Hal Drivers for L4
Dependents: BSP OneHopeOnePrayer FINAL_AUDIO_RECORD AudioDemo
Fork of STM32L4xx_HAL_Driver by
stm32l4xx_ll_lptim.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_ll_lptim.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header file of LPTIM LL module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32L4xx_LL_LPTIM_H 00040 #define __STM32L4xx_LL_LPTIM_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32l4xx.h" 00048 00049 /** @addtogroup STM32L4xx_LL_Driver 00050 * @{ 00051 */ 00052 00053 #if defined (LPTIM1) || defined (LPTIM2) 00054 00055 /** @defgroup LPTIM_LL LPTIM 00056 * @{ 00057 */ 00058 00059 /* Private types -------------------------------------------------------------*/ 00060 /* Private variables ---------------------------------------------------------*/ 00061 00062 /* Private constants ---------------------------------------------------------*/ 00063 00064 /* Private macros ------------------------------------------------------------*/ 00065 00066 /* Exported types ------------------------------------------------------------*/ 00067 /* Exported constants --------------------------------------------------------*/ 00068 /** @defgroup LPTIM_LL_Exported_Constants LPTIM Exported Constants 00069 * @{ 00070 */ 00071 00072 /** @defgroup LPTIM_LL_EC_GET_FLAG Get Flags Defines 00073 * @brief Flags defines which can be used with LL_LPTIM_ReadReg function 00074 * @{ 00075 */ 00076 #define LL_LPTIM_ISR_CMPM LPTIM_ISR_CMPM 00077 #define LL_LPTIM_ISR_ARRM LPTIM_ISR_ARRM 00078 #define LL_LPTIM_ISR_EXTTRIG LPTIM_ISR_EXTTRIG 00079 #define LL_LPTIM_ISR_CMPOK LPTIM_ISR_CMPOK 00080 #define LL_LPTIM_ISR_ARROK LPTIM_ISR_ARROK 00081 #define LL_LPTIM_ISR_UP LPTIM_ISR_UP 00082 #define LL_LPTIM_ISR_DOWN LPTIM_ISR_DOWN 00083 /** 00084 * @} 00085 */ 00086 00087 /** @defgroup LPTIM_LL_EC_IT IT Defines 00088 * @brief IT defines which can be used with LL_LPTIM_ReadReg and LL_LPTIM_WriteReg functions 00089 * @{ 00090 */ 00091 #define LL_LPTIM_IER_CMPMIE LPTIM_IER_CMPMIE 00092 #define LL_LPTIM_IER_ARRMIE LPTIM_IER_ARRMIE 00093 #define LL_LPTIM_IER_EXTTRIGIE LPTIM_IER_EXTTRIGIE 00094 #define LL_LPTIM_IER_CMPOKIE LPTIM_IER_CMPOKIE 00095 #define LL_LPTIM_IER_ARROKIE LPTIM_IER_ARROKIE 00096 #define LL_LPTIM_IER_UPIE LPTIM_IER_UPIE 00097 #define LL_LPTIM_IER_DOWNIE LPTIM_IER_DOWNIE 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup LPTIM_LL_EC_OPERATING_MODE OPERATING MODE 00103 * @{ 00104 */ 00105 #define LL_LPTIM_OPERATING_MODE_CONTINUOUS LPTIM_CR_CNTSTRT /*!<LP Timer starts in continuous mode*/ 00106 #define LL_LPTIM_OPERATING_MODE_ONESHOT LPTIM_CR_SNGSTRT /*!<LP Tilmer starts in single mode*/ 00107 /** 00108 * @} 00109 */ 00110 00111 /** @defgroup LPTIM_LL_EC_UPDATE_MODE UPDATE MODE 00112 * @{ 00113 */ 00114 #define LL_LPTIM_UPDATE_MODE_IMMEDIATE ((uint32_t)0x00000000)/*!<Preload is disabled: registers are updated after each APB bus write access*/ 00115 #define LL_LPTIM_UPDATE_MODE_ENDOFPERIOD LPTIM_CFGR_PRELOAD/*!<preload is enabled: registers are updated at the end of the current LPTIM period*/ 00116 /** 00117 * @} 00118 */ 00119 00120 /** @defgroup LPTIM_LL_EC_COUNTER_MODE COUNTER MODE 00121 * @{ 00122 */ 00123 #define LL_LPTIM_COUNTER_MODE_INTERNAL ((uint32_t)0x00000000) /*!<The counter is incremented following each internal clock pulse*/ 00124 #define LL_LPTIM_COUNTER_MODE_EXTERNAL LPTIM_CFGR_COUNTMODE /*!<The counter is incremented following each valid clock pulse on the LPTIM external Input1*/ 00125 /** 00126 * @} 00127 */ 00128 00129 /** @defgroup LPTIM_LL_EC_OUTPUT_WAVEFORM OUTPUT WAVEFORM 00130 * @{ 00131 */ 00132 #define LL_LPTIM_OUTPUT_WAVEFORM_PWM ((uint32_t)0x00000000) /*!<LPTIM generates either a PWM waveform or a One pulse waveform depending on chosen operating mode CONTINOUS or SINGLE*/ 00133 #define LL_LPTIM_OUTPUT_WAVEFORM_SETONCE LPTIM_CFGR_WAVE/*!<LPTIM generates a Set Once waveform*/ 00134 /** 00135 * @} 00136 */ 00137 00138 /** @defgroup LPTIM_LL_EC_OUTPUT_POLARITY OUTPUT POLARITY 00139 * @{ 00140 */ 00141 #define LL_LPTIM_OUTPUT_POLARITY_REGULAR ((uint32_t) 0x00000000) /*!<The LPTIM output reflects the compare results between LPTIMx_ARR and LPTIMx_CMP registers*/ 00142 #define LL_LPTIM_OUTPUT_POLARITY_INVERSE LPTIM_CFGR_WAVPOL /*!<The LPTIM output reflects the inverse of the compare results between LPTIMx_ARR and LPTIMx_CMP registers*/ 00143 /** 00144 * @} 00145 */ 00146 00147 /** @defgroup LPTIM_LL_EC_PRESCALER PRESCALER 00148 * @{ 00149 */ 00150 #define LL_LPTIM_PRESCALER_DIV1 ((uint32_t)0x000000) /*!<Prescaler division factor is set to 1*/ 00151 #define LL_LPTIM_PRESCALER_DIV2 LPTIM_CFGR_PRESC_0 /*!<Prescaler division factor is set to 2*/ 00152 #define LL_LPTIM_PRESCALER_DIV4 LPTIM_CFGR_PRESC_1 /*!<Prescaler division factor is set to 4*/ 00153 #define LL_LPTIM_PRESCALER_DIV8 (LPTIM_CFGR_PRESC_1 | LPTIM_CFGR_PRESC_0) /*!<Prescaler division factor is set to 8*/ 00154 #define LL_LPTIM_PRESCALER_DIV16 LPTIM_CFGR_PRESC_2 /*!<Prescaler division factor is set to 16*/ 00155 #define LL_LPTIM_PRESCALER_DIV32 (LPTIM_CFGR_PRESC_2 | LPTIM_CFGR_PRESC_0) /*!<Prescaler division factor is set to 32*/ 00156 #define LL_LPTIM_PRESCALER_DIV64 (LPTIM_CFGR_PRESC_2 | LPTIM_CFGR_PRESC_1) /*!<Prescaler division factor is set to 64*/ 00157 #define LL_LPTIM_PRESCALER_DIV128 LPTIM_CFGR_PRESC /*!<Prescaler division factor is set to 128*/ 00158 /** 00159 * @} 00160 */ 00161 00162 /** @defgroup LPTIM_LL_EC_TRIG_SOURCE TRIG SOURCE 00163 * @{ 00164 */ 00165 #define LL_LPTIM_TRIG_SOURCE_GPIO ((uint32_t)0x00000000) /*!<External input trigger is connected to TIMx_ETR input*/ 00166 #define LL_LPTIM_TRIG_SOURCE_RTCALARMA LPTIM_CFGR_TRIGSEL_0 /*!<External input trigger is connected to RTC Alarm A*/ 00167 #define LL_LPTIM_TRIG_SOURCE_RTCALARMB LPTIM_CFGR_TRIGSEL_1 /*!<External input trigger is connected to RTC Alarm B*/ 00168 #define LL_LPTIM_TRIG_SOURCE_RTCTAMP1 (LPTIM_CFGR_TRIGSEL_1 | LPTIM_CFGR_TRIGSEL_0) /*!<External input trigger is connected to RTC Tamper 1*/ 00169 #define LL_LPTIM_TRIG_SOURCE_RTCTAMP2 LPTIM_CFGR_TRIGSEL_2 /*!<External input trigger is connected to RTC Tamper 2*/ 00170 #define LL_LPTIM_TRIG_SOURCE_RTCTAMP3 (LPTIM_CFGR_TRIGSEL_2 | LPTIM_CFGR_TRIGSEL_0) /*!<External input trigger is connected to RTC Tamper 3*/ 00171 #define LL_LPTIM_TRIG_SOURCE_COMP1 (LPTIM_CFGR_TRIGSEL_2 | LPTIM_CFGR_TRIGSEL_1) /*!