Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_ll_usb.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_ll_usb.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of USB Core HAL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_LL_USB_H 00040 #define __STM32L4xx_LL_USB_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 #if defined(STM32L475xx) || defined(STM32L476xx) || defined(STM32L485xx) || defined(STM32L486xx) 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include "stm32l4xx_hal_def.h" 00050 00051 /** @addtogroup STM32L4xx_HAL 00052 * @{ 00053 */ 00054 00055 /** @addtogroup USB_Core 00056 * @{ 00057 */ 00058 00059 /* Exported types ------------------------------------------------------------*/ 00060 00061 /** 00062 * @brief USB Mode definition 00063 */ 00064 typedef enum 00065 { 00066 USB_OTG_DEVICE_MODE = 0, 00067 USB_OTG_HOST_MODE = 1, 00068 USB_OTG_DRD_MODE = 2 00069 00070 }USB_OTG_ModeTypeDef; 00071 00072 /** 00073 * @brief URB States definition 00074 */ 00075 typedef enum { 00076 URB_IDLE = 0, 00077 URB_DONE, 00078 URB_NOTREADY, 00079 URB_NYET, 00080 URB_ERROR, 00081 URB_STALL 00082 00083 }USB_OTG_URBStateTypeDef; 00084 00085 /** 00086 * @brief Host channel States definition 00087 */ 00088 typedef enum { 00089 HC_IDLE = 0, 00090 HC_XFRC, 00091 HC_HALTED, 00092 HC_NAK, 00093 HC_NYET, 00094 HC_STALL, 00095 HC_XACTERR, 00096 HC_BBLERR, 00097 HC_DATATGLERR 00098 00099 }USB_OTG_HCStateTypeDef; 00100 00101 /** 00102 * @brief PCD Initialization Structure definition 00103 */ 00104 typedef struct 00105 { 00106 uint32_t dev_endpoints; /*!< Device Endpoints number. 00107 This parameter depends on the used USB core. 00108 This parameter must be a number between Min_Data = 1 and Max_Data = 15 */ 00109 00110 uint32_t Host_channels; /*!< Host Channels number. 00111 This parameter Depends on the used USB core. 00112 This parameter must be a number between Min_Data = 1 and Max_Data = 15 */ 00113 00114 uint32_t speed; /*!< USB Core speed. 00115 This parameter can be any value of @ref USB_Core_Speed_ */ 00116 00117 uint32_t dma_enable ; /*!< Enable or disable of the USB embedded DMA. */ 00118 00119 uint32_t ep0_mps; /*!< Set the Endpoint 0 Max Packet size. 00120 This parameter can be any value of @ref USB_EP0_MPS_ */ 00121 00122 uint32_t phy_itface; /*!< Select the used PHY interface. 00123 This parameter can be any value of @ref USB_Core_PHY_ */ 00124 00125 uint32_t Sof_enable ; /*!< Enable or disable the output of the SOF signal. */ 00126 00127 uint32_t low_power_enable ; /*!< Enable or disable the low power mode. */ 00128 00129 uint32_t lpm_enable ; /*!< Enable or disable Battery charging. */ 00130 00131 uint32_t battery_charging_enable ; /*!< Enable or disable Battery charging. */ 00132 00133 uint32_t vbus_sensing_enable ; /*!< Enable or disable the VBUS Sensing feature. */ 00134 00135 uint32_t use_dedicated_ep1 ; /*!< Enable or disable the use of the dedicated EP1 interrupt. */ 00136 00137 uint32_t use_external_vbus ; /*!< Enable or disable the use of the external VBUS. */ 00138 00139 }USB_OTG_CfgTypeDef; 00140 00141 typedef struct 00142 { 00143 uint8_t num; /*!