
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "MobileLCD.h" 00003 00004 #define PI 3.1415926535897 00005 MobileLCD lcd(5, 6, 7, 8, 9); 00006 struct point 00007 { 00008 int x; 00009 int y; 00010 }; 00011 00012 point points[3]; 00013 point current; 00014 00015 int main() 00016 { 00017 for(int i=0; i<3; i++) 00018 { 00019 points[i].x = 65+60*sin(2*PI*i/3); 00020 points[i].y = 65+60*cos(2*PI*i/3); 00021 } 00022 lcd.background(0xFFFFFF); 00023 lcd.cls(); 00024 current = points[rand()%3]; 00025 while(1) 00026 { 00027 lcd.pixel(current.x, current.y, 0x000000); 00028 point nextpoint = points[rand()%3]; 00029 current.x = (current.x + nextpoint.x)/2; 00030 current.y = (current.y + nextpoint.y)/2; 00031 wait(0.01); 00032 } 00033 }
Generated on Tue Jul 12 2022 18:38:22 by
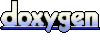