<External input trigger is connected to COMP1 output*/ 00172 #define LL_LPTIM_TRIG_SOURCE_COMP2 LPTIM_CFGR_TRIGSEL /*!<External input trigger is connected to COMP2 output*/ 00173 /** 00174 * @} 00175 */ 00176 00177 /** @defgroup LPTIM_LL_EC_TRIG_FILTER TRIG FILTER 00178 * @{ 00179 */ 00180 #define LL_LPTIM_TRIG_FILTER_NONE ((uint32_t)0x00000000) /*!<Any trigger active level change is considered as a valid trigger*/ 00181 #define LL_LPTIM_TRIG_FILTER_2 LPTIM_CFGR_TRGFLT_0 /*!<Trigger active level change must be stable for at least 2 clock periods before it is considered as valid trigger*/ 00182 #define LL_LPTIM_TRIG_FILTER_4 LPTIM_CFGR_TRGFLT_1 /*!<Trigger active level change must be stable for at least 4 clock periods before it is considered as valid trigger*/ 00183 #define LL_LPTIM_TRIG_FILTER_8 LPTIM_CFGR_TRGFLT /*!<Trigger active level change must be stable for at least 8 clock periods before it is considered as valid trigger*/ 00184 /** 00185 * @} 00186 */ 00187 00188 /** @defgroup LPTIM_LL_EC_TRIG_POLARITY TRIG POLARITY 00189 * @{ 00190 */ 00191 #define LL_LPTIM_TRIG_POLARITY_RISING LPTIM_CFGR_TRIGEN_0 /*!<LPTIM counter starts when a rising edge is detected*/ 00192 #define LL_LPTIM_TRIG_POLARITY_FALLING LPTIM_CFGR_TRIGEN_1 /*!<LPTIM counter starts when a falling edge is detected*/ 00193 #define LL_LPTIM_TRIG_POLARITY_RISING_FALLING LPTIM_CFGR_TRIGEN /*!<LPTIM counter starts when a rising or a falling edge is detected*/ 00194 /** 00195 * @} 00196 */ 00197 00198 /** @defgroup LPTIM_LL_EC_CLK_SOURCE CLK SOURCE 00199 * @{ 00200 */ 00201 #define LL_LPTIM_CLK_SOURCE_INTERNAL ((uint32_t)0x00000000) /*!<LPTIM is clocked by internal clock source (APB clock or any of the embedded oscillators)*/ 00202 #define LL_LPTIM_CLK_SOURCE_EXTERNAL LPTIM_CFGR_CKSEL /*!<LPTIM is clocked by an external clock source through the LPTIM external Input1*/ 00203 /** 00204 * @} 00205 */ 00206 00207 /** @defgroup LPTIM_LL_EC_CLK_FILTER CLK FILTER 00208 * @{ 00209 */ 00210 #define LL_LPTIM_CLK_FILTER_NONE ((uint32_t)0x00000000) /*!<Any external clock signal level change is considered as a valid transition*/ 00211 #define LL_LPTIM_CLK_FILTER_2 LPTIM_CFGR_CKFLT_0 /*!<External clock signal level change must be stable for at least 2 clock periods before it is considered as valid transition*/ 00212 #define LL_LPTIM_CLK_FILTER_4 LPTIM_CFGR_CKFLT_1 /*!<External clock signal level change must be stable for at least 4 clock periods before it is considered as valid transition*/ 00213 #define LL_LPTIM_CLK_FILTER_8 LPTIM_CFGR_CKFLT /*!<External clock signal level change must be stable for at least 8 clock periods before it is considered as valid transition*/ 00214 /** 00215 * @} 00216 */ 00217 00218 /** @defgroup LPTIM_LL_EC_CLK_POLARITY CLK POLARITY 00219 * @{ 00220 */ 00221 #define LL_LPTIM_CLK_POLARITY_RISING ((uint32_t)0x00000000) /*!< The rising edge is the active edge used for counting*/ 00222 #define LL_LPTIM_CLK_POLARITY_FALLING LPTIM_CFGR_CKPOL_0 /*!< The falling edge is the active edge used for counting*/ 00223 #define LL_LPTIM_CLK_POLARITY_RISING_FALLING LPTIM_CFGR_CKPOL_1 /*!< Both edges are active edges*/ 00224 /** 00225 * @} 00226 */ 00227 00228 /** @defgroup LPTIM_LL_EC_ENCODER_MODE ENCODER MODE 00229 * @{ 00230 */ 00231 #define LL_LPTIM_ENCODER_MODE_RISING ((uint32_t)0x00000000) /*!< The rising edge is the active edge used for counting*/ 00232 #define LL_LPTIM_ENCODER_MODE_FALLING LPTIM_CFGR_CKPOL_0 /*!< The falling edge is the active edge used for counting*/ 00233 #define LL_LPTIM_ENCODER_MODE_RISING_FALLING LPTIM_CFGR_CKPOL_1 /*!< Both edges are active edges*/ 00234 /** 00235 * @} 00236 */ 00237 00238 /** @defgroup LPTIM_EC_INPUT1_SRC INPUT1 SOURCE 00239 * @{ 00240 */ 00241 00242 #define LL_LPTIM_INPUT1_SRC_GPIO ((uint32_t)0x00000000) /*!< For LPTIM1 and LPTIM2 */ 00243 #define LL_LPTIM_INPUT1_SRC_COMP1 LPTIM_OR_OR_0 /*!< For LPTIM1 and LPTIM2 */ 00244 #define LL_LPTIM_INPUT1_SRC_COMP2 LPTIM_OR_OR_1 /*!< For LPTIM2 */ 00245 #define LL_LPTIM_INPUT1_SRC_COMP1_COMP2 LPTIM_OR_OR /*!< For LPTIM2 */ 00246 /** 00247 * @} 00248 */ 00249 00250 /** @defgroup LPTIM_EC_INPUT2_SRC INPUT2 SOURCE 00251 * @{ 00252 */ 00253 00254 #define LL_LPTIM_INPUT2_SRC_GPIO ((uint32_t)0x00000000) /*!< For LPTIM1 */ 00255 #define LL_LPTIM_INPUT2_SRC_COMP2 LPTIM_OR_OR_1 /*!< For LPTIM1 */ 00256 /** 00257 * @} 00258 */ 00259 00260 /** 00261 * @} 00262 */ 00263 00264 /* Exported macro ------------------------------------------------------------*/ 00265 /** @defgroup LPTIM_LL_Exported_Macros LPTIM Exported Macros 00266 * @{ 00267 */ 00268 00269 /** @defgroup LPTIM_LL_EM_WRITE_READ Common Write and read registers Macros 00270 * @{ 00271 */ 00272 00273 /** 00274 * @brief Write a value in LPTIM register 00275 * @param __INSTANCE__ LPTIM Instance 00276 * @param __REG__ Register to be written 00277 * @param __VALUE__ Value to be written in the register 00278 * @retval None 00279 */ 00280 #define LL_LPTIM_WriteReg(__INSTANCE__, __REG__, __VALUE__) WRITE_REG(__INSTANCE__->__REG__, (__VALUE__)) 00281 00282 /** 00283 * @brief Read a value in LPTIM register 00284 * @param __INSTANCE__ LPTIM Instance 00285 * @param __REG__ Register to be read 00286 * @retval Register value 00287 */ 00288 #define LL_LPTIM_ReadReg(__INSTANCE__, __REG__) READ_REG(__INSTANCE__->__REG__) 00289 /** 00290 * @} 00291 */ 00292 00293 /** 00294 * @} 00295 */ 00296 00297 00298 /* Exported functions --------------------------------------------------------*/ 00299 /** @defgroup LPTIM_LL_Exported_Functions LPTIM Exported Functions 00300 * @{ 00301 */ 00302 00303 /** @defgroup LPTIM_LL_EF_LPTIM_Configuration LPTIM_Configuration 00304 * @{ 00305 */ 00306 00307 /** 00308 * @brief Enable the LPTIM instance 00309 * @note After setting the ENABLE bit, a delay of two counter clock is needed 00310 * before the LPTIM instance is actually enabled. 00311 * @rmtoll CR ENABLE LL_LPTIM_Enable 00312 * @param LPTIMx Low-Power Timer instance 00313 * @retval None 00314 */ 00315 __STATIC_INLINE void LL_LPTIM_Enable(LPTIM_TypeDef * LPTIMx) 00316 { 00317 SET_BIT(LPTIMx->CR, LPTIM_CR_ENABLE); 00318 } 00319 00320 /** 00321 * @brief Disable the LPTIM instance 00322 * @rmtoll CR ENABLE LL_LPTIM_Disable 00323 * @param LPTIMx Low-Power Timer instance 00324 * @retval None 00325 */ 00326 __STATIC_INLINE void LL_LPTIM_Disable(LPTIM_TypeDef * LPTIMx) 00327 { 00328 CLEAR_BIT(LPTIMx->CR, LPTIM_CR_ENABLE); 00329 } 00330 00331 /** 00332 * @brief Starts the LPTIM counter in the desired mode. 00333 * @note LPTIM instance must be enabled before starting the counter. 