< Endpoint number 00144 This parameter must be a number between Min_Data = 1 and Max_Data = 15 */ 00145 00146 uint8_t is_in; /*!< Endpoint direction 00147 This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ 00148 00149 uint8_t is_stall; /*!< Endpoint stall condition 00150 This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ 00151 00152 uint8_t type; /*!< Endpoint type 00153 This parameter can be any value of @ref USB_EP_Type_ */ 00154 00155 uint8_t data_pid_start; /*!< Initial data PID 00156 This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ 00157 00158 uint8_t even_odd_frame; /*!< IFrame parity 00159 This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ 00160 00161 uint16_t tx_fifo_num; /*!< Transmission FIFO number 00162 This parameter must be a number between Min_Data = 1 and Max_Data = 15 */ 00163 00164 uint32_t maxpacket; /*!< Endpoint Max packet size 00165 This parameter must be a number between Min_Data = 0 and Max_Data = 64KB */ 00166 00167 uint8_t *xfer_buff; /*!< Pointer to transfer buffer */ 00168 00169 uint32_t dma_addr; /*!< 32 bits aligned transfer buffer address */ 00170 00171 uint32_t xfer_len; /*!< Current transfer length */ 00172 00173 uint32_t xfer_count; /*!< Partial transfer length in case of multi packet transfer */ 00174 00175 }USB_OTG_EPTypeDef; 00176 00177 typedef struct 00178 { 00179 uint8_t dev_addr ; /*!< USB device address. 00180 This parameter must be a number between Min_Data = 1 and Max_Data = 255 */ 00181 00182 uint8_t ch_num; /*!< Host channel number. 00183 This parameter must be a number between Min_Data = 1 and Max_Data = 15 */ 00184 00185 uint8_t ep_num; /*!< Endpoint number. 00186 This parameter must be a number between Min_Data = 1 and Max_Data = 15 */ 00187 00188 uint8_t ep_is_in; /*!< Endpoint direction 00189 This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ 00190 00191 uint8_t speed; /*!< USB Host speed. 00192 This parameter can be any value of @ref USB_Core_Speed_ */ 00193 00194 uint8_t do_ping; /*!< Enable or disable the use of the PING protocol for HS mode. */ 00195 00196 uint8_t process_ping; /*!< Execute the PING protocol for HS mode. */ 00197 00198 uint8_t ep_type; /*!< Endpoint Type. 00199 This parameter can be any value of @ref USB_EP_Type_ */ 00200 00201 uint16_t max_packet; /*!< Endpoint Max packet size. 00202 This parameter must be a number between Min_Data = 0 and Max_Data = 64KB */ 00203 00204 uint8_t data_pid; /*!< Initial data PID. 00205 This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ 00206 00207 uint8_t *xfer_buff; /*!< Pointer to transfer buffer. */ 00208 00209 uint32_t xfer_len; /*!< Current transfer length. */ 00210 00211 uint32_t xfer_count; /*!< Partial transfer length in case of multi packet transfer. */ 00212 00213 uint8_t toggle_in; /*!< IN transfer current toggle flag. 00214 This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ 00215 00216 uint8_t toggle_out; /*!< OUT transfer current toggle flag 00217 This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ 00218 00219 uint32_t dma_addr; /*!< 32 bits aligned transfer buffer address. */ 00220 00221 uint32_t ErrCnt; /*!< Host channel error count.