Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_ll_sdmmc.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_ll_sdmmc.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of low layer SDMMC HAL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_LL_SDMMC_H 00040 #define __STM32L4xx_LL_SDMMC_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx_hal_def.h" 00048 00049 /** @addtogroup STM32L4xx_Driver 00050 * @{ 00051 */ 00052 00053 /** @addtogroup SDMMC_LL 00054 * @{ 00055 */ 00056 00057 /* Exported types ------------------------------------------------------------*/ 00058 /** @defgroup SDMMC_LL_Exported_Types SDMMC_LL Exported Types 00059 * @{ 00060 */ 00061 00062 /** 00063 * @brief SDMMC Configuration Structure definition 00064 */ 00065 typedef struct 00066 { 00067 uint32_t ClockEdge; /*!< Specifies the clock transition on which the bit capture is made. 00068 This parameter can be a value of @ref SDMMC_LL_Clock_Edge */ 00069 00070 uint32_t ClockBypass; /*!< Specifies whether the SDMMC Clock divider bypass is 00071 enabled or disabled. 00072 This parameter can be a value of @ref SDMMC_LL_Clock_Bypass */ 00073 00074 uint32_t ClockPowerSave; /*!< Specifies whether SDMMC Clock output is enabled or 00075 disabled when the bus is idle. 00076 This parameter can be a value of @ref SDMMC_LL_Clock_Power_Save */ 00077 00078 uint32_t BusWide; /*!< Specifies the SDMMC bus width. 00079 This parameter can be a value of @ref SDMMC_LL_Bus_Wide */ 00080 00081 uint32_t HardwareFlowControl; /*!< Specifies whether the SDMMC hardware flow control is enabled or disabled. 00082 This parameter can be a value of @ref SDMMC_LL_Hardware_Flow_Control */ 00083 00084 uint32_t ClockDiv; /*!< Specifies the clock frequency of the SDMMC controller. 00085 This parameter can be a value between Min_Data = 0 and Max_Data = 255 */ 00086 00087 }SDMMC_InitTypeDef; 00088 00089 00090 /** 00091 * @brief SDMMC Command Control structure 00092 */ 00093 typedef struct 00094 { 00095 uint32_t Argument; /*!< Specifies the SDMMC command argument which is sent 00096 to a card as part of a command message. If a command 00097 contains an argument, it must be loaded into this register 00098 before writing the command to the command register. */ 00099 00100 uint32_t CmdIndex; /*!< Specifies the SDMMC command index. It must be Min_Data = 0 and 00101 Max_Data = 64 */ 00102 00103 uint32_t Response; /*!< Specifies the SDMMC response type. 00104 This parameter can be a value of @ref SDMMC_LL_Response_Type */ 00105 00106 uint32_t WaitForInterrupt; /*!< Specifies whether SDMMC wait for interrupt request is 00107 enabled or disabled. 00108 This parameter can be a value of @ref SDMMC_LL_Wait_Interrupt_State */ 00109 00110 uint32_t CPSM; /*!< Specifies whether SDMMC Command path state machine (CPSM) 00111 is enabled or disabled. 00112 This parameter can be a value of @ref SDMMC_LL_CPSM_State */ 00113 }SDMMC_CmdInitTypeDef; 00114 00115 00116 /** 00117 * @brief SDMMC Data Control structure 00118 */ 00119 typedef struct 00120 { 00121 uint32_t DataTimeOut ; /*!< Specifies the data timeout period in card bus clock periods. */ 00122 00123 uint32_t DataLength ; /*!< Specifies the number of data bytes to be transferred. */ 00124 00125 uint32_t DataBlockSize; /*!< Specifies the data block size for block transfer. 00126 This parameter can be a value of @ref SDMMC_LL_Data_Block_Size */ 00127 00128 uint32_t TransferDir; /*!< Specifies the data transfer direction, whether the transfer 00129 is a read or write. 00130 This parameter can be a value of @ref SDMMC_LL_Transfer_Direction */ 00131 00132 uint32_t TransferMode; /*!< Specifies whether data transfer is in stream or block mode. 00133 This parameter can be a value of @ref SDMMC_LL_Transfer_Type */ 00134 00135 uint32_t DPSM; /*!< Specifies whether SDMMC Data path state machine (DPSM) 00136 is enabled or disabled. 