00334 * @note It is possible to change on the fly from One Shot mode to 00335 * Continuous mode. 00336 * @rmtoll CR CNTSTRT LL_LPTIM_StartCounter\n 00337 * CR SNGSTRT LL_LPTIM_StartCounter 00338 * @param LPTIMx Low-Power Timer instance 00339 * @param OperatingMode This parameter can be one of the following values: 00340 * @arg @ref LL_LPTIM_OPERATING_MODE_CONTINUOUS 00341 * @arg @ref LL_LPTIM_OPERATING_MODE_ONESHOT 00342 * @retval None 00343 */ 00344 __STATIC_INLINE void LL_LPTIM_StartCounter(LPTIM_TypeDef * LPTIMx, uint32_t OperatingMode) 00345 { 00346 MODIFY_REG(LPTIMx->CR, LPTIM_CR_CNTSTRT | LPTIM_CR_SNGSTRT, OperatingMode); 00347 } 00348 00349 /** 00350 * @brief Set the LPTIM registers update mode (enable/disable register preload) 00351 * @note This function must be called when the LPTIM instance is disabled. 00352 * @rmtoll CFGR PRELOAD LL_LPTIM_SetUpdateMode 00353 * @param LPTIMx Low-Power Timer instance 00354 * @param UpdateMode This parameter can be one of the following values: 00355 * @arg @ref LL_LPTIM_UPDATE_MODE_IMMEDIATE 00356 * @arg @ref LL_LPTIM_UPDATE_MODE_ENDOFPERIOD 00357 * @retval None 00358 */ 00359 __STATIC_INLINE void LL_LPTIM_SetUpdateMode(LPTIM_TypeDef * LPTIMx, uint32_t UpdateMode) 00360 { 00361 MODIFY_REG(LPTIMx->CFGR, LPTIM_CFGR_PRELOAD, UpdateMode); 00362 } 00363 00364 /** 00365 * @brief Get the LPTIM registers update mode 00366 * @rmtoll CFGR PRELOAD LL_LPTIM_GetUpdateMode 00367 * @param LPTIMx Low-Power Timer instance 00368 * @retval Returned value can be one of the following values: 00369 * @arg @ref LL_LPTIM_UPDATE_MODE_IMMEDIATE 00370 * @arg @ref LL_LPTIM_UPDATE_MODE_ENDOFPERIOD 00371 */ 00372 __STATIC_INLINE uint32_t LL_LPTIM_GetUpdateMode(LPTIM_TypeDef * LPTIMx) 00373 { 00374 return (uint32_t)(READ_BIT(LPTIMx->CFGR, LPTIM_CFGR_PRELOAD)); 00375 } 00376 00377 /** 00378 * @brief Set the auto reload value 00379 * @note The LPTIMx_ARR register content must only be modified when the LPTIM is enabled 00380 * @note After a write to the LPTIMx_ARR register a new write operation to the 00381 * same register can only be performed when the previous write operation 00382 * is completed. Any successive write before the ARROK flag be set, will 00383 * lead to unpredictable results. 00384 * @note autoreload value be strictly greater than the compare value. 00385 * @rmtoll ARR ARR LL_LPTIM_SetAutoReload 00386 * @param LPTIMx Low-Power Timer instance 00387 * @param AutoReload Value between 0 and 0xFFFF 00388 * @retval None 00389 */ 00390 __STATIC_INLINE void LL_LPTIM_SetAutoReload(LPTIM_TypeDef * LPTIMx, uint32_t AutoReload) 00391 { 00392 MODIFY_REG(LPTIMx->ARR, LPTIM_ARR_ARR, AutoReload); 00393 } 00394 00395 /** 00396 * @brief Get actual auto reload value 00397 * @rmtoll ARR ARR LL_LPTIM_GetAutoReload 00398 * @param LPTIMx Low-Power Timer instance 00399 * @retval AutoReload Value between 0 and 0xFFFF 00400 */ 00401 __STATIC_INLINE uint32_t LL_LPTIM_GetAutoReload(LPTIM_TypeDef * LPTIMx) 00402 { 00403 return (uint32_t)(READ_BIT(LPTIMx->ARR, LPTIM_ARR_ARR)); 00404 } 00405 00406 /** 00407 * @brief Set the compare value 00408 * @note After a write to the LPTIMx_CMP register a new write operation to the 00409 * same register can only be performed when the previous write operation 00410 * is completed. Any successive write before the CMPOK flag be set, will 00411 * lead to unpredictable results. 00412 * @rmtoll CMP CMP LL_LPTIM_SetCompare 00413 * @param LPTIMx Low-Power Timer instance 00414 * @param CompareValue Value between 0 and 0xFFFF 00415 * @retval None 00416 */ 00417 __STATIC_INLINE void LL_LPTIM_SetCompare(LPTIM_TypeDef * LPTIMx, uint32_t CompareValue) 00418 { 00419 MODIFY_REG(LPTIMx->CMP, LPTIM_CMP_CMP, CompareValue); 00420 } 00421 00422 /** 00423 * @brief Get actual compare value 00424 * @rmtoll CMP CMP LL_LPTIM_GetCompare 00425 * @param LPTIMx Low-Power Timer instance 00426 * @retval CompareValue Value between 0 and 0xFFFF 00427 */ 00428 __STATIC_INLINE uint32_t LL_LPTIM_GetCompare(LPTIM_TypeDef * LPTIMx) 00429 { 00430 return (uint32_t)(READ_BIT(LPTIMx->CMP, LPTIM_CMP_CMP)); 00431 } 00432 00433 /** 00434 * @brief Get actual counter value 00435 * @note When the LPTIM instance is running with an asynchronous clock, reading 00436 * the LPTIMx_CNT register may return unreliable values. So in this case 00437 * it is necessary to perform two consecutive read accesses and verify 00438 * that the two returned values are identical. 00439 * @rmtoll CNT CNT LL_LPTIM_GetCounter 00440 * @param LPTIMx Low-Power Timer instance 00441 * @retval Counter value 00442 */ 00443 __STATIC_INLINE uint32_t LL_LPTIM_GetCounter(LPTIM_TypeDef * LPTIMx) 00444 { 00445 return (uint32_t)(READ_BIT(LPTIMx->CNT, LPTIM_CNT_CNT)); 00446 } 00447 00448 /** 00449 * @brief Set the counter mode (selection of the LPTIM counter clock source). 00450 * @note The counter mode can be set only when the LPTIM instance is disabled. 00451 * @rmtoll CFGR COUNTMODE LL_LPTIM_SetCounterMode 00452 * @param LPTIMx Low-Power Timer instance 00453 * @param CounterMode This parameter can be one of the following values: 00454 * @arg @ref LL_LPTIM_COUNTER_MODE_INTERNAL 00455 * @arg @ref LL_LPTIM_COUNTER_MODE_EXTERNAL 00456 * @retval None 00457 */ 00458 __STATIC_INLINE void LL_LPTIM_SetCounterMode(LPTIM_TypeDef * LPTIMx, uint32_t CounterMode) 00459 { 00460 MODIFY_REG(LPTIMx->CFGR, LPTIM_CFGR_COUNTMODE, CounterMode); 00461 } 00462 00463 /** 00464 * @brief Get the counter mode 00465 * @rmtoll CFGR COUNTMODE LL_LPTIM_GetCounterMode 00466 * @param LPTIMx Low-Power Timer instance 00467 * @retval Returned value can be one of the following values: 00468 * @arg @ref LL_LPTIM_COUNTER_MODE_INTERNAL 00469 * @arg @ref LL_LPTIM_COUNTER_MODE_EXTERNAL 00470 */ 00471 __STATIC_INLINE uint32_t LL_LPTIM_GetCounterMode(LPTIM_TypeDef * LPTIMx) 00472 { 00473 return (uint32_t)(READ_BIT(LPTIMx->CFGR, LPTIM_CFGR_COUNTMODE)); 00474 } 00475 00476 /** 00477 * @brief Configure the LPTIM instance output (LPTIMx_OUT) 00478 * @note This function must be called when the LPTIM instance is disabled. 