Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_ll_rng.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_ll_rng.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of RNG LL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_LL_RNG_H 00040 #define __STM32L4xx_LL_RNG_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx.h" 00048 00049 /** @addtogroup STM32L4xx_LL_Driver 00050 * @{ 00051 */ 00052 00053 #if defined (RNG) 00054 00055 /** @defgroup RNG_LL RNG 00056 * @{ 00057 */ 00058 00059 /* Private types -------------------------------------------------------------*/ 00060 /* Private variables ---------------------------------------------------------*/ 00061 00062 /* Private constants ---------------------------------------------------------*/ 00063 00064 /* Private macros ------------------------------------------------------------*/ 00065 00066 /* Exported types ------------------------------------------------------------*/ 00067 /* Exported constants --------------------------------------------------------*/ 00068 /** @defgroup RNG_LL_Exported_Constants RNG Exported Constants 00069 * @{ 00070 */ 00071 00072 /** @defgroup RNG_LL_EC_GET_FLAG Get Flags Defines 00073 * @brief Flags defines which can be used with LL_RNG_ReadReg function 00074 * @{ 00075 */ 00076 #define LL_RNG_SR_DRDY RNG_SR_DRDY 00077 #define LL_RNG_SR_CECS RNG_SR_CECS 00078 #define LL_RNG_SR_SECS RNG_SR_SECS 00079 #define LL_RNG_SR_CEIS RNG_SR_CEIS 00080 #define LL_RNG_SR_SEIS RNG_SR_SEIS 00081 /** 00082 * @} 00083 */ 00084 00085 /** @defgroup RNG_LL_EC_IT IT Defines 00086 * @brief IT defines which can be used with LL_RNG_ReadReg and LL_RNG_WriteReg macros 00087 * @{ 00088 */ 00089 #define LL_RNG_CR_IE RNG_CR_IE 00090 /** 00091 * @} 00092 */ 00093 00094 /** 00095 * @} 00096 */ 00097 00098 /* Exported macro ------------------------------------------------------------*/ 00099 /** @defgroup RNG_LL_Exported_Macros RNG Exported Macros 00100 * @{ 00101 */ 00102 00103 /** @defgroup RNG_LL_EM_WRITE_READ Common Write and read registers Macros 00104 * @{ 00105 */ 00106 00107 /** 00108 * @brief Write a value in RNG register 00109 * @param __INSTANCE__ RNG Instance 00110 * @param __REG__ Register to be written 00111 * @param __VALUE__ Value to be written in the register 00112 * @retval None 00113 */ 00114 #define LL_RNG_WriteReg(__INSTANCE__, __REG__, __VALUE__) WRITE_REG(__INSTANCE__->__REG__, (__VALUE__)) 00115 00116 /** 00117 * @brief Read a value in RNG register 00118 * @param __INSTANCE__ RNG Instance 00119 * @param __REG__ Register to be read 00120 * @retval Register value 00121 */ 00122 #define LL_RNG_ReadReg(__INSTANCE__, __REG__) READ_REG(__INSTANCE__->__REG__) 00123 /** 00124 * @} 00125 */ 00126 00127 /** 00128 * @} 00129 */ 00130 00131 /* Exported functions --------------------------------------------------------*/ 00132 /** @defgroup RNG_LL_Exported_Functions RNG Exported Functions 