00137 This parameter can be a value of @ref SDMMC_LL_DPSM_State */ 00138 }SDMMC_DataInitTypeDef; 00139 00140 /** 00141 * @} 00142 */ 00143 00144 /* Exported constants --------------------------------------------------------*/ 00145 /** @defgroup SDMMC_LL_Exported_Constants SDMMC_LL Exported Constants 00146 * @{ 00147 */ 00148 00149 /** @defgroup SDMMC_LL_Clock_Edge Clock Edge 00150 * @{ 00151 */ 00152 #define SDMMC_CLOCK_EDGE_RISING ((uint32_t)0x00000000) 00153 #define SDMMC_CLOCK_EDGE_FALLING SDMMC_CLKCR_NEGEDGE 00154 00155 #define IS_SDMMC_CLOCK_EDGE(EDGE) (((EDGE) == SDMMC_CLOCK_EDGE_RISING) || \ 00156 ((EDGE) == SDMMC_CLOCK_EDGE_FALLING)) 00157 /** 00158 * @} 00159 */ 00160 00161 /** @defgroup SDMMC_LL_Clock_Bypass Clock Bypass 00162 * @{ 00163 */ 00164 #define SDMMC_CLOCK_BYPASS_DISABLE ((uint32_t)0x00000000) 00165 #define SDMMC_CLOCK_BYPASS_ENABLE SDMMC_CLKCR_BYPASS 00166 00167 #define IS_SDMMC_CLOCK_BYPASS(BYPASS) (((BYPASS) == SDMMC_CLOCK_BYPASS_DISABLE) || \ 00168 ((BYPASS) == SDMMC_CLOCK_BYPASS_ENABLE)) 00169 /** 00170 * @} 00171 */ 00172 00173 /** @defgroup SDMMC_LL_Clock_Power_Save Clock Power Saving 00174 * @{ 00175 */ 00176 #define SDMMC_CLOCK_POWER_SAVE_DISABLE ((uint32_t)0x00000000) 00177 #define SDMMC_CLOCK_POWER_SAVE_ENABLE SDMMC_CLKCR_PWRSAV 00178 00179 #define IS_SDMMC_CLOCK_POWER_SAVE(SAVE) (((SAVE) == SDMMC_CLOCK_POWER_SAVE_DISABLE) || \ 00180 ((SAVE) == SDMMC_CLOCK_POWER_SAVE_ENABLE)) 00181 /** 00182 * @} 00183 */ 00184 00185 /** @defgroup SDMMC_LL_Bus_Wide Bus Width 00186 * @{ 00187 */ 00188 #define SDMMC_BUS_WIDE_1B ((uint32_t)0x00000000) 00189 #define SDMMC_BUS_WIDE_4B SDMMC_CLKCR_WIDBUS_0 00190 #define SDMMC_BUS_WIDE_8B SDMMC_CLKCR_WIDBUS_1 00191 00192 #define IS_SDMMC_BUS_WIDE(WIDE) (((WIDE) == SDMMC_BUS_WIDE_1B) || \ 00193 ((WIDE) == SDMMC_BUS_WIDE_4B) || \ 00194 ((WIDE) == SDMMC_BUS_WIDE_8B)) 00195 /** 00196 * @} 00197 */ 00198 00199 /** @defgroup SDMMC_LL_Hardware_Flow_Control Hardware Flow Control 00200 * @{ 00201 */ 00202 #define SDMMC_HARDWARE_FLOW_CONTROL_DISABLE ((uint32_t)0x00000000) 00203 #define SDMMC_HARDWARE_FLOW_CONTROL_ENABLE SDMMC_CLKCR_HWFC_EN 00204 00205 #define IS_SDMMC_HARDWARE_FLOW_CONTROL(CONTROL) (((CONTROL) == SDMMC_HARDWARE_FLOW_CONTROL_DISABLE) || \ 00206 ((CONTROL) == SDMMC_HARDWARE_FLOW_CONTROL_ENABLE)) 00207 /** 00208 * @} 00209 */ 00210 00211 /** @defgroup SDMMC_LL_Clock_Division Clock Division 00212 * @{ 00213 */ 00214 #define IS_SDMMC_CLKDIV(DIV) ((DIV) <= 0xFF) 00215 /** 00216 * @} 00217 */ 00218 00219 /** @defgroup SDMMC_LL_Command_Index Command Index 00220 * @{ 00221 */ 00222 #define IS_SDMMC_CMD_INDEX(INDEX) ((INDEX) < 0x40) 00223 /** 00224 * @} 00225 */ 00226 00227 /** @defgroup SDMMC_LL_Response_Type Response Type 00228 * @{ 00229 */ 00230 #define SDMMC_RESPONSE_NO ((uint32_t)0x00000000) 00231 #define SDMMC_RESPONSE_SHORT SDMMC_CMD_WAITRESP_0 00232 #define SDMMC_RESPONSE_LONG SDMMC_CMD_WAITRESP 00233 00234 #define IS_SDMMC_RESPONSE(RESPONSE) (((RESPONSE) == SDMMC_RESPONSE_NO) || \ 00235 ((RESPONSE) == SDMMC_RESPONSE_SHORT) || \ 00236 ((RESPONSE) == SDMMC_RESPONSE_LONG)) 00237 /** 00238 * @} 00239 */ 00240 00241 /** @defgroup SDMMC_LL_Wait_Interrupt_State Wait Interrupt 00242 * @{ 00243 */ 00244 #define SDMMC_WAIT_NO ((uint32_t)0x00000000) 00245 #define SDMMC_WAIT_IT SDMMC_CMD_WAITINT 00246 #define SDMMC_WAIT_PEND SDMMC_CMD_WAITPEND 00247 00248 #define IS_SDMMC_WAIT(WAIT) (((WAIT) == SDMMC_WAIT_NO) || \ 00249 ((WAIT) == SDMMC_WAIT_IT) || \ 00250 ((WAIT) == SDMMC_WAIT_PEND)) 00251 /** 00252 * @} 00253 */ 00254 00255 /** @defgroup SDMMC_LL_CPSM_State CPSM State 00256 * @{ 00257 */ 00258 #define SDMMC_CPSM_DISABLE ((uint32_t)0x00000000) 00259 #define SDMMC_CPSM_ENABLE SDMMC_CMD_CPSMEN 00260 00261 #define IS_SDMMC_CPSM(CPSM) (((CPSM) == SDMMC_CPSM_DISABLE) || \ 00262 ((CPSM) == SDMMC_CPSM_ENABLE)) 00263 /** 00264 * @} 00265 */ 00266 00267 /** @defgroup SDMMC_LL_Response_Registers Response Register 00268 * @{ 00269 */ 00270 #define SDMMC_RESP1 ((uint32_t)0x00000000) 00271 #define