*/ 00222 00223 USB_OTG_URBStateTypeDef urb_state; /*!< URB state. 00224 This parameter can be any value of @ref USB_OTG_URBStateTypeDef */ 00225 00226 USB_OTG_HCStateTypeDef state; /*!< Host Channel state. 00227 This parameter can be any value of @ref USB_OTG_HCStateTypeDef */ 00228 00229 }USB_OTG_HCTypeDef; 00230 00231 /* Exported constants --------------------------------------------------------*/ 00232 00233 /** @defgroup PCD_Exported_Constants PCD Exported Constants 00234 * @{ 00235 */ 00236 00237 /** @defgroup USB_Core_Mode_ USB Core Mode 00238 * @{ 00239 */ 00240 #define USB_OTG_MODE_DEVICE 0 00241 #define USB_OTG_MODE_HOST 1 00242 #define USB_OTG_MODE_DRD 2 00243 /** 00244 * @} 00245 */ 00246 00247 /** @defgroup USB_Core_Speed_ USB Core Speed 00248 * @{ 00249 */ 00250 #define USB_OTG_SPEED_HIGH 0 00251 #define USB_OTG_SPEED_HIGH_IN_FULL 1 00252 #define USB_OTG_SPEED_LOW 2 00253 #define USB_OTG_SPEED_FULL 3 00254 /** 00255 * @} 00256 */ 00257 00258 /** @defgroup USB_Core_PHY_ USB Core PHY 00259 * @{ 00260 */ 00261 #define USB_OTG_EMBEDDED_PHY 1 00262 /** 00263 * @} 00264 */ 00265 00266 /** @defgroup USB_Core_MPS_ USB Core MPS 00267 * @{ 00268 */ 00269 #define USB_OTG_FS_MAX_PACKET_SIZE 64 00270 #define USB_OTG_MAX_EP0_SIZE 64 00271 /** 00272 * @} 00273 */ 00274 00275 /** @defgroup USB_Core_Phy_Frequency_ USB Core Phy Frequency 00276 * @{ 00277 */ 00278 #define DSTS_ENUMSPD_HS_PHY_30MHZ_OR_60MHZ (0 << 1) 00279 #define DSTS_ENUMSPD_FS_PHY_30MHZ_OR_60MHZ (1 << 1) 00280 #define DSTS_ENUMSPD_LS_PHY_6MHZ (2 << 1) 00281 #define DSTS_ENUMSPD_FS_PHY_48MHZ (3 << 1) 00282 /** 00283 * @} 00284 */ 00285 00286 /** @defgroup USB_CORE_Frame_Interval_ USB CORE Frame Interval 00287 * @{ 00288 */ 00289 #define DCFG_FRAME_INTERVAL_80 0 00290 #define DCFG_FRAME_INTERVAL_85 1 00291 #define DCFG_FRAME_INTERVAL_90 2 00292 #define DCFG_FRAME_INTERVAL_95 3 00293 /** 00294 * @} 00295 */ 00296 00297 /** @defgroup USB_EP0_MPS_ USB EP0 MPS 00298 * @{ 00299 */ 00300 #define DEP0CTL_MPS_64 0 00301 #define DEP0CTL_MPS_32 1 00302 #define DEP0CTL_MPS_16 2 00303 #define DEP0CTL_MPS_8 3 00304 /** 00305 * @} 00306 */ 00307 00308 /** @defgroup USB_EP_Speed_ USB EP Speed 00309 * @{ 00310 */ 00311 #define EP_SPEED_LOW 0 00312 #define EP_SPEED_FULL 1 00313 #define EP_SPEED_HIGH 2 00314 /** 00315 * @} 00316 */ 00317 00318 /** @defgroup USB_EP_Type_ USB EP Type 00319 * @{ 00320 */ 00321 #define EP_TYPE_CTRL 0 00322 #define EP_TYPE_ISOC 1 00323 #define EP_TYPE_BULK 2 00324 #define EP_TYPE_INTR 3 00325 #define EP_TYPE_MSK 3 00326 /** 00327 * @} 00328 */ 00329 00330 /** @defgroup USB_STS_Defines_ USB STS Defines 00331 * @{ 00332 */ 00333 #define STS_GOUT_NAK 1 00334 #define STS_DATA_UPDT 2 00335 #define STS_XFER_COMP 3 00336 #define STS_SETUP_COMP 4 00337 #define STS_SETUP_UPDT 6 00338 /** 00339 * @} 00340 */ 00341 00342 /** @defgroup HCFG_SPEED_Defines_ HCFG SPEED Defines 00343 * @{ 00344 */ 00345 #define HCFG_30_60_MHZ 0 00346 #define HCFG_48_MHZ 1 00347 #define HCFG_6_MHZ 2 00348 /** 00349 * @} 00350 */ 00351 00352 /** @defgroup HPRT0_PRTSPD_SPEED_Defines_ HPRT0 PRTSPD SPEED Defines 00353 * @{ 00354 */ 00355 #define HPRT0_PRTSPD_HIGH_SPEED 