
Show/hide line numbers
app_error.h
Go to the documentation of this file.
00001 /* Copyright (c) 2013 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 * 00011 */ 00012 00013 /** @file 00014 * 00015 * @defgroup app_error Common application error handler 00016 * @{ 00017 * @ingroup app_common 00018 * 00019 * @brief Common application error handler and macros for utilizing a common error handler. 00020 */ 00021 00022 #ifndef APP_ERROR_H__ 00023 #define APP_ERROR_H__ 00024 00025 #include <stdint.h> 00026 #include <stdio.h> 00027 #include <stdbool.h> 00028 #include "nrf.h" 00029 #include "sdk_errors.h " 00030 #include "nordic_common.h" 00031 #include "nrf_log.h" 00032 #include "app_error_weak.h " 00033 00034 #define NRF_FAULT_ID_SDK_RANGE_START 0x00004000 /**< The start of the range of error IDs defined in the SDK. */ 00035 00036 /**@defgroup APP_ERROR_FAULT_IDS Fault ID types 00037 * @{ */ 00038 #define NRF_FAULT_ID_SDK_ERROR NRF_FAULT_ID_SDK_RANGE_START + 1 /**< An error stemming from a call to @ref APP_ERROR_CHECK or @ref APP_ERROR_CHECK_BOOL. The info parameter is a pointer to an @ref error_info_t variable. */ 00039 #define NRF_FAULT_ID_SDK_ASSERT NRF_FAULT_ID_SDK_RANGE_START + 2 /**< An error stemming from a call to ASSERT (nrf_assert.h). The info parameter is a pointer to an @ref assert_info_t variable. */ 00040 /**@} */ 00041 00042 /**@brief Structure containing info about an error of the type @ref NRF_FAULT_ID_SDK_ERROR. 00043 */ 00044 typedef struct 00045 { 00046 uint16_t line_num; /**< The line number where the error occurred. */ 00047 uint8_t const * p_file_name; /**< The file in which the error occurred. */ 00048 uint32_t err_code; /**< The error code representing the error that occurred. */ 00049 } error_info_t; 00050 00051 /**@brief Structure containing info about an error of the type @ref NRF_FAULT_ID_SDK_ASSERT. 00052 */ 00053 typedef struct 00054 { 00055 uint16_t line_num; /**< The line number where the error occurred. */ 00056 uint8_t const * p_file_name; /**< The file in which the error occurred. */ 00057 } assert_info_t; 00058 00059 /**@brief Function for error handling, which is called when an error has occurred. 00060 * 00061 * @param[in] error_code Error code supplied to the handler. 00062 * @param[in] line_num Line number where the handler is called. 00063 * @param[in] p_file_name Pointer to the file name. 00064 */ 00065 void app_error_handler(uint32_t error_code, uint32_t line_num, const uint8_t * p_file_name); 00066 00067 /**@brief Function for error handling, which is called when an error has occurred. 00068 * 00069 * @param[in] error_code Error code supplied to the handler. 00070 */ 00071 void app_error_handler_bare(ret_code_t error_code); 00072 00073 /**@brief Function for saving the parameters and entering an eternal loop, for debug purposes. 00074 * 00075 * @param[in] id Fault identifier. See @ref NRF_FAULT_IDS. 00076 * @param[in] pc The program counter of the instruction that triggered the fault, or 0 if 00077 * unavailable. 00078 * @param[in] info Optional additional information regarding the fault. Refer to each fault 00079 * identifier for details. 00080 */ 00081 void app_error_save_and_stop(uint32_t id, uint32_t pc, uint32_t info); 00082 00083 /**@brief Function for printing all error info (using nrf_log). 00084 * 00085 * @details Nrf_log library must be initialized using NRF_LOG_INIT macro before calling 00086 * this function. 00087 * 00088 * @param[in] id Fault identifier. See @ref NRF_FAULT_IDS. 00089 * @param[in] pc The program counter of the instruction that triggered the fault, or 0 if 00090 * unavailable. 00091 * @param[in] info Optional additional information regarding the fault. Refer to each fault 00092 * identifier for details. 