SDMMC_RESP2 ((uint32_t)0x00000004) 00272 #define SDMMC_RESP3 ((uint32_t)0x00000008) 00273 #define SDMMC_RESP4 ((uint32_t)0x0000000C) 00274 00275 #define IS_SDMMC_RESP(RESP) (((RESP) == SDMMC_RESP1) || \ 00276 ((RESP) == SDMMC_RESP2) || \ 00277 ((RESP) == SDMMC_RESP3) || \ 00278 ((RESP) == SDMMC_RESP4)) 00279 /** 00280 * @} 00281 */ 00282 00283 /** @defgroup SDMMC_LL_Data_Length Data Lenght 00284 * @{ 00285 */ 00286 #define IS_SDMMC_DATA_LENGTH(LENGTH) ((LENGTH) <= 0x01FFFFFF) 00287 /** 00288 * @} 00289 */ 00290 00291 /** @defgroup SDMMC_LL_Data_Block_Size Data Block Size 00292 * @{ 00293 */ 00294 #define SDMMC_DATABLOCK_SIZE_1B ((uint32_t)0x00000000) 00295 #define SDMMC_DATABLOCK_SIZE_2B SDMMC_DCTRL_DBLOCKSIZE_0 00296 #define SDMMC_DATABLOCK_SIZE_4B SDMMC_DCTRL_DBLOCKSIZE_1 00297 #define SDMMC_DATABLOCK_SIZE_8B (SDMMC_DCTRL_DBLOCKSIZE_0|SDMMC_DCTRL_DBLOCKSIZE_1) 00298 #define SDMMC_DATABLOCK_SIZE_16B SDMMC_DCTRL_DBLOCKSIZE_2 00299 #define SDMMC_DATABLOCK_SIZE_32B (SDMMC_DCTRL_DBLOCKSIZE_0|SDMMC_DCTRL_DBLOCKSIZE_2) 00300 #define SDMMC_DATABLOCK_SIZE_64B (SDMMC_DCTRL_DBLOCKSIZE_1|SDMMC_DCTRL_DBLOCKSIZE_2) 00301 #define SDMMC_DATABLOCK_SIZE_128B (SDMMC_DCTRL_DBLOCKSIZE_0|SDMMC_DCTRL_DBLOCKSIZE_1|SDMMC_DCTRL_DBLOCKSIZE_2) 00302 #define SDMMC_DATABLOCK_SIZE_256B SDMMC_DCTRL_DBLOCKSIZE_3 00303 #define SDMMC_DATABLOCK_SIZE_512B (SDMMC_DCTRL_DBLOCKSIZE_0|SDMMC_DCTRL_DBLOCKSIZE_3) 00304 #define SDMMC_DATABLOCK_SIZE_1024B (SDMMC_DCTRL_DBLOCKSIZE_1|SDMMC_DCTRL_DBLOCKSIZE_3) 00305 #define SDMMC_DATABLOCK_SIZE_2048B (SDMMC_DCTRL_DBLOCKSIZE_0|SDMMC_DCTRL_DBLOCKSIZE_1|SDMMC_DCTRL_DBLOCKSIZE_3) 00306 #define SDMMC_DATABLOCK_SIZE_4096B (SDMMC_DCTRL_DBLOCKSIZE_2|SDMMC_DCTRL_DBLOCKSIZE_3) 00307 #define SDMMC_DATABLOCK_SIZE_8192B (SDMMC_DCTRL_DBLOCKSIZE_0|SDMMC_DCTRL_DBLOCKSIZE_2|SDMMC_DCTRL_DBLOCKSIZE_3) 00308 #define SDMMC_DATABLOCK_SIZE_16384B (SDMMC_DCTRL_DBLOCKSIZE_1|SDMMC_DCTRL_DBLOCKSIZE_2|SDMMC_DCTRL_DBLOCKSIZE_3) 00309 00310 #define IS_SDMMC_BLOCK_SIZE(SIZE) (((SIZE) == SDMMC_DATABLOCK_SIZE_1B) || \ 00311 ((SIZE) == SDMMC_DATABLOCK_SIZE_2B) || \ 00312 ((SIZE) == SDMMC_DATABLOCK_SIZE_4B) || \ 00313 ((SIZE) == SDMMC_DATABLOCK_SIZE_8B) || \ 00314 ((SIZE) == SDMMC_DATABLOCK_SIZE_16B) || \ 00315 ((SIZE) == SDMMC_DATABLOCK_SIZE_32B) || \ 00316 ((SIZE) == SDMMC_DATABLOCK_SIZE_64B) || \ 00317 ((SIZE) == SDMMC_DATABLOCK_SIZE_128B) || \ 00318 ((SIZE) == SDMMC_DATABLOCK_SIZE_256B) || \ 00319 ((SIZE) == SDMMC_DATABLOCK_SIZE_512B) || \ 00320 ((SIZE) == SDMMC_DATABLOCK_SIZE_1024B) || \ 00321 ((SIZE) == SDMMC_DATABLOCK_SIZE_2048B) || \ 00322 ((SIZE) == SDMMC_DATABLOCK_SIZE_4096B) || \ 00323 ((SIZE) == SDMMC_DATABLOCK_SIZE_8192B) || \ 00324 ((SIZE) == SDMMC_DATABLOCK_SIZE_16384B)) 00325 /** 00326 * @} 00327 */ 00328 00329 /** @defgroup SDMMC_LL_Transfer_Direction Transfer Direction 00330 * @{ 00331 */ 00332 #define SDMMC_TRANSFER_DIR_TO_CARD ((uint32_t)0x00000000) 00333 #define SDMMC_TRANSFER_DIR_TO_SDMMC SDMMC_DCTRL_DTDIR 00334 00335 #define IS_SDMMC_TRANSFER_DIR(DIR) (((DIR) == SDMMC_TRANSFER_DIR_TO_CARD) || \ 00336 ((DIR) == SDMMC_TRANSFER_DIR_TO_SDMMC)) 00337 /** 00338 * @} 00339 */ 00340 00341 /** @defgroup SDMMC_LL_Transfer_Type Transfer Type 00342 * @{ 00343 */ 00344 #define SDMMC_TRANSFER_MODE_BLOCK ((uint32_t)0x00000000) 00345 #define SDMMC_TRANSFER_MODE_STREAM SDMMC_DCTRL_DTMODE 00346 00347 #define IS_SDMMC_TRANSFER_MODE(MODE) (((MODE) == SDMMC_TRANSFER_MODE_BLOCK) || \ 00348 ((MODE) == SDMMC_TRANSFER_MODE_STREAM)) 00349 /** 00350 * @} 00351 */ 00352 00353 /** @defgroup SDMMC_LL_DPSM_State DPSM State 00354 * @{ 00355 */ 00356 #define SDMMC_DPSM_DISABLE ((uint32_t)0x00000000) 00357 #define SDMMC_DPSM_ENABLE SDMMC_DCTRL_DTEN 00358 00359 #define IS_SDMMC_DPSM(DPSM) (((DPSM) == SDMMC_DPSM_DISABLE) ||\ 00360 ((DPSM) == SDMMC_DPSM_ENABLE)) 00361 /** 00362 * @} 00363 */ 00364 00365 /** @defgroup SDMMC_LL_Read_Wait_Mode Read Wait Mode 00366 * @{ 00367 */ 00368 #define SDMMC_READ_WAIT_MODE_DATA2 ((uint32_t)0x00000000) 00369 #define SDMMC_READ_WAIT_MODE_CLK (SDMMC_DCTRL_RWMOD) 00370 00371 #define IS_SDMMC_READWAIT_MODE(MODE) (((MODE) == SDMMC_READ_WAIT_MODE_CLK) || \ 00372 ((MODE) == SDMMC_READ_WAIT_MODE_DATA2)) 00373 /** 00374 * @} 00375 */ 00376 00377 /** @defgroup SDMMC_LL_Interrupt_sources Interrupt Sources 00378 * @{ 00379 */ 00380 #define SDMMC_IT_CCRCFAIL SDMMC_STA_CCRCFAIL 00381 #define SDMMC_IT_DCRCFAIL SDMMC_STA_DCRCFAIL 00382 #define SDMMC_IT_CTIMEOUT SDMMC_STA_CTIMEOUT 00383 #define SDMMC_IT_DTIMEOUT SDMMC_STA_DTIMEOUT 00384 #define SDMMC_IT_TXUNDERR SDMMC_STA_TXUNDERR 00385 #define SDMMC_IT_RXOVERR SDMMC_STA_RXOVERR 00386 #define SDMMC_IT_CMDREND SDMMC_STA_CMDREND 00387 #define SDMMC_IT_CMDSENT SDMMC_STA_CMDSENT 00388 #define SDMMC_IT_DATAEND SDMMC_STA_DATAEND 00389 #define SDMMC_IT_DBCKEND SDMMC_STA_DBCKEND 00390 #define SDMMC_IT_CMDACT SDMMC_STA_CMDACT 00391 #define SDMMC_IT_TXACT SDMMC_STA_TXACT 00392 #define SDMMC_IT_RXACT SDMMC_STA_RXACT 00393 #define SDMMC_IT_TXFIFOHE SDMMC_STA_TXFIFOHE 00394 #define SDMMC_IT_RXFIFOHF SDMMC_STA_RXFIFOHF 00395 #define SDMMC_IT_TXFIFOF SDMMC_STA_TXFIFOF 00396 #define SDMMC_IT_RXFIFOF SDMMC_STA_RXFIFOF 00397 #define SDMMC_IT_TXFIFOE SDMMC_STA_TXFIFOE 00398 #define SDMMC_IT_RXFIFOE SDMMC_STA_RXFIFOE 00399 #define SDMMC_IT_TXDAVL SDMMC_STA_TXDAVL 00400 #define SDMMC_IT_RXDAVL SDMMC_STA_RXDAVL 00401 #define SDMMC_IT_SDIOIT SDMMC_STA_SDIOIT 00402 /** 00403 * @} 00404 */ 00405 00406 /** @defgroup SDMMC_LL_Flags Flags 00407 * @{ 00408 */ 00409 #define SDMMC_FLAG_CCRCFAIL SDMMC_STA_CCRCFAIL 00410 #define SDMMC_FLAG_DCRCFAIL SDMMC_STA_DCRCFAIL 00411 #define SDMMC_FLAG_CTIMEOUT SDMMC_STA_CTIMEOUT 00412 #define SDMMC_FLAG_DTIMEOUT SDMMC_STA_DTIMEOUT 00413 #define SDMMC_FLAG_TXUNDERR SDMMC_STA_TXUNDERR 00414 #define SDMMC_FLAG_RXOVERR SDMMC_STA_RXOVERR 00415 #define SDMMC_FLAG_CMDREND SDMMC_STA_CMDREND 00416 #define SDMMC_FLAG_CMDSENT SDMMC_STA_CMDSENT 00417 #define SDMMC_FLAG_DATAEND SDMMC_STA_DATAEND 00418 #define SDMMC_FLAG_DBCKEND SDMMC_STA_DBCKEND 00419 #define SDMMC_FLAG_CMDACT SDMMC_STA_CMDACT 00420 #define SDMMC_FLAG_TXACT SDMMC_STA_TXACT 00421 #define SDMMC_FLAG_RXACT SDMMC_STA_RXACT 00422 #define SDMMC_FLAG_TXFIFOHE SDMMC_STA_TXFIFOHE 00423 #define SDMMC_FLAG_RXFIFOHF SDMMC_STA_RXFIFOHF 00424 #define SDMMC_FLAG_TXFIFOF SDMMC_STA_TXFIFOF 00425 #define SDMMC_FLAG_RXFIFOF SDMMC_STA_RXFIFOF 00426 #define SDMMC_FLAG_TXFIFOE SDMMC_STA_TXFIFOE 00427 #define SDMMC_FLAG_RXFIFOE SDMMC_STA_RXFIFOE 00428 #define SDMMC_FLAG_TXDAVL SDMMC_STA_TXDAVL 00429 #define SDMMC_FLAG_RXDAVL SDMMC_STA_RXDAVL 00430 #define SDMMC_FLAG_SDIOIT SDMMC_STA_SDIOIT 00431 /** 00432 * @} 00433 */ 00434 00435 /** 00436 * @} 00437 */ 00438 00439 /* Exported macro ------------------------------------------------------------*/ 00440 /** @defgroup SDMMC_LL_Exported_macros SDMMC_LL Exported Macros 00441 * @{ 00442 */ 00443 00444 /** @defgroup SDMMC_LL_Register Bits And Addresses Definitions 00445 * @brief SDMMC_LL registers bit address in the alias region 00446 * @{ 00447 */ 00448 /* ---------------------- SDMMC registers bit mask --------------------------- */ 00449 /* --- CLKCR Register ---*/ 00450 /* CLKCR register clear mask */ 00451 #define CLKCR_CLEAR_MASK ((uint32_t)(SDMMC_CLKCR_CLKDIV | SDMMC_CLKCR_PWRSAV |\ 00452 SDMMC_CLKCR_BYPASS | SDMMC_CLKCR_WIDBUS |\ 00453 SDMMC_CLKCR_NEGEDGE | SDMMC_CLKCR_HWFC_EN)) 00454 00455 /* --- DCTRL Register ---*/ 00456 /* SDMMC DCTRL Clear Mask */ 00457 #define DCTRL_CLEAR_MASK ((uint32_t)(SDMMC_DCTRL_DTEN | SDMMC_DCTRL_DTDIR |\ 00458 SDMMC_DCTRL_DTMODE | SDMMC_DCTRL_DBLOCKSIZE)) 00459 00460 /* --- CMD Register ---*/ 00461 /* CMD Register clear mask */ 00462 #define CMD_CLEAR_MASK ((uint32_t)(SDMMC_CMD_CMDINDEX | SDMMC_CMD_WAITRESP |\ 00463 SDMMC_CMD_WAITINT | SDMMC_CMD_WAITPEND |\ 00464 SDMMC_CMD_CPSMEN | SDMMC_CMD_SDIOSUSPEND)) 00465 00466 /* SDMMC Intialization Frequency (400KHz max) */ 00467 #define SDMMC_INIT_CLK_DIV ((uint8_t)0x76) 00468 00469 /* SDMMC Data Transfer Frequency (25MHz max) */ 00470 #define SDMMC_TRANSFER_CLK_DIV ((uint8_t)0x0) 00471 00472 /** 00473 * @} 00474 */ 00475 00476 /** @defgroup SDMMC_LL_Interrupt_Clock Interrupt And Clock Configuration 00477 * @brief macros to handle interrupts and specific clock configurations 00478 * @{ 00479 */ 00480 00481 /** 00482 * @brief Enable the SDMMC device. 