0 00356 #define HPRT0_PRTSPD_FULL_SPEED 1 00357 #define HPRT0_PRTSPD_LOW_SPEED 2 00358 /** 00359 * @} 00360 */ 00361 00362 #define HCCHAR_CTRL 0 00363 #define HCCHAR_ISOC 1 00364 #define HCCHAR_BULK 2 00365 #define HCCHAR_INTR 3 00366 00367 #define HC_PID_DATA0 0 00368 #define HC_PID_DATA2 1 00369 #define HC_PID_DATA1 2 00370 #define HC_PID_SETUP 3 00371 00372 #define GRXSTS_PKTSTS_IN 2 00373 #define GRXSTS_PKTSTS_IN_XFER_COMP 3 00374 #define GRXSTS_PKTSTS_DATA_TOGGLE_ERR 5 00375 #define GRXSTS_PKTSTS_CH_HALTED 7 00376 00377 #define USBx_PCGCCTL *(__IO uint32_t *)((uint32_t)USBx + USB_OTG_PCGCCTL_BASE) 00378 #define USBx_HPRT0 *(__IO uint32_t *)((uint32_t)USBx + USB_OTG_HOST_PORT_BASE) 00379 00380 #define USBx_DEVICE ((USB_OTG_DeviceTypeDef *)((uint32_t )USBx + USB_OTG_DEVICE_BASE)) 00381 #define USBx_INEP(i) ((USB_OTG_INEndpointTypeDef *)((uint32_t)USBx + USB_OTG_IN_ENDPOINT_BASE + (i)*USB_OTG_EP_REG_SIZE)) 00382 #define USBx_OUTEP(i) ((USB_OTG_OUTEndpointTypeDef *)((uint32_t)USBx + USB_OTG_OUT_ENDPOINT_BASE + (i)*USB_OTG_EP_REG_SIZE)) 00383 #define USBx_DFIFO(i) *(__IO uint32_t *)((uint32_t)USBx + USB_OTG_FIFO_BASE + (i) * USB_OTG_FIFO_SIZE) 00384 00385 #define USBx_HOST ((USB_OTG_HostTypeDef *)((uint32_t )USBx + USB_OTG_HOST_BASE)) 00386 #define USBx_HC(i) ((USB_OTG_HostChannelTypeDef *)((uint32_t)USBx + USB_OTG_HOST_CHANNEL_BASE + (i)*USB_OTG_HOST_CHANNEL_SIZE)) 00387 /** 00388 * @} 00389 */ 00390 00391 /* Exported macro ------------------------------------------------------------*/ 00392 #define USB_MASK_INTERRUPT(__INSTANCE__, __INTERRUPT__) ((__INSTANCE__)->GINTMSK &= ~(__INTERRUPT__)) 00393 #define USB_UNMASK_INTERRUPT(__INSTANCE__, __INTERRUPT__) ((__INSTANCE__)->GINTMSK |= (__INTERRUPT__)) 00394 00395 #define CLEAR_IN_EP_INTR(__EPNUM__, __INTERRUPT__) (USBx_INEP(__EPNUM__)->DIEPINT = (__INTERRUPT__)) 00396 #define CLEAR_OUT_EP_INTR(__EPNUM__, __INTERRUPT__) (USBx_OUTEP(__EPNUM__)->DOEPINT = (__INTERRUPT__)) 00397 00398 /* Exported functions --------------------------------------------------------*/ 00399 HAL_StatusTypeDef USB_CoreInit(USB_OTG_GlobalTypeDef *USBx, USB_OTG_CfgTypeDef Init); 00400 HAL_StatusTypeDef USB_DevInit(USB_OTG_GlobalTypeDef *USBx, USB_OTG_CfgTypeDef Init); 00401 HAL_StatusTypeDef USB_EnableGlobalInt(USB_OTG_GlobalTypeDef *USBx); 00402 HAL_StatusTypeDef USB_DisableGlobalInt(USB_OTG_GlobalTypeDef *USBx); 00403 HAL_StatusTypeDef USB_SetCurrentMode(USB_OTG_GlobalTypeDef *USBx , USB_OTG_ModeTypeDef mode); 00404 HAL_StatusTypeDef USB_SetDevSpeed(USB_OTG_GlobalTypeDef *USBx , uint8_t speed); 00405 HAL_StatusTypeDef USB_FlushRxFifo (USB_OTG_GlobalTypeDef *USBx); 00406 HAL_StatusTypeDef USB_FlushTxFifo (USB_OTG_GlobalTypeDef *USBx, uint32_t num ); 00407 HAL_StatusTypeDef USB_ActivateEndpoint(USB_OTG_GlobalTypeDef *USBx, USB_OTG_EPTypeDef *ep); 00408 HAL_StatusTypeDef USB_DeactivateEndpoint(USB_OTG_GlobalTypeDef *USBx, USB_OTG_EPTypeDef *ep); 00409 HAL_StatusTypeDef USB_ActivateDedicatedEndpoint(USB_OTG_GlobalTypeDef *USBx, USB_OTG_EPTypeDef *ep); 00410 HAL_StatusTypeDef USB_DeactivateDedicatedEndpoint(USB_OTG_GlobalTypeDef *USBx, USB_OTG_EPTypeDef *ep); 00411 HAL_StatusTypeDef