00133 * @{ 00134 */ 00135 /** @defgroup RNG_LL_EF_Configuration RNG Configuration functions 00136 * @{ 00137 */ 00138 00139 /** 00140 * @brief Enable Random Number Generation 00141 * @rmtoll CR RNGEN LL_RNG_Enable 00142 * @param RNGx RNG Instance 00143 * @retval None 00144 */ 00145 __STATIC_INLINE void LL_RNG_Enable(RNG_TypeDef *RNGx) 00146 { 00147 SET_BIT(RNGx->CR, RNG_CR_RNGEN); 00148 } 00149 00150 /** 00151 * @brief Disable Random Number Generation 00152 * @rmtoll CR RNGEN LL_RNG_Disable 00153 * @param RNGx RNG Instance 00154 * @retval None 00155 */ 00156 __STATIC_INLINE void LL_RNG_Disable(RNG_TypeDef *RNGx) 00157 { 00158 CLEAR_BIT(RNGx->CR, RNG_CR_RNGEN); 00159 } 00160 00161 /** 00162 * @brief Check if Random Number Generator is enabled 00163 * @rmtoll CR RNGEN LL_RNG_IsEnabled 00164 * @param RNGx RNG Instance 00165 * @retval State of bit (1 or 0). 00166 */ 00167 __STATIC_INLINE uint32_t LL_RNG_IsEnabled(RNG_TypeDef *RNGx) 00168 { 00169 return (READ_BIT(RNGx->CR, RNG_CR_RNGEN) == (RNG_CR_RNGEN)); 00170 } 00171 00172 /** 00173 * @} 00174 */ 00175 00176 /** @defgroup RNG_LL_EF_FLAG_Management FLAG_Management 00177 * @{ 00178 */ 00179 00180 /** 00181 * @brief Indicate if the RNG Data ready Flag is set or not 00182 * @rmtoll SR DRDY LL_RNG_IsActiveFlag_DRDY 00183 * @param RNGx RNG Instance 00184 * @retval State of bit (1 or 0). 00185 */ 00186 __STATIC_INLINE uint32_t LL_RNG_IsActiveFlag_DRDY(RNG_TypeDef *RNGx) 00187 { 00188 return (READ_BIT(RNGx->SR, RNG_SR_DRDY) == (RNG_SR_DRDY)); 00189 } 00190 00191 /** 00192 * @brief Indicate if the Clock Error Current Status Flag is set or not 00193 * @rmtoll SR CECS LL_RNG_IsActiveFlag_CECS 00194 * @param RNGx RNG Instance 00195 * @retval State of bit (1 or 0). 00196 */ 00197 __STATIC_INLINE uint32_t LL_RNG_IsActiveFlag_CECS(RNG_TypeDef *RNGx) 00198 { 00199 return (READ_BIT(RNGx->SR, RNG_SR_CECS) == (RNG_SR_CECS)); 00200 } 00201 00202 /** 00203 * @brief Indicate if the Seed Error Current Status Flag is set or not 00204 * @rmtoll SR SECS LL_RNG_IsActiveFlag_SECS 00205 * @param RNGx RNG Instance 00206 * @retval State of bit (1 or 0). 00207 */ 00208 __STATIC_INLINE uint32_t LL_RNG_IsActiveFlag_SECS(RNG_TypeDef *RNGx) 00209 { 00210 return (READ_BIT(RNGx->SR, RNG_SR_SECS) == (RNG_SR_SECS)); 00211 } 00212 00213 /** 00214 * @brief Indicate if the Clock Error Interrupt Status Flag is set or not 00215 * @rmtoll SR CEIS LL_RNG_IsActiveFlag_CEIS 00216 * @param RNGx RNG Instance 00217 * @retval State of bit (1 or 0). 00218 */ 00219 __STATIC_INLINE uint32_t LL_RNG_IsActiveFlag_CEIS(RNG_TypeDef *RNGx) 00220 { 00221 return (READ_BIT(RNGx->SR, RNG_SR_CEIS) == (RNG_SR_CEIS)); 00222 } 00223 00224 /** 00225 * @brief Indicate if the Seed Error Interrupt Status Flag is set or not 00226 * @rmtoll SR SEIS LL_RNG_IsActiveFlag_SEIS 00227 * @param RNGx RNG Instance 00228 * @retval State of bit (1 or 0). 