00479 * @note Regarding the LPTIM output polarity the change takes effect 00480 * immediately, so the output default value will change immediately after 00481 * the polarity is re-configured, even before the timer is enabled. 00482 * @rmtoll CFGR WAVE LL_LPTIM_ConfigOutput\n 00483 * CFGR WAVPOL LL_LPTIM_ConfigOutput 00484 * @param LPTIMx Low-Power Timer instance 00485 * @param Waveform This parameter can be one of the following values: 00486 * @arg @ref LL_LPTIM_OUTPUT_WAVEFORM_PWM 00487 * @arg @ref LL_LPTIM_OUTPUT_WAVEFORM_SETONCE 00488 * @param Polarity This parameter can be one of the following values: 00489 * @arg @ref LL_LPTIM_OUTPUT_POLARITY_REGULAR 00490 * @arg @ref LL_LPTIM_OUTPUT_POLARITY_INVERSE 00491 * @retval None 00492 */ 00493 __STATIC_INLINE void LL_LPTIM_ConfigOutput(LPTIM_TypeDef * LPTIMx, uint32_t Waveform, uint32_t Polarity) 00494 { 00495 MODIFY_REG(LPTIMx->CFGR, LPTIM_CFGR_WAVE | LPTIM_CFGR_WAVPOL, Waveform | Polarity); 00496 } 00497 00498 /** 00499 * @brief Get actual waveform shape 00500 * @rmtoll CFGR WAVE LL_LPTIM_GetWaveform 00501 * @param LPTIMx Low-Power Timer instance 00502 * @retval Returned value can be one of the following values: 00503 * @arg @ref LL_LPTIM_OUTPUT_WAVEFORM_PWM 00504 * @arg @ref LL_LPTIM_OUTPUT_WAVEFORM_SETONCE 00505 */ 00506 __STATIC_INLINE uint32_t LL_LPTIM_GetWaveform(LPTIM_TypeDef * LPTIMx) 00507 { 00508 return (uint32_t)(READ_BIT(LPTIMx->CFGR, LPTIM_CFGR_WAVE)); 00509 } 00510 00511 /** 00512 * @brief Get actual output polarity 00513 * @rmtoll CFGR WAVPOL LL_LPTIM_GetPolarity 00514 * @param LPTIMx Low-Power Timer instance 00515 * @retval Returned value can be one of the following values: 00516 * @arg @ref LL_LPTIM_OUTPUT_POLARITY_REGULAR 00517 * @arg @ref LL_LPTIM_OUTPUT_POLARITY_INVERSE 00518 */ 00519 __STATIC_INLINE uint32_t LL_LPTIM_GetPolarity(LPTIM_TypeDef * LPTIMx) 00520 { 00521 return (uint32_t)(READ_BIT(LPTIMx->CFGR, LPTIM_CFGR_WAVPOL)); 00522 } 00523 00524 /** 00525 * @brief Set actual prescaler division ratio. 00526 * @note This function must be called when the LPTIM instance is disabled. 00527 * @note When the LPTIM is configured to be clocked by an internal clock source 00528 * and the LPTIM counter is configured to be updated by active edges 00529 * detected on the LPTIM external Input1, the internal clock provided to 00530 * the LPTIM must be not be prescaled. 00531 * @rmtoll CFGR PRESC LL_LPTIM_SetPrescaler 00532 * @param LPTIMx Low-Power Timer instance 00533 * @param Prescaler This parameter can be one of the following values: 00534 * @arg @ref LL_LPTIM_PRESCALER_DIV1 00535 * @arg @ref LL_LPTIM_PRESCALER_DIV2 00536 * @arg @ref LL_LPTIM_PRESCALER_DIV4 00537 * @arg @ref LL_LPTIM_PRESCALER_DIV8 00538 * @arg @ref LL_LPTIM_PRESCALER_DIV16 00539 * @arg @ref LL_LPTIM_PRESCALER_DIV32 00540 * @arg @ref LL_LPTIM_PRESCALER_DIV64 00541 * @arg @ref LL_LPTIM_PRESCALER_DIV128 00542 * @retval None 00543 */ 00544 __STATIC_INLINE void LL_LPTIM_SetPrescaler(LPTIM_TypeDef * LPTIMx, uint32_t Prescaler) 00545 { 00546 MODIFY_REG(LPTIMx->CFGR, LPTIM_CFGR_PRESC, Prescaler); 00547 } 00548 00549 /** 00550 * @brief Get actual prescaler division ratio. 00551 * @rmtoll CFGR PRESC LL_LPTIM_GetPrescaler 00552 * @param LPTIMx Low-Power Timer instance 00553 * @retval Returned value can be one of the following values: 00554 * @arg @ref LL_LPTIM_PRESCALER_DIV1 00555 * @arg @ref LL_LPTIM_PRESCALER_DIV2 00556 * @arg @ref LL_LPTIM_PRESCALER_DIV4 00557 * @arg @ref LL_LPTIM_PRESCALER_DIV8 00558 * @arg @ref LL_LPTIM_PRESCALER_DIV16 00559 * @arg @ref LL_LPTIM_PRESCALER_DIV32 00560 * @arg @ref LL_LPTIM_PRESCALER_DIV64 00561 * @arg @ref LL_LPTIM_PRESCALER_DIV128 00562 */ 00563 __STATIC_INLINE uint32_t LL_LPTIM_GetPrescaler(LPTIM_TypeDef * LPTIMx) 00564 { 00565 return (uint32_t)(READ_BIT(LPTIMx->CFGR, LPTIM_CFGR_PRESC)); 00566 } 00567 00568 /** 00569 * @brief Set LPTIM input 1 source (default GPIO). 00570 * @rmtoll OR OR_0 LL_LPTIM_SetInput1Src 00571 * @rmtoll OR OR_1 LL_LPTIM_SetInput1Src 00572 * @param LPTIMx Low-Power Timer instance 00573 * @param Src This parameter can be one of the following values: 00574 * @arg @ref LL_LPTIM_INPUT1_SRC_GPIO 00575 * @arg @ref LL_LPTIM_INPUT1_SRC_COMP1 00576 * @arg @ref LL_LPTIM_INPUT1_SRC_COMP2 00577 * @arg @ref LL_LPTIM_INPUT1_SRC_COMP1_COMP2 00578 * @retval None 00579 */ 00580 __STATIC_INLINE void LL_LPTIM_SetInput1Src(LPTIM_TypeDef * LPTIMx, uint32_t Src) 00581 { 00582 WRITE_REG(LPTIMx->OR, Src); 00583 } 00584 00585 /** 00586 * @brief Set LPTIM input 2 source (default GPIO). 00587 * @rmtoll OR OR_0 LL_LPTIM_SetInput2Src 00588 * @param LPTIMx Low-Power Timer instance 00589 * @param Src This parameter can be one of the following values: 00590 * @arg @ref LL_LPTIM_INPUT2_SRC_GPIO 00591 * @arg @ref LL_LPTIM_INPUT2_SRC_COMP2 00592 * @retval None 00593 */ 00594 __STATIC_INLINE void LL_LPTIM_SetInput2Src(LPTIM_TypeDef * LPTIMx, uint32_t Src) 00595 { 00596 WRITE_REG(LPTIMx->OR, Src); 00597 } 00598 00599 /** 00600 * @} 00601 */ 00602 00603 /** @defgroup LPTIM_LL_EF_Trigger_Configuration Trigger_Configuration 00604 * @{ 00605 */ 00606 00607 /** 00608 * @brief Enable the timeout function 00609 * @note This function must be called when the LPTIM instance is disabled. 00610 * @note The first trigger event will start the timer, any successive trigger 00611 * event will reset the counter and the timer will restart. 00612 * @note The timeout value corresponds to the compare value; if no trigger 00613 * occurs within the expected time frame, the MCU is waked-up by the 00614 * compare match event. 00615 * @rmtoll CFGR TIMOUT LL_LPTIM_EnableTimeout 00616 * @param LPTIMx Low-Power Timer instance 00617 * @retval None 00618 */ 00619 __STATIC_INLINE void LL_LPTIM_EnableTimeout(LPTIM_TypeDef * LPTIMx) 00620 { 00621 SET_BIT(LPTIMx->CFGR, LPTIM_CFGR_TIMOUT); 00622 } 00623 00624 /** 00625 * @brief Disable the timeout function 00626 * @note This function must be called when the LPTIM instance is disabled. 00627 * @note A trigger event arriving when the timer is already started will be 00628 * ignored. 00629 * @rmtoll CFGR TIMOUT LL_LPTIM_DisableTimeout 00630 * @param LPTIMx Low-Power Timer instance 00631 * @retval None 00632 */ 00633 __STATIC_INLINE void LL_LPTIM_DisableTimeout(LPTIM_TypeDef * LPTIMx) 00634 { 00635 CLEAR_BIT(LPTIMx->CFGR, LPTIM_CFGR_TIMOUT); 00636 } 00637 00638 /** 00639 * @brief Indicate whether the timeout function is enabled. 