00483 * @param __INSTANCE__: SDMMC Instance 00484 * @retval None 00485 */ 00486 #define __SDMMC_ENABLE(__INSTANCE__) ((__INSTANCE__)->CLKCR |= SDMMC_CLKCR_CLKEN) 00487 00488 /** 00489 * @brief Disable the SDMMC device. 00490 * @param __INSTANCE__: SDMMC Instance 00491 * @retval None 00492 */ 00493 #define __SDMMC_DISABLE(__INSTANCE__) ((__INSTANCE__)->CLKCR &= ~SDMMC_CLKCR_CLKEN) 00494 00495 /** 00496 * @brief Enable the SDMMC DMA transfer. 00497 * @param None 00498 * @retval None 00499 */ 00500 #define __SDMMC_DMA_ENABLE(__INSTANCE__) ((__INSTANCE__)->DCTRL |= SDMMC_DCTRL_DMAEN) 00501 /** 00502 * @brief Disable the SDMMC DMA transfer. 00503 * @param None 00504 * @retval None 00505 */ 00506 #define __SDMMC_DMA_DISABLE(__INSTANCE__) ((__INSTANCE__)->DCTRL &= ~SDMMC_DCTRL_DMAEN) 00507 00508 /** 00509 * @brief Enable the SDMMC device interrupt. 00510 * @param __INSTANCE__: Pointer to SDMMC register base 00511 * @param __INTERRUPT__: specifies the SDMMC interrupt sources to be enabled. 00512 * This parameter can be one or a combination of the following values: 00513 * @arg SDMMC_IT_CCRCFAIL: Command response received (CRC check failed) interrupt 00514 * @arg SDMMC_IT_DCRCFAIL: Data block sent/received (CRC check failed) interrupt 00515 * @arg SDMMC_IT_CTIMEOUT: Command response timeout interrupt 00516 * @arg SDMMC_IT_DTIMEOUT: Data timeout interrupt 00517 * @arg SDMMC_IT_TXUNDERR: Transmit FIFO underrun error interrupt 00518 * @arg SDMMC_IT_RXOVERR: Received FIFO overrun error interrupt 00519 * @arg SDMMC_IT_CMDREND: Command response received (CRC check passed) interrupt 00520 * @arg SDMMC_IT_CMDSENT: Command sent (no response required) interrupt 00521 * @arg SDMMC_IT_DATAEND: Data end (data counter, SDIDCOUNT, is zero) interrupt 00522 * @arg SDMMC_IT_DBCKEND: Data block sent/received (CRC check passed) interrupt 00523 * @arg SDMMC_IT_CMDACT: Command transfer in progress interrupt 00524 * @arg SDMMC_IT_TXACT: Data transmit in progress interrupt 00525 * @arg SDMMC_IT_RXACT: Data receive in progress interrupt 00526 * @arg SDMMC_IT_TXFIFOHE: Transmit FIFO Half Empty interrupt 00527 * @arg SDMMC_IT_RXFIFOHF: Receive FIFO Half Full interrupt 00528 * @arg SDMMC_IT_TXFIFOF: Transmit FIFO full interrupt 00529 * @arg SDMMC_IT_RXFIFOF: Receive FIFO full interrupt 00530 * @arg SDMMC_IT_TXFIFOE: Transmit FIFO empty interrupt 00531 * @arg SDMMC_IT_RXFIFOE: Receive FIFO empty interrupt 00532 * @arg SDMMC_IT_TXDAVL: Data available in transmit FIFO interrupt 00533 * @arg SDMMC_IT_RXDAVL: Data available in receive FIFO interrupt 00534 * @arg SDMMC_IT_SDIOIT: SD I/O interrupt received interrupt 00535 * @retval None 00536 */ 00537 #define __SDMMC_ENABLE_IT(__INSTANCE__, __INTERRUPT__) ((__INSTANCE__)->MASK |= (__INTERRUPT__)) 00538 00539 /** 00540 * @brief Disable the SDMMC device interrupt. 00541 * @param __INSTANCE__: Pointer to SDMMC register base 00542 * @param __INTERRUPT__: specifies the SDMMC interrupt sources to be disabled. 