BLE_Nano nRF51 Central heart rate
Embed:
(wiki syntax)
Show/hide line numbers
app_error.c
Go to the documentation of this file.
00001 /* Copyright (c) 2014 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 * 00011 */ 00012 00013 /** @file 00014 * 00015 * @defgroup app_error Common application error handler 00016 * @{ 00017 * @ingroup app_common 00018 * 00019 * @brief Common application error handler. 00020 */ 00021 00022 #include "nrf.h" 00023 #include <stdio.h> 00024 #include "app_error.h " 00025 #include "nordic_common.h" 00026 #include "sdk_errors.h " 00027 #include "nrf_log.h" 00028 00029 #ifdef DEBUG 00030 #include "bsp.h" 00031 #endif 00032 00033 00034 00035 /**@brief Function for error handling, which is called when an error has occurred. 00036 * 00037 * @warning This handler is an example only and does not fit a final product. You need to analyze 00038 * how your product is supposed to react in case of error. 00039 * 00040 * @param[in] error_code Error code supplied to the handler. 00041 * @param[in] line_num Line number where the handler is called. 00042 * @param[in] p_file_name Pointer to the file name. 00043 */ 00044 00045 /*lint -save -e14 */ 00046 void app_error_handler(ret_code_t error_code, uint32_t line_num, const uint8_t * p_file_name) 00047 { 00048 error_info_t error_info = 00049 { 00050 .line_num = line_num, 00051 .p_file_name = p_file_name, 00052 .err_code = error_code, 00053 }; 00054 app_error_fault_handler(NRF_FAULT_ID_SDK_ERROR, 0, (uint32_t)(&error_info)); 00055 00056 UNUSED_VARIABLE(error_info); 00057 } 00058 00059 /*lint -save -e14 */ 00060 void app_error_handler_bare(ret_code_t error_code) 00061 { 00062 error_info_t error_info = 00063 { 00064 .line_num = 0, 00065 .p_file_name = NULL, 00066 .err_code = error_code, 00067 }; 00068 app_error_fault_handler(NRF_FAULT_ID_SDK_ERROR, 0, (uint32_t)(&error_info)); 00069 00070 UNUSED_VARIABLE(error_info); 00071 } 00072 00073 00074 void app_error_save_and_stop(uint32_t id, uint32_t pc, uint32_t info) 00075 { 00076 /* static error variables - in order to prevent removal by optimizers */ 00077 static volatile struct 00078 { 00079 uint32_t fault_id; 00080 uint32_t pc; 00081 uint32_t error_info; 00082 assert_info_t * p_assert_info; 00083 error_info_t * p_error_info; 00084 ret_code_t err_code; 00085 uint32_t line_num; 00086 const uint8_t * p_file_name; 00087 } m_error_data = {0}; 00088 00089 // The following variable helps Keil keep the call stack visible, in addition, it can be set to 00090 // 0 in the debugger to continue executing code after the error check. 00091 volatile bool loop = true; 00092 UNUSED_VARIABLE(loop); 00093 00094 m_error_data.fault_id = id; 00095 m_error_data.pc = pc; 00096 m_error_data.error_info = info; 00097 00098 switch (id) 00099 { 00100 case NRF_FAULT_ID_SDK_ASSERT: 00101 m_error_data.p_assert_info = (assert_info_t *)info; 00102 m_error_data.line_num = m_error_data.p_assert_info->line_num; 00103 m_error_data.p_file_name = m_error_data.p_assert_info->p_file_name; 00104 break; 00105 00106 case NRF_FAULT_ID_SDK_ERROR: 00107 m_error_data.p_error_info = (error_info_t *)info; 00108 m_error_data.err_code = m_error_data.p_error_info->err_code; 00109 m_error_data.line_num = m_error_data.p_error_info->line_num; 00110 m_error_data.p_file_name = m_error_data.p_error_info->p_file_name; 00111 break; 00112 } 00113 00114 UNUSED_VARIABLE(m_error_data); 00115 00116 // If printing is disrupted, remove the irq calls, or set the loop variable to 0 in the debugger. 00117 __disable_irq(); 00118 00119 while(loop); 00120 00121 __enable_irq(); 00122 } 00123 00124 /*lint -restore */ 00125
Generated on Wed Jul 13 2022 07:07:19 by
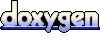