USB_EPStartXfer(USB_OTG_GlobalTypeDef *USBx , USB_OTG_EPTypeDef *ep, uint8_t dma); 00412 HAL_StatusTypeDef USB_EP0StartXfer(USB_OTG_GlobalTypeDef *USBx , USB_OTG_EPTypeDef *ep, uint8_t dma); 00413 HAL_StatusTypeDef USB_WritePacket(USB_OTG_GlobalTypeDef *USBx, uint8_t *src, uint8_t ch_ep_num, uint16_t len, uint8_t dma); 00414 void * USB_ReadPacket(USB_OTG_GlobalTypeDef *USBx, uint8_t *dest, uint16_t len); 00415 HAL_StatusTypeDef USB_EPSetStall(USB_OTG_GlobalTypeDef *USBx , USB_OTG_EPTypeDef *ep); 00416 HAL_StatusTypeDef USB_EPClearStall(USB_OTG_GlobalTypeDef *USBx , USB_OTG_EPTypeDef *ep); 00417 HAL_StatusTypeDef USB_SetDevAddress (USB_OTG_GlobalTypeDef *USBx, uint8_t address); 00418 HAL_StatusTypeDef USB_DevConnect (USB_OTG_GlobalTypeDef *USBx); 00419 HAL_StatusTypeDef USB_DevDisconnect (USB_OTG_GlobalTypeDef *USBx); 00420 HAL_StatusTypeDef USB_StopDevice(USB_OTG_GlobalTypeDef *USBx); 00421 HAL_StatusTypeDef USB_ActivateSetup (USB_OTG_GlobalTypeDef *USBx); 00422 HAL_StatusTypeDef USB_EP0_OutStart(USB_OTG_GlobalTypeDef *USBx, uint8_t dma, uint8_t *psetup); 00423 uint8_t USB_GetDevSpeed(USB_OTG_GlobalTypeDef *USBx); 00424 uint32_t USB_GetMode(USB_OTG_GlobalTypeDef *USBx); 00425 uint32_t USB_ReadInterrupts (USB_OTG_GlobalTypeDef *USBx); 00426 uint32_t USB_ReadDevAllOutEpInterrupt (USB_OTG_GlobalTypeDef *USBx); 00427 uint32_t USB_ReadDevOutEPInterrupt (USB_OTG_GlobalTypeDef *USBx , uint8_t epnum); 00428 uint32_t USB_ReadDevAllInEpInterrupt (USB_OTG_GlobalTypeDef *USBx); 00429 uint32_t USB_ReadDevInEPInterrupt (USB_OTG_GlobalTypeDef *USBx , uint8_t epnum); 00430 void USB_ClearInterrupts (USB_OTG_GlobalTypeDef *USBx, uint32_t interrupt); 00431 00432 HAL_StatusTypeDef USB_HostInit (USB_OTG_GlobalTypeDef *USBx, USB_OTG_CfgTypeDef cfg); 00433 HAL_StatusTypeDef USB_InitFSLSPClkSel(USB_OTG_GlobalTypeDef *USBx , uint8_t freq); 00434 HAL_StatusTypeDef USB_ResetPort(USB_OTG_GlobalTypeDef *USBx); 00435 HAL_StatusTypeDef USB_DriveVbus (USB_OTG_GlobalTypeDef *USBx, uint8_t state); 00436 uint32_t USB_GetHostSpeed (USB_OTG_GlobalTypeDef *USBx); 00437 uint32_t USB_GetCurrentFrame (USB_OTG_GlobalTypeDef *USBx); 00438 HAL_StatusTypeDef USB_HC_Init(USB_OTG_GlobalTypeDef *USBx, 00439 uint8_t ch_num, 00440 uint8_t epnum, 00441 uint8_t dev_address, 00442 uint8_t speed, 00443 uint8_t ep_type, 00444 uint16_t mps); 00445 HAL_StatusTypeDef USB_HC_StartXfer(USB_OTG_GlobalTypeDef *USBx, USB_OTG_HCTypeDef *hc, uint8_t dma); 00446 uint32_t USB_HC_ReadInterrupt (USB_OTG_GlobalTypeDef *USBx); 00447 HAL_StatusTypeDef USB_HC_Halt(USB_OTG_GlobalTypeDef *USBx , uint8_t hc_num); 00448 HAL_StatusTypeDef USB_DoPing(USB_OTG_GlobalTypeDef *USBx , uint8_t ch_num); 00449 HAL_StatusTypeDef USB_StopHost(USB_OTG_GlobalTypeDef *USBx); 00450 00451 /** 00452 * @} 00453 */ 00454 00455 /** 00456 * @} 00457 */ 00458 00459 #endif /* STM32L475xx || STM32L476xx || STM32L485xx || STM32L486xx */ 00460 00461 #ifdef __cplusplus 00462 } 00463 #endif 00464 00465 00466 #endif /* __STM32L4xx_LL_USB_H */ 00467 00468 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00469
Generated on Tue Jul 12 2022 11:35:17 by
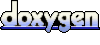