00543 * This parameter can be one or a combination of the following values: 00544 * @arg SDMMC_IT_CCRCFAIL: Command response received (CRC check failed) interrupt 00545 * @arg SDMMC_IT_DCRCFAIL: Data block sent/received (CRC check failed) interrupt 00546 * @arg SDMMC_IT_CTIMEOUT: Command response timeout interrupt 00547 * @arg SDMMC_IT_DTIMEOUT: Data timeout interrupt 00548 * @arg SDMMC_IT_TXUNDERR: Transmit FIFO underrun error interrupt 00549 * @arg SDMMC_IT_RXOVERR: Received FIFO overrun error interrupt 00550 * @arg SDMMC_IT_CMDREND: Command response received (CRC check passed) interrupt 00551 * @arg SDMMC_IT_CMDSENT: Command sent (no response required) interrupt 00552 * @arg SDMMC_IT_DATAEND: Data end (data counter, SDIDCOUNT, is zero) interrupt 00553 * @arg SDMMC_IT_DBCKEND: Data block sent/received (CRC check passed) interrupt 00554 * @arg SDMMC_IT_CMDACT: Command transfer in progress interrupt 00555 * @arg SDMMC_IT_TXACT: Data transmit in progress interrupt 00556 * @arg SDMMC_IT_RXACT: Data receive in progress interrupt 00557 * @arg SDMMC_IT_TXFIFOHE: Transmit FIFO Half Empty interrupt 00558 * @arg SDMMC_IT_RXFIFOHF: Receive FIFO Half Full interrupt 00559 * @arg SDMMC_IT_TXFIFOF: Transmit FIFO full interrupt 00560 * @arg SDMMC_IT_RXFIFOF: Receive FIFO full interrupt 00561 * @arg SDMMC_IT_TXFIFOE: Transmit FIFO empty interrupt 00562 * @arg SDMMC_IT_RXFIFOE: Receive FIFO empty interrupt 00563 * @arg SDMMC_IT_TXDAVL: Data available in transmit FIFO interrupt 00564 * @arg SDMMC_IT_RXDAVL: Data available in receive FIFO interrupt 00565 * @arg SDMMC_IT_SDIOIT: SD I/O interrupt received interrupt 00566 * @retval None 00567 */ 00568 #define __SDMMC_DISABLE_IT(__INSTANCE__, __INTERRUPT__) ((__INSTANCE__)->MASK &= ~(__INTERRUPT__)) 00569 00570 /** 00571 * @brief Checks whether the specified SDMMC flag is set or not. 00572 * @param __INSTANCE__: Pointer to SDMMC register base 00573 * @param __FLAG__: specifies the flag to check. 00574 * This parameter can be one of the following values: 00575 * @arg SDMMC_FLAG_CCRCFAIL: Command response received (CRC check failed) 00576 * @arg SDMMC_FLAG_DCRCFAIL: Data block sent/received (CRC check failed) 00577 * @arg SDMMC_FLAG_CTIMEOUT: Command response timeout 00578 * @arg SDMMC_FLAG_DTIMEOUT: Data timeout 00579 * @arg SDMMC_FLAG_TXUNDERR: Transmit FIFO underrun error 00580 * @arg SDMMC_FLAG_RXOVERR: Received FIFO overrun error 00581 * @arg SDMMC_FLAG_CMDREND: Command response received (CRC check passed) 00582 * @arg SDMMC_FLAG_CMDSENT: Command sent (no response required) 00583 * @arg SDMMC_FLAG_DATAEND: Data end (data counter, SDIDCOUNT, is zero) 00584 * @arg SDMMC_FLAG_DBCKEND: Data block sent/received (CRC check passed) 00585 * @arg SDMMC_FLAG_CMDACT: Command transfer in progress 00586 * @arg SDMMC_FLAG_TXACT: Data transmit in progress 00587 * @arg SDMMC_FLAG_RXACT: Data receive in progress 00588 * @arg SDMMC_FLAG_TXFIFOHE: Transmit FIFO Half Empty 00589 * @arg SDMMC_FLAG_RXFIFOHF: Receive FIFO Half Full 00590 * @arg SDMMC_FLAG_TXFIFOF: Transmit FIFO full 00591 * @arg SDMMC_FLAG_RXFIFOF: Receive FIFO full 00592 * @arg SDMMC_FLAG_TXFIFOE: Transmit FIFO empty 00593 * @arg SDMMC_FLAG_RXFIFOE: Receive FIFO empty 00594 * @arg SDMMC_FLAG_TXDAVL: Data available in transmit FIFO 00595 * @arg SDMMC_FLAG_RXDAVL: Data available in receive FIFO 00596 * @arg SDMMC_FLAG_SDMMCIT: SD I/O interrupt received 00597 * @retval The new state of SDMMC_FLAG (SET or RESET). 00598 */ 00599 #define __SDMMC_GET_FLAG(__INSTANCE__, __FLAG__) (((__INSTANCE__)->STA &(__FLAG__)) != RESET) 00600 00601 00602 /** 00603 * @brief Clears the SDMMC pending flags. 00604 * @param __INSTANCE__: Pointer to SDMMC register base 00605 * @param __FLAG__: specifies the flag to clear. 00606 * This parameter can be one or a combination of the following values: 00607 * @arg SDMMC_FLAG_CCRCFAIL: Command response received (CRC check failed) 00608 * @arg SDMMC_FLAG_DCRCFAIL: Data block sent/received (CRC check failed) 00609 * @arg SDMMC_FLAG_CTIMEOUT: Command response timeout 00610 * @arg SDMMC_FLAG_DTIMEOUT: Data timeout 00611 * @arg SDMMC_FLAG_TXUNDERR: Transmit FIFO underrun error 00612 * @arg SDMMC_FLAG_RXOVERR: Received FIFO overrun error 00613 * @arg SDMMC_FLAG_CMDREND: Command response received (CRC check passed) 00614 * @arg SDMMC_FLAG_CMDSENT: Command sent (no response required) 00615 * @arg SDMMC_FLAG_DATAEND: Data end (data counter, SDIDCOUNT, is zero) 00616 * @arg SDMMC_FLAG_DBCKEND: Data block sent/received (CRC check passed) 00617 * @arg SDMMC_FLAG_SDMMCIT: SD I/O interrupt received 00618 * @retval None 00619 */ 00620 #define __SDMMC_CLEAR_FLAG(__INSTANCE__, __FLAG__) ((__INSTANCE__)->ICR = (__FLAG__)) 00621 00622 /** 00623 * @brief Checks whether the specified SDMMC interrupt has occurred or not. 00624 * @param __INSTANCE__: Pointer to SDMMC register base 00625 * @param __INTERRUPT__: specifies the SDMMC interrupt source to check. 