00640 * @rmtoll CFGR TIMOUT LL_LPTIM_IsEnabledTimeout 00641 * @param LPTIMx Low-Power Timer instance 00642 * @retval State of bit (1 or 0). 00643 */ 00644 __STATIC_INLINE uint32_t LL_LPTIM_IsEnabledTimeout(LPTIM_TypeDef * LPTIMx) 00645 { 00646 return (READ_BIT(LPTIMx->CFGR, LPTIM_CFGR_TIMOUT) == (LPTIM_CFGR_TIMOUT)); 00647 } 00648 00649 /** 00650 * @brief Start the LPTIM counter 00651 * @note This function must be called when the LPTIM instance is disabled. 00652 * @rmtoll CFGR TRIGEN LL_LPTIM_TrigSw 00653 * @param LPTIMx Low-Power Timer instance 00654 * @retval None 00655 */ 00656 __STATIC_INLINE void LL_LPTIM_TrigSw(LPTIM_TypeDef * LPTIMx) 00657 { 00658 CLEAR_BIT(LPTIMx->CFGR, LPTIM_CFGR_TRIGEN); 00659 } 00660 00661 /** 00662 * @brief Configure the external trigger used as a trigger event for the LPTIM. 00663 * @note This function must be called when the LPTIM instance is disabled. 00664 * @note An internal clock source must be present when a digital filter is 00665 * required for the trigger. 00666 * @rmtoll CFGR TRIGSEL LL_LPTIM_ConfigTrigger\n 00667 * CFGR TRGFLT LL_LPTIM_ConfigTrigger\n 00668 * CFGR TRIGEN LL_LPTIM_ConfigTrigger 00669 * @param LPTIMx Low-Power Timer instance 00670 * @param Source This parameter can be one of the following values: 00671 * @arg @ref LL_LPTIM_TRIG_SOURCE_GPIO 00672 * @arg @ref LL_LPTIM_TRIG_SOURCE_RTCALARMA 00673 * @arg @ref LL_LPTIM_TRIG_SOURCE_RTCALARMB 00674 * @arg @ref LL_LPTIM_TRIG_SOURCE_RTCTAMP1 00675 * @arg @ref LL_LPTIM_TRIG_SOURCE_RTCTAMP2 00676 * @arg @ref LL_LPTIM_TRIG_SOURCE_RTCTAMP3 00677 * @arg @ref LL_LPTIM_TRIG_SOURCE_COMP1 00678 * @arg @ref LL_LPTIM_TRIG_SOURCE_COMP2 00679 * @param Filter This parameter can be one of the following values: 00680 * @arg @ref LL_LPTIM_TRIG_FILTER_NONE 00681 * @arg @ref LL_LPTIM_TRIG_FILTER_2 00682 * @arg @ref LL_LPTIM_TRIG_FILTER_4 00683 * @arg @ref LL_LPTIM_TRIG_FILTER_8 00684 * @param Polarity This parameter can be one of the following values: 00685 * @arg @ref LL_LPTIM_TRIG_POLARITY_RISING 00686 * @arg @ref LL_LPTIM_TRIG_POLARITY_FALLING 00687 * @arg @ref LL_LPTIM_TRIG_POLARITY_RISING_FALLING 00688 * @retval None 00689 */ 00690 __STATIC_INLINE void LL_LPTIM_ConfigTrigger(LPTIM_TypeDef * LPTIMx, uint32_t Source, uint32_t Filter, uint32_t Polarity) 00691 { 00692 MODIFY_REG(LPTIMx->CFGR, LPTIM_CFGR_TRIGSEL | LPTIM_CFGR_TRGFLT | LPTIM_CFGR_TRIGEN, Source | Filter | Polarity); 00693 } 00694 00695 /** 00696 * @brief Get actual external trigger source. 00697 * @rmtoll CFGR TRIGSEL LL_LPTIM_GetTriggerSource 00698 * @param LPTIMx Low-Power Timer instance 00699 * @retval Returned value can be one of the following values: 00700 * @arg @ref LL_LPTIM_TRIG_SOURCE_GPIO 00701 * @arg @ref LL_LPTIM_TRIG_SOURCE_RTCALARMA 00702 * @arg @ref LL_LPTIM_TRIG_SOURCE_RTCALARMB 00703 * @arg @ref LL_LPTIM_TRIG_SOURCE_RTCTAMP1 00704 * @arg @ref LL_LPTIM_TRIG_SOURCE_RTCTAMP2 00705 * @arg @ref LL_LPTIM_TRIG_SOURCE_RTCTAMP3 00706 * @arg @ref LL_LPTIM_TRIG_SOURCE_COMP1 00707 * @arg @ref LL_LPTIM_TRIG_SOURCE_COMP2 00708 */ 00709 __STATIC_INLINE uint32_t LL_LPTIM_GetTriggerSource(LPTIM_TypeDef * LPTIMx) 00710 { 00711 return (uint32_t)(READ_BIT(LPTIMx->CFGR, LPTIM_CFGR_TRIGSEL)); 00712 } 00713 00714 /** 00715 * @brief Get actual external trigger filter. 00716 * @rmtoll CFGR TRGFLT LL_LPTIM_GetTriggerFilter 00717 * @param LPTIMx Low-Power Timer instance 00718 * @retval Returned value can be one of the following values: 00719 * @arg @ref LL_LPTIM_TRIG_FILTER_NONE 00720 * @arg @ref LL_LPTIM_TRIG_FILTER_2 00721 * @arg @ref LL_LPTIM_TRIG_FILTER_4 00722 * @arg @ref LL_LPTIM_TRIG_FILTER_8 00723 */ 00724 __STATIC_INLINE uint32_t LL_LPTIM_GetTriggerFilter(LPTIM_TypeDef * LPTIMx) 00725 { 00726 return (uint32_t)(READ_BIT(LPTIMx->CFGR, LPTIM_CFGR_TRGFLT)); 00727 } 00728 00729 /** 00730 * @brief Get actual external trigger polarity. 00731 * @rmtoll CFGR TRIGEN LL_LPTIM_GetTriggerPolarity 00732 * @param LPTIMx Low-Power Timer instance 00733 * @retval Returned value can be one of the following values: 00734 * @arg @ref LL_LPTIM_TRIG_POLARITY_RISING 00735 * @arg @ref LL_LPTIM_TRIG_POLARITY_FALLING 00736 * @arg @ref LL_LPTIM_TRIG_POLARITY_RISING_FALLING 00737 */ 00738 __STATIC_INLINE uint32_t LL_LPTIM_GetTriggerPolarity(LPTIM_TypeDef * LPTIMx) 00739 { 00740 return (uint32_t)(READ_BIT(LPTIMx->CFGR, LPTIM_CFGR_TRIGEN)); 00741 } 00742 00743 /** 00744 * @} 00745 */ 00746 00747 /** @defgroup LPTIM_LL_EF_Clock_Configuration Clock_Configuration 00748 * @{ 00749 */ 00750 00751 /** 00752 * @brief Set the source of the clock used by the LPTIM instance. 00753 * @note This function must be called when the LPTIM instance is disabled. 00754 * @rmtoll CFGR CKSEL LL_LPTIM_SetClockSource 00755 * @param LPTIMx Low-Power Timer instance 00756 * @param ClockSource This parameter can be one of the following values: 00757 * @arg @ref LL_LPTIM_CLK_SOURCE_INTERNAL 00758 * @arg @ref LL_LPTIM_CLK_SOURCE_EXTERNAL 00759 * @retval None 00760 */ 00761 __STATIC_INLINE void LL_LPTIM_SetClockSource(LPTIM_TypeDef * LPTIMx, uint32_t ClockSource) 00762 { 00763 MODIFY_REG(LPTIMx->CFGR, LPTIM_CFGR_CKSEL, ClockSource); 00764 } 00765 00766 /** 00767 * @brief Get actual LPTIM instance clock source. 00768 * @rmtoll CFGR CKSEL LL_LPTIM_GetClockSource 00769 * @param LPTIMx Low-Power Timer instance 00770 * @retval Returned value can be one of the following values: 00771 * @arg @ref LL_LPTIM_CLK_SOURCE_INTERNAL 00772 * @arg @ref LL_LPTIM_CLK_SOURCE_EXTERNAL 00773 */ 00774 __STATIC_INLINE uint32_t LL_LPTIM_GetClockSource(LPTIM_TypeDef * LPTIMx) 00775 { 00776 return (uint32_t)(READ_BIT(LPTIMx->CFGR, LPTIM_CFGR_CKSEL)); 00777 } 00778 00779 /** 00780 * @brief Configure the active edge or edges used by the counter when the LPTIM is clocked by an external clock source. 00781 * @note This function must be called when the LPTIM instance is disabled. 00782 * @note When both external clock signal edges are considered active ones, 00783 * the LPTIM must also be clocked by an internal clock source with a 00784 * frequency equal to at least four times the external clock frequency. 00785 * @note An internal clock source must be present when a digital filter is 00786 * required for external clock. 