00229 */ 00230 __STATIC_INLINE uint32_t LL_RNG_IsActiveFlag_SEIS(RNG_TypeDef *RNGx) 00231 { 00232 return (READ_BIT(RNGx->SR, RNG_SR_SEIS) == (RNG_SR_SEIS)); 00233 } 00234 00235 /** 00236 * @brief Clear Clock Error interrupt Status (CEIS) Flag 00237 * @rmtoll SR CEIS LL_RNG_ClearFlag_CEIS 00238 * @param RNGx RNG Instance 00239 * @retval None 00240 */ 00241 __STATIC_INLINE void LL_RNG_ClearFlag_CEIS(RNG_TypeDef *RNGx) 00242 { 00243 WRITE_REG(RNGx->SR, ~RNG_SR_CEIS); 00244 } 00245 00246 /** 00247 * @brief Clear Seed Error interrupt Status (SEIS) Flag 00248 * @rmtoll SR SEIS LL_RNG_ClearFlag_SEIS 00249 * @param RNGx RNG Instance 00250 * @retval None 00251 */ 00252 __STATIC_INLINE void LL_RNG_ClearFlag_SEIS(RNG_TypeDef *RNGx) 00253 { 00254 WRITE_REG(RNGx->SR, ~RNG_SR_SEIS); 00255 } 00256 00257 /** 00258 * @} 00259 */ 00260 00261 /** @defgroup RNG_LL_EF_IT_Management IT_Management 00262 * @{ 00263 */ 00264 00265 /** 00266 * @brief Enable Random Number Generator Interrupt 00267 * (applies for either Seed error, Clock Error or Data ready interrupts) 00268 * @rmtoll CR IE LL_RNG_EnableIT 00269 * @param RNGx RNG Instance 00270 * @retval None 00271 */ 00272 __STATIC_INLINE void LL_RNG_EnableIT(RNG_TypeDef *RNGx) 00273 { 00274 SET_BIT(RNGx->CR, RNG_CR_IE); 00275 } 00276 00277 /** 00278 * @brief Disable Random Number Generator Interrupt 00279 * (applies for either Seed error, Clock Error or Data ready interrupts) 00280 * @rmtoll CR IE LL_RNG_DisableIT 00281 * @param RNGx RNG Instance 00282 * @retval None 00283 */ 00284 __STATIC_INLINE void LL_RNG_DisableIT(RNG_TypeDef *RNGx) 00285 { 00286 CLEAR_BIT(RNGx->CR, RNG_CR_IE); 00287 } 00288 00289 /** 00290 * @brief Check if Random Number Generator Interrupt is enabled 00291 * (applies for either Seed error, Clock Error or Data ready interrupts) 00292 * @rmtoll CR IE LL_RNG_IsEnabledIT 00293 * @param RNGx RNG Instance 00294 * @retval State of bit (1 or 0). 00295 */ 00296 __STATIC_INLINE uint32_t LL_RNG_IsEnabledIT(RNG_TypeDef *RNGx) 00297 { 00298 return (READ_BIT(RNGx->CR, RNG_CR_IE) == (RNG_CR_IE)); 00299 } 00300 00301 /** 00302 * @} 00303 */ 00304 00305 /** @defgroup RNG_LL_EF_Data_Management Data_Management 00306 * @{ 00307 */ 00308 00309 /** 00310 * @brief Return32-bit Random Number value 00311 * @rmtoll DR RNDATA LL_RNG_ReadRandData32 00312 * @param RNGx RNG Instance 00313 * @retval Generated 32-bit random value 00314 */ 00315 __STATIC_INLINE uint32_t LL_RNG_ReadRandData32(RNG_TypeDef *RNGx) 00316 { 00317 return (uint32_t)(READ_REG(RNGx->DR)); 00318 } 00319 00320 /** 00321 * @} 00322 */ 00323 00324 00325 /** 00326 * @} 00327 */ 00328 00329 /** 00330 * @} 00331 */ 00332 00333 #endif /* RNG */ 00334 00335 /** 00336 * @} 00337 */ 00338 00339 #ifdef __cplusplus 00340 } 00341 #endif 00342 00343 #endif /* __STM32L4xx_LL_RNG_H */ 00344 00345 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00346
Generated on Tue Jul 12 2022 11:35:16 by
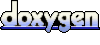