00626 * This parameter can be one of the following values: 00627 * @arg SDMMC_IT_CCRCFAIL: Command response received (CRC check failed) interrupt 00628 * @arg SDMMC_IT_DCRCFAIL: Data block sent/received (CRC check failed) interrupt 00629 * @arg SDMMC_IT_CTIMEOUT: Command response timeout interrupt 00630 * @arg SDMMC_IT_DTIMEOUT: Data timeout interrupt 00631 * @arg SDMMC_IT_TXUNDERR: Transmit FIFO underrun error interrupt 00632 * @arg SDMMC_IT_RXOVERR: Received FIFO overrun error interrupt 00633 * @arg SDMMC_IT_CMDREND: Command response received (CRC check passed) interrupt 00634 * @arg SDMMC_IT_CMDSENT: Command sent (no response required) interrupt 00635 * @arg SDMMC_IT_DATAEND: Data end (data counter, SDIDCOUNT, is zero) interrupt 00636 * @arg SDMMC_IT_DBCKEND: Data block sent/received (CRC check passed) interrupt 00637 * @arg SDMMC_IT_CMDACT: Command transfer in progress interrupt 00638 * @arg SDMMC_IT_TXACT: Data transmit in progress interrupt 00639 * @arg SDMMC_IT_RXACT: Data receive in progress interrupt 00640 * @arg SDMMC_IT_TXFIFOHE: Transmit FIFO Half Empty interrupt 00641 * @arg SDMMC_IT_RXFIFOHF: Receive FIFO Half Full interrupt 00642 * @arg SDMMC_IT_TXFIFOF: Transmit FIFO full interrupt 00643 * @arg SDMMC_IT_RXFIFOF: Receive FIFO full interrupt 00644 * @arg SDMMC_IT_TXFIFOE: Transmit FIFO empty interrupt 00645 * @arg SDMMC_IT_RXFIFOE: Receive FIFO empty interrupt 00646 * @arg SDMMC_IT_TXDAVL: Data available in transmit FIFO interrupt 00647 * @arg SDMMC_IT_RXDAVL: Data available in receive FIFO interrupt 00648 * @arg SDMMC_IT_SDIOIT: SD I/O interrupt received interrupt 00649 * @retval The new state of SDMMC_IT (SET or RESET). 00650 */ 00651 #define __SDMMC_GET_IT(__INSTANCE__, __INTERRUPT__) (((__INSTANCE__)->STA &(__INTERRUPT__)) == (__INTERRUPT__)) 00652 00653 /** 00654 * @brief Clears the SDMMC's interrupt pending bits. 00655 * @param __INSTANCE__: Pointer to SDMMC register base 00656 * @param __INTERRUPT__: specifies the interrupt pending bit to clear. 00657 * This parameter can be one or a combination of the following values: 00658 * @arg SDMMC_IT_CCRCFAIL: Command response received (CRC check failed) interrupt 00659 * @arg SDMMC_IT_DCRCFAIL: Data block sent/received (CRC check failed) interrupt 00660 * @arg SDMMC_IT_CTIMEOUT: Command response timeout interrupt 00661 * @arg SDMMC_IT_DTIMEOUT: Data timeout interrupt 00662 * @arg SDMMC_IT_TXUNDERR: Transmit FIFO underrun error interrupt 00663 * @arg SDMMC_IT_RXOVERR: Received FIFO overrun error interrupt 00664 * @arg SDMMC_IT_CMDREND: Command response received (CRC check passed) interrupt 00665 * @arg SDMMC_IT_CMDSENT: Command sent (no response required) interrupt 00666 * @arg SDMMC_IT_DATAEND: Data end (data counter, SDMMC_DCOUNT, is zero) interrupt 00667 * @arg SDMMC_IT_SDIOIT: SD I/O interrupt received interrupt 00668 * @retval None 00669 */ 00670 #define __SDMMC_CLEAR_IT(__INSTANCE__, __INTERRUPT__) ((__INSTANCE__)->ICR = (__INTERRUPT__)) 00671 00672 /** 00673 * @brief Enable Start the SD I/O Read Wait operation. 00674 * @param __INSTANCE__: Pointer to SDMMC register base 00675 * @retval None 00676 */ 00677 #define __SDMMC_START_READWAIT_ENABLE(__INSTANCE__) ((__INSTANCE__)->DCTRL |= SDMMC_DCTRL_RWSTART) 00678 00679 /** 00680 * @brief Disable Start the SD I/O Read Wait operations. 00681 * @param __INSTANCE__: Pointer to SDMMC register base 00682 * @retval None 00683 */ 00684 #define __SDMMC_START_READWAIT_DISABLE(__INSTANCE__) ((__INSTANCE__)->DCTRL &= ~SDMMC_DCTRL_RWSTART) 00685 00686 /** 00687 * @brief Enable Start the SD I/O Read Wait operation. 