00787 * @rmtoll CFGR CKFLT LL_LPTIM_ConfigClock\n 00788 * CFGR CKPOL LL_LPTIM_ConfigClock 00789 * @param LPTIMx Low-Power Timer instance 00790 * @param ClockFilter This parameter can be one of the following values: 00791 * @arg @ref LL_LPTIM_CLK_FILTER_NONE 00792 * @arg @ref LL_LPTIM_CLK_FILTER_2 00793 * @arg @ref LL_LPTIM_CLK_FILTER_4 00794 * @arg @ref LL_LPTIM_CLK_FILTER_8 00795 * @param ClockPolarity This parameter can be one of the following values: 00796 * @arg @ref LL_LPTIM_CLK_POLARITY_RISING 00797 * @arg @ref LL_LPTIM_CLK_POLARITY_FALLING 00798 * @arg @ref LL_LPTIM_CLK_POLARITY_RISING_FALLING 00799 * @retval None 00800 */ 00801 __STATIC_INLINE void LL_LPTIM_ConfigClock(LPTIM_TypeDef * LPTIMx, uint32_t ClockFilter, uint32_t ClockPolarity) 00802 { 00803 MODIFY_REG(LPTIMx->CFGR, LPTIM_CFGR_CKFLT | LPTIM_CFGR_CKPOL, ClockFilter | ClockPolarity); 00804 } 00805 00806 /** 00807 * @brief Get actual clock polarity 00808 * @rmtoll CFGR CKPOL LL_LPTIM_GetClockPolarity 00809 * @param LPTIMx Low-Power Timer instance 00810 * @retval Returned value can be one of the following values: 00811 * @arg @ref LL_LPTIM_CLK_POLARITY_RISING 00812 * @arg @ref LL_LPTIM_CLK_POLARITY_FALLING 00813 * @arg @ref LL_LPTIM_CLK_POLARITY_RISING_FALLING 00814 */ 00815 __STATIC_INLINE uint32_t LL_LPTIM_GetClockPolarity(LPTIM_TypeDef * LPTIMx) 00816 { 00817 return (uint32_t)(READ_BIT(LPTIMx->CFGR, LPTIM_CFGR_CKPOL)); 00818 } 00819 00820 /** 00821 * @brief Get actual clock digital filter 00822 * @rmtoll CFGR CKFLT LL_LPTIM_GetClockFilter 00823 * @param LPTIMx Low-Power Timer instance 00824 * @retval Returned value can be one of the following values: 00825 * @arg @ref LL_LPTIM_CLK_FILTER_NONE 00826 * @arg @ref LL_LPTIM_CLK_FILTER_2 00827 * @arg @ref LL_LPTIM_CLK_FILTER_4 00828 * @arg @ref LL_LPTIM_CLK_FILTER_8 00829 */ 00830 __STATIC_INLINE uint32_t LL_LPTIM_GetClockFilter(LPTIM_TypeDef * LPTIMx) 00831 { 00832 return (uint32_t)(READ_BIT(LPTIMx->CFGR, LPTIM_CFGR_CKFLT)); 00833 } 00834 00835 /** 00836 * @} 00837 */ 00838 00839 /** @defgroup LPTIM_LL_EF_Encoder_Mode Encoder_Mode 00840 * @{ 00841 */ 00842 00843 /** 00844 * @brief Configure the encoder mode. 00845 * @note This function must be called when the LPTIM instance is disabled. 00846 * @rmtoll CFGR CKPOL LL_LPTIM_SetEncoderMode 00847 * @param LPTIMx Low-Power Timer instance 00848 * @param EncoderMode This parameter can be one of the following values: 00849 * @arg @ref LL_LPTIM_ENCODER_MODE_RISING 00850 * @arg @ref LL_LPTIM_ENCODER_MODE_FALLING 00851 * @arg @ref LL_LPTIM_ENCODER_MODE_RISING_FALLING 00852 * @retval None 00853 */ 00854 __STATIC_INLINE void LL_LPTIM_SetEncoderMode(LPTIM_TypeDef * LPTIMx, uint32_t EncoderMode) 00855 { 00856 MODIFY_REG(LPTIMx->CFGR, LPTIM_CFGR_CKPOL, EncoderMode); 00857 } 00858 00859 /** 00860 * @brief Get actual encoder mode. 00861 * @rmtoll CFGR CKPOL LL_LPTIM_GetEncoderMode 00862 * @param LPTIMx Low-Power Timer instance 00863 * @retval Returned value can be one of the following values: 00864 * @arg @ref LL_LPTIM_ENCODER_MODE_RISING 00865 * @arg @ref LL_LPTIM_ENCODER_MODE_FALLING 00866 * @arg @ref LL_LPTIM_ENCODER_MODE_RISING_FALLING 00867 */ 00868 __STATIC_INLINE uint32_t LL_LPTIM_GetEncoderMode(LPTIM_TypeDef * LPTIMx) 00869 { 00870 return (uint32_t)(READ_BIT(LPTIMx->CFGR, LPTIM_CFGR_CKPOL)); 00871 } 00872 00873 /** 00874 * @brief Enable the encoder mode 00875 * @note This function must be called when the LPTIM instance is disabled. 00876 * @note In this mode the LPTIM instance must be clocked by an internal clock 00877 * source. Also, the prescaler division ratio must be equal to 1. 00878 * @note LPTIM instance must be configured in continuous mode prior enabling 00879 * the encoder mode. 00880 * @rmtoll CFGR ENC LL_LPTIM_EnableEncoderMode 00881 * @param LPTIMx Low-Power Timer instance 00882 * @retval None 00883 */ 00884 __STATIC_INLINE void LL_LPTIM_EnableEncoderMode(LPTIM_TypeDef * LPTIMx) 00885 { 00886 SET_BIT(LPTIMx->CFGR, LPTIM_CFGR_ENC); 00887 } 00888 00889 /** 00890 * @brief Disable the encoder mode 00891 * @note This function must be called when the LPTIM instance is disabled. 00892 * @rmtoll CFGR ENC LL_LPTIM_DisableEncoderMode 00893 * @param LPTIMx Low-Power Timer instance 00894 * @retval None 00895 */ 00896 __STATIC_INLINE void LL_LPTIM_DisableEncoderMode(LPTIM_TypeDef * LPTIMx) 00897 { 00898 CLEAR_BIT(LPTIMx->CFGR, LPTIM_CFGR_ENC); 00899 } 00900 00901 /** 00902 * @brief Indicates whether the LPTIM operates in encoder mode. 00903 * @rmtoll CFGR ENC LL_LPTIM_IsEnabledEncoderMode 00904 * @param LPTIMx Low-Power Timer instance 00905 * @retval State of bit (1 or 0). 00906 */ 00907 __STATIC_INLINE uint32_t LL_LPTIM_IsEnabledEncoderMode(LPTIM_TypeDef * LPTIMx) 00908 { 00909 return (READ_BIT(LPTIMx->CFGR, LPTIM_CFGR_ENC) == (LPTIM_CFGR_ENC)); 00910 } 00911 00912 /** 00913 * @} 00914 */ 00915 00916 /** @defgroup LPTIM_LL_EF_FLAG_Management FLAG_Management 00917 * @{ 00918 */ 00919 00920 /** 00921 * @brief Clear the compare match flag (CMPMCF) 00922 * @rmtoll ICR CMPMCF LL_LPTIM_ClearFLAG_CMPM 00923 * @param LPTIMx Low-Power Timer instance 00924 * @retval None 00925 */ 00926 __STATIC_INLINE void LL_LPTIM_ClearFLAG_CMPM(LPTIM_TypeDef * LPTIMx) 00927 { 00928 SET_BIT(LPTIMx->ICR, LPTIM_ICR_CMPMCF); 00929 } 00930 00931 /** 00932 * @brief Inform application whether a compare match interrupt has occurred. 00933 * @rmtoll ISR CMPM LL_LPTIM_IsActiveFlag_CMPM 00934 * @param LPTIMx Low-Power Timer instance 00935 * @retval State of bit (1 or 0). 00936 */ 00937 __STATIC_INLINE uint32_t LL_LPTIM_IsActiveFlag_CMPM(LPTIM_TypeDef * LPTIMx) 00938 { 00939 return (READ_BIT(LPTIMx->ISR, LPTIM_ISR_CMPM) == (LPTIM_ISR_CMPM)); 00940 } 00941 00942 /** 00943 * @brief Clear the autoreload match flag (ARRMCF) 00944 * @rmtoll ICR ARRMCF LL_LPTIM_ClearFLAG_ARRM 00945 * @param LPTIMx Low-Power Timer instance 00946 * @retval None 00947 */ 00948 __STATIC_INLINE void LL_LPTIM_ClearFLAG_ARRM(LPTIM_TypeDef * LPTIMx) 00949 { 00950 SET_BIT(LPTIMx->ICR, LPTIM_ICR_ARRMCF); 00951 } 00952 00953 /** 00954 * @brief Inform application whether a autoreload match interrupt has occured. 00955 * @rmtoll ISR ARRM LL_LPTIM_IsActiveFlag_ARRM 00956 * @param LPTIMx Low-Power Timer instance 00957 * @retval State of bit (1 or 0). 00958 */ 00959 __STATIC_INLINE uint32_t LL_LPTIM_IsActiveFlag_ARRM(LPTIM_TypeDef * LPTIMx) 00960 { 00961 return (READ_BIT(LPTIMx->ISR, LPTIM_ISR_ARRM) == (LPTIM_ISR_ARRM)); 00962 } 00963 00964 /** 00965 * @brief Clear the external trigger valid edge flag(EXTTRIGCF). 