
B+IMU+SD
Dependencies: BMI160 RTC SDFileSystem USBDevice max32630fthr sd-driver
Fork of MPSMAXbutton by
Default.h
00001 00002 #ifndef DEFAULT_H_ 00003 #define DEFAULT_H_ 00004 00005 #include "sc_types.h" 00006 00007 #ifdef __cplusplus 00008 extern "C" { 00009 #endif 00010 00011 /*! \file Header of the state machine 'default'. 00012 */ 00013 00014 00015 /*! Enumeration of all states */ 00016 typedef enum 00017 { 00018 Default_last_state, 00019 Default_main_region_Functions, 00020 Default_main_region_Menu, 00021 Default_main_region_Ready, 00022 Default_main_region_Function_Selected, 00023 Default_r1_Function_Ready, 00024 Default_r1_Function_Active, 00025 Default_r1_Alarm, 00026 Default_r1_Move_Down, 00027 Default_r1_Move_Up 00028 } DefaultStates; 00029 00030 /*! Type definition of the data structure for the DefaultIface interface scope. */ 00031 typedef struct 00032 { 00033 sc_boolean mode_raised; 00034 sc_boolean down_raised; 00035 sc_boolean up_raised; 00036 sc_boolean back_raised; 00037 sc_boolean home_raised; 00038 sc_integer select1; 00039 sc_integer select2; 00040 } DefaultIface ; 00041 00042 /*! Type definition of the data structure for the DefaultIfaceFunc interface scope. */ 00043 typedef struct 00044 { 00045 sc_boolean Selected_raised; 00046 sc_boolean Deselect_raised; 00047 sc_boolean Alarmset_raised; 00048 sc_boolean MoveDown_raised; 00049 sc_boolean MoveUp_raised; 00050 sc_integer t; 00051 } DefaultIfaceFunc ; 00052 00053 /*! Type definition of the data structure for the DefaultTimeEvents interface scope. */ 00054 typedef struct 00055 { 00056 sc_boolean default_r1_Move_Down_tev0_raised; 00057 sc_boolean default_r1_Move_Up_tev0_raised; 00058 } DefaultTimeEvents ; 00059 00060 00061 /*! Define dimension of the state configuration vector for orthogonal states. */ 00062 #define DEFAULT_MAX_ORTHOGONAL_STATES 2 00063 00064 /*! Define maximum number of time events that can be active at once */ 00065 #define DEFAULT_MAX_PARALLEL_TIME_EVENTS 1 00066 00067 /*! Define indices of states in the StateConfVector */ 00068 #define SCVI_DEFAULT_MAIN_REGION_FUNCTIONS 0 00069 #define SCVI_DEFAULT_MAIN_REGION_MENU 0 00070 #define SCVI_DEFAULT_MAIN_REGION_READY 0 00071 #define SCVI_DEFAULT_MAIN_REGION_FUNCTION_SELECTED 0 00072 #define SCVI_DEFAULT_R1_FUNCTION_READY 1 00073 #define SCVI_DEFAULT_R1_FUNCTION_ACTIVE 1 00074 #define SCVI_DEFAULT_R1_ALARM 1 00075 #define SCVI_DEFAULT_R1_MOVE_DOWN 1 00076 #define SCVI_DEFAULT_R1_MOVE_UP 1 00077 00078 /*! 00079 * Type definition of the data structure for the Default state machine. 00080 * This data structure has to be allocated by the client code. 00081 */ 00082 typedef struct 00083 { 00084 DefaultStates stateConfVector[DEFAULT_MAX_ORTHOGONAL_STATES]; 00085 sc_ushort stateConfVectorPosition; 00086 00087 DefaultIface iface; 00088 DefaultIfaceFunc ifaceFunc; 00089 DefaultTimeEvents timeEvents; 00090 } Default ; 00091 00092 00093 /*! Initializes the Default state machine data structures. Must be called before first usage.*/ 00094 extern void default_init(Default * handle); 00095 00096 /*! Activates the state machine */ 00097 extern void default_enter(Default * handle); 00098 00099 /*! Deactivates the state machine */ 00100 extern void default_exit(Default * handle); 00101 00102 /*! Performs a 'run to completion' step. */ 00103 extern void default_runCycle(Default * handle); 00104 00105 /*! Raises a time event. */ 00106 extern void default_raiseTimeEvent(const Default * handle, sc_eventid evid); 00107 00108 /*! Raises the in event 'mode' that is defined in the default interface