00688 * @param __INSTANCE__: Pointer to SDMMC register base 00689 * @retval None 00690 */ 00691 #define __SDMMC_STOP_READWAIT_ENABLE(__INSTANCE__) ((__INSTANCE__)->DCTRL |= SDMMC_DCTRL_RWSTOP) 00692 00693 /** 00694 * @brief Disable Stop the SD I/O Read Wait operations. 00695 * @param __INSTANCE__: Pointer to SDMMC register base 00696 * @retval None 00697 */ 00698 #define __SDMMC_STOP_READWAIT_DISABLE(__INSTANCE__) ((__INSTANCE__)->DCTRL &= ~SDMMC_DCTRL_RWSTOP) 00699 00700 /** 00701 * @brief Enable the SD I/O Mode Operation. 00702 * @param __INSTANCE__: Pointer to SDMMC register base 00703 * @retval None 00704 */ 00705 #define __SDMMC_OPERATION_ENABLE(__INSTANCE__) ((__INSTANCE__)->DCTRL |= SDMMC_DCTRL_SDIOEN) 00706 00707 /** 00708 * @brief Disable the SD I/O Mode Operation. 00709 * @param __INSTANCE__: Pointer to SDMMC register base 00710 * @retval None 00711 */ 00712 #define __SDMMC_OPERATION_DISABLE(__INSTANCE__) ((__INSTANCE__)->DCTRL &= ~SDMMC_DCTRL_SDIOEN) 00713 00714 /** 00715 * @brief Enable the SD I/O Suspend command sending. 00716 * @param __INSTANCE__: Pointer to SDMMC register base 00717 * @retval None 00718 */ 00719 #define __SDMMC_SUSPEND_CMD_ENABLE(__INSTANCE__) ((__INSTANCE__)->CMD |= SDMMC_CMD_SDIOSUSPEND) 00720 00721 /** 00722 * @brief Disable the SD I/O Suspend command sending. 00723 * @param __INSTANCE__: Pointer to SDMMC register base 00724 * @retval None 00725 */ 00726 #define __SDMMC_SUSPEND_CMD_DISABLE(__INSTANCE__) ((__INSTANCE__)->CMD &= ~SDMMC_CMD_SDIOSUSPEND) 00727 00728 /** 00729 * @} 00730 */ 00731 00732 /** 00733 * @} 00734 */ 00735 00736 /* Exported functions --------------------------------------------------------*/ 00737 /** @addtogroup SDMMC_LL_Exported_Functions 00738 * @{ 00739 */ 00740 00741 /* Initialization/de-initialization functions **********************************/ 00742 /** @addtogroup HAL_SDMMC_LL_Group1 00743 * @{ 00744 */ 00745 HAL_StatusTypeDef SDMMC_Init(SDMMC_TypeDef *SDMMCx, SDMMC_InitTypeDef Init); 00746 /** 00747 * @} 00748 */ 00749 00750 /* I/O operation functions *****************************************************/ 00751 /** @addtogroup HAL_SDMMC_LL_Group2 00752 * @{ 00753 */ 00754 /* Blocking mode: Polling */ 00755 uint32_t SDMMC_ReadFIFO(SDMMC_TypeDef *SDMMCx); 00756 HAL_StatusTypeDef SDMMC_WriteFIFO(SDMMC_TypeDef *SDMMCx, uint32_t *pWriteData); 00757 /** 00758 * @} 00759 */ 00760 00761 /* Peripheral Control functions ************************************************/ 00762 /** @addtogroup HAL_SDMMC_LL_Group3 00763 * @{ 00764 */ 00765 HAL_StatusTypeDef SDMMC_PowerState_ON(SDMMC_TypeDef *SDMMCx); 00766 HAL_StatusTypeDef SDMMC_PowerState_OFF(SDMMC_TypeDef *SDMMCx); 00767 uint32_t SDMMC_GetPowerState(SDMMC_TypeDef *SDMMCx); 00768 00769 /* Command path state machine (CPSM) management functions */ 00770 HAL_StatusTypeDef SDMMC_SendCommand(SDMMC_TypeDef *SDMMCx, SDMMC_CmdInitTypeDef *Command); 00771 uint8_t SDMMC_GetCommandResponse(SDMMC_TypeDef *SDMMCx); 00772 uint32_t SDMMC_GetResponse(SDMMC_TypeDef *SDMMCx, uint32_t Response); 00773 00774 /* Data path state machine (DPSM) management functions */ 00775 HAL_StatusTypeDef SDMMC_DataConfig(SDMMC_TypeDef *SDMMCx, SDMMC_DataInitTypeDef* Data); 00776 uint32_t SDMMC_GetDataCounter(SDMMC_TypeDef *SDMMCx); 00777 uint32_t SDMMC_GetFIFOCount(SDMMC_TypeDef *SDMMCx); 00778 00779 /* SDMMC Cards mode management functions */ 00780 HAL_StatusTypeDef SDMMC_SetSDMMCReadWaitMode(SDMMC_TypeDef *SDMMCx, uint32_t SDMMC_ReadWaitMode); 00781 00782 /** 00783 * @} 00784 */ 00785 00786 /** 00787 * @} 00788 */ 00789 00790 /** 00791 * @} 00792 */ 00793 00794 /** 00795 * @} 00796 */ 00797 00798 #ifdef __cplusplus 00799 } 00800 #endif 00801 00802 #endif /* __STM32L4xx_LL_SDMMC_H */ 00803 00804 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00805
Generated on Tue Jul 12 2022 11:35:16 by
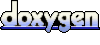