00966 * @rmtoll ICR EXTTRIGCF LL_LPTIM_ClearFlag_EXTTRIG 00967 * @param LPTIMx Low-Power Timer instance 00968 * @retval None 00969 */ 00970 __STATIC_INLINE void LL_LPTIM_ClearFlag_EXTTRIG(LPTIM_TypeDef * LPTIMx) 00971 { 00972 SET_BIT(LPTIMx->ICR, LPTIM_ICR_EXTTRIGCF); 00973 } 00974 00975 /** 00976 * @brief Inform application whether a valid edge on the selected external trigger input has occurred. 00977 * @rmtoll ISR EXTTRIG LL_LPTIM_IsActiveFlag_EXTTRIG 00978 * @param LPTIMx Low-Power Timer instance 00979 * @retval State of bit (1 or 0). 00980 */ 00981 __STATIC_INLINE uint32_t LL_LPTIM_IsActiveFlag_EXTTRIG(LPTIM_TypeDef * LPTIMx) 00982 { 00983 return (READ_BIT(LPTIMx->ISR, LPTIM_ISR_EXTTRIG) == (LPTIM_ISR_EXTTRIG)); 00984 } 00985 00986 /** 00987 * @brief Clear the compare register update interrupt flag (CMPOKCF). 00988 * @rmtoll ICR CMPOKCF LL_LPTIM_ClearFlag_CMPOK 00989 * @param LPTIMx Low-Power Timer instance 00990 * @retval None 00991 */ 00992 __STATIC_INLINE void LL_LPTIM_ClearFlag_CMPOK(LPTIM_TypeDef * LPTIMx) 00993 { 00994 SET_BIT(LPTIMx->ICR, LPTIM_ICR_CMPOKCF); 00995 } 00996 00997 /** 00998 * @brief Informs application whether the APB bus write operation to the LPTIMx_CMP register has been successfully completed. If so, a new one can be initiated. 00999 * @rmtoll ISR CMPOK LL_LPTIM_IsActiveFlag_CMPOK 01000 * @param LPTIMx Low-Power Timer instance 01001 * @retval State of bit (1 or 0). 01002 */ 01003 __STATIC_INLINE uint32_t LL_LPTIM_IsActiveFlag_CMPOK(LPTIM_TypeDef * LPTIMx) 01004 { 01005 return (READ_BIT(LPTIMx->ISR, LPTIM_ISR_CMPOK) == (LPTIM_ISR_CMPOK)); 01006 } 01007 01008 /** 01009 * @brief Clear the autoreload register update interrupt flag (ARROKCF). 01010 * @rmtoll ICR ARROKCF LL_LPTIM_ClearFlag_ARROK 01011 * @param LPTIMx Low-Power Timer instance 01012 * @retval None 01013 */ 01014 __STATIC_INLINE void LL_LPTIM_ClearFlag_ARROK(LPTIM_TypeDef * LPTIMx) 01015 { 01016 SET_BIT(LPTIMx->ICR, LPTIM_ICR_ARROKCF); 01017 } 01018 01019 /** 01020 * @brief Informs application whether the APB bus write operation to the LPTIMx_ARR register has been successfully completed. If so, a new one can be initiated. 01021 * @rmtoll ISR ARROK LL_LPTIM_IsActiveFlag_ARROK 01022 * @param LPTIMx Low-Power Timer instance 01023 * @retval State of bit (1 or 0). 01024 */ 01025 __STATIC_INLINE uint32_t LL_LPTIM_IsActiveFlag_ARROK(LPTIM_TypeDef * LPTIMx) 01026 { 01027 return (READ_BIT(LPTIMx->ISR, LPTIM_ISR_ARROK) == (LPTIM_ISR_ARROK)); 01028 } 01029 01030 /** 01031 * @brief Clear the counter direction change to up interrupt flag (UPCF). 01032 * @rmtoll ICR UPCF LL_LPTIM_ClearFlag_UP 01033 * @param LPTIMx Low-Power Timer instance 01034 * @retval None 01035 */ 01036 __STATIC_INLINE void LL_LPTIM_ClearFlag_UP(LPTIM_TypeDef * LPTIMx) 01037 { 01038 SET_BIT(LPTIMx->ICR, LPTIM_ICR_UPCF); 01039 } 01040 01041 /** 01042 * @brief Informs the application whether the counter direction has changed from down to up (when the LPTIM instance operates in encoder mode). 01043 * @rmtoll ISR UP LL_LPTIM_IsActiveFlag_UP 01044 * @param LPTIMx Low-Power Timer instance 01045 * @retval State of bit (1 or 0). 01046 */ 01047 __STATIC_INLINE uint32_t LL_LPTIM_IsActiveFlag_UP(LPTIM_TypeDef * LPTIMx) 01048 { 01049 return (READ_BIT(LPTIMx->ISR, LPTIM_ISR_UP) == (LPTIM_ISR_UP)); 01050 } 01051 01052 /** 01053 * @brief Clear the counter direction change to down interrupt flag (DOWNCF). 01054 * @rmtoll ICR DOWNCF LL_LPTIM_ClearFlag_DOWN 01055 * @param LPTIMx Low-Power Timer instance 01056 * @retval None 01057 */ 01058 __STATIC_INLINE void LL_LPTIM_ClearFlag_DOWN(LPTIM_TypeDef * LPTIMx) 01059 { 01060 SET_BIT(LPTIMx->ICR, LPTIM_ICR_DOWNCF); 01061 } 01062 01063 /** 01064 * @brief Informs the application whether the counter direction has changed from up to down (when the LPTIM instance operates in encoder mode). 01065 * @rmtoll ISR DOWN LL_LPTIM_IsActiveFlag_DOWN 01066 * @param LPTIMx Low-Power Timer instance 01067 * @retval State of bit (1 or 0). 01068 */ 01069 __STATIC_INLINE uint32_t LL_LPTIM_IsActiveFlag_DOWN(LPTIM_TypeDef * LPTIMx) 01070 { 01071 return (READ_BIT(LPTIMx->ISR, LPTIM_ISR_DOWN) == (LPTIM_ISR_DOWN)); 01072 } 01073 01074 /** 01075 * @} 01076 */ 01077 01078 /** @defgroup LPTIM_LL_EF_IT_Management IT_Management 01079 * @{ 01080 */ 01081 01082 /** 01083 * @brief Enable compare match interrupt (CMPMIE). 01084 * @rmtoll IER CMPMIE LL_LPTIM_EnableIT_CMPM 01085 * @param LPTIMx Low-Power Timer instance 01086 * @retval None 01087 */ 01088 __STATIC_INLINE void LL_LPTIM_EnableIT_CMPM(LPTIM_TypeDef * LPTIMx) 01089 { 01090 SET_BIT(LPTIMx->IER, LPTIM_IER_CMPMIE); 01091 } 01092 01093 /** 01094 * @brief Disable compare match interrupt (CMPMIE). 01095 * @rmtoll IER CMPMIE LL_LPTIM_DisableIT_CMPM 01096 * @param LPTIMx Low-Power Timer instance 01097 * @retval None 01098 */ 01099 __STATIC_INLINE void LL_LPTIM_DisableIT_CMPM(LPTIM_TypeDef * LPTIMx) 01100 { 01101 CLEAR_BIT(LPTIMx->IER, LPTIM_IER_CMPMIE); 01102 } 01103 01104 /** 01105 * @brief Indicates whether the compare match interrupt (CMPMIE) is enabled. 01106 * @rmtoll IER CMPMIE LL_LPTIM_IsEnabledIT_CMPM 01107 * @param LPTIMx Low-Power Timer instance 01108 * @retval State of bit (1 or 0). 01109 */ 01110 __STATIC_INLINE uint32_t LL_LPTIM_IsEnabledIT_CMPM(LPTIM_TypeDef * LPTIMx) 01111 { 01112 return (READ_BIT(LPTIMx->IER, LPTIM_IER_CMPMIE) == (LPTIM_IER_CMPMIE)); 01113 } 01114 01115 /** 01116 * @brief Enable autoreload match interrupt (ARRMIE). 01117 * @rmtoll IER ARRMIE LL_LPTIM_EnableIT_ARRM 01118 * @param LPTIMx Low-Power Timer instance 01119 * @retval None 01120 */ 01121 __STATIC_INLINE void LL_LPTIM_EnableIT_ARRM(LPTIM_TypeDef * LPTIMx) 01122 { 01123 SET_BIT(LPTIMx->IER, LPTIM_IER_ARRMIE); 01124 } 01125 01126 /** 01127 * @brief Disable autoreload match interrupt (ARRMIE). 01128 * @rmtoll IER ARRMIE LL_LPTIM_DisableIT_ARRM 01129 * @param LPTIMx Low-Power Timer instance 01130 * @retval None 01131 */ 01132 __STATIC_INLINE void LL_LPTIM_DisableIT_ARRM(LPTIM_TypeDef * LPTIMx) 01133 { 01134 CLEAR_BIT(LPTIMx->IER, LPTIM_IER_ARRMIE); 01135 } 01136 01137 /** 01138 * @brief Indicates whether the autoreload match interrupt (ARRMIE) is enabled. 01139 * @rmtoll IER ARRMIE LL_LPTIM_IsEnabledIT_ARRM 01140 * @param LPTIMx Low-Power Timer instance 01141 * @retval State of bit (1 or 0). 