scope. */ 00109 extern void defaultIface_raise_mode(Default * handle); 00110 00111 /*! Raises the in event 'down' that is defined in the default interface scope. */ 00112 extern void defaultIface_raise_down(Default * handle); 00113 00114 /*! Raises the in event 'up' that is defined in the default interface scope. */ 00115 extern void defaultIface_raise_up(Default * handle); 00116 00117 /*! Raises the in event 'back' that is defined in the default interface scope. */ 00118 extern void defaultIface_raise_back(Default * handle); 00119 00120 /*! Raises the in event 'home' that is defined in the default interface scope. */ 00121 extern void defaultIface_raise_home(Default * handle); 00122 00123 /*! Gets the value of the variable 'select1' that is defined in the default interface scope. */ 00124 extern sc_integer defaultIface_get_select1(const Default * handle); 00125 /*! Sets the value of the variable 'select1' that is defined in the default interface scope. */ 00126 extern void defaultIface_set_select1(Default * handle, sc_integer value); 00127 /*! Gets the value of the variable 'select2' that is defined in the default interface scope. */ 00128 extern sc_integer defaultIface_get_select2(const Default * handle); 00129 /*! Sets the value of the variable 'select2' that is defined in the default interface scope. */ 00130 extern void defaultIface_set_select2(Default * handle, sc_integer value); 00131 /*! Raises the in event 'Selected' that is defined in the interface scope 'Func'. */ 00132 extern void defaultIfaceFunc_raise_selected(Default * handle); 00133 00134 /*! Raises the in event 'Deselect' that is defined in the interface scope 'Func'. */ 00135 extern void defaultIfaceFunc_raise_deselect(Default * handle); 00136 00137 /*! Raises the in event 'Alarmset' that is defined in the interface scope 'Func'. */ 00138 extern void defaultIfaceFunc_raise_alarmset(Default * handle); 00139 00140 /*! Raises the in event 'MoveDown' that is defined in the interface scope 'Func'. */ 00141 extern void defaultIfaceFunc_raise_moveDown(Default * handle); 00142 00143 /*! Raises the in event 'MoveUp' that is defined in the interface scope 'Func'. */ 00144 extern void defaultIfaceFunc_raise_moveUp(Default * handle); 00145 00146 /*! Gets the value of the variable 't' that is defined in the interface scope 'Func'. */ 00147 extern sc_integer defaultIfaceFunc_get_t(const Default * handle); 00148 /*! Sets the value of the variable 't' that is defined in the interface scope 'Func'. */ 00149 extern void defaultIfaceFunc_set_t(Default * handle, sc_integer value); 00150 00151 /*! 00152 * Checks whether the state machine is active (until 2.4.1 this method was used for states). 00153 * A state machine is active if it was entered. It is inactive if it has not been entered at all or if it has been exited. 00154 */ 00155 extern sc_boolean default_isActive(const Default * handle); 00156 00157 /*! 00158 * Checks if all active states are final. 00159 * If there are no active states then the state machine is considered being inactive. In this case this method returns false. 00160 */ 00161 extern sc_boolean default_isFinal(const Default * handle); 00162 00163 /*! Checks if the specified state is active (until 2.4.1 the used method for states was called isActive()). */ 00164 extern sc_boolean default_isStateActive(const Default * handle, DefaultStates state); 00165 00166 00167 00168 #ifdef __cplusplus 00169 } 00170 #endif 00171 00172 #endif /* DEFAULT_H_ */ 00173
Generated on Tue Jul 12 2022 19:03:03 by
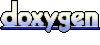