00093 */ 00094 static __INLINE void app_error_log(uint32_t id, uint32_t pc, uint32_t info) 00095 { 00096 switch (id) 00097 { 00098 case NRF_FAULT_ID_SDK_ASSERT: 00099 NRF_LOG(NRF_LOG_COLOR_RED "\n*** ASSERTION FAILED ***\n"); 00100 if (((assert_info_t *)(info))->p_file_name) 00101 { 00102 NRF_LOG_PRINTF(NRF_LOG_COLOR_WHITE "Line Number: %u\n", (unsigned int) ((assert_info_t *)(info))->line_num); 00103 NRF_LOG_PRINTF("File Name: %s\n", ((assert_info_t *)(info))->p_file_name); 00104 } 00105 NRF_LOG_PRINTF(NRF_LOG_COLOR_DEFAULT "\n"); 00106 break; 00107 00108 case NRF_FAULT_ID_SDK_ERROR: 00109 NRF_LOG(NRF_LOG_COLOR_RED "\n*** APPLICATION ERROR *** \n" NRF_LOG_COLOR_WHITE); 00110 if (((error_info_t *)(info))->p_file_name) 00111 { 00112 NRF_LOG_PRINTF("Line Number: %u\n", (unsigned int) ((error_info_t *)(info))->line_num); 00113 NRF_LOG_PRINTF("File Name: %s\n", ((error_info_t *)(info))->p_file_name); 00114 } 00115 NRF_LOG_PRINTF("Error Code: 0x%X\n" NRF_LOG_COLOR_DEFAULT "\n", (unsigned int) ((error_info_t *)(info))->err_code); 00116 break; 00117 } 00118 } 00119 00120 /**@brief Function for printing all error info (using printf). 00121 * 00122 * @param[in] id Fault identifier. See @ref NRF_FAULT_IDS. 00123 * @param[in] pc The program counter of the instruction that triggered the fault, or 0 if 00124 * unavailable. 00125 * @param[in] info Optional additional information regarding the fault. Refer to each fault 00126 * identifier for details. 00127 */ 00128 //lint -save -e438 00129 static __INLINE void app_error_print(uint32_t id, uint32_t pc, uint32_t info) 00130 { 00131 unsigned int tmp = id; 00132 printf("app_error_print():\r\n"); 00133 printf("Fault identifier: 0x%X\r\n", tmp); 00134 printf("Program counter: 0x%X\r\n", tmp = pc); 00135 printf("Fault information: 0x%X\r\n", tmp = info); 00136 00137 switch (id) 00138 { 00139 case NRF_FAULT_ID_SDK_ASSERT: 00140 printf("Line Number: %u\r\n", tmp = ((assert_info_t *)(info))->line_num); 00141 printf("File Name: %s\r\n", ((assert_info_t *)(info))->p_file_name); 00142 break; 00143 00144 case NRF_FAULT_ID_SDK_ERROR: 00145 printf("Line Number: %u\r\n", tmp = ((error_info_t *)(info))->line_num); 00146 printf("File Name: %s\r\n", ((error_info_t *)(info))->p_file_name); 00147 printf("Error Code: 0x%X\r\n", tmp = ((error_info_t *)(info))->err_code); 00148 break; 00149 } 00150 } 00151 //lint -restore 00152 00153 00154 /**@brief Macro for calling error handler function. 00155 * 00156 * @param[in] ERR_CODE Error code supplied to the error handler. 00157 */ 00158 #ifdef DEBUG 00159 #define APP_ERROR_HANDLER(ERR_CODE) \ 00160 do \ 00161 { \ 00162 app_error_handler((ERR_CODE), __LINE__, (uint8_t*) __FILE__); \ 00163 } while (0) 00164 #else 00165 #define APP_ERROR_HANDLER(ERR_CODE) \ 00166 do \ 00167 { \ 00168 app_error_handler_bare((ERR_CODE)); \ 00169 } while (0) 00170 #endif 00171 /**@brief Macro for calling error handler function if supplied error code any other than NRF_SUCCESS. 00172 * 00173 * @param[in] ERR_CODE Error code supplied to the error handler. 00174 */ 00175 #define APP_ERROR_CHECK(ERR_CODE) \ 00176 do \ 00177 { \ 00178 const uint32_t LOCAL_ERR_CODE = (ERR_CODE); \ 00179 if (LOCAL_ERR_CODE != NRF_SUCCESS) \ 00180 { \ 00181 APP_ERROR_HANDLER(LOCAL_ERR_CODE); \ 00182 } \ 00183 } while (0) 00184 00185 /**@brief Macro for calling error handler function if supplied boolean value is false. 00186 * 00187 * @param[in] BOOLEAN_VALUE Boolean value to be evaluated. 00188 */ 00189 #define APP_ERROR_CHECK_BOOL(BOOLEAN_VALUE) \ 00190 do \ 00191 { \ 00192 const uint32_t LOCAL_BOOLEAN_VALUE = (BOOLEAN_VALUE); \ 00193 if (!LOCAL_BOOLEAN_VALUE) \ 00194 { \ 00195 APP_ERROR_HANDLER(0); \ 00196 } \ 00197 } while (0) 00198 00199 #endif // APP_ERROR_H__ 00200 00201 /** @} */ 00202
Generated on Wed Jul 13 2022 07:07:19 by
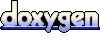