01142 */ 01143 __STATIC_INLINE uint32_t LL_LPTIM_IsEnabledIT_ARRM(LPTIM_TypeDef * LPTIMx) 01144 { 01145 return (READ_BIT(LPTIMx->IER, LPTIM_IER_ARRMIE) == (LPTIM_IER_ARRMIE)); 01146 } 01147 01148 /** 01149 * @brief Enable external trigger valid edge interrupt (EXTTRIGIE). 01150 * @rmtoll IER EXTTRIGIE LL_LPTIM_EnableIT_EXTTRIG 01151 * @param LPTIMx Low-Power Timer instance 01152 * @retval None 01153 */ 01154 __STATIC_INLINE void LL_LPTIM_EnableIT_EXTTRIG(LPTIM_TypeDef * LPTIMx) 01155 { 01156 SET_BIT(LPTIMx->IER, LPTIM_IER_EXTTRIGIE); 01157 } 01158 01159 /** 01160 * @brief Disable external trigger valid edge interrupt (EXTTRIGIE). 01161 * @rmtoll IER EXTTRIGIE LL_LPTIM_DisableIT_EXTTRIG 01162 * @param LPTIMx Low-Power Timer instance 01163 * @retval None 01164 */ 01165 __STATIC_INLINE void LL_LPTIM_DisableIT_EXTTRIG(LPTIM_TypeDef * LPTIMx) 01166 { 01167 CLEAR_BIT(LPTIMx->IER, LPTIM_IER_EXTTRIGIE); 01168 } 01169 01170 /** 01171 * @brief Indicates external trigger valid edge interrupt (EXTTRIGIE) is enabled. 01172 * @rmtoll IER EXTTRIGIE LL_LPTIM_IsEnabledIT_EXTTRIG 01173 * @param LPTIMx Low-Power Timer instance 01174 * @retval State of bit (1 or 0). 01175 */ 01176 __STATIC_INLINE uint32_t LL_LPTIM_IsEnabledIT_EXTTRIG(LPTIM_TypeDef * LPTIMx) 01177 { 01178 return (READ_BIT(LPTIMx->IER, LPTIM_IER_EXTTRIGIE) == (LPTIM_IER_EXTTRIGIE)); 01179 } 01180 01181 /** 01182 * @brief Enable compare register write completed interrupt (CMPOKIE). 01183 * @rmtoll IER CMPOKIE LL_LPTIM_EnableIT_CMPOK 01184 * @param LPTIMx Low-Power Timer instance 01185 * @retval None 01186 */ 01187 __STATIC_INLINE void LL_LPTIM_EnableIT_CMPOK(LPTIM_TypeDef * LPTIMx) 01188 { 01189 SET_BIT(LPTIMx->IER, LPTIM_IER_CMPOKIE); 01190 } 01191 01192 /** 01193 * @brief Disable compare register write completed interrupt (CMPOKIE). 01194 * @rmtoll IER CMPOKIE LL_LPTIM_DisableIT_CMPOK 01195 * @param LPTIMx Low-Power Timer instance 01196 * @retval None 01197 */ 01198 __STATIC_INLINE void LL_LPTIM_DisableIT_CMPOK(LPTIM_TypeDef * LPTIMx) 01199 { 01200 CLEAR_BIT(LPTIMx->IER, LPTIM_IER_CMPOKIE); 01201 } 01202 01203 /** 01204 * @brief Indicates whether the compare register write completed interrupt (CMPOKIE) is enabled. 01205 * @rmtoll IER CMPOKIE LL_LPTIM_IsEnabledIT_CMPOK 01206 * @param LPTIMx Low-Power Timer instance 01207 * @retval State of bit (1 or 0). 01208 */ 01209 __STATIC_INLINE uint32_t LL_LPTIM_IsEnabledIT_CMPOK(LPTIM_TypeDef * LPTIMx) 01210 { 01211 return (READ_BIT(LPTIMx->IER, LPTIM_IER_CMPOKIE) == (LPTIM_IER_CMPOKIE)); 01212 } 01213 01214 /** 01215 * @brief Enable autoreload register write completed interrupt (ARROKIE). 01216 * @rmtoll IER ARROKIE LL_LPTIM_EnableIT_ARROK 01217 * @param LPTIMx Low-Power Timer instance 01218 * @retval None 01219 */ 01220 __STATIC_INLINE void LL_LPTIM_EnableIT_ARROK(LPTIM_TypeDef * LPTIMx) 01221 { 01222 SET_BIT(LPTIMx->IER, LPTIM_IER_ARROKIE); 01223 } 01224 01225 /** 01226 * @brief Disable autoreload register write completed interrupt (ARROKIE). 01227 * @rmtoll IER ARROKIE LL_LPTIM_DisableIT_ARROK 01228 * @param LPTIMx Low-Power Timer instance 01229 * @retval None 01230 */ 01231 __STATIC_INLINE void LL_LPTIM_DisableIT_ARROK(LPTIM_TypeDef * LPTIMx) 01232 { 01233 CLEAR_BIT(LPTIMx->IER, LPTIM_IER_ARROKIE); 01234 } 01235 01236 /** 01237 * @brief Indicates whether the autoreload register write completed interrupt (ARROKIE) is enabled. 01238 * @rmtoll IER ARROKIE LL_LPTIM_IsEnabledIT_ARROK 01239 * @param LPTIMx Low-Power Timer instance 01240 * @retval State of bit (1 or 0). 01241 */ 01242 __STATIC_INLINE uint32_t LL_LPTIM_IsEnabledIT_ARROK(LPTIM_TypeDef * LPTIMx) 01243 { 01244 return (READ_BIT(LPTIMx->IER, LPTIM_IER_ARROKIE) == (LPTIM_IER_ARROKIE)); 01245 } 01246 01247 /** 01248 * @brief Enable direction change to up interrupt (UPIE). 01249 * @rmtoll IER UPIE LL_LPTIM_EnableIT_UP 01250 * @param LPTIMx Low-Power Timer instance 01251 * @retval None 01252 */ 01253 __STATIC_INLINE void LL_LPTIM_EnableIT_UP(LPTIM_TypeDef * LPTIMx) 01254 { 01255 SET_BIT(LPTIMx->IER, LPTIM_IER_UPIE); 01256 } 01257 01258 /** 01259 * @brief Disable direction change to up interrupt (UPIE). 01260 * @rmtoll IER UPIE LL_LPTIM_DisableIT_UP 01261 * @param LPTIMx Low-Power Timer instance 01262 * @retval None 01263 */ 01264 __STATIC_INLINE void LL_LPTIM_DisableIT_UP(LPTIM_TypeDef * LPTIMx) 01265 { 01266 CLEAR_BIT(LPTIMx->IER, LPTIM_IER_UPIE); 01267 } 01268 01269 /** 01270 * @brief Indicates whether the direction change to up interrupt (UPIE) is enabled. 01271 * @rmtoll IER UPIE LL_LPTIM_IsEnabledIT_UP 01272 * @param LPTIMx Low-Power Timer instance 01273 * @retval State of bit (1 or 0). 01274 */ 01275 __STATIC_INLINE uint32_t LL_LPTIM_IsEnabledIT_UP(LPTIM_TypeDef * LPTIMx) 01276 { 01277 return (READ_BIT(LPTIMx->IER, LPTIM_IER_UPIE) == (LPTIM_IER_UPIE)); 01278 } 01279 01280 /** 01281 * @brief Enable direction change to down interrupt (DOWNIE). 01282 * @rmtoll IER DOWNIE LL_LPTIM_EnableIT_DOWN 01283 * @param LPTIMx Low-Power Timer instance 01284 * @retval None 01285 */ 01286 __STATIC_INLINE void LL_LPTIM_EnableIT_DOWN(LPTIM_TypeDef * LPTIMx) 01287 { 01288 SET_BIT(LPTIMx->IER, LPTIM_IER_DOWNIE); 01289 } 01290 01291 /** 01292 * @brief Disable direction change to down interrupt (DOWNIE). 01293 * @rmtoll IER DOWNIE LL_LPTIM_DisableIT_DOWN 01294 * @param LPTIMx Low-Power Timer instance 01295 * @retval None 01296 */ 01297 __STATIC_INLINE void LL_LPTIM_DisableIT_DOWN(LPTIM_TypeDef * LPTIMx) 01298 { 01299 CLEAR_BIT(LPTIMx->IER, LPTIM_IER_DOWNIE); 01300 } 01301 01302 /** 01303 * @brief Indicates whether the direction change to down interrupt (DOWNIE) is enabled. 01304 * @rmtoll IER DOWNIE LL_LPTIM_IsEnabledIT_DOWN 01305 * @param LPTIMx Low-Power Timer instance 01306 * @retval State of bit (1 or 0). 01307 */ 01308 __STATIC_INLINE uint32_t LL_LPTIM_IsEnabledIT_DOWN(LPTIM_TypeDef * LPTIMx) 01309 { 01310 return (READ_BIT(LPTIMx->IER, LPTIM_IER_DOWNIE) == (LPTIM_IER_DOWNIE)); 01311 } 01312 01313 /** 01314 * @} 01315 */ 01316 01317 01318 /** 01319 * @} 01320 */ 01321 01322 /** 01323 * @} 01324 */ 01325 01326 #endif /* defined (LPTIM1) || defined (LPTIM2) */ 01327 01328 /** 01329 * @} 01330 */ 01331 01332 #ifdef __cplusplus 01333 } 01334 #endif 01335 01336 #endif /* __STM32L4xx_LL_LPTIM_H */ 01337 01338 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 01339
Generated on Tue Jul 12 2022 11:35:16 by
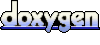