Wiznet W750Sr Mqtt , IFTT to Voice control your fish tank from anywhere in the world
Dependencies: MQTT WIZnetInterface mbed-src
main.cpp
00001 #include "mbed.h" 00002 #include "MQTTEthernet.h" 00003 #include "MQTTClient.h" 00004 #include <sstream> 00005 #include <string> 00006 00007 Serial s(PA_13,PA_14); // Communication with the Arduino Board 00008 00009 Serial out(USBTX,USBRX); // Debuging through USB 00010 00011 void messageArrived(MQTT::MessageData& md) 00012 { 00013 MQTT::Message &message = md.message; 00014 out.printf("Message arrived: qos %d, retained %d, dup %d, packetid %d\n", message.qos, message.retained, message.dup, message.id); 00015 out.printf("Payload %.*s\n", message.payloadlen, (char*)message.payload); 00016 s.printf("%.*s\n", message.payloadlen, (char*)message.payload);//To send serial data to arduino 00017 int i = atoi((char*)message.payload); 00018 switch(i) { 00019 case 0: //start servo to feed the fish 00020 out.printf("Feeding"); 00021 s.printf("Feed"); 00022 break; 00023 case 1: //turn lights on 00024 out.printf("Lights on"); 00025 s.printf("ON"); 00026 00027 break; 00028 case 2: //turn lights off 00029 out.printf("Lights off"); 00030 s.printf("OFF"); 00031 00032 break; 00033 case 3: //turn Dancing led strip on 00034 out.printf("Led Flashing"); 00035 s.printf("Dance"); 00036 00037 break; 00038 case 4: //turn Dancing led strip off 00039 out.printf("Led Not Flashing"); 00040 s.printf("NoDance"); 00041 00042 break; 00043 00044 } 00045 } 00046 00047 void baud(int baudrate) 00048 { 00049 00050 s.baud(baudrate); 00051 } 00052 00053 00054 00055 00056 int main(void) 00057 { 00058 00059 char* topic1 = "******/feeds/welcome-feed"; 00060 // char* topic2 = "******/feeds/lights"; //****** type your username on IFTT instead of the stars 00061 //char* topic3 = "******/feeds/dance"; 00062 00063 printf("starting...\n"); 00064 00065 00066 MQTTEthernet ipstack = MQTTEthernet(); 00067 00068 MQTT::Client<MQTTEthernet, Countdown> client = MQTT::Client<MQTTEthernet, Countdown>(ipstack); 00069 00070 char* hostname = "io.adafruit.com";//your MQTT broker host 00071 int port = 1883; // broker port number 00072 00073 int rc = ipstack.connect(hostname, port); 00074 00075 out.printf("rc from TCP connect is %d\n", rc); 00076 00077 if (rc != 0) 00078 out.printf("rc from TCP connect is %d\n", rc); 00079 00080 char MQTTClientID[30]; 00081 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00082 data.MQTTVersion = 3; 00083 sprintf(MQTTClientID,"WIZwiki-%d",rand()%1000); 00084 00085 data.clientID.cstring = MQTTClientID; 00086 data.username.cstring = "*******"; //****** type your username on IFTT instead of the stars 00087 data.password.cstring = "************************"; //****** type your Password on IFTT instead of the stars 00088 if ((rc = client.connect(data)) != 0) 00089 printf("rc from MQTT connect is %d\n", rc); 00090 00091 00092 if ((rc = client.subscribe(topic1, MQTT::QOS0, messageArrived)) != 0) { 00093 out.printf("rc from MQTT subscribe is %d\n", rc); 00094 00095 00096 } 00097 00098 while (true) { 00099 00100 00101 00102 client.yield(6); 00103 00104 } 00105 }
Generated on Wed Jul 13 2022 14:24:21 by
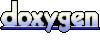